How Can You Restart a While Loop in Python?
In the world of programming, loops are essential tools that allow us to execute a block of code repeatedly until a certain condition is met. Among the various types of loops, the `while` loop stands out for its flexibility, enabling developers to create dynamic and responsive applications. However, there are times when you may find yourself needing to restart a `while` loop under specific conditions, whether to re-evaluate user input, reset a process, or handle unexpected scenarios. Understanding how to effectively restart a `while` loop in Python can enhance your coding skills and lead to more robust and efficient programs.
Restarting a `while` loop in Python may seem straightforward, but it involves a nuanced understanding of control flow and loop management. At its core, the ability to restart a loop hinges on the conditions that dictate its execution. By strategically placing control statements and leveraging flags or counters, you can create loops that adapt to changing circumstances, ensuring that your code behaves as intended. This flexibility not only improves user experience but also contributes to cleaner, more maintainable code.
As we delve deeper into the mechanics of restarting a `while` loop, we will explore various techniques and best practices that can help you implement this functionality effectively. From utilizing `break` and `continue` statements
Understanding the While Loop
A while loop in Python is a control flow statement that allows code to be executed repeatedly based on a given condition. It continues to run as long as the condition evaluates to True. The basic syntax of a while loop is as follows:
“`python
while condition:
code block
“`
When you need to restart a while loop, it generally involves modifying the control variable that dictates the loop’s continuation. This can be achieved using several techniques, which can help you to effectively manage the flow of your program.
Using a Break and a Continue Statement
In Python, the `break` and `continue` statements can help manage the flow within a while loop. Here’s how each can be used:
- break: This statement terminates the loop entirely and exits out of it.
- continue: This statement skips the rest of the code inside the loop for the current iteration and jumps back to the condition check.
To illustrate how to restart a while loop, consider the following example:
“`python
count = 0
while count < 5: count += 1 if count == 3: print("Restarting the loop.") count = 0 Resetting count restarts the loop print(count) ``` In this example, when `count` reaches 3, it is reset to 0, effectively restarting the while loop.
Using Functions to Restart a While Loop
Another effective method to restart a while loop is by encapsulating the loop within a function. This allows for a clean reset by simply calling the function again. Here’s an example:
“`python
def run_loop():
count = 0
while count < 5:
count += 1
if count == 3:
print("Restarting the loop.")
return run_loop() Call the function again to restart
print(count)
run_loop()
```
In this scenario, once the count hits 3, the function is called again, allowing the while loop to restart.
Table of Control Flow Statements
Statement | Description |
---|---|
break | Exits the loop immediately. |
continue | Skips the current iteration and continues with the next. |
return | Exits a function and can be used to restart the loop by calling the function. |
By utilizing control flow statements like `break`, `continue`, and `return`, you can manage the execution of while loops efficiently. Understanding these concepts will enhance your ability to control program flow in Python.
Methods to Restart a While Loop in Python
To restart a `while` loop in Python, developers can utilize various techniques based on the specific requirements of their program. Below are several methods to achieve this, along with code examples for clarity.
Using `continue` Statement
The `continue` statement allows the loop to skip the current iteration and proceed to the next iteration of the loop. You can use it to restart the loop from the beginning based on a certain condition.
“`python
while True:
user_input = input(“Enter a number (or ‘exit’ to quit): “)
if user_input == ‘exit’:
break
if not user_input.isdigit():
print(“Invalid input, please try again.”)
continue Restarts the loop for invalid input
number = int(user_input)
print(f”You entered: {number}”)
“`
In this example, if the user inputs something that is not a digit, the loop restarts without executing further statements.
Using a Function for Loop Logic
Encapsulating the loop logic within a function can facilitate a clean restart of the loop. You can call the function again when needed.
“`python
def repeatable_loop():
while True:
user_input = input(“Enter a number (or ‘exit’ to quit): “)
if user_input == ‘exit’:
break
if not user_input.isdigit():
print(“Invalid input, please try again.”)
continue
number = int(user_input)
print(f”You entered: {number}”)
repeatable_loop()
“`
In this design, if you need to restart the logic for any reason, you can simply call `repeatable_loop()` again.
Using a Flag Variable
A flag variable can be used to control the flow of the loop. This method provides flexibility to restart the loop based on the flag’s state.
“`python
restart_loop = True
while restart_loop:
user_input = input(“Enter a number (or ‘exit’ to quit): “)
if user_input == ‘exit’:
restart_loop =
elif not user_input.isdigit():
print(“Invalid input, please try again.”)
else:
number = int(user_input)
print(f”You entered: {number}”)
“`
Here, if the user enters an invalid input, the loop continues due to the flag remaining `True`.
Using Exception Handling
Another technique involves using exception handling to manage restart scenarios when unexpected conditions arise.
“`python
while True:
try:
user_input = input(“Enter a number (or ‘exit’ to quit): “)
if user_input == ‘exit’:
break
number = int(user_input)
print(f”You entered: {number}”)
except ValueError:
print(“Invalid input, please enter a valid number.”)
“`
In this example, if an invalid input is provided, a `ValueError` is raised, and the loop continues without needing an explicit restart mechanism.
Restarting a `while` loop in Python can be achieved through multiple methodologies, each offering different advantages. Depending on the context and requirements of the application, developers can select the approach that best fits their needs.
Expert Insights on Restarting While Loops in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To effectively restart a while loop in Python, one can utilize a control variable that dictates the loop’s continuation. By adjusting this variable within the loop based on specific conditions, the loop can be restarted seamlessly without exiting and re-entering the loop structure.”
Michael Thompson (Lead Software Engineer, CodeCrafters). “A common approach to restarting a while loop is to encapsulate the loop logic within a function. This allows you to call the function again whenever you need to restart the loop, providing a clean and manageable way to handle repeated executions.”
Sarah Lee (Python Programming Instructor, Code Academy). “When teaching how to restart a while loop, I emphasize the importance of using flags or sentinel values. By setting a flag to indicate whether the loop should continue or restart, learners can gain a deeper understanding of control flow in Python.”
Frequently Asked Questions (FAQs)
How can I restart a while loop in Python?
To restart a while loop in Python, you can use the `continue` statement. This statement skips the current iteration and jumps to the next iteration of the loop.
Can I use a function to restart a while loop?
Yes, you can encapsulate the while loop within a function. By calling the function again, you effectively restart the while loop from the beginning.
Is there a way to reset loop variables when restarting a while loop?
Yes, you can reset loop variables before the `continue` statement or at the beginning of the loop to ensure they are initialized correctly for the next iteration.
What happens if I use a break statement before a restart in a while loop?
Using a `break` statement will terminate the while loop entirely, preventing any further iterations or restarts. If you need to restart, avoid using `break` unless you are exiting the loop conditionally.
Can I control how many times a while loop restarts?
Yes, you can control the number of restarts by using a counter variable. Increment the counter each time the loop restarts and use a conditional statement to exit the loop after a certain number of restarts.
Are there any performance considerations when restarting a while loop frequently?
Frequent restarts of a while loop can lead to performance issues, especially if the loop contains complex operations. It’s advisable to optimize the loop logic and minimize unnecessary iterations for better efficiency.
In Python, restarting a while loop can be achieved through various control flow mechanisms, such as using the `continue` statement, modifying loop conditions, or employing functions to encapsulate the loop logic. The `continue` statement allows the loop to skip the current iteration and proceed to the next one, effectively “restarting” the loop from the beginning of its block. This is particularly useful when certain conditions are met that require the loop to bypass subsequent code and re-evaluate its condition.
Another approach to restarting a while loop involves adjusting the loop’s condition dynamically. By changing the variables that influence the loop’s condition within the loop body, you can control when the loop should continue or terminate. This method provides flexibility in managing the loop’s execution flow based on real-time data or user input.
Additionally, encapsulating the while loop within a function can offer a structured way to restart the loop. By calling the function again, the loop can be reinitialized, allowing for a fresh start with potentially different parameters. This method enhances code organization and reusability, making it easier to manage complex looping scenarios.
In summary, restarting a while loop in Python can be effectively managed through the use of control statements, dynamic condition
Author Profile
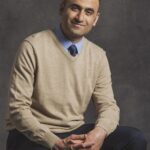
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?