How Can You Read an XML File in Python Effectively?
In the ever-evolving landscape of data formats, XML (eXtensible Markup Language) remains a cornerstone for data interchange across diverse platforms and applications. Its structured, human-readable format makes it a popular choice for configuration files, web services, and more. However, for many developers and data enthusiasts, the challenge lies not in the creation of XML files but in effectively reading and manipulating them using programming languages like Python. If you’ve ever found yourself staring at an XML document, unsure of how to extract the information you need, you’re not alone. This article is designed to guide you through the process of reading XML files in Python, unlocking the potential of this versatile data format.
Understanding how to read XML files in Python opens up a world of possibilities for data manipulation and analysis. Python, with its rich ecosystem of libraries, provides several tools that simplify the task of parsing and navigating XML structures. From built-in libraries like `xml.etree.ElementTree` to more advanced options like `lxml`, Python offers a range of solutions tailored to different needs and complexities. Whether you’re working with simple data structures or intricate nested elements, mastering XML reading techniques can significantly enhance your data processing capabilities.
As we delve deeper into this topic, you’ll discover the fundamental concepts behind XML parsing
Using ElementTree to Parse XML
The ElementTree module is part of the Python Standard Library and provides a simple and efficient way to parse XML files. It allows you to read, manipulate, and create XML data easily. To read an XML file using ElementTree, follow these steps:
- Import the `ElementTree` module.
- Use the `parse` method to read the XML file.
- Navigate through the elements using various methods like `find`, `findall`, and `iter`.
Here is an example of how to read an XML file with ElementTree:
“`python
import xml.etree.ElementTree as ET
Parse the XML file
tree = ET.parse(‘file.xml’)
root = tree.getroot()
Accessing elements
for child in root:
print(child.tag, child.attrib)
“`
This code snippet reads an XML file named `file.xml`, parses it, and prints the tag and attributes of each child element under the root.
Reading XML with lxml
For more advanced XML processing, the `lxml` library offers extensive capabilities and better performance. It supports XPath and XSLT, which can be beneficial for complex XML structures. To read an XML file using `lxml`, follow these steps:
- Install the `lxml` library if it is not already installed.
- Use the `etree` module to parse the XML file.
- Utilize XPath expressions to query specific parts of the XML.
To install `lxml`, use pip:
“`bash
pip install lxml
“`
Here is a code example using `lxml`:
“`python
from lxml import etree
Parse the XML file
tree = etree.parse(‘file.xml’)
root = tree.getroot()
Using XPath to find elements
for element in root.xpath(‘//tagname’):
print(element.text)
“`
In this example, replace `tagname` with the actual tag you are interested in to retrieve its text content.
XML File Structure Example
Understanding the structure of your XML file is crucial for effective parsing. Below is a simple representation of an XML file:
Tag | Description |
---|---|
<note> | The root element. |
<to> | Recipient of the note. |
<from> | Sender of the note. |
<heading> | Title of the note. |
<body> | The main content of the note. |
This XML structure illustrates a simple note format, which can be parsed using the methods discussed.
Error Handling While Reading XML
When reading XML files, it’s essential to include error handling to manage potential issues, such as malformed XML or missing files. You can use try-except blocks to catch exceptions. Here’s an example:
“`python
try:
tree = ET.parse(‘file.xml’)
root = tree.getroot()
except FileNotFoundError:
print(“The specified file was not found.”)
except ET.ParseError:
print(“Error parsing the XML file.”)
“`
This code will gracefully handle file-not-found errors and parsing errors, providing feedback to the user without crashing the program.
Using the ElementTree Module
The `xml.etree.ElementTree` module is a built-in Python library that provides efficient tools for parsing and creating XML data. It is user-friendly and suitable for most XML parsing tasks.
Reading an XML File
To read an XML file using the ElementTree module, follow these steps:
- Import the Module: Ensure that you import the ElementTree module.
- Parse the XML File: Use the `parse()` method to load the XML file.
- Access Elements: Navigate through the XML structure using element methods.
Example Code
“`python
import xml.etree.ElementTree as ET
Parse the XML file
tree = ET.parse(‘example.xml’)
root = tree.getroot()
Iterate through elements
for child in root:
print(child.tag, child.attrib)
“`
Using the lxml Library
The `lxml` library is a powerful XML processing library that offers a richer set of features compared to ElementTree. It provides better performance and supports XPath and XSLT.
Installation
To use `lxml`, install it via pip if it’s not already installed:
“`bash
pip install lxml
“`
Reading an XML File
The process of reading an XML file using `lxml` is similar to ElementTree but includes additional functionalities.
Example Code
“`python
from lxml import etree
Parse the XML file
tree = etree.parse(‘example.xml’)
root = tree.getroot()
Access elements with XPath
for element in root.xpath(‘//child’):
print(element.tag, element.text)
“`
Using the minidom Module
The `xml.dom.minidom` module is another option for parsing XML in Python. It provides a DOM (Document Object Model) interface, which can be useful for certain applications.
Reading an XML File
You can read an XML file with minidom as follows:
Example Code
“`python
from xml.dom import minidom
Parse the XML file
doc = minidom.parse(‘example.xml’)
Get elements by tag name
elements = doc.getElementsByTagName(‘child’)
for elem in elements:
print(elem.tagName, elem.firstChild.nodeValue)
“`
Comparative Overview
The following table outlines the key features of the three libraries discussed:
Feature | ElementTree | lxml | minidom |
---|---|---|---|
Built-in | Yes | No | Yes |
Performance | Moderate | High | Low |
XPath Support | Limited | Yes | No |
XSLT Support | No | Yes | No |
Memory Usage | Low | Moderate | High |
Choosing the right library depends on your specific requirements, such as performance needs, the complexity of XML, and the use of advanced features like XPath or XSLT.
Expert Insights on Reading XML Files in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Utilizing Python’s built-in libraries such as `xml.etree.ElementTree` is essential for efficiently parsing XML files. This library provides a simple and effective way to navigate and manipulate XML data, making it an ideal choice for data extraction tasks.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For those dealing with complex XML structures, I recommend using the `lxml` library. It offers robust support for XPath and XSLT, allowing developers to perform advanced queries and transformations on XML data seamlessly.”
Sarah Thompson (Technical Writer, Python Programming Magazine). “When working with XML in Python, it is crucial to handle exceptions and ensure proper encoding. Implementing error handling will prevent runtime issues and ensure that your application can gracefully manage unexpected XML formats.”
Frequently Asked Questions (FAQs)
How can I read an XML file in Python?
You can read an XML file in Python using the `xml.etree.ElementTree` module. First, import the module, then use `ElementTree.parse()` to load the XML file and `getroot()` to access the root element.
What libraries are available for parsing XML in Python?
Several libraries are available for parsing XML in Python, including `xml.etree.ElementTree`, `lxml`, and `minidom`. Each library has its own features and advantages depending on the complexity of the XML data.
What is the difference between `xml.etree.ElementTree` and `lxml`?
`xml.etree.ElementTree` is part of the Python standard library and is suitable for simple XML parsing. `lxml` is a third-party library that provides more advanced features, better performance, and support for XPath and XSLT.
How do I handle namespaces when reading XML files in Python?
When handling namespaces, use the `find()` or `findall()` methods with the namespace defined in a dictionary. This allows you to correctly access elements that are within a specific namespace.
Can I convert XML data into a Python dictionary?
Yes, you can convert XML data into a Python dictionary using libraries like `xmltodict`. This library simplifies the process by transforming XML into a nested dictionary structure, making it easier to work with in Python.
What should I do if my XML file is large and causes memory issues?
For large XML files, consider using the `iterparse()` method from `xml.etree.ElementTree`, which allows you to process the file incrementally. This approach reduces memory usage by parsing the XML file in chunks rather than loading it entirely into memory.
Reading XML files in Python is a straightforward process that can be accomplished using various libraries designed for parsing XML data. The most commonly used libraries include `xml.etree.ElementTree`, `lxml`, and `xml.dom.minidom`. Each of these libraries offers different functionalities and levels of complexity, allowing developers to choose the one that best fits their needs.
ElementTree is part of Python’s standard library and provides a simple and efficient way to parse and navigate XML trees. It allows users to read XML files, access elements, and manipulate data with ease. For more complex XML structures or performance-intensive applications, the `lxml` library is recommended due to its speed and additional features, such as XPath support. Alternatively, `xml.dom.minidom` offers a Document Object Model (DOM) approach, which may be more intuitive for those familiar with DOM manipulation in web development.
In summary, the choice of library for reading XML files in Python largely depends on the specific requirements of the project, such as performance needs and the complexity of the XML data. Understanding the strengths and weaknesses of each library can help developers make informed decisions and efficiently handle XML data in their applications.
Author Profile
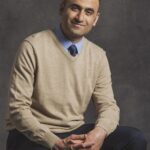
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?