How Can You Return a Tuple in Python?
In the world of Python programming, tuples are often celebrated for their simplicity and efficiency. These immutable sequences allow developers to store collections of items in a single, cohesive structure, making them an invaluable tool in any programmer’s toolkit. But what happens when you want to return a tuple from a function? Understanding how to effectively return tuples can enhance your coding practices, streamline your data handling, and elevate your overall programming skills. Whether you’re a novice eager to learn or a seasoned coder looking to refine your techniques, mastering tuple return will open up new avenues for your projects.
Returning a tuple in Python is a straightforward yet powerful concept that can significantly impact how you manage and organize data within your functions. When you return a tuple, you can encapsulate multiple values in a single return statement, allowing for cleaner and more efficient code. This approach not only simplifies the process of sending multiple outputs from a function but also ensures that your data remains organized and easy to access.
Moreover, tuples provide a level of immutability that can safeguard your data from unintended modifications, making them an ideal choice for certain programming scenarios. As we delve deeper into the mechanics of returning tuples, you’ll discover various techniques and best practices that will help you harness the full potential of this versatile data structure. Get ready to explore the
Returning a Tuple from a Function
In Python, returning a tuple from a function is straightforward and can be done using a simple return statement. A tuple is defined by placing a comma-separated sequence of values within parentheses. When a function returns multiple values, they can be encapsulated in a tuple automatically.
Here is how you can return a tuple in Python:
“`python
def get_coordinates():
x = 10
y = 20
return (x, y) Explicitly returning a tuple
Usage
coordinates = get_coordinates()
print(coordinates) Output: (10, 20)
“`
Alternatively, you can omit the parentheses when returning multiple values. Python will automatically create a tuple for you:
“`python
def get_dimensions():
width = 30
height = 50
return width, height Implicitly returning a tuple
Usage
dimensions = get_dimensions()
print(dimensions) Output: (30, 50)
“`
This implicit tuple creation is a feature of Python, making the syntax cleaner and more concise.
Accessing Tuple Elements
Once a tuple is returned from a function, its elements can be accessed in various ways, including unpacking. Here are some methods to access the values:
- Direct Indexing: You can access each element using its index.
- Tuple Unpacking: You can assign the returned tuple directly to multiple variables.
Example of Accessing Tuple Elements:
“`python
Using direct indexing
result = get_coordinates()
print(result[0]) Output: 10
print(result[1]) Output: 20
Using tuple unpacking
x, y = get_coordinates()
print(x) Output: 10
print(y) Output: 20
“`
Returning Multiple Data Types in a Tuple
Tuples in Python can also hold mixed data types, allowing for flexible function returns. This feature is particularly useful when you need to return related but different types of data from a function.
Example of Returning Mixed Types:
“`python
def get_user_info():
username = “john_doe”
age = 30
is_active = True
return username, age, is_active Implicitly returning a tuple
Usage
user_info = get_user_info()
print(user_info) Output: (‘john_doe’, 30, True)
“`
Variable | Type |
---|---|
username | String |
age | Integer |
is_active | Boolean |
Using this approach, you can effectively return and handle multiple related values of different types, enhancing your function’s utility and readability.
Returning Tuples from Functions
In Python, a tuple can be returned from a function by using the `return` statement followed by a comma-separated list of values. The values will be packed into a tuple automatically. Here’s how you can implement this:
“`python
def return_tuple():
return 1, 2, 3 This returns a tuple (1, 2, 3)
result = return_tuple()
print(result) Output: (1, 2, 3)
“`
The `return` statement can return any number of values, and they will be received as a tuple by the caller. This feature allows for the efficient packaging of multiple return values.
Tuple Assignment
When a function returns a tuple, you can unpack the values directly into variables. This can enhance code readability and make it clear what each value represents.
“`python
def coordinates():
return 10, 20
x, y = coordinates()
print(f”x: {x}, y: {y}”) Output: x: 10, y: 20
“`
In this example, the returned tuple is unpacked into `x` and `y`, demonstrating how to effectively utilize returned tuples in your code.
Returning Tuples with Multiple Data Types
Tuples are versatile and can contain mixed data types. For instance, a function can return a combination of integers, strings, or even other tuples.
“`python
def employee_info():
return “Alice”, 30, “Engineer” Returns a tuple with mixed types
name, age, profession = employee_info()
print(f”Name: {name}, Age: {age}, Profession: {profession}”)
“`
This illustrates how to manage complex data structures with tuples, supporting both clarity and flexibility in your function design.
Returning Tuples in a Dictionary Context
Tuples can also be beneficial when returning multiple values as part of a dictionary. This approach allows for a more descriptive return type.
“`python
def get_user_data():
return {
“user”: (“Bob”, 25), Tuple within a dictionary
“status”: “active”
}
data = get_user_data()
user_info = data[“user”]
print(f”User: {user_info[0]}, Age: {user_info[1]}”) Output: User: Bob, Age: 25
“`
Here, the function returns a dictionary containing a tuple, which can be accessed and manipulated easily.
Example Use Cases
Returning tuples can be particularly useful in various scenarios:
- Mathematical Functions: Returning multiple results from a calculation (e.g., min, max).
- Database Queries: Fetching multiple fields from a database record.
- Coordinate Systems: Returning x and y coordinates from a function.
By leveraging tuples effectively, you can improve the structure and clarity of your code while enhancing its functionality.
Expert Insights on Returning Tuples in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Returning a tuple in Python is straightforward and efficient. You can simply use the return statement followed by the tuple, which can be created using parentheses. This allows you to return multiple values from a function seamlessly, enhancing code readability and maintainability.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “When returning a tuple in Python, it’s essential to remember that tuples are immutable. This characteristic can be beneficial when you want to return a fixed set of values without the risk of modification. Utilizing tuples effectively can lead to cleaner and more robust code.”
Sarah Lin (Python Educator, LearnPython.org). “For beginners, understanding how to return a tuple can significantly improve their grasp of function outputs in Python. I always recommend practicing with simple examples, such as returning coordinates or RGB values, to see how tuples can encapsulate related data in a single return statement.”
Frequently Asked Questions (FAQs)
How do I return a tuple from a function in Python?
You can return a tuple from a function by simply placing the values you want to include in parentheses, separated by commas. For example:
“`python
def my_function():
return (1, 2, 3)
“`
Can I return multiple values without using a tuple?
Yes, you can return multiple values without explicitly creating a tuple. Python automatically creates a tuple when you separate values with commas in the return statement. For example:
“`python
def my_function():
return 1, 2, 3
“`
What is the syntax for unpacking a tuple returned from a function?
You can unpack a tuple by assigning the returned values to multiple variables in a single line. For example:
“`python
a, b, c = my_function()
“`
Are there any performance implications of returning tuples in Python?
Returning tuples in Python is generally efficient, as tuples are lightweight and immutable. However, if you are returning large amounts of data, consider the memory overhead and performance trade-offs.
Can I return a tuple with different data types?
Yes, tuples can contain elements of different data types. For instance, you can return a tuple that includes integers, strings, and lists:
“`python
def my_function():
return (1, “hello”, [1, 2, 3])
“`
What are the advantages of using tuples over lists when returning values?
Tuples are immutable, which makes them safer for returning fixed collections of values. They also have a smaller memory footprint compared to lists, and their immutability can help prevent accidental modification of the returned data.
In Python, returning a tuple from a function is a straightforward process that enhances the versatility of the language. A tuple is an immutable sequence type that can hold multiple items, making it ideal for returning multiple values from a function. To return a tuple, you simply need to use parentheses to group the values you wish to return, and the function will output them as a single tuple object.
One of the key advantages of using tuples is their ability to store heterogeneous data types, allowing for greater flexibility in function design. Additionally, tuples are immutable, which means that once they are created, their contents cannot be altered. This property makes tuples particularly useful for returning fixed collections of values that should not be modified, thus ensuring data integrity.
When working with functions that return tuples, it is also important to understand how to unpack the returned values. Python allows for easy unpacking of tuples into individual variables, which can enhance code readability and maintainability. By leveraging the tuple return feature, developers can write cleaner and more efficient code that effectively communicates the intended data structure.
Author Profile
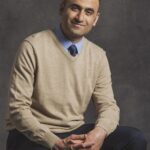
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?