How Can You Run a Python Script on Linux?
Introduction
In the world of programming, Python stands out as one of the most versatile and user-friendly languages, making it a popular choice for developers, data scientists, and hobbyists alike. Whether you’re automating mundane tasks, analyzing data, or developing complex applications, knowing how to run a Python script on Linux can significantly enhance your productivity and streamline your workflow. This article will guide you through the essentials of executing Python scripts in a Linux environment, unlocking the full potential of this powerful programming language.
Running a Python script on Linux is a straightforward process, but it involves several key steps that can vary depending on your setup and specific requirements. From ensuring you have the correct version of Python installed to navigating the terminal and executing your scripts, understanding the fundamentals will empower you to harness Python’s capabilities effectively. Additionally, you’ll learn about the various ways to run scripts, whether it’s through the command line or by utilizing integrated development environments (IDEs).
As we delve deeper into the intricacies of executing Python scripts, you’ll discover tips and tricks that can simplify your coding experience. Whether you’re a seasoned developer or just starting your programming journey, this guide will equip you with the knowledge needed to run Python scripts seamlessly on your Linux system, paving the way for your next coding adventure.
Executing Python Scripts from the Terminal
To run a Python script on a Linux system, you will typically use the terminal. The terminal serves as a command line interface where you can execute commands directly. Here are the steps to follow:
- Open the Terminal: You can usually find the terminal application in your system’s applications menu. Alternatively, you can use the shortcut `Ctrl + Alt + T`.
- Navigate to the Script’s Directory: Use the `cd` command to change the directory to where your Python script is located. For example:
cd /path/to/your/script
- Run the Script: You can execute the script by typing `python` or `python3`, followed by the script name. For instance:
python3 my_script.py
### Shebang Line
Including a shebang line at the top of your Python script can simplify execution. The shebang line tells the system which interpreter to use. Add the following line as the first line of your script:
python
#!/usr/bin/env python3
Make sure to give execution permissions to your script:
bash
chmod +x my_script.py
After this, you can run your script directly:
bash
./my_script.py
Using Virtual Environments
When working with Python, especially for larger projects, it’s advisable to use virtual environments. This helps to manage dependencies effectively. Here’s how to create and activate a virtual environment:
- Install `venv` (if not installed):
bash
sudo apt install python3-venv
- Create a Virtual Environment:
bash
python3 -m venv myenv
- Activate the Virtual Environment:
bash
source myenv/bin/activate
Once activated, you can install packages using `pip`, and run your Python scripts as mentioned earlier.
### Common Commands for Python Execution
Below is a table summarizing common commands used to run Python scripts:
Command | Description |
---|---|
python script.py | Runs the script using the default Python interpreter. |
python3 script.py | Runs the script using Python 3. |
./script.py | Executes the script directly if it has a shebang and execute permissions. |
python -m module_name | Runs a module as a script. |
Running Scripts in the Background
If you need to run a script in the background, you can append an ampersand (`&`) at the end of your command:
bash
python3 my_script.py &
To see the background jobs, you can use the `jobs` command. If you need to bring a job back to the foreground, use the `fg` command followed by the job number.
Using these methods, you can efficiently run Python scripts on your Linux system, leveraging the terminal and virtual environments to manage your development workflow effectively.
Setting Up Python on Linux
To run a Python script on Linux, ensure that Python is installed on your system. Most Linux distributions come with Python pre-installed, but verifying the installation is essential.
To check your Python installation, open a terminal and enter:
bash
python –version
or for Python 3:
bash
python3 –version
If Python is not installed, you can install it using your distribution’s package manager. Here are commands for common distributions:
- Debian/Ubuntu:
bash
sudo apt update
sudo apt install python3
- Fedora:
bash
sudo dnf install python3
- Arch Linux:
bash
sudo pacman -S python
Writing a Python Script
Create a Python script using any text editor of your choice. The following example demonstrates a simple script:
- Open a terminal and create a new file:
bash
nano my_script.py
- Write your Python code inside the file. For instance:
python
print(“Hello, World!”)
- Save and exit the editor (in nano, press `CTRL + X`, then `Y`, and `Enter`).
Running the Python Script
To execute the Python script, navigate to the directory where your script is located. Use the `cd` command to change directories. For example:
bash
cd /path/to/your/script
Once in the correct directory, run the script using one of the following commands, depending on your Python version:
- For Python 3:
bash
python3 my_script.py
- For Python 2 (if applicable):
bash
python my_script.py
Making the Script Executable
To run a Python script without explicitly calling Python, you can make it executable. Follow these steps:
- Add a shebang line at the top of your script:
python
#!/usr/bin/env python3
- Change the file permissions to make it executable:
bash
chmod +x my_script.py
- Now you can run the script directly:
bash
./my_script.py
Using Virtual Environments
For managing dependencies, using a virtual environment is recommended. This isolates your project’s packages from the system Python installation. To create and use a virtual environment:
- Install the `venv` module if not already available:
bash
sudo apt install python3-venv
- Create a virtual environment:
bash
python3 -m venv myenv
- Activate the virtual environment:
bash
source myenv/bin/activate
- Install any required packages using `pip` within the virtual environment:
bash
pip install package_name
- Run your script as before while the virtual environment is active.
Troubleshooting Common Issues
When running Python scripts, you may encounter various issues. Here are some common problems and solutions:
Issue | Solution |
---|---|
Command not found | Ensure Python is installed and added to your PATH. |
Permission denied | Check file permissions or run with `sudo` if necessary. |
ModuleNotFoundError | Install the required module via `pip` or check the virtual environment. |
By following these guidelines, you can effectively run Python scripts on a Linux system while adhering to best practices for environment management and script execution.
Expert Insights on Running Python Scripts in Linux
Dr. Emily Carter (Senior Software Engineer, Open Source Innovations). “To effectively run a Python script on Linux, one must ensure that Python is installed and accessible via the command line. Utilizing the terminal, you can execute your script by navigating to its directory and using the command ‘python script_name.py’ or ‘python3 script_name.py’ depending on your Python version.”
Michael Chen (Linux Systems Administrator, Tech Solutions Inc.). “When running Python scripts on Linux, it is crucial to set the executable permission for your script file. This can be achieved by using the command ‘chmod +x script_name.py’. After this, you can run the script directly with ‘./script_name.py’ if the shebang line is correctly set at the top of the file.”
Sarah Johnson (DevOps Engineer, CloudTech Enterprises). “For automation purposes, consider using a task scheduler like cron to run your Python scripts at specified intervals. This approach not only saves time but also ensures that your scripts are executed consistently without manual intervention.”
Frequently Asked Questions (FAQs)
How do I run a Python script from the terminal in Linux?
To run a Python script from the terminal, navigate to the directory containing the script using the `cd` command, then execute the script by typing `python script_name.py` or `python3 script_name.py`, depending on your Python version.
What permissions are required to run a Python script on Linux?
The script must have execute permissions. You can set these permissions using the command `chmod +x script_name.py`. After setting the permissions, you can run the script with `./script_name.py`.
Can I run a Python script without specifying the Python interpreter?
Yes, you can use a shebang line at the top of your script, such as `#!/usr/bin/env python3`, which allows you to run the script directly with `./script_name.py` after making it executable.
What should I do if I encounter a “command not found” error?
This error typically indicates that Python is not installed or not added to your PATH. Ensure Python is installed by running `python –version` or `python3 –version`, and if necessary, install it using your package manager.
How can I pass command-line arguments to my Python script?
You can pass command-line arguments by adding them after the script name in the terminal, like this: `python script_name.py arg1 arg2`. Inside your script, use the `sys.argv` list to access these arguments.
Is it possible to schedule a Python script to run automatically on Linux?
Yes, you can use `cron` to schedule your Python script. Edit the crontab with `crontab -e` and add a line specifying the schedule and the command to run your script, such as `0 * * * * /usr/bin/python3 /path/to/script_name.py`.
Running a Python script on Linux is a straightforward process that can be accomplished through several methods. The most common approach involves using the terminal, where users can navigate to the directory containing the script and execute it using the `python` or `python3` command followed by the script’s filename. It is essential to ensure that the Python interpreter is installed on the system, which can typically be verified by running `python –version` or `python3 –version` in the terminal.
Another effective method for executing Python scripts is by making the script file executable. This can be done by adding a shebang line at the top of the script (e.g., `#!/usr/bin/env python3`) and then changing the file permissions using the `chmod +x script_name.py` command. Once this is set, the script can be run directly from the terminal by simply typing `./script_name.py`, enhancing the convenience of execution.
Additionally, users can employ integrated development environments (IDEs) or text editors that support Python, such as PyCharm or Visual Studio Code, to run scripts more interactively. These tools provide features like debugging, code completion, and version control integration, which can significantly enhance the development experience. Overall,
Author Profile
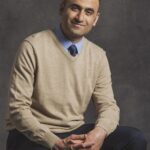
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?