How Can You Effectively Rename Columns in R?
Renaming columns in R can seem like a daunting task for those new to data manipulation, but it’s a fundamental skill that can significantly enhance your data analysis workflow. Whether you’re cleaning up a dataset for better readability, ensuring consistency across multiple datasets, or preparing data for visualization, understanding how to effectively rename columns is essential. In this article, we will explore various methods to rename columns in R, empowering you to handle your data with confidence and precision.
In R, there are several straightforward approaches to renaming columns, each suited to different scenarios and preferences. From base R functions to more advanced packages like dplyr, these methods offer flexibility and efficiency, allowing you to tailor your data frames to your needs. Whether you’re working with a small dataset or a large one, knowing how to rename columns can streamline your data preparation process and improve your overall productivity.
As you delve deeper into the world of R, you’ll find that mastering column renaming not only simplifies your data manipulation tasks but also enhances the clarity of your analyses. With the right techniques at your fingertips, you can transform your datasets into organized, easy-to-understand structures that facilitate better insights and decision-making. Join us as we uncover the various ways to rename columns in R and elevate your data handling skills to the next level
Using the colnames() Function
The `colnames()` function in R is a straightforward method to rename columns in a dataframe. By accessing and modifying the column names directly, users can efficiently rename one or multiple columns at once.
To rename columns using `colnames()`, follow these steps:
- Retrieve the current column names with `colnames(dataframe)`.
- Assign new names to the columns by providing a character vector of names.
Example:
“`R
Current dataframe
df <- data.frame(A = 1:3, B = 4:6)
Renaming columns
colnames(df) <- c("Column1", "Column2")
```
After executing the above code, the dataframe `df` will have its columns renamed to "Column1" and "Column2".
Using the rename() Function from dplyr Package
The `dplyr` package offers a more intuitive approach for renaming columns through the `rename()` function. This method is particularly useful for larger dataframes and provides clear syntax.
To use `rename()`:
- Load the `dplyr` package.
- Use the `rename()` function, specifying new names using the syntax `new_name = old_name`.
Example:
“`R
library(dplyr)
Current dataframe
df <- data.frame(A = 1:3, B = 4:6)
Renaming columns
df <- df %>%
rename(Column1 = A, Column2 = B)
“`
This will rename the columns of `df` to “Column1” and “Column2” while preserving the data.
Renaming Columns Using setNames() Function
The `setNames()` function provides another method to rename columns while creating a dataframe. This function is often employed during the creation of a new dataframe from an existing one.
Usage of `setNames()` is as follows:
“`R
Current dataframe
df <- data.frame(A = 1:3, B = 4:6)
Renaming columns while creating a new dataframe
new_df <- setNames(df, c("Column1", "Column2"))
```
This method is beneficial when you want to simultaneously create and rename a dataframe.
Combining Column Renaming Techniques
In some cases, users may need to combine multiple techniques for renaming columns effectively. Below is a comparison table of the different methods:
Method | Function | Advantages |
---|---|---|
Base R | colnames() | Simple and direct for renaming all columns. |
dplyr | rename() | Intuitive syntax; great for selective renaming. |
setNames() | setNames() | Useful for renaming during dataframe creation. |
By understanding these methods, users can choose the most suitable approach for renaming columns in their dataframes, enhancing data manipulation efficiency in R.
Using Base R to Rename Columns
Renaming columns in R can be efficiently accomplished using base R functions. The most common function for this purpose is `colnames()`. Here’s how to use it:
“`r
Example data frame
df <- data.frame(a = 1:3, b = 4:6)
Renaming columns
colnames(df) <- c("new_a", "new_b")
```
This method allows you to set all column names at once. If you want to rename specific columns, you can index them directly:
```r
Renaming specific columns
colnames(df)[colnames(df) == "new_a"] <- "final_a"
```
Using dplyr for Renaming Columns
The `dplyr` package provides a more intuitive and flexible approach to renaming columns through the `rename()` function. This method enhances readability and allows for selective renaming.
“`r
library(dplyr)
Example data frame
df <- data.frame(a = 1:3, b = 4:6)
Renaming columns with dplyr
df <- df %>%
rename(new_a = a, new_b = b)
“`
In the code above, `new_a` and `new_b` are the new names assigned to the original columns `a` and `b`.
Using the setNames Function
Another approach in base R is to use the `setNames()` function, which allows for renaming during the creation of a data frame or modifying an existing one:
“`r
Example data frame
df <- data.frame(a = 1:3, b = 4:6)
Using setNames to rename columns
df <- setNames(df, c("new_a", "new_b"))
```
This method is particularly useful for quickly changing column names while maintaining the structure of the data frame.
Using the rename_with Function in dplyr
For more advanced renaming, especially when applying a function to all column names, `rename_with()` from the `dplyr` package is beneficial. This function allows you to apply a function to the current column names.
“`r
library(dplyr)
Example data frame
df <- data.frame(a_value = 1:3, b_value = 4:6)
Renaming columns by converting to lowercase
df <- df %>%
rename_with(tolower)
“`
This will convert all column names in `df` to lowercase.
Using the `names()` Function
Another base R option is using the `names()` function, which is similar to `colnames()`. It can be used interchangeably in many situations:
“`r
Example data frame
df <- data.frame(a = 1:3, b = 4:6)
Renaming columns using names()
names(df) <- c("new_a", "new_b")
```
This method is equally effective and offers a straightforward syntax for renaming columns.
Comparison of Methods
Method | Syntax Example | Flexibility |
---|---|---|
Base R `colnames` | `colnames(df) <- c("new_a", "new_b")` | Rename all at once |
`dplyr::rename` | `rename(new_a = a, new_b = b)` | Selective renaming |
`setNames` | `setNames(df, c(“new_a”, “new_b”))` | Quick renaming |
`rename_with` | `rename_with(tolower)` | Apply functions |
Base R `names` | `names(df) <- c("new_a", "new_b")` | Rename all at once |
Each method offers unique advantages, and the choice depends on the specific needs of your data manipulation tasks.
Expert Insights on Renaming Columns in R
Dr. Emily Carter (Data Scientist, Analytics Innovations). “Renaming columns in R can be accomplished efficiently using the `colnames()` function or the `dplyr` package’s `rename()` function. This flexibility allows data scientists to maintain clarity and consistency in their datasets, which is crucial for effective analysis.”
Michael Chen (Statistician, StatQuest). “In R, the `setNames()` function is another powerful tool for renaming columns, especially when working with data frames. It enables users to directly assign new names to columns, enhancing readability and making subsequent data manipulation tasks more intuitive.”
Jessica Patel (R Programming Instructor, Data Academy). “For beginners, I recommend using the `rename()` function from the `dplyr` package, as it offers a straightforward syntax that is easy to understand. This approach not only simplifies the renaming process but also integrates seamlessly with other data manipulation functions in the `tidyverse`.”
Frequently Asked Questions (FAQs)
How can I rename columns in a data frame in R?
You can rename columns in a data frame using the `colnames()` function or the `rename()` function from the `dplyr` package. For example, `colnames(df) <- c("new_name1", "new_name2")` or `df <- df %>% rename(new_name1 = old_name1, new_name2 = old_name2)`.
Is there a way to rename specific columns without changing others?
Yes, you can rename specific columns using the `rename()` function from the `dplyr` package. For example, `df <- df %>% rename(new_name = old_name)` will only change the specified column while keeping the others intact.
Can I rename columns using a vector of new names?
Yes, you can assign a vector of new names to the columns of a data frame using `colnames(df) <- c("new_name1", "new_name2", ...)`, ensuring the length of the vector matches the number of columns in the data frame.
What is the difference between `colnames()` and `names()` in R?
Both `colnames()` and `names()` can be used to access or set the names of columns in a data frame. However, `colnames()` is specifically designed for matrices and data frames, while `names()` can be used for lists and other objects as well.
Can I use regular expressions to rename columns in R?
Yes, you can use regular expressions in combination with the `gsub()` function to rename columns. For example, `colnames(df) <- gsub("old_pattern", "new_pattern", colnames(df))` will replace the specified pattern in all column names.
Is it possible to rename columns in a tibble differently than in a data frame?
No, the method for renaming columns in a tibble is similar to that of a data frame. You can use `rename()` from the `dplyr` package or the `colnames()` function to achieve the same results.
Renaming columns in R is a fundamental task that enhances data manipulation and analysis. There are several methods available to accomplish this, including using the `colnames()` function, the `names()` function, and the `dplyr` package’s `rename()` function. Each method allows for flexibility in modifying column names, catering to various user preferences and coding styles.
The `colnames()` and `names()` functions are straightforward and effective for renaming all columns or specific columns in a data frame. However, the `dplyr` package offers a more intuitive and readable syntax, particularly beneficial for users who are already utilizing the tidyverse framework. This package allows for selective renaming and can be easily integrated into a larger data manipulation workflow.
In summary, understanding how to rename columns in R is essential for effective data management. By leveraging the appropriate functions, users can ensure their datasets are organized and easily interpretable, ultimately leading to more efficient analysis and reporting. Mastering these techniques will significantly enhance one’s proficiency in R and contribute to more streamlined data processing tasks.
Author Profile
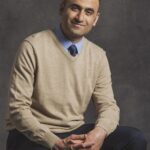
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?