How Can You Easily Rename a File in Python?
Renaming files is a fundamental task in programming that can often be overlooked, yet it plays a crucial role in maintaining organization and clarity within your projects. Whether you’re managing a collection of images, processing data files, or simply tidying up your workspace, knowing how to rename files efficiently in Python can save you time and enhance your workflow. In this article, we will explore the various methods and best practices for renaming files using Python, empowering you to handle your file management tasks with ease and confidence.
Python, with its straightforward syntax and powerful libraries, offers several ways to rename files. The built-in `os` module is one of the most commonly used tools for this purpose, allowing you to interact with the operating system and manipulate files seamlessly. Additionally, the `pathlib` module provides a more modern approach to file handling, making it easier to work with file paths in an object-oriented manner. Understanding these options will enable you to choose the method that best fits your specific needs.
As we delve deeper into the topic, we will cover practical examples and scenarios that illustrate how to effectively rename files in Python. From basic renaming tasks to more complex operations involving multiple files, you’ll gain insights into the techniques that can streamline your coding process. Whether you’re a beginner or an experienced developer
Using the `os` Module
Renaming files in Python can be efficiently accomplished using the `os` module, which provides a portable way of using operating system-dependent functionality. The `os.rename()` function is specifically designed for this purpose. To rename a file, you need to specify the current filename and the new filename.
Here’s a simple example of how to use the `os` module to rename a file:
“`python
import os
Current file name
current_name = ‘old_filename.txt’
New file name
new_name = ‘new_filename.txt’
Renaming the file
os.rename(current_name, new_name)
“`
It’s important to ensure that the file you want to rename exists in the specified directory; otherwise, you will encounter a `FileNotFoundError`. Additionally, if the target filename already exists, Python will overwrite it without any warning.
Using the `pathlib` Module
The `pathlib` module, introduced in Python 3.4, offers an object-oriented approach to handling filesystem paths. The `Path` class provides a method called `rename()` which can also be utilized to rename files.
Here’s how to rename a file using `pathlib`:
“`python
from pathlib import Path
Specify the file path
file_path = Path(‘old_filename.txt’)
New file path
new_file_path = Path(‘new_filename.txt’)
Renaming the file
file_path.rename(new_file_path)
“`
This method is more intuitive and makes code easier to read. Like the `os` module, `pathlib` will also throw an error if the file does not exist or if the new filename already exists.
Handling Exceptions
When renaming files, it is crucial to handle potential exceptions that may arise during the process. Using a try-except block can help manage errors gracefully.
“`python
import os
current_name = ‘old_filename.txt’
new_name = ‘new_filename.txt’
try:
os.rename(current_name, new_name)
print(f’Renamed {current_name} to {new_name}’)
except FileNotFoundError:
print(f’Error: {current_name} does not exist.’)
except FileExistsError:
print(f’Error: {new_name} already exists.’)
except Exception as e:
print(f’An unexpected error occurred: {e}’)
“`
Comparison of Methods
Below is a comparison table of the two methods for renaming files in Python:
Method | Module | Advantages | Disadvantages |
---|---|---|---|
os.rename() | os | – Simple to use – Well-established |
– Less readable for complex paths |
Path.rename() | pathlib | – Object-oriented – More readable syntax |
– Requires Python 3.4 or newer |
Both methods are effective for renaming files, and the choice between them often comes down to personal preference and the specific requirements of the project at hand.
Using the `os` Module
The `os` module in Python provides a straightforward way to rename files. The `os.rename()` function takes two arguments: the current filename and the new filename.
“`python
import os
Renaming a file
os.rename(‘old_filename.txt’, ‘new_filename.txt’)
“`
Important Considerations
- Ensure that the file you want to rename exists in the specified path.
- If the new filename already exists, it will be overwritten without warning.
- Proper error handling should be implemented to catch exceptions.
Using the `pathlib` Module
The `pathlib` module is a modern way to handle filesystem paths and offers an object-oriented approach. Renaming a file can be achieved with the `rename()` method of a `Path` object.
“`python
from pathlib import Path
Creating a Path object
file_path = Path(‘old_filename.txt’)
Renaming the file
file_path.rename(‘new_filename.txt’)
“`
Advantages of `pathlib`
- More intuitive syntax for file operations.
- Cross-platform compatibility is better managed through path objects.
- Enhanced readability of the code.
Error Handling
When renaming files, various errors may arise. Implementing error handling is crucial to avoid program crashes and to provide informative feedback to users.
“`python
import os
try:
os.rename(‘old_filename.txt’, ‘new_filename.txt’)
except FileNotFoundError:
print(“The file does not exist.”)
except PermissionError:
print(“You do not have permission to rename this file.”)
except Exception as e:
print(f”An error occurred: {e}”)
“`
Common Exceptions
Exception | Description |
---|---|
FileNotFoundError | Raised when the specified file does not exist. |
PermissionError | Raised when the program lacks permissions to rename the file. |
OSError | General error for issues related to the operating system. |
Renaming Files in a Directory
To rename multiple files within a directory, you can use loops combined with the renaming methods. Below is an example using `os` to rename all `.txt` files by appending a suffix.
“`python
import os
Directory containing the files
directory = ‘path/to/directory/’
for filename in os.listdir(directory):
if filename.endswith(‘.txt’):
old_file = os.path.join(directory, filename)
new_file = os.path.join(directory, f”{filename[:-4]}_new.txt”)
os.rename(old_file, new_file)
“`
Tips for Bulk Renaming
- Always make a backup of files before bulk operations.
- Use clear and consistent naming conventions to avoid confusion.
- Test your renaming script with a few files before applying it to a larger dataset.
Conclusion on File Renaming Methods
Utilizing either the `os` or `pathlib` module provides effective methods for renaming files in Python. By implementing error handling and considering bulk renaming strategies, you can enhance the robustness and usability of your file management scripts.
Expert Insights on Renaming Files in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). Renaming files in Python can be efficiently accomplished using the `os` module, specifically the `os.rename()` function. This method allows developers to specify both the current file name and the new name, ensuring a straightforward approach to file management.
Michael Chen (Lead Python Developer, Tech Innovations Inc.). It is essential to handle exceptions when renaming files in Python. Utilizing a try-except block can prevent your program from crashing due to issues like file not found or permission errors, which are common when working with file systems.
Sarah Patel (Data Scientist, AI Insights Group). When renaming files in bulk, consider using loops in conjunction with the `os` module. This approach can automate the renaming process and is particularly useful for organizing datasets or managing large collections of files efficiently.
Frequently Asked Questions (FAQs)
How can I rename a file in Python?
You can rename a file in Python using the `os` module’s `rename()` function. For example, `os.rename(‘old_filename.txt’, ‘new_filename.txt’)` will change the name of the specified file.
What if the file I want to rename does not exist?
If the file does not exist, the `rename()` function will raise a `FileNotFoundError`. Ensure that the file path is correct before attempting to rename it.
Can I rename a file to a different directory in Python?
Yes, you can rename a file and move it to a different directory in one operation by specifying the new path in the `rename()` function. For example, `os.rename(‘old_path/old_filename.txt’, ‘new_path/new_filename.txt’)` will move and rename the file.
Is it possible to rename multiple files at once in Python?
You can rename multiple files using a loop. For instance, you can iterate over a list of filenames and apply the `rename()` function to each one, allowing for batch renaming.
What permissions are required to rename a file in Python?
You must have write permissions for the directory containing the file you wish to rename. If you lack the necessary permissions, a `PermissionError` will be raised.
Are there any libraries other than `os` to rename files in Python?
Yes, you can use the `shutil` library, which provides a `move()` function that can also rename files. For example, `shutil.move(‘old_filename.txt’, ‘new_filename.txt’)` achieves the same result as `os.rename()`.
Renaming a file in Python is a straightforward task that can be accomplished using the built-in `os` module. The primary function utilized for this purpose is `os.rename()`, which takes two arguments: the current file name and the new file name. This function effectively changes the name of the specified file, provided that the file exists and the user has the necessary permissions to make changes to it.
It is essential to handle potential exceptions that may arise during the renaming process. Common issues include the file not existing, permission errors, or attempting to rename a file to a name that already exists. Utilizing try-except blocks can help manage these exceptions gracefully, ensuring that the program does not crash and that the user receives informative feedback regarding any errors encountered.
Furthermore, when renaming files, it is advisable to consider the file’s path. If the file is not in the current working directory, the full path must be specified. This attention to detail prevents confusion and ensures that the correct file is targeted for renaming. Overall, understanding these fundamental aspects of file renaming in Python allows developers to implement effective file management practices in their applications.
Author Profile
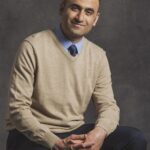
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?