How Can You Remove a Key from a Dictionary in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow developers to store and manipulate data in key-value pairs, making it easy to access and modify information. However, as your applications evolve, there may come a time when you need to remove a key from a dictionary. Whether you’re cleaning up data, optimizing performance, or simply restructuring your code, understanding how to effectively manage dictionary keys is a vital skill for any Python programmer.
Removing a key from a dictionary in Python is a straightforward process, but it’s essential to grasp the various methods available to ensure you’re using the most efficient approach for your needs. Each method has its own nuances, advantages, and potential pitfalls, which can affect your program’s behavior and performance. From using the `del` statement to the `pop()` method, or even employing dictionary comprehensions, there are multiple techniques to achieve the same goal.
As we delve deeper into the topic, we’ll explore these different methods, providing you with the knowledge and tools necessary to manipulate dictionaries with confidence. By the end of this article, you’ll not only know how to remove keys effectively but also understand the implications of each method, empowering you to write cleaner and more efficient Python code.
Using the `del` Statement
The `del` statement is a straightforward and efficient way to remove a key from a dictionary in Python. By specifying the key you wish to delete, you can effectively remove it along with its associated value.
Example:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
del my_dict[‘age’]
After executing this code, `my_dict` will become `{‘name’: ‘Alice’, ‘city’: ‘New York’}`.
Using the `pop()` Method
The `pop()` method serves a dual purpose: it removes the specified key from the dictionary and returns its corresponding value. This can be particularly useful when you need to use the value after deletion.
Example:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
age = my_dict.pop(‘age’)
After this operation, `my_dict` will be `{‘name’: ‘Alice’, ‘city’: ‘New York’}`, and the variable `age` will hold the value `30`.
Using the `popitem()` Method
If you need to remove an arbitrary key-value pair from a dictionary, you can use the `popitem()` method. This method removes and returns a tuple of the last inserted key-value pair. It is important to note that this method works only in dictionaries that preserve insertion order (Python 3.7+).
Example:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
key, value = my_dict.popitem()
After execution, `my_dict` will have one less item, and `key` and `value` will hold the last inserted pair.
Using Dictionary Comprehension
For more complex scenarios where you may want to remove multiple keys or filter keys based on a condition, dictionary comprehension is a powerful tool. This method allows you to create a new dictionary without the unwanted keys.
Example:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
keys_to_remove = [‘age’, ‘city’]
filtered_dict = {k: v for k, v in my_dict.items() if k not in keys_to_remove}
The resulting `filtered_dict` will contain only the remaining key-value pairs.
Comparison of Methods
The table below summarizes the different methods of removing keys from a dictionary in Python:
Method | Description | Returns Value |
---|---|---|
del | Removes the specified key and its value | No |
pop() | Removes the specified key and returns its value | Yes |
popitem() | Removes and returns the last inserted key-value pair | Yes (as a tuple) |
Dictionary Comprehension | Creates a new dictionary excluding specified keys | No (creates new dict) |
Each method has its own use case, and the choice largely depends on the specific requirements of your code.
Methods to Remove a Key from a Dictionary in Python
In Python, dictionaries are mutable collections of key-value pairs, allowing for dynamic modifications. To remove a key from a dictionary, several methods can be employed, each with its own characteristics and use cases.
Using the `del` Statement
The `del` statement can be utilized to delete a specified key from a dictionary. This approach is straightforward and raises an error if the key does not exist.
python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
del my_dict[‘b’]
- Pros:
- Simple syntax.
- Immediate removal of the key-value pair.
- Cons:
- Raises a `KeyError` if the key is absent.
Using the `pop()` Method
The `pop()` method removes a key and returns its associated value. If the specified key is not found, it can return a default value instead of raising an error.
python
value = my_dict.pop(‘b’, ‘Key not found’)
- Pros:
- Returns the value associated with the removed key.
- Allows for a default return value to avoid errors.
- Cons:
- If no default is provided and the key does not exist, it raises a `KeyError`.
Using the `popitem()` Method
The `popitem()` method removes the last inserted key-value pair from the dictionary. This is particularly useful in scenarios where the order of insertion matters.
python
last_item = my_dict.popitem()
- Pros:
- Efficient way to remove items when order is important.
- Cons:
- Only removes the last item; not suitable for targeted removals.
Using Dictionary Comprehension for Conditional Removal
If a key must be removed based on a condition, dictionary comprehension provides a flexible approach by creating a new dictionary that excludes specified keys.
python
my_dict = {k: v for k, v in my_dict.items() if k != ‘b’}
- Pros:
- Allows for complex conditions for removal.
- Creates a new dictionary while leaving the original intact.
- Cons:
- Less efficient for large dictionaries due to the creation of a new object.
Key Considerations
When choosing a method for removing keys from a dictionary, consider the following aspects:
Method | Raises Error | Returns Value | Use Case |
---|---|---|---|
`del` | Yes | No | When you are sure the key exists. |
`pop()` | Optional | Yes | When you want the value or handle absent keys gracefully. |
`popitem()` | No | Yes | When you need to remove the last inserted item. |
Comprehension | No | No | When you need conditional removal. |
The appropriate method for removing a key from a dictionary in Python depends on the specific requirements of your application and the expected behavior regarding key absence. Choose the method that best aligns with your needs, ensuring efficient and error-free modifications to your dictionary structure.
Expert Insights on Removing Keys from Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To effectively remove a key from a dictionary in Python, the most straightforward method is to use the `del` statement. This approach not only removes the key but also frees up memory associated with it, which is essential for optimizing performance in larger applications.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “An alternative method to remove a key from a dictionary is by using the `pop()` method. This is particularly useful when you want to retrieve the value associated with the key before deletion. It allows for more dynamic handling of dictionary data, especially in cases where the existence of the key is uncertain.”
Sarah Lee (Data Scientist, Analytics Hub). “For those working with data processing in Python, utilizing dictionary comprehensions can be an elegant way to remove multiple keys at once. By creating a new dictionary that excludes the unwanted keys, developers can maintain clean and efficient data structures without modifying the original dictionary directly.”
Frequently Asked Questions (FAQs)
How can I remove a key from a dictionary in Python?
You can remove a key from a dictionary using the `del` statement or the `pop()` method. For example, `del my_dict[key]` or `my_dict.pop(key)` will both remove the specified key.
What happens if I try to remove a key that does not exist?
Using `del` on a non-existent key will raise a `KeyError`. However, using `pop()` allows you to specify a default value to return if the key is not found, preventing the error.
Is there a method to remove multiple keys from a dictionary at once?
Python does not provide a built-in method to remove multiple keys directly. You can use a loop to iterate over a list of keys and remove each one individually, or use dictionary comprehension to create a new dictionary excluding the unwanted keys.
Can I remove a key while iterating through a dictionary?
It is not safe to modify a dictionary while iterating over it. Instead, create a list of keys to remove and then iterate over that list to delete the keys after the iteration.
What is the difference between `pop()` and `popitem()` methods?
The `pop()` method removes a specified key and returns its value, while `popitem()` removes and returns the last inserted key-value pair as a tuple. If the dictionary is empty, `popitem()` will raise a `KeyError`.
How do I check if a key exists before removing it?
You can use the `in` keyword to check for a key’s existence. For example, `if key in my_dict:` allows you to safely proceed with removal without encountering a `KeyError`.
Removing a key from a dictionary in Python can be accomplished using several methods, each with its own use case and implications. The most common methods include using the `del` statement, the `pop()` method, and the `popitem()` method. Each of these methods allows for the removal of keys, but they differ in their behavior and the way they handle the absence of the specified key.
The `del` statement is a straightforward way to remove a key-value pair from a dictionary. It raises a `KeyError` if the key does not exist, which means it is essential to ensure the key is present before using this method. On the other hand, the `pop()` method not only removes the specified key but also returns its associated value. This method can also take a second argument, which serves as a default value if the key is not found, thus avoiding potential errors.
Another method, `popitem()`, is useful when you want to remove the last inserted key-value pair from the dictionary. This method is particularly beneficial in scenarios where the order of insertion matters, especially in Python versions 3.7 and later, where dictionaries maintain insertion order. Understanding the nuances of these methods allows developers to choose the most appropriate
Author Profile
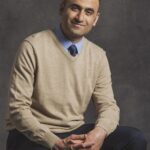
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?