How Can You Rename a Column in Python?
Renaming columns in Python is a fundamental yet essential skill for anyone working with data, whether you’re a seasoned data scientist or a beginner exploring the world of data manipulation. As datasets grow in complexity, clarity becomes paramount. A well-named column can enhance readability, improve data management, and streamline analysis, making your work not only more efficient but also more impactful. In this article, we will delve into the various methods available for renaming columns in Python, equipping you with the tools you need to transform your data effortlessly.
Python offers a variety of libraries tailored for data manipulation, with Pandas being the most prominent among them. This powerful library provides intuitive functions that allow users to easily rename columns in a DataFrame, catering to different needs and preferences. Whether you’re looking to rename a single column or multiple columns at once, understanding the syntax and options available can significantly enhance your data processing workflow.
Beyond just the mechanics of renaming, it’s important to consider the best practices that can make your datasets more understandable and maintainable. From ensuring consistency in naming conventions to avoiding common pitfalls, this article will guide you through the nuances of column renaming in Python, empowering you to handle your data with confidence and precision. Get ready to unlock the potential of your datasets as we explore
Using Pandas to Rename Columns
Pandas is a powerful library in Python commonly used for data manipulation and analysis, particularly in tabular data formats such as DataFrames. Renaming columns in a DataFrame is straightforward and can be achieved using the `rename()` method or by directly assigning a new list of column names.
To rename columns using the `rename()` method, you can pass a dictionary mapping the old column names to the new names. Here’s an example:
“`python
import pandas as pd
Sample DataFrame
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6]
}
df = pd.DataFrame(data)
Renaming columns
df.rename(columns={‘A’: ‘Column1’, ‘B’: ‘Column2’}, inplace=True)
“`
In this example, the columns ‘A’ and ‘B’ are renamed to ‘Column1’ and ‘Column2’, respectively. The `inplace=True` parameter modifies the original DataFrame directly.
Alternatively, if you want to rename all columns at once, you can assign a new list to the `columns` attribute of the DataFrame:
“`python
Renaming all columns
df.columns = [‘First’, ‘Second’]
“`
This method is effective when you want to replace all the column names in one go.
Renaming Columns with a Function
You can also rename columns using a function applied to the existing column names. This is particularly useful for consistent formatting, such as converting all names to lowercase or replacing spaces with underscores.
Here’s how to do it:
“`python
Renaming columns using a function
df.columns = df.columns.str.lower().str.replace(‘ ‘, ‘_’)
“`
This approach is beneficial when dealing with datasets where column names may vary in case or formatting.
Comparative Summary of Methods
Below is a comparison of the different methods to rename columns in a Pandas DataFrame:
Method | Description | Example |
---|---|---|
rename() | Rename specific columns using a dictionary. | df.rename(columns={‘old_name’: ‘new_name’}) |
Assigning a list | Replace all column names with a new list. | df.columns = [‘new_name1’, ‘new_name2’] |
Using a function | Apply a function to modify existing column names. | df.columns = df.columns.str.lower() |
Understanding how to rename columns effectively allows for better data management and analysis in Python. By leveraging the versatility of the Pandas library, you can ensure that your DataFrame’s column names are clear, consistent, and meaningful, facilitating easier data manipulation and interpretation.
Renaming Columns in Pandas DataFrames
Pandas is a powerful data manipulation library in Python, commonly used for data analysis. Renaming columns in a DataFrame can be accomplished using various methods. Below are the most common techniques to achieve this.
Using the `rename()` Method
The `rename()` method allows for renaming specific columns by providing a dictionary where the keys are the current column names and the values are the new column names.
“`python
import pandas as pd
Sample DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6]
})
Renaming columns
df.rename(columns={‘A’: ‘Alpha’, ‘B’: ‘Beta’}, inplace=True)
“`
- Parameters:
- `columns`: A dictionary mapping current names to new names.
- `inplace`: If `True`, modifies the DataFrame in place.
Assigning New Column Names Directly
If you need to rename all columns, you can assign a new list of column names directly to the `columns` attribute of the DataFrame.
“`python
df.columns = [‘New_Alpha’, ‘New_Beta’]
“`
- Considerations: Ensure the new list matches the length of the existing column names.
Using List Comprehension for Conditional Renaming
For more complex renaming scenarios, such as adding a prefix or suffix to existing column names, list comprehension can be utilized.
“`python
df.columns = [f’prefix_{col}’ for col in df.columns]
“`
- Example: This adds the prefix “prefix_” to each column name.
Renaming Columns with `set_axis()` Method
The `set_axis()` method can also be used to rename columns, allowing you to specify the axis along with the new names.
“`python
df.set_axis([‘First’, ‘Second’], axis=1, inplace=True)
“`
- Parameters:
- `labels`: List of new column names.
- `axis`: Set to `1` for columns or `0` for rows.
Using `add_prefix()` and `add_suffix()` Methods
If you want to add a prefix or suffix to all column names, Pandas provides `add_prefix()` and `add_suffix()` methods.
“`python
df = df.add_prefix(‘new_’)
or
df = df.add_suffix(‘_old’)
“`
- Use Cases: These methods are useful when adjusting names for clarity or categorization without renaming each column individually.
Example of Renaming Columns
Here is a practical example demonstrating several renaming techniques in a single script:
“`python
import pandas as pd
Create a DataFrame
data = {‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [25, 30]}
df = pd.DataFrame(data)
Using rename()
df.rename(columns={‘Name’: ‘First_Name’}, inplace=True)
Assign new column names directly
df.columns = [‘First_Name’, ‘Years’]
Using add_prefix
df = df.add_prefix(‘User_’)
Final DataFrame
print(df)
“`
User_First_Name | User_Years |
---|---|
Alice | 25 |
Bob | 30 |
This example showcases different methods for renaming columns while maintaining clarity in your DataFrame’s structure. Each technique has its advantages depending on the specific needs of your data manipulation tasks.
Expert Insights on Renaming Columns in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “Renaming columns in Python is often accomplished using the Pandas library, which provides a straightforward method. The `rename()` function allows for clear and concise renaming of columns by passing a dictionary that maps old names to new ones. This method not only enhances code readability but also ensures that data manipulation remains efficient and organized.”
Michael Chen (Software Engineer, DataTech Solutions). “When working with large datasets, it is crucial to maintain clarity in column names. Using the `rename()` method in Pandas is a best practice that helps prevent confusion. Additionally, for quick renaming, one can directly assign a new list of column names to the `columns` attribute of the DataFrame. This approach can be particularly useful when renaming multiple columns at once.”
Jessica Patel (Python Developer, CodeCraft). “In Python, renaming columns is not just about changing names; it is about enhancing data quality. The `rename()` function is versatile, allowing for both single and batch renaming. Moreover, using this function with the `inplace=True` parameter can streamline the process by modifying the DataFrame directly, which is beneficial in scenarios where memory efficiency is a concern.”
Frequently Asked Questions (FAQs)
How can I rename a column in a Pandas DataFrame?
You can rename a column in a Pandas DataFrame using the `rename()` method. For example: `df.rename(columns={‘old_name’: ‘new_name’}, inplace=True)`.
Is it possible to rename multiple columns at once in Python?
Yes, you can rename multiple columns by passing a dictionary to the `rename()` method. For example: `df.rename(columns={‘old_name1’: ‘new_name1’, ‘old_name2’: ‘new_name2’}, inplace=True)`.
What is the syntax for renaming columns using the `set_axis()` method?
You can use the `set_axis()` method to rename columns by providing a list of new column names. For example: `df.set_axis([‘new_name1’, ‘new_name2’], axis=1, inplace=True)`.
Can I rename columns in a NumPy array?
NumPy arrays do not have named columns. However, you can convert a NumPy array to a Pandas DataFrame and then rename the columns using the methods mentioned above.
How do I rename columns in a CSV file after loading it into Python?
After loading a CSV file into a Pandas DataFrame using `pd.read_csv()`, you can rename the columns using the `rename()` method or by directly assigning a new list of names to `df.columns`.
What happens if I try to rename a column that does not exist?
If you attempt to rename a column that does not exist, Pandas will raise a `KeyError`. It is advisable to check the existing column names before renaming.
Renaming columns in Python is a common task, especially when working with data manipulation libraries such as pandas. The most straightforward method to rename columns is by using the `rename()` function, which allows users to specify a dictionary mapping of old column names to new ones. This method provides flexibility and clarity, enabling precise changes without altering the entire DataFrame structure.
Another effective approach to rename columns is by directly assigning a new list of column names to the `columns` attribute of a DataFrame. This method is particularly useful when the user wants to rename all columns simultaneously. It is essential to ensure that the new list of names matches the length of the existing columns to avoid errors.
Additionally, utilizing the `set_axis()` method offers a versatile option for renaming columns. This method allows for renaming along with the option to modify the axis in place. Each of these methods has its advantages, and the choice between them often depends on the specific requirements of the task at hand.
renaming columns in Python can be efficiently accomplished using various methods provided by libraries like pandas. Understanding these methods enhances data manipulation capabilities and promotes better organization of data. By selecting the appropriate method, users can ensure their DataFrame
Author Profile
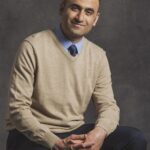
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?