How Can You Run a File in Python? A Step-by-Step Guide
In the vast landscape of programming, Python stands out as one of the most accessible and versatile languages available today. Whether you’re a seasoned developer or a curious beginner, the ability to run files in Python is a fundamental skill that opens the door to countless possibilities. From automating mundane tasks to developing complex applications, understanding how to execute Python scripts effectively can significantly enhance your coding journey. In this article, we will explore the various methods to run Python files, providing you with the tools and knowledge to bring your ideas to life.
Running a file in Python may seem straightforward, but it encompasses a variety of techniques suited to different environments and use cases. Whether you prefer the simplicity of command-line execution, the convenience of integrated development environments (IDEs), or the flexibility of online interpreters, each method has its own set of advantages. As you delve deeper into the world of Python, mastering these execution methods will empower you to test your code, debug errors, and streamline your development process.
Moreover, understanding how to run Python files is not just about execution; it’s also about grasping the underlying principles that govern how Python interacts with your operating system and the runtime environment. This knowledge will not only bolster your coding skills but also enhance your problem-solving abilities as you navigate through various projects.
Running a Python Script from the Command Line
To execute a Python file from the command line, you need to ensure that Python is installed on your system and that the command line interface is set up correctly. Here’s how you can run a Python script:
- Open your command line interface (CLI). This can be Command Prompt on Windows, Terminal on macOS, or a shell on Linux.
- Navigate to the directory where your Python file is located using the `cd` command. For example:
bash
cd path/to/your/script
- Run the script by typing the following command:
bash
python filename.py
Alternatively, if you are using Python 3 specifically, you might need to use:
bash
python3 filename.py
It is essential to replace `filename.py` with the actual name of your Python file.
Using an Integrated Development Environment (IDE)
Running a Python file can also be accomplished using an Integrated Development Environment (IDE) such as PyCharm, Visual Studio Code, or Jupyter Notebook. Here’s a brief overview of how to run a script in these environments:
- PyCharm:
- Open the project where your Python file is located.
- Right-click on the file in the project explorer.
- Select “Run ‘filename'” from the context menu.
- Visual Studio Code:
- Open the folder containing your Python file.
- Open the file and click on the green play button in the top right corner or use the terminal within VS Code:
bash
python filename.py
- Jupyter Notebook:
- Open Jupyter Notebook in your browser.
- Create a new notebook or open an existing one.
- Run code cells containing Python code by clicking on the “Run” button or pressing `Shift + Enter`.
Executing Python Scripts from Within Another Python Script
You can also run a Python file from within another Python script using the `import` statement or the `subprocess` module. Here’s how each method works:
- Using `import`:
- If you want to use functions or classes defined in another Python file, you can import it:
python
import filename
filename.function_name()
- Using `subprocess`:
- To execute the script as a separate process:
python
import subprocess
subprocess.run([‘python’, ‘filename.py’])
Common Issues and Troubleshooting
When running Python scripts, you may encounter various issues. Here are some common problems and their solutions:
Issue | Solution |
---|---|
Python is not recognized | Ensure Python is installed and added to PATH. |
Syntax errors in the script | Check the script for any syntax errors or typos. |
Module not found | Install the required module using pip. |
Permissions denied | Run the script with the necessary permissions. |
By following these methods and troubleshooting tips, you can efficiently run Python files on different platforms and within various environments.
Running a Python File from the Command Line
To execute a Python script from the command line, ensure you have Python installed on your system. The following steps outline how to run a Python file using various operating systems.
Windows
- Open the Command Prompt.
- Navigate to the directory where your Python file is located using the `cd` command.
Example:
bash
cd path\to\your\script
- Run the Python file by entering:
bash
python filename.py
macOS/Linux
- Open a terminal window.
- Change the directory to where your Python file resides:
bash
cd /path/to/your/script
- Execute the file with:
bash
python3 filename.py
Using an Integrated Development Environment (IDE)
Running a Python file within an IDE is straightforward and often more user-friendly. Popular IDEs include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
Steps to Run in an IDE:
- Open your IDE.
- Load your Python file.
- Look for a “Run” option, usually represented by a play button or through a menu option.
- Alternatively, use keyboard shortcuts, typically `Shift + F10` in PyCharm or `F5` in Visual Studio Code.
Executing Python Scripts in a Jupyter Notebook
Jupyter Notebook allows for interactive execution of Python code in blocks. To run a Python file:
- Launch Jupyter Notebook from your terminal:
bash
jupyter notebook
- Navigate to the directory containing your Python file.
- Open the file by clicking on it.
- Run individual cells using `Shift + Enter`, or run all cells via the menu.
Running Python Files as Executables
You can make Python scripts executable on Unix-like systems. This is useful for scripts that you wish to run without explicitly invoking the Python interpreter.
Steps:
- Add a shebang line to the top of your Python script:
python
#!/usr/bin/env python3
- Change the file permissions to make it executable:
bash
chmod +x filename.py
- Run the script directly:
bash
./filename.py
Running Python Files with Arguments
Often, Python scripts require command-line arguments. You can pass these when executing your script.
Example Command:
bash
python filename.py arg1 arg2
Accessing Arguments in Code:
Use the `sys` module to handle command-line arguments:
python
import sys
# sys.argv is a list that contains command-line arguments
arg1 = sys.argv[1]
arg2 = sys.argv[2]
Common Errors and Troubleshooting
When running Python files, you may encounter errors. Here are some common issues and their solutions:
Error Type | Description | Solution |
---|---|---|
`ModuleNotFoundError` | A module is not found. | Ensure the module is installed (e.g., `pip install module_name`). |
`SyntaxError` | There’s a syntax issue in your code. | Check for typos and improper syntax usage. |
`PermissionError` | Lack of permissions to execute the file. | Change file permissions using `chmod`. |
Ensure to review error messages carefully as they often indicate the issue’s location within the code.
Expert Insights on Running Files in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To run a file in Python, one must ensure that the Python interpreter is correctly installed. The command ‘python filename.py’ in the terminal or command prompt will execute the script, provided the file is in the current directory.”
Michael Thompson (Lead Python Developer, CodeCraft Solutions). “Utilizing an Integrated Development Environment (IDE) like PyCharm or VSCode can streamline the process of running Python files. These tools offer features such as debugging and code completion, which enhance productivity.”
Sarah Patel (Python Instructor, LearnPython Academy). “For beginners, it is essential to understand the difference between running scripts in interactive mode versus script mode. Using the command line to execute scripts is a foundational skill that will aid in mastering Python programming.”
Frequently Asked Questions (FAQs)
How do I run a Python file from the command line?
To run a Python file from the command line, navigate to the directory containing the file and use the command `python filename.py`, replacing `filename.py` with the actual name of your Python file.
Can I run a Python file directly from an IDE?
Yes, most Integrated Development Environments (IDEs) such as PyCharm, Visual Studio Code, or Jupyter Notebook allow you to run Python files directly by clicking a “Run” button or using a specific keyboard shortcut.
What is the difference between running a Python script and a Python module?
Running a Python script typically refers to executing a standalone file, while running a module involves importing it into another script or interactive session to utilize its functions and classes.
How can I run a Python file in the background?
To run a Python file in the background, you can use the command `python filename.py &` in Unix-based systems or use a task scheduler in Windows to execute the script without a command prompt window.
Is it possible to run a Python file with arguments?
Yes, you can run a Python file with arguments by using the command `python filename.py arg1 arg2`, and you can access these arguments within your script using the `sys.argv` list.
What should I do if I encounter a “Permission denied” error when running a Python file?
If you encounter a “Permission denied” error, ensure that you have the necessary permissions to execute the file. You may need to change the file’s permissions using `chmod +x filename.py` in Unix-based systems or run the command prompt as an administrator in Windows.
Running a file in Python is a straightforward process that can be accomplished using various methods, depending on the context and requirements of the task. The most common approach is to use the command line or terminal, where you can execute a Python script by typing `python filename.py` or `python3 filename.py`, depending on your Python installation. This method is efficient for running standalone scripts and is widely used in development environments.
Another effective way to run a Python file is through Integrated Development Environments (IDEs) or code editors, such as PyCharm, Visual Studio Code, or Jupyter Notebook. These tools provide user-friendly interfaces that allow you to run scripts with a simple click or keyboard shortcut, enhancing productivity and reducing the likelihood of errors. Additionally, IDEs often come with debugging features that can be invaluable during the development process.
For those looking to execute Python code programmatically, the `exec()` function can be utilized to run Python code contained in a string or file. This method is less common but can be useful for dynamic code execution scenarios. However, it is essential to use this approach cautiously, as executing arbitrary code can pose security risks.
In summary, whether you choose to run a Python file via
Author Profile
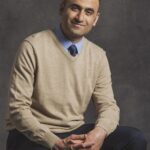
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?