How Can You Reverse an Array in Python Efficiently?
Reversing an array is a fundamental operation in programming that can come in handy in various scenarios, from data manipulation to algorithm design. Whether you’re a novice coder looking to understand the basics of Python or an experienced developer seeking to refine your skills, mastering the art of reversing an array can enhance your coding toolkit. In this article, we will explore different methods to reverse an array in Python, showcasing the language’s flexibility and power.
Arrays, or lists as they are known in Python, are versatile data structures that allow you to store and manage collections of items efficiently. Reversing an array can be a simple yet profound task, often used in algorithms that require data to be processed in reverse order. Understanding how to achieve this can not only improve your programming skills but also deepen your comprehension of data structures and their manipulation.
In the following sections, we will delve into various techniques for reversing an array in Python, from built-in functions to custom implementations. Each method has its own advantages and use cases, making it essential to understand the nuances of each approach. So, whether you’re looking for a quick solution or a deeper understanding of the underlying mechanics, this guide will equip you with the knowledge you need to reverse arrays like a pro.
Using Python’s Built-in Functions
Python provides several built-in methods to reverse an array, with the most common being the `reverse()` method and slicing.
The `reverse()` method modifies the list in place, meaning it will change the original array. Here’s how to use it:
python
arr = [1, 2, 3, 4, 5]
arr.reverse()
print(arr) # Output: [5, 4, 3, 2, 1]
Alternatively, you can reverse an array using slicing, which creates a new reversed list without altering the original:
python
arr = [1, 2, 3, 4, 5]
reversed_arr = arr[::-1]
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
Using the `reversed()` Function
Another effective way to reverse an array is by using the `reversed()` function. This function returns an iterator that accesses the given array in reverse order.
Example usage:
python
arr = [1, 2, 3, 4, 5]
reversed_arr = list(reversed(arr))
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
This approach is beneficial when you want to preserve the original array while obtaining a reversed version.
Custom Function to Reverse an Array
In some cases, you may want to implement your own logic for reversing an array. Here’s a simple function that demonstrates this concept:
python
def reverse_array(arr):
start = 0
end = len(arr) – 1
while start < end:
arr[start], arr[end] = arr[end], arr[start] # Swap elements
start += 1
end -= 1
return arr
arr = [1, 2, 3, 4, 5]
print(reverse_array(arr)) # Output: [5, 4, 3, 2, 1]
This method swaps elements from both ends of the array until it reaches the center.
Comparison of Methods
The following table summarizes the different methods of reversing an array in Python, including their characteristics and usage scenarios.
Method | In-place Modification | Returns New List | Complexity |
---|---|---|---|
reverse() | Yes | No | O(n) |
Slicing | No | Yes | O(n) |
reversed() | No | Yes | O(n) |
Custom Function | Yes | No | O(n) |
Each of these methods can be chosen based on the specific requirements of your application, such as whether you need to retain the original array or not.
Methods to Reverse an Array in Python
Reversing an array in Python can be accomplished through various methods, each suited to different scenarios and preferences. Below are some effective techniques to achieve this:
Using the Built-in `reverse()` Method
The `reverse()` method is a built-in function that modifies the list in place. This method does not return a new list but reverses the elements of the original list.
python
arr = [1, 2, 3, 4, 5]
arr.reverse()
print(arr) # Output: [5, 4, 3, 2, 1]
Using Slicing
Python’s slicing feature allows for a concise way to reverse an array. This method creates a new list that is a reversed version of the original.
python
arr = [1, 2, 3, 4, 5]
reversed_arr = arr[::-1]
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
Using the `reversed()` Function
The `reversed()` function returns an iterator that accesses the given list in the reverse order. It can be converted back to a list if needed.
python
arr = [1, 2, 3, 4, 5]
reversed_arr = list(reversed(arr))
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
Using a Loop
A manual approach involves iterating through the array and appending elements to a new list in reverse order. This method is more verbose but can be useful for educational purposes.
python
arr = [1, 2, 3, 4, 5]
reversed_arr = []
for i in range(len(arr) – 1, -1, -1):
reversed_arr.append(arr[i])
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
Using List Comprehension
List comprehension provides a compact way to create a new list by iterating through the original list in reverse order.
python
arr = [1, 2, 3, 4, 5]
reversed_arr = [arr[i] for i in range(len(arr) – 1, -1, -1)]
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
Performance Comparison
Method | In-Place | Time Complexity | Space Complexity |
---|---|---|---|
`reverse()` | Yes | O(n) | O(1) |
Slicing | No | O(n) | O(n) |
`reversed()` | No | O(n) | O(n) |
Manual Loop | No | O(n) | O(n) |
List Comprehension | No | O(n) | O(n) |
Each method has its own use cases. For instance, `reverse()` is ideal for in-place operations, while slicing or `reversed()` is preferred for creating new lists. Choose the method that best fits your needs based on performance and clarity.
Expert Insights on Reversing an Array in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Reversing an array in Python can be efficiently achieved using slicing. The syntax `array[::-1]` is not only concise but also leverages Python’s powerful slicing capabilities, making it a preferred method among data scientists for its readability and performance.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “While there are multiple methods to reverse an array in Python, such as using the `reverse()` method or the `reversed()` function, I recommend the slicing method for its elegance and efficiency. It allows for a quick reversal without modifying the original array, which is crucial in many applications.”
Linda Zhang (Python Developer, Open Source Community). “In Python, reversing an array is not just about the method but also about understanding the implications of each approach. For instance, using `list.reverse()` alters the original list in place, which is useful in certain scenarios, while `reversed()` returns an iterator, providing flexibility in handling data.”
Frequently Asked Questions (FAQs)
How can I reverse an array in Python using slicing?
You can reverse an array in Python using slicing by utilizing the syntax `array[::-1]`. This creates a new array that contains the elements of the original array in reverse order.
Is there a built-in method to reverse a list in Python?
Yes, Python provides a built-in method called `reverse()`. You can call this method on a list, which will reverse the list in place without creating a new list.
Can I reverse a string in Python using the same method?
Yes, you can reverse a string in Python using slicing as well. For example, `string[::-1]` will return a new string that is the reverse of the original string.
What is the time complexity of reversing an array in Python?
The time complexity of reversing an array in Python is O(n), where n is the number of elements in the array. This is because each element must be accessed and rearranged.
Are there any libraries that provide functions to reverse an array?
Yes, the NumPy library offers a function called `numpy.flip()`, which can reverse the order of elements in an array. This is particularly useful for multi-dimensional arrays.
Can I reverse an array in Python using a loop?
Yes, you can reverse an array using a loop by iterating through the array from the last index to the first index and appending each element to a new array. This method is straightforward but less efficient than slicing or the `reverse()` method.
Reversing an array in Python can be accomplished through various methods, each suited to different use cases and preferences. The most common approaches include using Python’s built-in list methods, such as the `reverse()` method, which modifies the list in place, or the slicing technique, which creates a new reversed list. Additionally, the `reversed()` function can be utilized to obtain an iterator that yields elements in reverse order, allowing for flexible handling of the original array.
Each method has its advantages. The `reverse()` method is efficient for in-place modifications, while slicing is concise and often preferred for its readability. The `reversed()` function is particularly useful when you need to work with the reversed elements without altering the original array. Understanding these techniques enables developers to choose the most appropriate method based on the specific requirements of their applications.
In summary, reversing an array in Python is straightforward and can be achieved through multiple techniques. By familiarizing oneself with these methods, programmers can enhance their coding efficiency and maintain clarity in their code. Ultimately, selecting the right approach depends on the context of the task and the desired outcome.
Author Profile
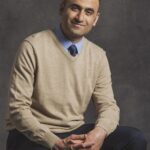
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?