How Can You Reassign a List Value in Python?
In the world of programming, lists are one of the most versatile and widely used data structures in Python. They allow you to store a collection of items, ranging from numbers and strings to more complex objects. However, as your program evolves, the need to modify or reassign values within these lists becomes essential. Whether you’re updating user data, managing inventory, or simply refining your code, understanding how to effectively reassign list values is crucial for any Python developer.
Reassigning a value in a list may seem straightforward, but it opens the door to a myriad of possibilities and techniques that can enhance your coding efficiency. From simple index-based assignments to more complex operations involving loops and conditions, Python provides a rich toolkit for manipulating lists. This flexibility not only allows for quick updates but also empowers developers to create dynamic and responsive applications.
As we delve deeper into the mechanics of reassigning list values in Python, we’ll explore various methods and best practices that can streamline your coding process. Whether you’re a beginner looking to grasp the fundamentals or an experienced programmer seeking to refine your skills, this guide will equip you with the knowledge needed to master list manipulation in Python. Get ready to unlock the potential of your code and elevate your programming prowess!
Understanding List Reassignment
In Python, lists are mutable data structures, which means that their contents can be changed after they are created. Reassigning a list value involves modifying an existing list or changing the reference of a list variable to point to a new list. This can be achieved through indexing or by assigning a new list to the variable.
Reassigning Elements in a List
To reassign an element at a specific index in a list, you simply reference the index and assign a new value. For example:
python
my_list = [1, 2, 3, 4, 5]
my_list[2] = 10 # Reassigning the value at index 2
print(my_list) # Output: [1, 2, 10, 4, 5]
This method allows you to maintain the original list while updating specific elements. It is important to ensure that the index you are accessing is within the bounds of the list.
Reassigning the Entire List
If you want to change the entire list, you can simply assign a new list to the variable. This will update the reference held by the variable to point to the new list:
python
my_list = [1, 2, 3, 4, 5]
my_list = [6, 7, 8] # Reassigning the entire list
print(my_list) # Output: [6, 7, 8]
In this case, the old list is no longer accessible through `my_list`, as it now points to a completely new list.
Considerations for Reassignment
When reassigning list values, consider the implications of mutability and references. Here are some key points to keep in mind:
- Indexing: Directly modifying elements using indices does not change the identity of the list. The original list remains intact.
- Reassigning the Variable: Reassigning the variable to a new list does not alter the original list; it merely changes what the variable points to.
- Shared References: If multiple variables reference the same list, modifying the list through one variable affects all references.
Operation | Effect on List | Original List |
---|---|---|
my_list[0] = 10 | Modifies first element | Changes value in the original list |
my_list = [10, 20] | Reassigns variable | Original list is unchanged |
other_list = my_list | Creates reference | Both variables reference the same list |
Understanding these concepts is crucial for effective list manipulation in Python, allowing for more control over data structures in your programs.
Reassigning List Values in Python
Reassigning values in a list in Python is straightforward. Lists in Python are mutable, which means you can change their contents without creating a new list.
Accessing List Elements
To reassign a value in a list, you first need to access the desired index of the element you want to change. Python uses zero-based indexing, meaning the first element is at index 0.
Example:
python
my_list = [10, 20, 30, 40]
my_list[1] = 25 # Changes the second element from 20 to 25
Reassigning Multiple Values
You can also reassign multiple values in a list using slicing. Slicing allows you to target a section of the list and replace it with new values.
Example:
python
my_list = [10, 20, 30, 40]
my_list[1:3] = [25, 35] # Changes the second and third elements
Using Loops for Reassignment
In scenarios where you want to modify multiple values based on certain conditions, loops can be particularly useful.
Example:
python
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list)):
if my_list[i] % 2 == 0: # Checks if the element is even
my_list[i] = my_list[i] * 2 # Reassigns the even element to its double
Conditional Reassignment
You can also use conditional expressions while reassigning values in a list.
Example:
python
my_list = [1, 2, 3, 4, 5]
my_list = [x * 2 if x % 2 == 0 else x for x in my_list] # Doubles even numbers
Using Functions to Reassign Values
Defining functions can encapsulate the logic for reassigning values, making your code more organized and reusable.
Example:
python
def double_even_numbers(lst):
for i in range(len(lst)):
if lst[i] % 2 == 0:
lst[i] = lst[i] * 2
return lst
my_list = [1, 2, 3, 4, 5]
my_list = double_even_numbers(my_list)
Performance Considerations
When reassigning values in large lists, consider the following points:
- Time Complexity: Reassignment generally operates in O(1) for single elements and O(n) for slices.
- Memory Usage: Slicing creates a new list in memory, which can lead to higher memory consumption during reassignments.
Common Pitfalls
- Attempting to access an index that is out of range will raise an `IndexError`.
- Using slicing incorrectly may lead to unexpected results if the dimensions do not match.
By understanding these principles, you can effectively manage and reassign values within lists in Python, enhancing the functionality of your code.
Expert Insights on Reassigning List Values in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, reassigning a value in a list is straightforward. You can directly access an index of the list and assign a new value to it using the syntax `list[index] = new_value`. This method is efficient and allows for dynamic updates of list contents, which is essential for data manipulation in various applications.
Michael Chen (Lead Python Developer, CodeCraft Solutions). When reassigning a list value in Python, it is crucial to ensure that the index you are accessing is within the bounds of the list. Attempting to access an index that does not exist will raise an IndexError. Therefore, always validate the index before performing the reassignment to maintain robust code.
Sarah Thompson (Data Scientist, Analytics Pro). In practice, reassigning list values can be part of data preprocessing in machine learning workflows. Using list comprehensions can also be an elegant way to modify list values conditionally. For instance, you can create a new list with modified values based on certain criteria, enhancing both readability and efficiency in your code.
Frequently Asked Questions (FAQs)
How can I reassign a specific index in a list in Python?
To reassign a specific index in a list, use the assignment operator. For example, if you have a list `my_list = [1, 2, 3]` and want to change the value at index 1, you can do `my_list[1] = 5`, resulting in `my_list` being `[1, 5, 3]`.
Can I reassign multiple values in a list at once?
Yes, you can reassign multiple values using slicing. For instance, if you want to change the first two elements, you can do `my_list[0:2] = [4, 5]`, which will update `my_list` accordingly.
What happens if I try to reassign an index that is out of range?
Attempting to reassign an index that is out of range will raise an `IndexError`. Ensure the index exists within the current bounds of the list before attempting reassignment.
Is it possible to reassign a list value using a loop?
Yes, you can use a loop to reassign values in a list. For example, using a `for` loop, you can iterate through indices and assign new values as needed, such as `for i in range(len(my_list)): my_list[i] = new_value`.
Can I reassign values in a list using a condition?
Absolutely. You can use a conditional statement within a loop to selectively reassign values. For example, `for i in range(len(my_list)): if my_list[i] < 3: my_list[i] = 0` will change all values less than 3 to 0.
What is the difference between reassigning a list value and appending a new value?
Reassigning a list value replaces an existing element at a specified index, while appending adds a new element to the end of the list. Use `my_list.append(new_value)` to add a value without modifying existing elements.
Reassigning a list value in Python is a fundamental operation that allows developers to manipulate data structures effectively. Lists in Python are mutable, meaning that their contents can be changed after creation. This mutability enables users to easily update, add, or remove elements from a list, making it a versatile tool for various programming tasks. The process of reassigning a value typically involves specifying the index of the element to be changed and assigning a new value to that index.
To reassign a list value, one can simply use the assignment operator. For example, if you have a list named `my_list` and you want to change the value at index 2, you would use the syntax `my_list[2] = new_value`. This straightforward approach highlights the simplicity and efficiency of list manipulation in Python. Additionally, lists can be reassigned as a whole, allowing for the replacement of multiple values or even the entire list with a new one.
Key takeaways from this discussion include understanding the mutable nature of lists in Python and the ease with which one can reassign values. This capability is crucial for dynamic programming, where data may need to be updated frequently. Moreover, mastering list reassignment is essential for effective data management,
Author Profile
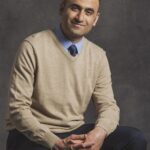
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?