How Can You Run a For Loop in Parallel in Python?
In the ever-evolving landscape of programming, efficiency is paramount, especially when it comes to executing tasks that can be time-consuming. Python, a beloved language for its simplicity and readability, offers a plethora of tools and libraries that can help developers optimize their code. One of the most powerful techniques for enhancing performance is parallel processing, which allows multiple operations to run simultaneously. If you’ve ever found yourself waiting impatiently for a for loop to complete its iterations, you might be wondering how to harness the power of parallelism in Python to speed things up.
Running a for loop in parallel can significantly reduce execution time, especially when dealing with large datasets or computationally intensive tasks. By distributing the workload across multiple processors or threads, you can take full advantage of your system’s capabilities. This article will explore various methods to implement parallel for loops in Python, including built-in libraries and third-party solutions. Whether you’re a seasoned developer or just starting your programming journey, understanding how to run loops in parallel can elevate your coding skills and enhance the performance of your applications.
As we delve deeper into the world of parallel processing in Python, we’ll examine the key concepts and tools that make it possible. From the multiprocessing module to concurrent.futures, you’ll learn about the different approaches available for running tasks concurrently. By
Using Threading for Parallel For Loops
Threading is one of the simplest ways to achieve parallelism in Python, especially for I/O-bound tasks. The `threading` module allows you to create multiple threads, each executing a portion of your loop concurrently.
python
import threading
def process_item(item):
# Function to process each item
print(f”Processing {item}”)
items = [1, 2, 3, 4, 5]
threads = []
for item in items:
thread = threading.Thread(target=process_item, args=(item,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
In this example, each item is processed in its own thread. Note that threading is best suited for I/O-bound tasks due to Python’s Global Interpreter Lock (GIL).
Using Multiprocessing for CPU-Bound Tasks
For CPU-bound tasks, the `multiprocessing` module is more appropriate as it bypasses the GIL by creating separate memory spaces for each process. This allows Python to utilize multiple CPU cores effectively.
python
from multiprocessing import Pool
def process_item(item):
# Function to process each item
return item * item
items = [1, 2, 3, 4, 5]
with Pool(processes=5) as pool:
results = pool.map(process_item, items)
print(results)
In this example, `Pool` manages a pool of worker processes, and `map` distributes the workload across them, returning results in a single list.
Leveraging Concurrent Futures
The `concurrent.futures` module provides a high-level interface for asynchronously executing callables. It supports both thread and process-based parallelism through `ThreadPoolExecutor` and `ProcessPoolExecutor`.
python
from concurrent.futures import ThreadPoolExecutor
def process_item(item):
return item * item
items = [1, 2, 3, 4, 5]
with ThreadPoolExecutor() as executor:
results = list(executor.map(process_item, items))
print(results)
This approach simplifies code readability and error handling while allowing for easy scalability.
Performance Comparison
The choice between threading, multiprocessing, and concurrent futures depends on the nature of the task. Here’s a comparison table:
Method | Best For | Overhead | Example Use Case |
---|---|---|---|
Threading | I/O-bound tasks | Low | Web scraping |
Multiprocessing | CPU-bound tasks | High | Data processing |
Concurrent Futures | Both I/O and CPU-bound | Medium | Batch processing |
Understanding the strengths and weaknesses of each method enables you to choose the right approach based on your specific requirements and performance considerations.
Using the `concurrent.futures` Module
The `concurrent.futures` module is a high-level interface for asynchronously executing callables. It provides a simple way to manage threads or processes in parallel.
- ThreadPoolExecutor: Suitable for I/O-bound tasks.
- ProcessPoolExecutor: Best for CPU-bound tasks.
Example using ThreadPoolExecutor:
python
from concurrent.futures import ThreadPoolExecutor
def task(n):
return n * n
with ThreadPoolExecutor(max_workers=4) as executor:
results = list(executor.map(task, range(10)))
print(results)
Example using ProcessPoolExecutor:
python
from concurrent.futures import ProcessPoolExecutor
def task(n):
return n * n
with ProcessPoolExecutor(max_workers=4) as executor:
results = list(executor.map(task, range(10)))
print(results)
Utilizing the `multiprocessing` Module
The `multiprocessing` module allows the creation of multiple processes, enabling parallel execution of functions. This is particularly useful for CPU-intensive tasks.
Using Pool:
python
from multiprocessing import Pool
def task(n):
return n * n
if __name__ == ‘__main__’:
with Pool(processes=4) as pool:
results = pool.map(task, range(10))
print(results)
Key Features:
- Supports shared memory.
- Allows inter-process communication.
Leveraging `joblib` for Parallel Processing
`joblib` is a library that provides tools for lightweight pipelining in Python, including parallel computing capabilities.
Example:
python
from joblib import Parallel, delayed
def task(n):
return n * n
results = Parallel(n_jobs=4)(delayed(task)(i) for i in range(10))
print(results)
Advantages:
- Easy syntax.
- Efficient handling of large data.
Using Dask for Parallel Computing
Dask is a flexible library for parallel computing in Python. It integrates seamlessly with NumPy and Pandas.
Example:
python
import dask.array as da
x = da.from_array(np.random.random((10000, 10000)), chunks=(1000, 1000))
y = (x + x.T).sum(axis=1)
result = y.compute()
print(result)
Key Benefits:
- Scales from single machines to clusters.
- Handles complex workflows.
Implementing Asynchronous Programming with `asyncio`
For I/O-bound tasks, `asyncio` can be a powerful alternative, allowing for asynchronous execution.
Example:
python
import asyncio
async def task(n):
await asyncio.sleep(1)
return n * n
async def main():
results = await asyncio.gather(*(task(i) for i in range(10)))
print(results)
asyncio.run(main())
Considerations:
- Best suited for I/O-bound tasks.
- Requires understanding of coroutines and event loops.
Performance Considerations
When choosing a method to run loops in parallel, consider the following factors:
Method | Best For | Overhead |
---|---|---|
`concurrent.futures` | General tasks | Low |
`multiprocessing` | CPU-bound | Higher than threads |
`joblib` | Data processing | Low |
`Dask` | Large datasets | Moderate |
`asyncio` | I/O-bound | Very low |
Each method has its own strengths and weaknesses, and the choice depends on the specific requirements of the task at hand.
Strategies for Parallelizing For Loops in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “To effectively run for loops in parallel in Python, utilizing the `concurrent.futures` module is highly recommended. This module provides a simple interface for asynchronously executing callables, making it ideal for CPU-bound tasks.”
Mark Thompson (Software Engineer, Parallel Computing Solutions). “For I/O-bound tasks, consider using the `asyncio` library alongside the `async` and `await` keywords. This approach allows for concurrent execution of tasks without blocking the main thread, significantly improving performance in scenarios involving network or file operations.”
Linda Garcia (Python Developer, Open Source Projects). “When working with data processing tasks, leveraging the `multiprocessing` module can be highly effective. It allows you to create separate processes, each with its own Python interpreter, thus bypassing the Global Interpreter Lock (GIL) and achieving true parallelism.”
Frequently Asked Questions (FAQs)
What is parallel processing in Python?
Parallel processing in Python refers to the concurrent execution of multiple processes or threads to perform tasks simultaneously, leveraging multiple CPU cores for improved performance and efficiency.
How can I run a for loop in parallel in Python?
You can run a for loop in parallel using libraries such as `multiprocessing`, `concurrent.futures`, or `joblib`. These libraries provide tools to distribute tasks across multiple processors or threads.
What is the difference between threading and multiprocessing in Python?
Threading involves running multiple threads within a single process, sharing memory space, which is suitable for I/O-bound tasks. Multiprocessing, on the other hand, creates separate processes with their own memory space, making it more suitable for CPU-bound tasks.
Can I use the `map` function for parallel execution in Python?
Yes, the `map` function from the `concurrent.futures.ThreadPoolExecutor` or `ProcessPoolExecutor` can be used to execute a function in parallel across multiple input values, effectively distributing the workload.
Are there any limitations to running loops in parallel?
Yes, limitations include overhead from inter-process communication, the Global Interpreter Lock (GIL) in CPython affecting threading performance, and potential issues with shared state or data integrity.
What is the best library for running for loops in parallel in Python?
The best library depends on the specific use case. For CPU-bound tasks, `multiprocessing` or `concurrent.futures.ProcessPoolExecutor` is recommended. For I/O-bound tasks, `concurrent.futures.ThreadPoolExecutor` is often more efficient.
Running a for loop in parallel in Python can significantly enhance performance, especially for computationally intensive tasks. Traditional for loops execute sequentially, which can be a bottleneck in applications that could benefit from concurrent execution. By leveraging libraries such as `concurrent.futures`, `multiprocessing`, or `joblib`, developers can efficiently distribute tasks across multiple processors or threads, allowing for simultaneous execution of loop iterations.
One of the most common approaches is using the `ThreadPoolExecutor` or `ProcessPoolExecutor` from the `concurrent.futures` module. These executors manage a pool of threads or processes, respectively, and allow you to submit tasks that can run in parallel. This method is particularly useful for I/O-bound tasks when using threads or CPU-bound tasks when using processes. Additionally, the `map` function simplifies the application of a function to a list of inputs, returning results in an efficient manner.
Another effective method for parallelizing for loops is the `multiprocessing` module, which allows for the creation of separate processes that can run on different CPU cores. This is advantageous for CPU-bound operations due to Python’s Global Interpreter Lock (GIL), which can hinder the performance of multithreaded programs. The
Author Profile
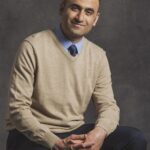
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?