How Can You Round Numbers in JavaScript Effectively?
### Introduction
In the world of programming, precision is key, especially when it comes to handling numerical data. Whether you’re developing a complex application, calculating financial transactions, or simply displaying user-friendly results, knowing how to round numbers effectively in JavaScript is an essential skill. Rounding numbers can help eliminate ambiguity, enhance readability, and ensure that your calculations yield the desired outcomes. As you delve into the nuances of JavaScript’s rounding capabilities, you’ll discover a variety of methods that cater to different scenarios, empowering you to present data in the most accurate and appealing way possible.
Understanding how to round numbers in JavaScript goes beyond just applying a simple function; it involves grasping the underlying principles of numerical representation and the various approaches available for rounding. JavaScript provides several built-in methods that cater to different rounding needs, whether you’re looking to round to the nearest integer, specify a certain number of decimal places, or even round towards positive or negative infinity. Each method serves a unique purpose, and knowing when to use them can greatly enhance the functionality of your code.
As you explore the topic further, you’ll learn about the intricacies of rounding techniques such as `Math.round()`, `Math.floor()`, and `Math.ceil()`, each offering distinct advantages depending on your requirements. By mastering
Rounding Numbers using Math.round()
The `Math.round()` function in JavaScript is a fundamental method for rounding numbers to the nearest integer. It operates by examining the decimal portion of a number and rounding it based on its value.
- If the decimal is 0.5 or greater, the number is rounded up.
- If the decimal is less than 0.5, the number is rounded down.
For example:
javascript
console.log(Math.round(4.5)); // Outputs: 5
console.log(Math.round(4.4)); // Outputs: 4
This method is straightforward and effective for simple rounding tasks.
Rounding Down with Math.floor()
In situations where you need to round a number down to the nearest integer, the `Math.floor()` function is the ideal choice. This function always rounds numbers towards negative infinity, effectively truncating the decimal part.
For instance:
javascript
console.log(Math.floor(4.9)); // Outputs: 4
console.log(Math.floor(-4.1)); // Outputs: -5
This method is particularly useful in applications where you need to ensure that values do not exceed a certain limit.
Rounding Up with Math.ceil()
Conversely, if your requirement is to round numbers up to the nearest integer, the `Math.ceil()` function should be utilized. This function rounds towards positive infinity, ensuring that any decimal value results in an increase of the integer value.
For example:
javascript
console.log(Math.ceil(4.1)); // Outputs: 5
console.log(Math.ceil(-4.9)); // Outputs: -4
Using `Math.ceil()` is advantageous when working with scenarios where you want to ensure a minimum threshold is met.
Rounding to a Specific Decimal Place
When rounding to a specific number of decimal places, a custom function can be created. This function utilizes multiplication and division to achieve the desired precision.
javascript
function roundToDecimalPlace(num, decimalPlaces) {
const factor = Math.pow(10, decimalPlaces);
return Math.round(num * factor) / factor;
}
console.log(roundToDecimalPlace(4.56789, 2)); // Outputs: 4.57
This method provides flexibility for applications requiring specific decimal rounding.
Summary of Rounding Methods
Method | Description | Example |
---|---|---|
Math.round() | Rounds to the nearest integer. | Math.round(4.5) returns 5 |
Math.floor() | Rounds down to the nearest integer. | Math.floor(4.9) returns 4 |
Math.ceil() | Rounds up to the nearest integer. | Math.ceil(4.1) returns 5 |
Custom Function | Rounds to a specified number of decimal places. | roundToDecimalPlace(4.56789, 2) returns 4.57 |
These methods provide a comprehensive toolkit for rounding numbers effectively within JavaScript applications.
Rounding Numbers in JavaScript
In JavaScript, rounding numbers can be accomplished through several built-in methods that provide flexibility depending on the desired outcome. The primary functions include `Math.round()`, `Math.floor()`, `Math.ceil()`, and `Math.trunc()`. Each function serves a different purpose and is suited for various scenarios.
Math.round()
The `Math.round()` function rounds a number to the nearest integer. If the fractional part is 0.5 or greater, it rounds up; otherwise, it rounds down.
javascript
console.log(Math.round(4.5)); // Outputs: 5
console.log(Math.round(4.4)); // Outputs: 4
Math.floor()
The `Math.floor()` function always rounds down to the nearest integer, regardless of the fractional part.
javascript
console.log(Math.floor(4.9)); // Outputs: 4
console.log(Math.floor(-4.1)); // Outputs: -5
Math.ceil()
The `Math.ceil()` function, in contrast to `Math.floor()`, always rounds up to the nearest integer.
javascript
console.log(Math.ceil(4.1)); // Outputs: 5
console.log(Math.ceil(-4.9)); // Outputs: -4
Math.trunc()
The `Math.trunc()` function removes the fractional part of a number, effectively truncating it to an integer without rounding.
javascript
console.log(Math.trunc(4.9)); // Outputs: 4
console.log(Math.trunc(-4.9)); // Outputs: -4
Rounding to a Specific Decimal Place
To round numbers to a specific decimal place, a combination of multiplication, rounding, and division is used. Here’s how to implement this:
- Multiply the number by 10 raised to the power of the desired decimal places.
- Apply the rounding method (`Math.round()`, `Math.floor()`, or `Math.ceil()`).
- Divide by the same power of 10.
javascript
function roundToDecimalPlace(num, decimalPlaces) {
const factor = Math.pow(10, decimalPlaces);
return Math.round(num * factor) / factor;
}
console.log(roundToDecimalPlace(4.5678, 2)); // Outputs: 4.57
console.log(roundToDecimalPlace(4.5678, 3)); // Outputs: 4.568
Using Number.toFixed()
The `Number.prototype.toFixed()` method formats a number using fixed-point notation. It returns a string representing the number with the specified number of digits after the decimal point.
javascript
let num = 4.5678;
console.log(num.toFixed(2)); // Outputs: “4.57”
console.log(num.toFixed(3)); // Outputs: “4.568”
Note that `toFixed()` returns a string, so further numeric operations may require conversion back to a number.
Comparative Table of Rounding Functions
Function | Behavior | Example |
---|---|---|
Math.round() | Rounds to nearest integer | Math.round(4.5) // 5 |
Math.floor() | Rounds down | Math.floor(4.9) // 4 |
Math.ceil() | Rounds up | Math.ceil(4.1) // 5 |
Math.trunc() | Removes decimal part | Math.trunc(4.9) // 4 |
Number.toFixed() | Formats number to fixed decimal | (4.5678).toFixed(2) // “4.57” |
Expert Insights on Rounding Numbers in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Rounding numbers in JavaScript can be accomplished using built-in methods such as Math.round(), Math.floor(), and Math.ceil(). Each method serves a specific purpose, allowing developers to choose the appropriate rounding strategy based on their application’s requirements.”
Michael Chen (JavaScript Developer, CodeCraft Academy). “Understanding the nuances of rounding in JavaScript is crucial for accurate calculations. For instance, using Math.round() will round to the nearest integer, while Math.floor() and Math.ceil() will always round down or up, respectively. This distinction can significantly affect financial applications or any scenario requiring precision.”
Sarah Thompson (Technical Writer, Web Development Weekly). “When rounding numbers in JavaScript, it is essential to be aware of floating-point precision issues. Developers should consider using libraries like Decimal.js for more complex rounding tasks to avoid unexpected results that arise from JavaScript’s handling of floating-point arithmetic.”
Frequently Asked Questions (FAQs)
How do I round a number to the nearest integer in JavaScript?
You can use the `Math.round()` function. For example, `Math.round(4.5)` will return `5`, while `Math.round(4.4)` will return `4`.
What function should I use to round a number down in JavaScript?
To round a number down, use the `Math.floor()` function. For instance, `Math.floor(4.9)` will return `4`.
How can I round a number up in JavaScript?
Use the `Math.ceil()` function to round a number up. For example, `Math.ceil(4.1)` will return `5`.
Is there a way to round a number to a specific decimal place in JavaScript?
Yes, you can round to a specific decimal place by multiplying the number by a power of 10, applying `Math.round()`, and then dividing by the same power of 10. For example, to round `5.567` to two decimal places: `Math.round(5.567 * 100) / 100` will yield `5.57`.
Can I round numbers using the `toFixed()` method in JavaScript?
Yes, the `toFixed()` method can be used to format a number to a specified number of decimal places. For instance, `(5.567).toFixed(2)` will return the string `”5.57″`.
What is the difference between `Math.round()`, `Math.floor()`, and `Math.ceil()`?
`Math.round()` rounds to the nearest integer, `Math.floor()` always rounds down to the nearest integer, and `Math.ceil()` always rounds up to the nearest integer. Each function serves different rounding needs based on the desired outcome.
Rounding numbers in JavaScript is a fundamental operation that can be accomplished using several built-in methods. The primary functions for rounding include `Math.round()`, `Math.floor()`, and `Math.ceil()`, each serving a distinct purpose. `Math.round()` rounds a number to the nearest integer, while `Math.floor()` always rounds down to the nearest integer, and `Math.ceil()` rounds up to the nearest integer. Understanding these functions allows developers to manipulate numerical data effectively, catering to various requirements in programming.
In addition to these basic functions, JavaScript also provides `Math.trunc()`, which removes the decimal part of a number without rounding. This can be particularly useful when a simple truncation is needed rather than traditional rounding. Furthermore, for more complex rounding scenarios, such as rounding to a specific number of decimal places, developers can implement custom functions to achieve the desired results. This versatility in rounding methods highlights the flexibility of JavaScript in handling numerical operations.
Overall, mastering these rounding techniques is essential for any JavaScript programmer. By leveraging the appropriate rounding functions, developers can ensure that their applications handle numerical data accurately and efficiently. Whether it’s for financial calculations, user input processing, or data visualization, understanding how to
Author Profile
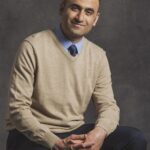
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?