How Can You Efficiently Repeat Code in Python?
In the world of programming, efficiency and elegance are paramount. As developers, we often find ourselves needing to execute the same block of code multiple times, whether it’s for processing data, running simulations, or simply automating repetitive tasks. In Python, a language celebrated for its readability and simplicity, there are several powerful techniques to help you repeat code effortlessly. Understanding these methods not only enhances your coding skills but also allows you to write cleaner, more efficient programs.
When it comes to repeating code in Python, there are a variety of approaches to choose from, each suited to different scenarios. From loops that iterate over a sequence of items to functions that encapsulate reusable blocks of code, Python offers a toolkit that can cater to both novice and experienced programmers alike. By mastering these techniques, you can significantly reduce redundancy in your code and improve its maintainability.
Moreover, the ability to repeat code effectively is foundational to grasping more complex programming concepts. As you delve deeper into Python, you’ll discover how these repetition mechanisms can be combined with other programming constructs to create sophisticated algorithms and solutions. Whether you’re building a simple script or a robust application, learning how to repeat code efficiently will empower you to tackle challenges with confidence and creativity.
Using Loops to Repeat Code
One of the most common methods to repeat code in Python is through the use of loops. Python provides two primary types of loops: `for` loops and `while` loops. Each serves different scenarios based on the requirements of your code.
For Loops
A `for` loop is typically used when you want to iterate over a sequence (like a list, tuple, or string). The loop continues until it has gone through every item in the sequence.
“`python
for i in range(5):
print(“This is iteration number:”, i)
“`
In this example, the loop will repeat the print statement five times, outputting the current iteration number each time.
- Structure of a for loop:
- Initialization: Set up the loop variable.
- Condition: Specify the range or sequence to iterate over.
- Execution: The block of code to repeat.
While Loops
A `while` loop continues to execute as long as a specified condition remains true. This type of loop is particularly useful when the number of iterations is not known beforehand.
“`python
count = 0
while count < 5:
print("This is iteration number:", count)
count += 1
```
In this case, the loop will repeat until the `count` variable reaches 5.
- Structure of a while loop:
- Initialization: Set a counter or condition variable.
- Condition: Define the condition that keeps the loop running.
- Execution: The block of code to repeat.
- Update: Modify the counter or condition to eventually break the loop.
Using Functions for Repetition
Another effective way to repeat code is by defining a function. This allows you to encapsulate your code and call it multiple times without rewriting it.
“`python
def repeat_message(message, times):
for _ in range(times):
print(message)
repeat_message(“Hello, World!”, 3)
“`
In this example, the function `repeat_message` takes a message and a number of repetitions as arguments, enabling you to easily adjust the output.
Comparison of Loop Types
The following table summarizes the differences between `for` loops and `while` loops:
Feature | For Loop | While Loop |
---|---|---|
Use Case | When the number of iterations is known | When the number of iterations is not known |
Syntax | for variable in sequence: | while condition: |
Control Structure | Iterates over a collection | Continues until a condition is |
Understanding these looping mechanisms allows for effective code repetition in Python, enabling developers to write more efficient and maintainable code.
Using Loops for Repetition
In Python, the most common way to repeat a block of code is through loops. The two primary types of loops are `for` loops and `while` loops.
For Loops
A `for` loop is typically used to iterate over a sequence (like a list, tuple, or string) or other iterable objects. The syntax is as follows:
“`python
for item in iterable:
Code block to repeat
“`
Example: Repeating a print statement for items in a list.
“`python
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
“`
This code will output each fruit in the list.
While Loops
A `while` loop continues to execute a block of code as long as a specified condition is true. The syntax is:
“`python
while condition:
Code block to repeat
“`
Example: Printing numbers until a condition is met.
“`python
count = 0
while count < 5:
print(count)
count += 1
```
This will print numbers from 0 to 4.
Using Functions for Code Repetition
Defining functions allows for code reuse without the need for explicit loops. A function can be called multiple times, effectively repeating the code within it.
Function Definition Syntax:
“`python
def function_name(parameters):
Code block
“`
Example: A function that prints a message.
“`python
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
greet(“Bob”)
“`
This will greet both Alice and Bob.
List Comprehensions for Concise Repetition
List comprehensions provide a concise way to create lists by iterating over iterables. This method is particularly useful for generating lists based on existing data.
Syntax:
“`python
new_list = [expression for item in iterable]
“`
Example: Creating a list of squares.
“`python
squares = [x**2 for x in range(10)]
print(squares)
“`
This will output the squares of numbers from 0 to 9.
Using the `repeat` Function from `itertools`
For scenarios where you need to repeat a single value multiple times, the `repeat` function from the `itertools` module can be useful.
Example:
“`python
from itertools import repeat
for item in repeat(“Hello”, 3):
print(item)
“`
This will print “Hello” three times.
Recursion for Repeating Code
Recursion involves a function calling itself to solve smaller instances of a problem. While powerful, it is essential to ensure a base case is defined to prevent infinite loops.
Example: Calculating factorial using recursion.
“`python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n – 1)
print(factorial(5)) Outputs 120
“`
In this example, the function calculates the factorial of a number by recursively calling itself.
Understanding these methods for repeating code in Python enhances code efficiency and readability. Depending on the task, one can choose between loops, functions, list comprehensions, and even recursion to achieve code repetition effectively.
Expert Insights on Repeating Code in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “In Python, repeating code can be efficiently achieved through the use of loops. For instance, ‘for’ loops and ‘while’ loops allow developers to execute a block of code multiple times, which is essential for tasks involving iteration over data sets.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Utilizing functions is another effective method for repeating code in Python. By encapsulating code within a function, developers can call that function multiple times throughout their program, enhancing both code reusability and readability.”
Sarah Patel (Python Instructor, LearnPython Academy). “It’s crucial to understand the importance of avoiding code duplication. Implementing loops and functions not only allows for repetition but also promotes better maintenance and scalability of the code, which is vital in professional programming environments.”
Frequently Asked Questions (FAQs)
How can I repeat a block of code in Python?
You can repeat a block of code in Python using loops, such as `for` loops or `while` loops. For example, a `for` loop can iterate over a range of numbers, while a `while` loop continues until a specified condition is no longer true.
What is the difference between a `for` loop and a `while` loop in Python?
A `for` loop is used for iterating over a sequence (like a list or a range), while a `while` loop continues executing as long as a specified condition remains true. Use `for` when the number of iterations is known, and `while` when it depends on a condition.
Can I use the `repeat` function to repeat code in Python?
Python does not have a built-in `repeat` function like some other languages. However, you can achieve similar functionality using loops or by defining a custom function that includes a loop to repeat the desired code.
How do I repeat a code snippet a specific number of times?
To repeat a code snippet a specific number of times, use a `for` loop with the `range()` function. For example, `for i in range(5):` will execute the code block five times.
Is there a way to repeat code indefinitely in Python?
Yes, you can use a `while` loop without a terminating condition, such as `while True:`, to repeat code indefinitely. However, ensure that there is a mechanism to break out of the loop to prevent infinite execution.
What is the best practice for repeating code in Python?
The best practice is to encapsulate repetitive code in functions. This promotes code reusability and maintainability. Use loops for iterations and functions for modular design, making your code cleaner and more efficient.
In Python, there are several effective methods to repeat a block of code, allowing for efficient execution of repetitive tasks. The most common approaches include using loops, such as ‘for’ loops and ‘while’ loops, as well as utilizing functions for code reuse. Each method serves specific use cases and can be tailored to fit the needs of the program being developed.
For instance, ‘for’ loops are particularly useful when the number of iterations is known beforehand, such as iterating over a list or a range of numbers. Conversely, ‘while’ loops are more suitable when the number of iterations is uncertain and depends on a condition being met. Additionally, defining functions allows for encapsulating code that can be called multiple times throughout a program, promoting modularity and clarity.
Key takeaways from the discussion on repeating code in Python include the importance of selecting the appropriate looping construct based on the specific requirements of the task. Understanding the differences between ‘for’ and ‘while’ loops, as well as the benefits of using functions, can significantly enhance code efficiency and readability. Mastering these concepts is essential for any Python programmer aiming to write clean and maintainable code.
Author Profile
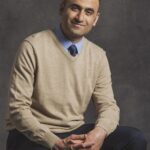
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?