How Can You Run a TypeScript File Effectively?
In the ever-evolving world of web development, TypeScript has emerged as a powerful ally for developers seeking to enhance their JavaScript projects with static typing and robust tooling. Whether you’re a seasoned programmer or just dipping your toes into the waters of TypeScript, knowing how to effectively run a TypeScript file is crucial for bringing your code to life. This article will guide you through the essential steps and best practices for executing TypeScript files, ensuring you harness the full potential of this versatile language.
As you embark on your TypeScript journey, it’s important to understand that running a TypeScript file involves a few key processes. Unlike traditional JavaScript files, TypeScript needs to be compiled into JavaScript before it can be executed in a browser or Node.js environment. This compilation process not only transforms your TypeScript code into a format that can be understood by the JavaScript engine but also provides opportunities for type-checking and error detection.
In the following sections, we will explore the various methods to run TypeScript files, from using the TypeScript compiler (tsc) to leveraging modern development environments and build tools. Whether you’re looking to streamline your workflow or simply curious about the mechanics behind executing TypeScript, this guide will equip you with the knowledge you need to confidently run your
Using Node.js to Run TypeScript Files
To execute a TypeScript file, the most common approach is to utilize Node.js along with the TypeScript compiler (tsc). This method allows you to run TypeScript code in a JavaScript runtime environment.
- Install Node.js: Ensure that you have Node.js installed on your machine. You can download it from the official [Node.js website](https://nodejs.org/).
- Install TypeScript: After installing Node.js, you can install TypeScript globally using npm (Node Package Manager). Run the following command in your terminal:
“`
npm install -g typescript
“`
- Compile the TypeScript File: Use the TypeScript compiler to compile your `.ts` file to JavaScript. For example, if your TypeScript file is named `app.ts`, execute:
“`
tsc app.ts
“`
This command will generate an `app.js` file in the same directory.
- Run the Compiled JavaScript File: After compilation, you can run the generated JavaScript file using Node.js:
“`
node app.js
“`
This process converts your TypeScript code into a format that Node.js can execute.
Using ts-node for Direct Execution
An alternative and more convenient way to run TypeScript files without manual compilation is to use `ts-node`. This utility allows you to run TypeScript files directly.
- Install ts-node: If you haven’t installed it yet, do so using npm:
“`
npm install -g ts-node
“`
- Run the TypeScript File: You can now execute your TypeScript file directly with the following command:
“`
ts-node app.ts
“`
This method saves time as it bypasses the need to compile your TypeScript code manually into JavaScript first.
Setting Up a TypeScript Project
For larger projects, it’s advisable to set up a TypeScript project with a configuration file. This file controls the compiler options and includes various settings.
- Initialize a Project: Create a new directory for your project and navigate into it. Then, run:
“`
npm init -y
“`
- Install TypeScript Locally: Install TypeScript as a development dependency:
“`
npm install –save-dev typescript
“`
- Create a tsconfig.json File: Generate a configuration file with:
“`
npx tsc –init
“`
This creates a `tsconfig.json` file with default settings. You can customize options like `target`, `module`, and `outDir` as needed.
- Compile and Run: You can compile the entire project with:
“`
npx tsc
“`
Then run the output JavaScript files using Node.js.
Comparative Overview of Running TypeScript
The following table summarizes the methods available to run TypeScript files:
Method | Description | Command Example |
---|---|---|
Node.js + tsc | Compile to JavaScript and run | tsc app.ts && node app.js |
ts-node | Run TypeScript files directly | ts-node app.ts |
Project Setup | Use tsconfig.json for configurations | npx tsc |
Each method has its own use cases depending on the project’s complexity and requirements. Choose the one that best fits your development workflow.
Setting Up Your Environment
To run a TypeScript file, you need to have your development environment properly configured. Follow these steps to ensure you are set up correctly:
- Install Node.js: TypeScript runs on Node.js, so ensure you have it installed. Download it from [Node.js official website](https://nodejs.org/) and follow the installation instructions for your operating system.
- Install TypeScript: Once Node.js is installed, open your terminal or command prompt and run the following command to install TypeScript globally:
“`bash
npm install -g typescript
“`
- Verify Installation: After installation, verify that TypeScript is installed correctly by checking the version:
“`bash
tsc -v
“`
Compiling TypeScript Files
TypeScript files have a `.ts` extension. To run a TypeScript file, it must first be compiled to JavaScript. Use the TypeScript compiler (`tsc`) for this process:
- Navigate to your project directory where the TypeScript file is located.
- Run the following command to compile your TypeScript file:
“`bash
tsc filename.ts
“`
This command generates a corresponding `.js` file in the same directory.
Running the Compiled JavaScript
After compiling the TypeScript file, you can execute the generated JavaScript using Node.js. Execute the following command:
“`bash
node filename.js
“`
This command runs the JavaScript file in the Node.js environment.
Using ts-node for Direct Execution
For a more streamlined approach, you can use `ts-node`, which allows you to run TypeScript files directly without manual compilation:
- Install ts-node: You can install `ts-node` globally using npm:
“`bash
npm install -g ts-node
“`
- Run TypeScript File: After installation, you can run your TypeScript file directly with:
“`bash
ts-node filename.ts
“`
This method is particularly useful for development and testing, as it eliminates the need for a separate compilation step.
Configuring TypeScript Options
To customize the TypeScript compilation process, you can create a `tsconfig.json` file in your project directory. Here’s how to set it up:
- Create tsconfig.json: Run the following command in your terminal:
“`bash
tsc –init
“`
- Modify tsconfig.json: Open the `tsconfig.json` file and adjust the options according to your needs. Key properties include:
- `target`: Specify the JavaScript version (e.g., “ES5”, “ES6”).
- `module`: Define the module system (e.g., “commonjs”, “esnext”).
- `outDir`: Specify the output directory for compiled files.
Here’s a sample configuration:
“`json
{
“compilerOptions”: {
“target”: “es6”,
“module”: “commonjs”,
“outDir”: “./dist”
}
}
“`
- Compile with tsconfig.json: Simply run:
“`bash
tsc
“`
This command compiles all TypeScript files in the project according to the specified options in `tsconfig.json`.
Best Practices for Running TypeScript
- Always keep your TypeScript version updated to leverage the latest features and improvements.
- Use a build tool like Webpack or Gulp for larger projects to automate the compilation process.
- Regularly test your TypeScript code to catch errors early in the development cycle.
Expert Insights on Running TypeScript Files
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To run a TypeScript file, it is essential first to ensure that TypeScript is installed globally on your machine using npm. After that, you can compile your TypeScript file into JavaScript using the `tsc` command, followed by the filename. This process generates a JavaScript file that can be executed using Node.js.”
Michael Patel (Lead Developer, CodeCraft Solutions). “Once you have your TypeScript file ready, running it can be done in a couple of ways. If you prefer a quick execution without manual compilation, consider using `ts-node`, which allows you to run TypeScript files directly. This tool is particularly useful during development for rapid testing and iteration.”
Sarah Thompson (Technical Writer, DevGuide Publications). “In addition to using `tsc` and `ts-node`, it’s beneficial to set up a build process using tools like Webpack or Gulp. This approach not only automates the compilation of TypeScript files but also integrates other tasks such as minification and bundling, which are crucial for production-ready applications.”
Frequently Asked Questions (FAQs)
How do I run a TypeScript file directly using Node.js?
To run a TypeScript file directly using Node.js, first, ensure that you have TypeScript installed globally. You can install it using `npm install -g typescript`. Then, compile the TypeScript file to JavaScript using `tsc filename.ts`, and run the resulting JavaScript file with `node filename.js`.
Can I run TypeScript files without compiling them first?
Yes, you can run TypeScript files without compiling them first by using `ts-node`. Install it globally with `npm install -g ts-node`, and then execute your TypeScript file directly using `ts-node filename.ts`.
What command do I use to compile a TypeScript file?
To compile a TypeScript file, use the command `tsc filename.ts`. This command generates a JavaScript file with the same name, which can then be executed using Node.js.
Is it necessary to have a `tsconfig.json` file to run TypeScript?
No, it is not strictly necessary to have a `tsconfig.json` file to run TypeScript files. However, having one allows you to configure compiler options and manage project settings more effectively.
How can I run TypeScript code in a browser?
To run TypeScript code in a browser, you need to compile the TypeScript file to JavaScript using `tsc filename.ts`, then include the resulting JavaScript file in your HTML. Alternatively, you can use tools like Webpack or Parcel to bundle your TypeScript code for browser usage.
What are the common errors when running TypeScript files?
Common errors when running TypeScript files include syntax errors, type mismatches, and missing type definitions. Ensure you have the correct types installed and check for any compilation errors in the terminal before executing the JavaScript output.
Running a TypeScript file involves several steps that ensure the code is properly compiled and executed. First, TypeScript needs to be installed on your system, which can be done using Node.js and npm. Once TypeScript is installed, you can write your TypeScript code in a `.ts` file. The next step is to compile the TypeScript file into JavaScript using the TypeScript compiler (`tsc`). This process converts the TypeScript code into a format that can be executed by the JavaScript runtime environment.
After compiling the TypeScript file, you can run the resulting JavaScript file using Node.js or in a browser environment. If you are using Node.js, you simply execute the command `node filename.js` in your terminal. If you are working in a browser, you can include the compiled JavaScript file in an HTML document, allowing it to be executed when the page loads.
In summary, running a TypeScript file requires installing TypeScript, writing the code, compiling it to JavaScript, and then executing the compiled file. This process highlights the importance of understanding both TypeScript and JavaScript, as well as the tools needed to facilitate development and execution.
Key takeaways from this discussion include
Author Profile
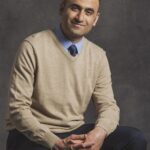
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?