How Can You Efficiently Repeat Python Code in Your Projects?
In the world of programming, efficiency and effectiveness are paramount. As you delve into Python, one of the most popular and versatile programming languages, you may find yourself needing to execute the same block of code multiple times. Whether you’re processing data, running simulations, or automating tasks, knowing how to repeat Python code can save you time and enhance your productivity. This article will guide you through the various techniques available in Python, empowering you to write cleaner, more efficient code.
Repeating code in Python can be accomplished through several powerful constructs that allow for flexibility and control. At the heart of this functionality are loops, which enable you to execute a sequence of statements multiple times based on specific conditions. Additionally, functions can be employed to encapsulate code, allowing you to call it repeatedly without redundancy. Understanding these concepts not only streamlines your coding process but also fosters better programming practices.
As you explore the methods for repeating code in Python, you’ll discover the importance of choosing the right approach for your specific task. From simple iterations to more complex recursive calls, each technique has its own advantages and use cases. By mastering these tools, you’ll be well-equipped to tackle a wide range of programming challenges with confidence and ease.
Using Loops to Repeat Code
One of the most common methods to repeat code in Python is through the use of loops. Loops allow you to execute a block of code multiple times based on a specified condition or a sequence of values. The two primary types of loops in Python are `for` loops and `while` loops.
- For Loops: Typically used when the number of iterations is known beforehand. It iterates over a sequence (like a list, tuple, or string).
“`python
for i in range(5):
print(“This will print 5 times.”)
“`
- While Loops: Best for situations where the number of iterations is not predetermined. It continues until a specified condition evaluates to .
“`python
count = 0
while count < 5:
print("This will also print 5 times.")
count += 1
```
Defining Functions for Code Repetition
Another effective way to repeat code is by defining functions. Functions encapsulate code into reusable blocks that can be called multiple times throughout your program. This approach enhances code readability and maintainability.
“`python
def greet(times):
for i in range(times):
print(“Hello, World!”)
greet(3) This will call the function and print “Hello, World!” three times.
“`
Utilizing List Comprehensions
List comprehensions provide a concise way to create lists and can be utilized for repeating actions in a more functional programming style. This method is particularly useful for generating lists based on existing lists or ranges.
“`python
squared_numbers = [x**2 for x in range(10)]
print(squared_numbers) This will output the squares of numbers from 0 to 9.
“`
Table of Loop and Function Usage
Method | Use Case | Example |
---|---|---|
For Loop | When the number of iterations is known | for i in range(3): print(i) |
While Loop | When the number of iterations is uncertain | while condition: do_something() |
Function | Reusable code blocks | def function_name(): do_something() |
List Comprehension | Creating lists from sequences | [x for x in iterable] |
Using Recursion for Code Repetition
Recursion is a technique where a function calls itself to solve smaller instances of the same problem. While powerful, recursion requires careful consideration of base cases to prevent infinite loops.
“`python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n – 1)
print(factorial(5)) Outputs 120
“`
In summary, Python offers various methods to repeat code, each suited for different scenarios. By understanding and utilizing these techniques effectively, you can write more efficient and maintainable code.
Using Loops to Repeat Code
In Python, loops are the primary constructs for repeating code. The two most commonly used loops are `for` loops and `while` loops.
For Loops
A `for` loop iterates over a sequence (like a list, tuple, or string) and executes a block of code for each item.
“`python
Example of a for loop
for i in range(5):
print(“This is repetition number”, i+1)
“`
This code will output:
“`
This is repetition number 1
This is repetition number 2
This is repetition number 3
This is repetition number 4
This is repetition number 5
“`
While Loops
A `while` loop continues to execute as long as a specified condition is true.
“`python
Example of a while loop
count = 0
while count < 5:
print("This is repetition number", count + 1)
count += 1
```
This code will produce the same output as the `for` loop example.
Defining Functions for Code Reuse
Functions allow you to encapsulate code into reusable blocks. You can define a function and call it multiple times as needed.
“`python
def repeat_message(n):
for i in range(n):
print(“This is repetition number”, i + 1)
Calling the function
repeat_message(5)
“`
The output will mirror that of the previous examples.
Using List Comprehensions for Conciseness
List comprehensions can be a succinct way to repeat actions when generating lists.
“`python
Example of list comprehension
messages = [“This is repetition number ” + str(i + 1) for i in range(5)]
for message in messages:
print(message)
“`
This generates the same output but does so with a more concise syntax.
Using the `itertools` Module for Advanced Repetition
The `itertools` module provides powerful tools for iteration. The `cycle` function can be used to repeat a sequence indefinitely.
“`python
import itertools
Example of using itertools.cycle
count = 0
for message in itertools.cycle([“This is repetition number 1”, “This is repetition number 2”]):
print(message)
count += 1
if count == 5: Stop after 5 repetitions
break
“`
This approach is useful for scenarios where you need to loop through a fixed set of values repeatedly.
Timing Repetitions with the `time` Module
When repeating code, you may want to control the timing of repetitions. The `time` module allows for pauses between iterations.
“`python
import time
for i in range(5):
print(“This is repetition number”, i + 1)
time.sleep(1) Pause for 1 second between messages
“`
This example introduces a delay, making each repetition more spaced out.
Using Recursion for Repetitive Tasks
Recursion can also be employed to repeat tasks, though it should be used cautiously due to potential stack overflow issues with deep recursion.
“`python
def recursive_repeat(n):
if n > 0:
print(“This is repetition number”, 6 – n)
recursive_repeat(n – 1)
recursive_repeat(5)
“`
This function will also output the same sequence, demonstrating a different approach to repeating code.
Summary of Repetition Techniques
The following table summarizes the different techniques discussed:
Technique | Description | Example Usage |
---|---|---|
For Loop | Iterates over a sequence | `for i in range(n)` |
While Loop | Executes while a condition is true | `while count < n` |
Function | Encapsulates code for reuse | `def function_name()` |
List Comprehension | Concise way to create lists | `[expression for item]` |
itertools.cycle | Repeats a sequence indefinitely | `itertools.cycle()` |
time.sleep | Adds delays between repetitions | `time.sleep(seconds)` |
Recursion | Calls function within itself to repeat tasks | `def recursive_function()` |
Expert Insights on Repeating Python Code
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively repeat Python code, one can utilize loops such as ‘for’ and ‘while’. These constructs allow for executing a block of code multiple times, which is essential for tasks that require iteration over data sets or repeated operations.”
Michael Thompson (Professor of Computer Science, University of Technology). “In Python, functions also play a crucial role in code repetition. By defining a function, you can encapsulate code that can be executed multiple times throughout your program, enhancing both reusability and readability.”
Sarah Lee (Python Developer, CodeCraft Solutions). “For more complex scenarios, utilizing recursion is another way to repeat code. This approach involves a function calling itself, which can be particularly useful for solving problems that can be broken down into smaller, similar subproblems.”
Frequently Asked Questions (FAQs)
How can I repeat a block of code in Python?
You can repeat a block of code in Python using loops such as `for` loops or `while` loops. A `for` loop iterates over a sequence, while a `while` loop continues until a specified condition is no longer true.
What is the syntax for a for loop in Python?
The syntax for a `for` loop is:
“`python
for variable in iterable:
code to execute
“`
This structure allows you to execute the indented code block for each item in the iterable.
How do I use a while loop to repeat code?
The syntax for a `while` loop is:
“`python
while condition:
code to execute
“`
This loop will execute the indented code block as long as the specified condition evaluates to true.
Can I repeat code using functions in Python?
Yes, you can define a function that contains the code you want to repeat. You can then call this function multiple times to execute the code. This promotes code reusability and organization.
What is the difference between a for loop and a while loop?
A `for` loop is used when the number of iterations is known or defined by a sequence, while a `while` loop is used when the number of iterations is not predetermined and depends on a condition being met.
How can I repeat code a specific number of times?
You can use a `for` loop with the `range()` function to repeat code a specific number of times. For example:
“`python
for i in range(n): where n is the number of repetitions
code to execute
“`
This will execute the indented code block `n` times.
Repeating code in Python is a fundamental concept that enhances efficiency and reduces redundancy in programming. There are several methods to achieve this, including using loops, functions, and recursion. Loops, such as `for` and `while`, allow for the execution of a block of code multiple times based on specified conditions. Functions enable code reuse by encapsulating logic that can be called whenever needed, while recursion involves a function calling itself to solve problems incrementally.
Understanding when and how to repeat code effectively can lead to cleaner and more maintainable codebases. Utilizing loops is particularly useful for iterating over collections or executing code a specific number of times. Functions, on the other hand, promote modularity and can be reused across different parts of a program, making it easier to manage changes. Recursion is a powerful technique for problems that can be broken down into smaller, similar subproblems, although it requires careful handling to avoid excessive memory use or stack overflow.
mastering the various methods of repeating code in Python is essential for any programmer looking to write efficient and organized code. By leveraging loops, functions, and recursion appropriately, developers can enhance their coding practices and improve the overall quality of their software solutions. Emphasizing
Author Profile
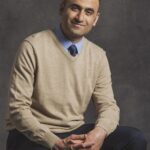
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?