How Can You Effectively Return a List in Python?
In the world of programming, efficiency and clarity are paramount, and Python excels in both areas. As one of the most popular programming languages, Python offers a plethora of features that simplify coding tasks, one of which is the ability to work with lists. Lists are versatile data structures that can hold an ordered collection of items, making them indispensable for developers. But what happens when you want to return a list from a function? Understanding how to effectively return a list in Python can significantly enhance your coding skills and improve the functionality of your programs.
Returning a list in Python is a fundamental concept that every programmer should grasp. It allows you to encapsulate data within functions and pass it back to the caller, enabling modular and reusable code. Whether you’re aggregating results from a series of computations, filtering data, or simply organizing information, knowing how to return a list can streamline your approach and make your code more efficient.
In this article, we will explore the various methods of returning lists in Python, discussing best practices and common pitfalls. From simple functions that return static lists to more complex scenarios involving dynamic data generation, you’ll gain insights that will empower you to leverage lists more effectively in your Python projects. Get ready to dive into the world of lists and unlock new possibilities in your
Returning a List from a Function
In Python, a function can return a list just like any other data type. To return a list, you define a function that creates and populates a list, and then use the `return` statement to send that list back to the caller. Below is a simple example illustrating this concept.
“`python
def create_list():
my_list = [1, 2, 3, 4, 5]
return my_list
“`
When you call `create_list()`, it will return the list `[1, 2, 3, 4, 5]`. You can assign this returned list to a variable for further use:
“`python
returned_list = create_list()
print(returned_list) Output: [1, 2, 3, 4, 5]
“`
Dynamic List Creation
You may want to create lists dynamically based on certain conditions or inputs. This can be achieved using loops or list comprehensions. Here’s an example using a loop:
“`python
def generate_even_numbers(n):
even_numbers = []
for i in range(n):
if i % 2 == 0:
even_numbers.append(i)
return even_numbers
“`
Calling `generate_even_numbers(10)` will return the list `[0, 2, 4, 6, 8]`.
Alternatively, you can use a list comprehension for a more concise expression:
“`python
def generate_even_numbers_comprehension(n):
return [i for i in range(n) if i % 2 == 0]
“`
Both functions will produce the same output.
Returning Multiple Lists
In some cases, you may need to return more than one list from a function. Python allows you to return multiple values packed in a tuple, which can include lists.
“`python
def split_even_odd(n):
evens = [i for i in range(n) if i % 2 == 0]
odds = [i for i in range(n) if i % 2 != 0]
return evens, odds
“`
When you call `split_even_odd(10)`, it will return two lists: one containing even numbers and another with odd numbers. You can unpack these lists as follows:
“`python
even_list, odd_list = split_even_odd(10)
print(even_list) Output: [0, 2, 4, 6, 8]
print(odd_list) Output: [1, 3, 5, 7, 9]
“`
Table of Common List Operations
The following table summarizes common operations you might perform with lists in Python:
Operation | Description | Example |
---|---|---|
Append | Add an element to the end of the list. | list.append(10) |
Extend | Add elements from another list. | list.extend([11, 12]) |
Insert | Add an element at a specific index. | list.insert(1, ‘a’) |
Remove | Remove the first occurrence of an element. | list.remove(‘a’) |
Pop | Remove and return an element at a given index. | list.pop(0) |
Returning a List from a Function
In Python, functions can easily return lists, allowing for flexible data manipulation and processing. The return statement is used to exit a function and send a value back to the caller.
To return a list from a function, follow this general structure:
“`python
def function_name():
Create a list
my_list = [1, 2, 3, 4, 5]
return my_list
“`
When you call `function_name()`, it will return the list `[1, 2, 3, 4, 5]`.
Examples of Returning Lists
Here are a few practical examples that illustrate different scenarios of returning lists in Python functions:
- Returning a List of Squares
This function generates a list of squares for numbers in a given range.
“`python
def squares_list(n):
squares = [x**2 for x in range(n)]
return squares
print(squares_list(5)) Output: [0, 1, 4, 9, 16]
“`
- Filtering Even Numbers
This function filters out even numbers from a given list.
“`python
def filter_even(numbers):
evens = [num for num in numbers if num % 2 == 0]
return evens
print(filter_even([1, 2, 3, 4, 5, 6])) Output: [2, 4, 6]
“`
- Combining Two Lists
This function merges two lists into one.
“`python
def combine_lists(list1, list2):
combined = list1 + list2
return combined
print(combine_lists([1, 2], [3, 4])) Output: [1, 2, 3, 4]
“`
Using Lists in Function Parameters
Functions can also accept lists as parameters, allowing for dynamic processing based on input data.
For example, consider a function that takes a list and returns its unique elements:
“`python
def unique_elements(input_list):
unique_list = list(set(input_list))
return unique_list
print(unique_elements([1, 2, 2, 3, 4, 4])) Output: [1, 2, 3, 4]
“`
In this case, the function leverages the `set` data structure to filter duplicates before returning a list.
Handling Multiple Return Values
Python allows functions to return multiple lists by packing them into a tuple. This can be useful when you want to return different categories of data.
“`python
def categorize_numbers(numbers):
evens = [num for num in numbers if num % 2 == 0]
odds = [num for num in numbers if num % 2 != 0]
return evens, odds
even_numbers, odd_numbers = categorize_numbers([1, 2, 3, 4, 5])
print(even_numbers) Output: [2, 4]
print(odd_numbers) Output: [1, 3, 5]
“`
The function `categorize_numbers` returns two lists, which can then be unpacked into separate variables.
Best Practices for Returning Lists
When returning lists from functions, consider the following best practices:
– **Type Hinting**: Use type hints to indicate the expected return type. This improves code readability and helps with debugging.
“`python
from typing import List
def generate_numbers(n: int) -> List[int]:
return [x for x in range(n)]
“`
- Immutable Returns: If possible, return immutable types (like tuples) if the list should not be modified after creation.
- Avoid Side Effects: Ensure that your function does not modify any external variables, maintaining encapsulation.
By following these guidelines, you can create robust and maintainable functions that effectively return lists in Python.
Expert Insights on Returning Lists in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Returning a list in Python is a fundamental skill that every programmer should master. It allows for efficient data handling and manipulation, making your code more modular and reusable. Utilizing the `return` statement effectively can greatly enhance the readability and functionality of your functions.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “When returning a list in Python, it is essential to understand the context of your application. For instance, using list comprehensions can streamline the process of creating and returning lists, thereby improving performance and reducing code complexity. This practice is particularly useful in data processing tasks.”
Lisa Nguyen (Python Educator, Future Coders Academy). “Teaching how to return a list in Python involves demonstrating both simple and advanced techniques. It is crucial for learners to grasp the concept of returning multiple values as lists, as well as the use of built-in functions like `map()` and `filter()` that can return lists based on specific criteria.”
Frequently Asked Questions (FAQs)
How do I return a list from a function in Python?
To return a list from a function in Python, define the function using the `def` keyword, create a list within the function, and use the `return` statement followed by the list variable. For example:
“`python
def my_function():
my_list = [1, 2, 3]
return my_list
“`
Can I return multiple lists from a function in Python?
Yes, you can return multiple lists from a function by returning them as a tuple. For example:
“`python
def return_lists():
list1 = [1, 2, 3]
list2 = [4, 5, 6]
return list1, list2
“`
What happens if I return a list without using the return statement?
If you do not use the `return` statement, the function will return `None` by default. The list will not be accessible outside the function.
Can I modify a list after returning it from a function?
Yes, you can modify a list after returning it from a function. However, modifications will affect the list object if it is mutable and referenced outside the function.
What is the difference between returning a list and printing it in Python?
Returning a list allows the caller to use the list in subsequent code, while printing it displays the list output to the console but does not provide access to the list for further operations.
Is it possible to return an empty list from a function?
Yes, you can return an empty list from a function by defining it as `[]` and using the `return` statement. For example:
“`python
def empty_list_function():
return []
“`
Returning a list in Python is a fundamental concept that allows developers to effectively manage and manipulate collections of data. In Python, a function can return a list simply by using the `return` statement followed by the list variable. This enables the encapsulation of logic within functions while providing the flexibility to retrieve and utilize lists as needed in various parts of a program.
When defining a function that returns a list, it is important to ensure that the list is properly constructed and populated with the desired elements before the return statement is executed. This practice not only enhances code readability but also improves maintainability. Additionally, understanding how to return lists can facilitate operations such as filtering, transforming, and aggregating data, which are common tasks in data processing and analysis.
Key takeaways include the importance of using the `return` statement effectively to pass lists from functions, as well as the ability to create dynamic lists based on input parameters or computations within the function. Mastery of this concept is essential for anyone looking to leverage Python’s capabilities in data handling and algorithm development.
Author Profile
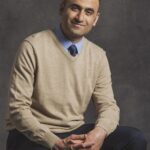
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?