How Can You Run a JavaScript File in the Terminal?
Introduction
In the ever-evolving world of web development, JavaScript stands as a cornerstone language, powering everything from interactive websites to complex server-side applications. While many developers are accustomed to running JavaScript in the browser, there’s a whole new dimension to explore when it comes to executing JavaScript files directly from the terminal. Whether you’re automating tasks, building command-line tools, or simply experimenting with code, knowing how to run a JavaScript file in the terminal can significantly enhance your development workflow.
As we delve into this topic, we’ll uncover the various methods and tools available for executing JavaScript outside of the browser environment. From leveraging popular runtime environments like Node.js to exploring alternative approaches, you’ll gain insights into the flexibility and power that terminal execution offers. This knowledge not only broadens your skill set but also opens up new avenues for creating efficient, scalable applications that can run seamlessly on your local machine.
Prepare to unlock the potential of your JavaScript skills as we guide you through the essential steps and best practices for running JavaScript files in the terminal. Whether you’re a seasoned developer or just starting your coding journey, this exploration promises to equip you with the tools you need to take your projects to the next level.
Using Node.js to Execute JavaScript Files
To run a JavaScript file in the terminal, you typically utilize Node.js, a JavaScript runtime built on Chrome’s V8 engine. Ensure Node.js is installed on your system. You can verify this by executing the command `node -v` in your terminal, which should return the version number.
Once Node.js is confirmed to be installed, follow these steps to execute a JavaScript file:
- Create a JavaScript file: Use a text editor to create a new file with a `.js` extension. For example, `app.js`. Write your JavaScript code within this file.
- Open the terminal: Navigate to the directory where your JavaScript file is located. You can use the `cd` command to change directories. For example:
bash
cd path/to/your/directory
- Run the JavaScript file: Execute the following command in the terminal:
bash
node app.js
This command tells Node.js to run the specified JavaScript file. Any output from your script will be displayed in the terminal.
Command Line Arguments
Node.js also allows you to pass command line arguments to your JavaScript file, enhancing its functionality. You can access these arguments using the `process.argv` array.
Example Usage:
- Modify your `app.js` file to include code that processes command line arguments:
javascript
const args = process.argv.slice(2);
console.log(‘Arguments passed:’, args);
- Run the file with arguments:
bash
node app.js arg1 arg2
This will output:
Arguments passed: [ ‘arg1’, ‘arg2’ ]
Common Errors and Troubleshooting
When executing JavaScript files in the terminal, you may encounter several common issues. Below is a table summarizing these errors and their solutions:
Error | Solution |
---|---|
Command not found | Ensure Node.js is installed and properly added to your system PATH. |
SyntaxError | Check your JavaScript code for syntax mistakes. |
Module not found | Make sure you have installed all required npm packages. |
By following these guidelines, you can effectively run JavaScript files in the terminal, enhancing your development workflow and enabling you to leverage the full power of JavaScript in a command line environment.
Prerequisites for Running JavaScript in the Terminal
To execute JavaScript files in the terminal, ensure you have the following prerequisites:
- Node.js Installed: Node.js is a JavaScript runtime built on Chrome’s V8 engine. It allows you to run JavaScript code outside of a web browser.
- Terminal Access: Familiarity with command-line interfaces is necessary, as you will be using terminal commands.
- Text Editor: A code editor or text editor (like VSCode, Sublime Text, or even Notepad) for writing your JavaScript code.
Installing Node.js
If Node.js is not yet installed on your system, follow these steps based on your operating system:
Operating System | Installation Steps |
---|---|
Windows |
|
macOS |
|
Linux |
|
Creating a JavaScript File
To run a JavaScript file, you first need to create one. Follow these steps:
- Open your text editor.
- Create a new file and save it with a `.js` extension. For example, `script.js`.
- Write your JavaScript code in the file. For example:
javascript
console.log(“Hello, World!”);
- Save the file.
Running the JavaScript File in Terminal
Once you have your JavaScript file ready, you can execute it in the terminal with the following steps:
- Open your terminal.
- Navigate to the directory where your JavaScript file is located using the `cd` command. For example:
bash
cd path/to/your/directory
- Run the JavaScript file using Node.js with the command:
bash
node script.js
Replace `script.js` with the name of your file.
Common Errors and Troubleshooting
While running JavaScript files, you may encounter some common errors. Here are solutions for a few of them:
- Error: command not found: This typically indicates that Node.js is not installed or not added to your system’s PATH. Ensure Node.js is properly installed.
- Error: Cannot find module: This error suggests that the specified JavaScript file does not exist in the directory you are currently in. Verify the file name and path.
- Syntax errors: These will be displayed in the terminal. Check your code for typos or incorrect syntax.
By following these steps, you can effectively run JavaScript files in the terminal using Node.js, allowing for efficient testing and development of your code.
Expert Insights on Running JavaScript Files in Terminal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a JavaScript file in the terminal, you need to have Node.js installed on your system. Once installed, you can execute your JavaScript file by navigating to its directory in the terminal and using the command ‘node filename.js’. This allows you to run server-side JavaScript easily.”
Michael Chen (Lead Developer, CodeCraft Solutions). “Using the terminal to run JavaScript files is a powerful way to test and debug your code. Make sure your terminal is open in the same directory as your JavaScript file, and remember to check for any syntax errors before executing the command, as they can halt the execution process.”
Sarah Patel (Web Development Instructor, Digital Academy). “For beginners, understanding how to run JavaScript files in the terminal is crucial. Start by familiarizing yourself with terminal commands and the Node.js environment. This skill will enhance your ability to develop and deploy applications effectively.”
Frequently Asked Questions (FAQs)
How do I run a JavaScript file in the terminal?
To run a JavaScript file in the terminal, use Node.js. First, ensure Node.js is installed on your system. Then, navigate to the directory containing your JavaScript file using the `cd` command and execute the file by typing `node filename.js`, replacing `filename.js` with the actual name of your file.
What is Node.js?
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to execute JavaScript code on the server side, enabling the creation of scalable network applications.
Can I run JavaScript files without Node.js?
Yes, you can run JavaScript files in a browser’s developer console. However, for terminal execution, Node.js is the most common and efficient method.
What do I need to install Node.js?
To install Node.js, download the installer from the official Node.js website. Follow the installation instructions for your operating system, and ensure that the installation path is added to your system’s environment variables.
How can I check if Node.js is installed?
You can check if Node.js is installed by typing `node -v` in your terminal. If Node.js is installed, this command will return the version number. If not, you will receive an error message.
What should I do if I encounter an error when running a JavaScript file?
If you encounter an error, check the error message for details. Common issues include syntax errors in your JavaScript code or incorrect file paths. Ensure your code is error-free and that you are in the correct directory when executing the command.
In summary, running a JavaScript file in the terminal is a straightforward process that primarily involves the use of Node.js, a popular JavaScript runtime. To execute a JavaScript file, one must first ensure that Node.js is installed on their system. Once installed, users can navigate to the directory containing the JavaScript file using terminal commands and then execute the file by typing `node filename.js`. This method allows for efficient testing and execution of JavaScript code outside of a browser environment.
Additionally, it is essential to understand that the terminal provides a powerful interface for running scripts, enabling developers to quickly debug and test their code. By using the terminal, developers can also leverage various command-line options that enhance their coding workflow, such as running scripts with different Node.js flags or using package managers like npm to manage dependencies.
Overall, mastering the ability to run JavaScript files in the terminal not only improves productivity but also deepens one’s understanding of JavaScript as a versatile programming language. It is a fundamental skill for developers looking to streamline their development process and effectively utilize JavaScript in various environments.
Author Profile
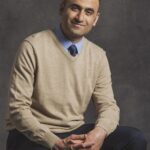
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?