How Can You Rename a Column in Python?
Renaming a column in Python is a fundamental yet essential skill for anyone working with data. Whether you’re a data analyst, scientist, or a developer, mastering this technique can significantly enhance your data manipulation abilities. Python, with its rich ecosystem of libraries such as Pandas, provides powerful tools that simplify the process of data handling. As datasets grow in complexity, the need for clear and meaningful column names becomes increasingly important. This article will guide you through the various methods to efficiently rename columns, empowering you to clean and organize your data like a pro.
At its core, renaming a column in Python involves understanding the structure of your dataset and the tools available to manipulate it. Python’s Pandas library is particularly popular for data analysis, offering straightforward functions to alter column names with ease. Whether you’re looking to correct a typo, standardize naming conventions, or make your data more readable, the process is both intuitive and flexible.
Moreover, renaming columns can be done in several ways, each suited to different scenarios. From simple one-liners to more complex operations involving multiple columns, Python provides the versatility needed to adapt to your specific requirements. As we delve deeper, you’ll discover practical examples and tips that will not only enhance your coding skills but also improve the clarity and usability of your
Renaming Columns in Pandas
Pandas is a powerful library in Python for data manipulation and analysis, often used for working with tabular data. Renaming columns in a DataFrame is a common task that can be accomplished with ease.
To rename a column, you can use the `rename()` method. This method allows you to specify a dictionary where the keys are the current column names and the values are the new column names. Below is an example:
“`python
import pandas as pd
Sample DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6]
})
Renaming columns
df.rename(columns={‘A’: ‘Column1’, ‘B’: ‘Column2’}, inplace=True)
“`
In this example, the columns ‘A’ and ‘B’ are renamed to ‘Column1’ and ‘Column2’, respectively. The `inplace=True` argument ensures that the changes are made directly to the original DataFrame.
Additionally, you can rename columns by assigning a new list of column names directly to the `columns` attribute of the DataFrame. This method is straightforward but requires you to provide a complete list of column names:
“`python
Renaming columns by assigning a new list
df.columns = [‘NewCol1’, ‘NewCol2’]
“`
Renaming Columns with the set_axis Method
Another approach to rename columns is to use the `set_axis()` method. This method can be useful when you want to change the names while keeping the original index:
“`python
Using set_axis method
df.set_axis([‘UpdatedCol1’, ‘UpdatedCol2’], axis=1, inplace=True)
“`
This method provides flexibility, particularly when you are transforming the DataFrame in various ways.
Renaming Columns Based on Conditions
Sometimes, renaming columns based on specific conditions is required. For example, you may want to rename columns that contain certain substrings. You can achieve this using a combination of the `map()` function and a lambda function:
“`python
Renaming columns conditionally
df.columns = df.columns.map(lambda x: ‘New_’ + x if ‘Col’ in x else x)
“`
This code snippet prepends ‘New_’ to any column name that contains ‘Col’, demonstrating the versatility of column renaming in Pandas.
Comparison of Methods
The following table summarizes the different methods for renaming columns in Pandas, including their advantages:
Method | Description | Advantages |
---|---|---|
rename() | Renames specified columns using a dictionary. | Intuitive, allows selective renaming. |
columns attribute | Assigns a new list of column names directly. | Simpler for renaming all columns at once. |
set_axis() | Sets new column names while preserving the index. | Flexible in modifying existing names. |
map() with lambda | Applies a function to modify column names based on conditions. | Powerful for dynamic renaming based on patterns. |
Each method has its use cases, and the choice of which to use depends on the specific requirements of your data manipulation tasks.
Renaming Columns in Pandas DataFrames
Pandas is a popular library in Python for data manipulation and analysis. Renaming columns in a Pandas DataFrame is a common task that can be accomplished in several ways.
Using the `rename()` Method
The `rename()` method is a straightforward way to change the names of one or more columns. This method allows you to specify a dictionary where the keys are the current column names and the values are the new names.
“`python
import pandas as pd
Sample DataFrame
df = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
Renaming columns
df.rename(columns={‘A’: ‘Column1’, ‘B’: ‘Column2’}, inplace=True)
print(df)
“`
- Parameters:
- `columns`: A dictionary mapping current column names to new column names.
- `inplace`: If `True`, modifies the original DataFrame. If “, returns a new DataFrame.
Assigning to `columns` Attribute
Another method to rename all columns at once is by directly assigning a new list of column names to the DataFrame’s `columns` attribute.
“`python
df.columns = [‘NewColumn1’, ‘NewColumn2’]
print(df)
“`
- Considerations: The number of new names must match the number of existing columns.
Using `set_axis()` Method
The `set_axis()` method can also be used to rename columns. This method allows you to set new column names along with specifying the axis.
“`python
df.set_axis([‘First’, ‘Second’], axis=1, inplace=True)
print(df)
“`
- Parameters:
- `labels`: A list of new column names.
- `axis`: Specify `0` for index and `1` for columns.
- `inplace`: Modifies the original DataFrame if set to `True`.
Renaming Columns with List Comprehension
For more advanced renaming, such as applying a function to modify the column names, list comprehension can be useful.
“`python
df.columns = [col.lower() for col in df.columns]
print(df)
“`
- Use Case: This approach is helpful for standardizing column names, such as converting them all to lowercase.
Examples of Common Renaming Scenarios
Scenario | Method Used | Code Example |
---|---|---|
Rename specific columns | `rename()` | `df.rename(columns={‘A’: ‘X’, ‘B’: ‘Y’}, inplace=True)` |
Rename all columns | Assigning to `columns` | `df.columns = [‘X’, ‘Y’]` |
Rename with a function | List comprehension | `df.columns = [col.upper() for col in df.columns]` |
Rename using `set_axis()` | `set_axis()` | `df.set_axis([‘X’, ‘Y’], axis=1, inplace=True)` |
These methods provide flexibility in renaming DataFrame columns in Python using the Pandas library. By selecting the appropriate method, you can achieve your data manipulation objectives effectively.
Expert Insights on Renaming Columns in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Renaming columns in Python, particularly using libraries like Pandas, is a straightforward process. Utilizing the `rename()` function allows for clear and efficient column name changes, enhancing data readability and management.”
Michael Chen (Software Engineer, CodeCraft Solutions). “When working with data frames in Python, it’s crucial to maintain clarity in your column names. The `rename()` method not only allows you to change names but can also be used in conjunction with other data manipulation techniques for streamlined data processing.”
Sarah Johnson (Python Programming Instructor, LearnPython Academy). “For beginners, understanding how to rename columns in Python can significantly improve their coding efficiency. I recommend practicing with the `rename()` function as it provides a solid foundation for more complex data handling tasks.”
Frequently Asked Questions (FAQs)
How do I rename a column in a pandas DataFrame?
To rename a column in a pandas DataFrame, use the `rename()` method. For example: `df.rename(columns={‘old_name’: ‘new_name’}, inplace=True)`.
Can I rename multiple columns at once in pandas?
Yes, you can rename multiple columns by passing a dictionary to the `columns` parameter in the `rename()` method. For example: `df.rename(columns={‘old_name1’: ‘new_name1’, ‘old_name2’: ‘new_name2’}, inplace=True)`.
What is the easiest way to rename columns when creating a DataFrame?
You can specify column names directly when creating a DataFrame by using the `columns` parameter. For example: `pd.DataFrame(data, columns=[‘new_name1’, ‘new_name2’])`.
Is there a method to rename columns in a DataFrame without using `rename()`?
Yes, you can directly assign a new list of column names to the `columns` attribute of the DataFrame. For example: `df.columns = [‘new_name1’, ‘new_name2’]`.
How can I rename columns in a DataFrame using the `set_axis()` method?
You can use the `set_axis()` method to rename columns by passing a new list of column names and specifying the `axis` parameter. For example: `df.set_axis([‘new_name1’, ‘new_name2’], axis=1, inplace=True)`.
Can I rename columns in a DataFrame based on a condition?
Yes, you can rename columns based on a condition by using a list comprehension. For example: `df.columns = [col if condition else ‘new_name’ for col in df.columns]`.
Renaming a column in Python is a common task when working with data, particularly when using libraries like Pandas. The primary method to achieve this involves utilizing the `rename()` function, which allows users to specify the existing column name and the new name they wish to assign. This function is versatile and can be applied to either a single column or multiple columns at once, making it a powerful tool for data manipulation.
Another approach to renaming columns is by directly assigning a new list of column names to the DataFrame’s `columns` attribute. This method is straightforward but requires that the new list matches the number of existing columns. It is particularly useful when a complete overhaul of column names is needed, ensuring clarity and consistency in the dataset.
In summary, whether using the `rename()` function for targeted changes or the `columns` attribute for broader modifications, Python provides flexible options for renaming columns. These methods enhance data readability and facilitate better data analysis, underscoring the importance of clear and descriptive column names in data science workflows.
Author Profile
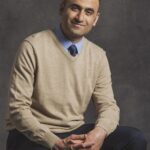
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?