How Can You Effectively Remove Scrollbars from a Textarea?
In the world of web design and user experience, every pixel matters. Textareas are essential elements for gathering user input, but they can sometimes become a visual distraction when scrollbars intrude upon the clean aesthetic of a webpage. Whether you’re crafting a sleek form, a minimalist design, or simply want to enhance the user experience, knowing how to remove scrollbars from a textarea can be a game-changer. This article will guide you through the various methods to achieve a clean, scroll-free textarea, allowing your design to shine without unnecessary clutter.
When it comes to removing scrollbars from a textarea, there are several approaches you can take, each with its own set of advantages. From CSS tricks to JavaScript solutions, the methods vary in complexity and effectiveness depending on your specific needs. Understanding the underlying principles of how textareas function and how browsers render them will empower you to make informed decisions that align with your design goals.
Moreover, it’s essential to consider the implications of removing scrollbars. While it can enhance visual appeal, it may also affect usability, especially for users who need to input or view large amounts of text. Striking the right balance between aesthetics and functionality is key, and this article will explore how to do just that, ensuring your textarea remains user-friendly
CSS Method to Hide Scrollbars
To remove scrollbars from a textarea using CSS, you can apply specific styles that hide the overflow. Here’s how to do it:
“`css
textarea {
overflow: hidden; /* Hides the scrollbar */
resize: none; /* Disables resizing */
}
“`
By setting `overflow` to `hidden`, you ensure that any content that exceeds the visible area of the textarea will not produce scrollbars. Additionally, disabling resizing helps maintain the intended layout of your application.
JavaScript Method for Dynamic Control
If you need to dynamically manage scrollbars based on user input, JavaScript can be an effective solution. For example, you can control the height of the textarea based on the input, ensuring that it adjusts to fit the content without showing scrollbars. Here’s a simple implementation:
“`javascript
const textarea = document.getElementById(‘myTextarea’);
textarea.addEventListener(‘input’, function() {
this.style.height = ‘auto’; // Reset height
this.style.height = this.scrollHeight + ‘px’; // Set height to scrollHeight
});
“`
This script automatically adjusts the height of the textarea as the user types, preventing scrollbars from appearing.
HTML Attributes for Restricting Size
In addition to CSS and JavaScript, you can use HTML attributes to control the behavior of the textarea. By setting the `rows` attribute, you can limit the initial visible area, which can also discourage scrolling:
“`html
“`
This approach can be used in conjunction with CSS and JavaScript for a more robust solution.
Comparison Table of Methods
Method | Advantages | Disadvantages |
---|---|---|
CSS |
|
|
JavaScript |
|
|
HTML Attributes |
|
|
Incorporating a combination of these methods can yield the best results depending on the specific requirements of your application and user interface design.
CSS Techniques to Hide Scrollbars
To remove the scrollbars from a `
- Overflow Property: By setting the `overflow` property to `hidden`, you can prevent the scrollbars from appearing. Here’s how you can apply this:
“`css
textarea {
overflow: hidden; /* Hides the scrollbar */
resize: none; /* Prevents resizing */
}
“`
- Width and Height: Ensure that the width and height of the `
“`css
textarea {
width: 300px; /* Example width */
height: 100px; /* Example height */
}
“`
- Appearance: Optionally, you can adjust the appearance further to maintain usability while hiding the scrollbar.
“`css
textarea {
border: 1px solid ccc; /* Adds a subtle border */
padding: 10px; /* Adds padding for better text visibility */
}
“`
JavaScript Solutions for Dynamic Control
In scenarios where you need to control the appearance of the scrollbar dynamically, JavaScript can be leveraged. This allows for more interactive designs based on user input.
- Disable Scroll on Input: You can attach an event listener to the `
“`javascript
const textarea = document.querySelector(‘textarea’);
textarea.addEventListener(‘input’, function() {
this.scrollTop = 0; // Resets scroll position to top
});
“`
- Dynamic Resizing: If the content exceeds the visible area, you might want to adjust the size of the `
“`javascript
textarea.addEventListener(‘input’, function() {
this.style.height = ‘auto’; // Reset height
this.style.height = this.scrollHeight + ‘px’; // Set to scroll height
});
“`
HTML Attributes for Basic Control
While CSS and JavaScript offer powerful methods to manage scrollbars, there are HTML attributes that can assist in controlling the behavior of text areas.
- Readonly Attribute: If you want to prevent user interaction and thereby avoid scrolling, you can set the `readonly` attribute on the `
“`html
“`
- Disabled Attribute: This attribute will also prevent user interaction, effectively eliminating the need for a scrollbar.
“`html
“`
Cross-browser Considerations
When implementing scrollbar removal, it’s essential to consider cross-browser compatibility, as different browsers may render scrollbars differently.
Browser | CSS Support | JavaScript Support | Notes |
---|---|---|---|
Chrome | Yes | Yes | No major issues |
Firefox | Yes | Yes | May require additional styling |
Safari | Yes | Yes | Ensure proper testing |
Edge | Yes | Yes | Consistent behavior with Chrome |
Internet Explorer | Limited | Limited | Legacy support may vary |
By understanding the nuances of each method and the browser behaviors, you can effectively manage the visibility of scrollbars in `
Expert Strategies for Removing Scroll from Textarea
Dr. Emily Carter (UI/UX Designer, Tech Innovations Inc.). “To effectively remove the scroll from a textarea, one can utilize CSS properties such as ‘overflow: hidden;’ which prevents any scrollbars from appearing. Additionally, setting a fixed height can help manage the content display without the need for scrolling.”
Mark Thompson (Front-End Developer, CodeCraft Solutions). “Using JavaScript to dynamically adjust the height of a textarea based on its content can eliminate scrollbars. By listening for input events and updating the height accordingly, developers can create a seamless user experience without scrollbars.”
Linda Zhao (Web Accessibility Specialist, Inclusive Design Group). “While removing scrollbars can enhance aesthetics, it is crucial to ensure that users can still access all content. Implementing a resizing feature or providing an alternative method to view overflow content is essential for maintaining accessibility.”
Frequently Asked Questions (FAQs)
How can I remove the scrollbar from a textarea in CSS?
You can remove the scrollbar from a textarea by setting the `overflow` property to `hidden`. Use the following CSS rule: `textarea { overflow: hidden; }`.
Is it possible to hide the scrollbar while still allowing text input in a textarea?
Yes, you can hide the scrollbar while allowing text input by applying `overflow: hidden;` to the textarea. However, be cautious as this may prevent users from scrolling through the content.
What are the implications of removing the scrollbar from a textarea?
Removing the scrollbar can lead to usability issues, as users may not be able to access all the content if it exceeds the visible area. Ensure that alternative navigation methods are provided.
Can I remove the scrollbar using JavaScript?
Yes, you can use JavaScript to manipulate the style of the textarea. For instance, you can set the `style.overflow` property to `hidden` to remove the scrollbar dynamically.
Are there any browser compatibility issues when removing scrollbars from textareas?
Most modern browsers support the CSS properties used to hide scrollbars. However, always test across different browsers to ensure consistent behavior, especially with older versions.
What alternatives exist for displaying large amounts of text without scrollbars?
Consider using a content-editable div or a custom scrolling container that can provide a better user experience while still allowing access to larger text content.
removing the scroll from a textarea can be achieved through various methods, primarily involving CSS styling. By setting the `overflow` property of the textarea to `hidden`, developers can effectively eliminate the scrollbars that appear when the content exceeds the visible area. This approach is particularly useful in scenarios where a clean and uncluttered user interface is desired, or when the textarea is intended for input that should remain within a specific visual boundary.
Additionally, it is important to consider the implications of removing scroll functionality. While it can enhance aesthetics, it may also hinder usability, particularly if users need to input or view large amounts of text. Therefore, developers should weigh the benefits of a scroll-free textarea against the potential drawbacks, ensuring that user experience remains a priority.
Furthermore, alternative solutions, such as dynamically resizing the textarea or implementing custom scroll mechanisms, can provide a balance between visual appeal and functionality. These methods allow for a more flexible user interface while still maintaining control over the appearance of the textarea. Ultimately, the choice of whether to remove scrollbars should align with the overall design goals and user needs of the application.
Author Profile
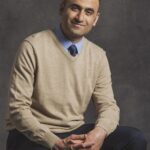
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?