How Can I Learn to Read JavaScript Effectively?
Introduction
In the ever-evolving landscape of web development, JavaScript stands as a cornerstone technology, breathing life into static web pages and transforming them into dynamic, interactive experiences. As the language of the web, understanding how to read JavaScript is not just a skill—it’s a gateway to unlocking the full potential of modern programming. Whether you’re a budding developer, a curious hobbyist, or someone looking to enhance your technical proficiency, mastering the art of reading JavaScript can empower you to navigate the complexities of coding with confidence and creativity.
Reading JavaScript involves more than just deciphering lines of code; it’s about grasping the underlying logic and structure that drive functionality. From understanding variables and functions to grasping control flow and event handling, each element plays a crucial role in how a web application operates. As you delve deeper into JavaScript, you’ll discover the nuances of syntax, the significance of various data types, and the importance of best practices that can elevate your coding skills.
Moreover, the journey of learning how to read JavaScript is enriched by the vast ecosystem surrounding it. With frameworks and libraries like React, Angular, and Vue.js, JavaScript has expanded its reach, offering developers innovative tools to create seamless user experiences. By immersing yourself in the world of
Understanding JavaScript Syntax
JavaScript syntax consists of the set of rules that define a correctly structured JavaScript program. Familiarizing yourself with basic syntax is critical for reading and writing JavaScript effectively.
Key components of JavaScript syntax include:
– **Variables**: Declared using `var`, `let`, or `const`.
– **Operators**: Such as arithmetic (`+`, `-`, `*`, `/`) and logical operators (`&&`, `||`, `!`).
– **Control Structures**: Including `if`, `else`, `switch`, and loops (`for`, `while`).
– **Functions**: Defined using the `function` keyword or arrow function syntax.
Here’s a simple example of JavaScript syntax:
javascript
let x = 10; // Variable declaration
if (x > 5) { // Control structure
console.log(“x is greater than 5”); // Output
}
Data Types in JavaScript
JavaScript is a dynamically typed language, which means that variables can hold any type of data. Understanding the various data types is essential for reading JavaScript code.
The main data types include:
- Primitive Types:
- Number
- String
- Boolean
- Null
- Undefined
- Symbol (ES6)
- Reference Types:
- Object
- Array
- Function
Here’s a table that summarizes the data types:
Data Type | Description |
---|---|
Number | Represents both integer and floating-point numbers. |
String | A sequence of characters enclosed in quotes. |
Boolean | Represents a true or value. |
Null | A special keyword denoting a null value. |
Undefined | A variable that has been declared but not assigned a value. |
Object | A collection of key-value pairs. |
Array | An ordered list of values. |
Function | A block of code designed to perform a particular task. |
Reading JavaScript Functions
Functions are fundamental building blocks in JavaScript. They allow you to encapsulate code for reuse and improve readability.
To read a function, pay attention to:
- Function Declaration: Defined with the `function` keyword.
- Parameters: Variables listed in the function definition.
- Return Statement: Specifies the value returned by the function.
Example of a simple function:
javascript
function add(a, b) {
return a + b; // Returns the sum of a and b
}
In this example, `add` is a function that takes two parameters, `a` and `b`, and returns their sum. Understanding how functions are structured and utilized is crucial for navigating through JavaScript code.
Working with Objects and Arrays
Objects and arrays are two of the most important data structures in JavaScript. Objects are collections of properties, while arrays are ordered lists of values.
To read and manipulate objects:
- Use dot notation (`object.property`) or bracket notation (`object[‘property’]`).
- Example:
javascript
let person = {
name: “Alice”,
age: 25
};
console.log(person.name); // Outputs: Alice
For arrays, you can access elements using their index:
- Example:
javascript
let fruits = [“apple”, “banana”, “cherry”];
console.log(fruits[1]); // Outputs: banana
Understanding how to interact with these data structures is essential for effective JavaScript programming.
Understanding JavaScript Syntax
JavaScript syntax is the set of rules that defines a correctly structured JavaScript program. It includes how to write statements, use variables, and implement functions.
- Variables: Used to store data values. Declare them using `var`, `let`, or `const`.
- Data Types:
- String: Represents text. Example: `”Hello, World!”`
- Number: Represents numerical values. Example: `42`
- Boolean: Represents true or values. Example: `true`
- Object: A collection of key-value pairs. Example: `{ name: “John”, age: 30 }`
- Array: An ordered list of values. Example: `[1, 2, 3]`
Reading JavaScript Statements
JavaScript statements are instructions that the browser executes. They can be expressions, variable declarations, or function calls.
- Expression: A combination of values and operators that evaluates to a value.
- Function Declaration: Defines a reusable block of code. Example:
javascript
function greet(name) {
return “Hello, ” + name;
}
Understanding Control Structures
Control structures dictate the flow of the program. They include conditional statements and loops.
– **Conditional Statements**:
– **if…else**: Executes code based on a condition.
– **switch**: Selects one of many blocks of code to execute.
Example of an `if` statement:
javascript
if (age >= 18) {
console.log(“Adult”);
} else {
console.log(“Minor”);
}
- Loops: Used to execute a block of code multiple times.
- for loop: Iterates a specified number of times.
- while loop: Executes as long as a condition is true.
Example of a `for` loop:
javascript
for (let i = 0; i < 5; i++) {
console.log(i);
}
Exploring Functions and Scope
Functions are fundamental to JavaScript. They encapsulate reusable code and can accept parameters.
– **Function Expressions**: Can be anonymous and assigned to variables.
– **Arrow Functions**: A concise syntax for writing functions.
Example of an arrow function:
javascript
const add = (a, b) => a + b;
Scope determines the accessibility of variables:
- Global Scope: Variables defined outside functions are accessible anywhere.
- Local Scope: Variables defined within a function are accessible only inside that function.
Debugging JavaScript Code
Effective debugging is essential for reading and understanding JavaScript.
- Console.log(): Use this function to print messages or variable values to the console for inspection.
- Browser Developer Tools: Most browsers come with built-in tools for debugging JavaScript. Access them via right-click and selecting “Inspect” or pressing F12.
Common debugging techniques include:
- Setting breakpoints to pause execution.
- Step through code line by line.
- Checking the call stack and local variables.
Using JavaScript Libraries and Frameworks
Libraries and frameworks can streamline JavaScript development, allowing for more efficient coding practices.
- Popular Libraries:
- jQuery: Simplifies DOM manipulation and event handling.
- Lodash: Provides utility functions for common programming tasks.
- Frameworks:
- React: A library for building user interfaces.
- Vue: A progressive framework for building UIs.
- Angular: A platform for building mobile and desktop web applications.
Utilizing these tools can enhance productivity and improve code readability.
Expert Insights on How to Read JavaScript Effectively
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To read JavaScript effectively, one must first understand its asynchronous nature and event-driven architecture. Familiarizing oneself with concepts like callbacks, promises, and async/await is crucial for grasping how JavaScript operates in a web environment.”
Michael Chen (JavaScript Instructor, Code Academy). “I recommend starting with the fundamentals of JavaScript syntax and structure. Once you are comfortable with variables, functions, and control flow, practice reading code from open-source projects. This will expose you to various coding styles and best practices.”
Lisa Patel (Frontend Developer, Creative Solutions LLC). “Utilizing browser developer tools is essential for reading JavaScript. By stepping through code with breakpoints and observing variable states in real-time, you can gain a deeper understanding of how JavaScript interacts with HTML and CSS on a live page.”
Frequently Asked Questions (FAQs)
What are the basic concepts I need to understand to read JavaScript?
To read JavaScript effectively, familiarize yourself with variables, data types, functions, control structures (like loops and conditionals), and objects. Understanding the Document Object Model (DOM) is also essential for interacting with web pages.
How can I improve my JavaScript reading skills?
Improving your JavaScript reading skills involves practice. Start by reading and analyzing existing code, using online resources like tutorials and documentation, and participating in coding communities. Regularly writing your own code will also enhance your comprehension.
What tools can assist in reading JavaScript code?
Utilize code editors like Visual Studio Code or Sublime Text, which offer syntax highlighting and code completion features. Browser developer tools are also invaluable for debugging and inspecting JavaScript in real-time.
Are there specific resources for learning JavaScript syntax and structure?
Yes, resources such as MDN Web Docs, JavaScript.info, and various online courses on platforms like Codecademy or freeCodeCamp provide comprehensive guides on JavaScript syntax and structure.
How do I interpret JavaScript error messages?
Interpreting JavaScript error messages requires understanding common error types, such as syntax errors, reference errors, and type errors. Each message typically indicates the line number and nature of the issue, guiding you towards resolution.
Can I read JavaScript code without prior programming experience?
While prior programming experience is beneficial, it is not strictly necessary. Beginners can start with foundational concepts and gradually build their understanding of JavaScript through structured learning and practice.
Reading JavaScript effectively requires a foundational understanding of its syntax, structure, and core concepts. Familiarity with the language’s basic constructs, such as variables, functions, and control flow statements, is essential. Additionally, recognizing the importance of the Document Object Model (DOM) and how JavaScript interacts with HTML and CSS is crucial for web development. By mastering these elements, one can begin to interpret and write JavaScript code with confidence.
Another significant aspect of reading JavaScript is the ability to utilize debugging tools and browser developer consoles. These tools allow developers to step through code, inspect variables, and understand the flow of execution. Furthermore, leveraging online resources, such as documentation, tutorials, and coding communities, can greatly enhance one’s ability to read and comprehend JavaScript code. Engaging with these resources fosters a deeper understanding of both common patterns and advanced techniques.
Lastly, practice plays a vital role in becoming proficient at reading JavaScript. Regularly engaging with code, whether through personal projects or contributing to open-source endeavors, helps solidify one’s skills. As one encounters various coding styles and frameworks, adaptability and continuous learning become key components of mastering JavaScript. Ultimately, a combination of theoretical knowledge, practical experience, and community engagement
Author Profile
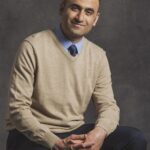
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?