How Can You Replace an Item in a Python List?
In the world of programming, lists are one of the most versatile and widely used data structures in Python. They allow you to store multiple items in a single variable, making it easy to manage collections of data. However, as you work with lists, you may find yourself needing to make changes—whether that’s updating a value, replacing an item, or even swapping elements around. Understanding how to effectively replace something in a list is a fundamental skill that can enhance your coding efficiency and open the door to more complex operations.
Replacing an item in a list might seem straightforward, but it involves understanding the underlying mechanics of indexing and list manipulation in Python. Lists are ordered collections, meaning each element has a specific position, or index, that allows you to access and modify them directly. Whether you’re dealing with a simple list of numbers or a more complex list of objects, knowing how to pinpoint and replace the right element is crucial for maintaining the integrity of your data.
As we delve deeper into the topic, we’ll explore various methods to replace items in a list, including direct assignment, using list comprehensions, and leveraging built-in functions. Each approach has its own nuances and use cases, providing you with a toolkit to handle different scenarios effectively. Whether you’re a beginner looking to grasp the basics or
Methods to Replace Elements in a Python List
To replace an element in a Python list, several methods can be employed, depending on the context and requirements. The most common approaches include direct indexing, the `list.index()` method combined with assignment, and list comprehensions. Each of these methods offers a different level of efficiency and flexibility.
Direct Indexing
The simplest and most straightforward method to replace an element is by using direct indexing. This approach allows you to access the element directly by its position in the list and assign a new value to it.
“`python
my_list = [1, 2, 3, 4, 5]
my_list[2] = 10 Replacing the element at index 2
print(my_list) Output: [1, 2, 10, 4, 5]
“`
This method is efficient, as it directly accesses the element without the need for additional functions.
Using the `list.index()` Method
If you do not know the index of the element you wish to replace, the `list.index()` method can be used to find the index of the first occurrence of the specified value. Once the index is retrieved, you can then replace the value at that index.
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
index = my_list.index(‘banana’) Find the index of ‘banana’
my_list[index] = ‘orange’ Replace ‘banana’ with ‘orange’
print(my_list) Output: [‘apple’, ‘orange’, ‘cherry’]
“`
This method is useful for replacing values without directly knowing their position in the list.
List Comprehensions
List comprehensions provide a compact way to process and transform lists. This method can be particularly useful when you need to replace multiple occurrences of an element or apply a condition during replacement.
“`python
my_list = [1, 2, 3, 2, 4]
my_list = [10 if x == 2 else x for x in my_list] Replace all occurrences of ‘2’ with ’10’
print(my_list) Output: [1, 10, 3, 10, 4]
“`
This approach is both elegant and efficient, especially when working with larger datasets.
Table of Replacement Methods
Method | Description | Example |
---|---|---|
Direct Indexing | Access and replace using index. | my_list[2] = new_value |
list.index() | Find index of value and replace. | index = my_list.index(‘value’) |
List Comprehension | Transform the list with conditions. | [new_value if x == old_value else x for x in my_list] |
In summary, depending on your specific needs, the method chosen for replacing elements in a list can vary. Direct indexing is ideal for known positions, while `list.index()` is useful when searching for specific values. List comprehensions offer a powerful way to handle more complex replacements efficiently.
Methods to Replace Elements in a List
To replace an element in a list in Python, several methods can be employed, depending on the specific needs of your application. Below are the most common approaches:
Using Index Assignment
The simplest way to replace an element is to access it via its index and assign a new value. This method is effective when you know the exact position of the element you want to replace.
“`python
my_list = [1, 2, 3, 4, 5]
my_list[2] = 99 Replace the element at index 2
print(my_list) Output: [1, 2, 99, 4, 5]
“`
Using the `list.replace()` Method
Python lists do not have a built-in `replace()` method like strings. However, you can create a custom function or use a list comprehension to achieve similar functionality.
“`python
def replace_element(lst, old, new):
return [new if x == old else x for x in lst]
my_list = [1, 2, 3, 2, 5]
my_list = replace_element(my_list, 2, 99)
print(my_list) Output: [1, 99, 3, 99, 5]
“`
Using the `index()` Method with Assignment
If you need to replace the first occurrence of a specific value and do not know its index, you can use the `index()` method.
“`python
my_list = [1, 2, 3, 2, 5]
index = my_list.index(2) Find the index of the first occurrence of 2
my_list[index] = 99 Replace it
print(my_list) Output: [1, 99, 3, 2, 5]
“`
Using List Comprehension for Multiple Replacements
For replacing multiple instances of a value throughout the list, a list comprehension is very effective.
“`python
my_list = [1, 2, 2, 3, 2, 4]
new_list = [99 if x == 2 else x for x in my_list]
print(new_list) Output: [1, 99, 99, 3, 99, 4]
“`
Replacing Elements Based on Conditions
You can also replace elements based on certain conditions using a list comprehension.
“`python
my_list = [1, 2, 3, 4, 5]
new_list = [x * 10 if x > 2 else x for x in my_list]
print(new_list) Output: [1, 2, 30, 40, 50]
“`
Example Table of Replacement Methods
Method | Description | Code Example |
---|---|---|
Index Assignment | Replace using direct index access | `my_list[2] = new_value` |
Custom Replace Function | Replace all occurrences with a custom function | `replace_element(my_list, old, new)` |
Using `index()` | Replace first occurrence found | `my_list[my_list.index(old)] = new` |
List Comprehension | Replace multiple occurrences based on value | `[new if x == old else x for x in my_list]` |
Conditional Replacement | Replace based on condition | `[x * 10 if x > 2 else x for x in my_list]` |
These methods provide flexibility to replace elements in a list based on different scenarios and requirements. Choose the method that best fits your use case for effective list manipulation in Python.
Expert Insights on Replacing Elements in Python Lists
Dr. Emily Chen (Senior Software Engineer, Python Development Group). “When replacing elements in a list, it is crucial to understand the indexing system in Python. Utilizing the index of the element you wish to replace allows for clear and efficient modifications. For instance, using `list[index] = new_value` is a direct and effective approach.”
James Patel (Data Scientist, Tech Innovations Inc.). “In scenarios where multiple occurrences of an element need to be replaced, employing a list comprehension can be particularly powerful. The syntax `new_list = [new_value if x == old_value else x for x in original_list]` provides a clean and Pythonic way to achieve this.”
Linda Garcia (Python Instructor, Code Academy). “For beginners, it is essential to grasp the difference between mutable and immutable types in Python. Lists are mutable, which means you can change their content without creating a new list. This property makes them ideal for dynamic data manipulation, including element replacement.”
Frequently Asked Questions (FAQs)
How can I replace an item in a list in Python?
You can replace an item in a list by assigning a new value to the specific index of that item. For example, `my_list[index] = new_value` will replace the item at the specified index.
What method can be used to replace all occurrences of a specific value in a list?
To replace all occurrences of a specific value, you can use a list comprehension. For example, `my_list = [new_value if x == old_value else x for x in my_list]` will replace all instances of `old_value` with `new_value`.
Is there a built-in function to replace items in a list?
Python does not have a built-in function specifically for replacing items in a list. However, you can use list comprehensions or the `map()` function to achieve similar results.
Can I replace an item in a nested list?
Yes, you can replace an item in a nested list by accessing the specific sublist and index. For instance, `my_list[sublist_index][item_index] = new_value` will replace the item in the specified sublist.
What happens if I try to replace an item at an index that does not exist?
If you attempt to replace an item at an index that does not exist, Python will raise an `IndexError`, indicating that the index is out of range for the list.
How do I replace items in a list while iterating over it?
To replace items while iterating, create a copy of the list or use a list comprehension to generate a new list. Modifying the list directly while iterating can lead to unexpected behavior.
In Python, replacing an item in a list can be achieved through various methods, each suited to different scenarios. The most straightforward approach is to directly assign a new value to a specific index of the list. For example, if you have a list called `my_list` and you want to replace the item at index `2`, you can simply use `my_list[2] = new_value`. This method is efficient when you know the exact index of the item you wish to replace.
Another common method involves using the `list.index()` function to find the index of the item you want to replace. Once you have the index, you can proceed with the assignment as previously mentioned. This is particularly useful when the value of the item is known, but its position in the list is not. Additionally, Python’s `list comprehension` can be utilized to create a new list with the desired replacements, allowing for more complex replacement logic based on conditions.
It is also important to note that lists in Python are mutable, meaning that modifications can be made in place without creating a new list. This characteristic allows for efficient memory usage and performance. However, when replacing multiple occurrences of a value, one might consider using a loop or the `
Author Profile
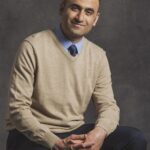
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?