How Can You Read Outlook Email Using Access VBA?
In today’s fast-paced digital landscape, effective communication is paramount, and Microsoft Outlook has become a cornerstone for managing emails in both personal and professional realms. For developers and data analysts, integrating Outlook with Microsoft Access can streamline workflows and enhance productivity. Imagine being able to pull important email data directly into your Access database, allowing for seamless reporting, analysis, and even automation of tasks. This powerful combination opens up a world of possibilities, making it easier to manage information and derive insights from your email communications.
Reading Outlook emails from Access using VBA (Visual Basic for Applications) is a skill that can significantly elevate your data management capabilities. By leveraging the built-in functionalities of both applications, you can create custom solutions tailored to your specific needs. Whether you’re looking to extract contact information, track project-related emails, or automate responses, understanding how to bridge these two platforms can save you time and effort.
As we delve deeper into this topic, you’ll discover the essential techniques and coding practices required to access and manipulate Outlook emails directly from Access. From setting up your environment to writing the necessary VBA code, this guide will equip you with the knowledge to harness the full potential of your email data, transforming the way you work with information. Get ready to unlock new efficiencies and insights that can propel your projects forward!
Setting Up Outlook Reference in Access
To read Outlook emails using VBA in Access, it’s essential to set up a reference to the Outlook library. This allows you to leverage Outlook’s objects, methods, and properties within your Access application. Follow these steps:
- Open your Access database.
- Press `ALT + F11` to open the Visual Basic for Applications (VBA) editor.
- In the VBA editor, go to `Tools` > `References`.
- In the References dialog box, scroll down until you find “Microsoft Outlook xx.x Object Library” (where xx.x corresponds to your version of Outlook).
- Check the box next to it and click `OK`.
By establishing this reference, you can now access Outlook’s functionalities directly from your Access VBA code.
Reading Emails from Outlook
Once the reference is set up, you can begin reading emails from your Outlook inbox. Below is a sample code snippet that demonstrates how to access and read the subject lines of emails in the inbox.
“`vba
Sub ReadOutlookEmails()
Dim OutlookApp As Object
Dim OutlookNamespace As Object
Dim Inbox As Object
Dim MailItem As Object
Dim i As Integer
‘ Create Outlook application object
Set OutlookApp = CreateObject(“Outlook.Application”)
Set OutlookNamespace = OutlookApp.GetNamespace(“MAPI”)
Set Inbox = OutlookNamespace.GetDefaultFolder(6) ‘ 6 refers to the Inbox
‘ Loop through the items in the Inbox
For i = 1 To Inbox.Items.Count
If TypeOf Inbox.Items(i) Is Outlook.MailItem Then
Set MailItem = Inbox.Items(i)
Debug.Print MailItem.Subject ‘ Display subject in the Immediate Window
End If
Next i
‘ Clean up
Set MailItem = Nothing
Set Inbox = Nothing
Set OutlookNamespace = Nothing
Set OutlookApp = Nothing
End Sub
“`
This code initializes the Outlook application, accesses the inbox, and iterates through the emails, printing the subject of each email to the Immediate Window.
Handling Various Email Properties
When reading emails, you may want to extract various properties beyond just the subject. The following table summarizes some of the commonly accessed properties of an Outlook email:
Property | Description |
---|---|
Subject | The subject line of the email. |
SenderName | The name of the person who sent the email. |
ReceivedTime | The date and time when the email was received. |
Body | The main content of the email. |
Attachments | Any files attached to the email. |
To access these properties, you can modify the loop in the previous code snippet. For example, to print both the sender’s name and the received time, you could do the following:
“`vba
Debug.Print “Subject: ” & MailItem.Subject
Debug.Print “From: ” & MailItem.SenderName
Debug.Print “Received: ” & MailItem.ReceivedTime
“`
This way, you can gather comprehensive information from each email in your inbox, enhancing your data processing capabilities within Access VBA.
Accessing Outlook Email through VBA
To read Outlook emails from Access using VBA, you first need to ensure that you have a reference to the Microsoft Outlook Object Library. This allows you to utilize Outlook’s functionalities directly within your Access application.
Setting Up the Reference
- Open your Access database.
- Press `ALT + F11` to open the VBA editor.
- In the VBA editor, go to `Tools` > `References`.
- Find and check `Microsoft Outlook xx.0 Object Library` (where `xx` corresponds to your version of Outlook).
- Click `OK` to close the dialog.
Example VBA Code to Read Emails
The following code snippet demonstrates how to connect to Outlook and read emails from the Inbox folder.
“`vba
Sub ReadOutlookEmails()
Dim OutlookApp As Object
Dim OutlookNamespace As Object
Dim InboxFolder As Object
Dim EmailItem As Object
Dim i As Integer
‘ Create an instance of Outlook
Set OutlookApp = CreateObject(“Outlook.Application”)
Set OutlookNamespace = OutlookApp.GetNamespace(“MAPI”)
Set InboxFolder = OutlookNamespace.GetDefaultFolder(6) ‘ 6 refers to the Inbox folder
‘ Loop through the items in the Inbox
For i = 1 To InboxFolder.Items.Count
Set EmailItem = InboxFolder.Items(i)
‘ Display the subject and sender
Debug.Print “Subject: ” & EmailItem.Subject
Debug.Print “Sender: ” & EmailItem.SenderName
Debug.Print “Received: ” & EmailItem.ReceivedTime
Debug.Print “————————————”
Next i
‘ Clean up
Set EmailItem = Nothing
Set InboxFolder = Nothing
Set OutlookNamespace = Nothing
Set OutlookApp = Nothing
End Sub
“`
Key Components of the Code
- Creating Outlook Objects: The code initializes Outlook application objects to connect to the mailbox.
- Accessing the Inbox: The method `GetDefaultFolder(6)` is used to access the Inbox. The number `6` corresponds to the Inbox folder in Outlook.
- Looping Through Emails: A `For` loop iterates through each email item in the Inbox, allowing you to read properties like `Subject`, `SenderName`, and `ReceivedTime`.
Error Handling
Incorporating error handling into your code is essential for a robust application. Here’s an example of how to implement basic error handling:
“`vba
On Error GoTo ErrorHandler
‘ Your email reading code here…
Exit Sub
ErrorHandler:
Debug.Print “An error occurred: ” & Err.Description
End Sub
“`
Additional Considerations
- Performance: Reading a large number of emails can take time. Consider filtering emails based on criteria such as date or sender to improve performance.
- Security Settings: Depending on your Outlook security settings, you may encounter prompts or restrictions when accessing emails through VBA.
- Permissions: Ensure that your Access application has the necessary permissions to interact with Outlook.
Useful Properties of Email Items
Property | Description |
---|---|
Subject | The subject line of the email |
SenderName | The name of the sender |
ReceivedTime | The date and time the email was received |
Body | The content of the email |
Attachments | Any attachments included in the email |
By following the steps and utilizing the provided code, you can effectively read and process Outlook emails directly from your Access application using VBA.
Expert Insights on Accessing Outlook Emails via VBA
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing VBA to read Outlook emails can significantly enhance data management processes within Access databases. By leveraging the Outlook Object Model, developers can automate the retrieval of emails, allowing for seamless integration of email data into Access applications.”
Michael Chen (Database Administrator, Data Solutions Group). “When implementing VBA to read Outlook emails, it is crucial to handle security permissions properly. Ensure that your Access application has the necessary permissions to access Outlook, and consider using late binding to avoid compatibility issues with different Outlook versions.”
Sarah Thompson (VBA Consultant, CodeCraft Solutions). “For effective email processing using Access VBA, I recommend creating a dedicated module that encapsulates all Outlook interactions. This modular approach not only improves code maintainability but also allows for easier debugging and enhancement of email handling functionalities.”
Frequently Asked Questions (FAQs)
How can I access Outlook emails using Access VBA?
You can access Outlook emails using Access VBA by referencing the Outlook Object Library in your VBA project and utilizing the Outlook Application object to interact with the email items.
What code is needed to establish a connection to Outlook from Access VBA?
To establish a connection, you can use the following code snippet:
“`vba
Dim olApp As Outlook.Application
Set olApp = New Outlook.Application
“`
This initializes a new instance of the Outlook application.
How do I retrieve emails from a specific folder in Outlook using Access VBA?
You can retrieve emails from a specific folder by accessing the `Folders` collection of the `Namespace` object. For example:
“`vba
Dim olNamespace As Outlook.Namespace
Set olNamespace = olApp.GetNamespace(“MAPI”)
Dim olFolder As Outlook.Folder
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
“`
Can I filter emails based on specific criteria using Access VBA?
Yes, you can filter emails by using the `Restrict` method on the `Items` collection of a folder. For example:
“`vba
Dim filteredItems As Outlook.Items
Set filteredItems = olFolder.Items.Restrict(“[Subject] = ‘Your Subject'”)
“`
What are some common errors when reading Outlook emails from Access VBA?
Common errors include issues with object references, such as not setting the Outlook application object correctly, or permission issues when accessing certain folders or items.
Is it possible to automate email processing with Access VBA?
Yes, you can automate email processing by writing VBA scripts that loop through emails, apply conditions, and perform actions such as moving or deleting emails based on your criteria.
In summary, reading Outlook emails from Access using VBA involves establishing a connection to the Outlook application through the Microsoft Outlook Object Library. By utilizing this library, developers can automate the process of accessing emails, allowing for efficient data extraction and manipulation directly within Access. The key steps include creating an Outlook application object, accessing the inbox, and iterating through the email items to retrieve desired information.
Furthermore, it is important to handle potential errors gracefully, as issues may arise if Outlook is not installed or if there are permission restrictions. Implementing error handling routines in the VBA code ensures that the application remains robust and user-friendly. Additionally, understanding the structure of Outlook items and properties is crucial for effectively extracting the required data.
Ultimately, leveraging Access VBA to read Outlook emails can significantly enhance productivity by automating routine tasks and integrating email data into database applications. This integration not only streamlines workflows but also provides valuable insights by combining email communications with other data sets within Access.
Author Profile
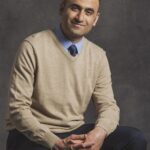
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?