How Can You Run JavaScript Code in Visual Studio Code?
### Introduction
In the ever-evolving landscape of web development, JavaScript stands out as a cornerstone technology that powers interactive and dynamic web applications. Whether you’re a seasoned developer or just starting your coding journey, mastering the art of running JavaScript code efficiently can significantly enhance your productivity. Visual Studio Code (VS Code), a powerful and versatile code editor, has become a favorite among developers for its rich features and seamless integration with various programming languages, including JavaScript. But how do you harness the full potential of this tool to execute your JavaScript code effortlessly?
Running JavaScript in Visual Studio Code is not just about writing code; it’s about creating an environment where your ideas can come to life. With its intuitive interface and robust extensions, VS Code allows you to set up a workspace tailored to your needs. From debugging to live server capabilities, this editor offers a plethora of features that streamline the coding process. In this article, we will explore the essential steps and tools you need to run your JavaScript code smoothly, ensuring that you can focus on what truly matters: building amazing applications.
As we delve deeper into the specifics of executing JavaScript in VS Code, you’ll discover the various methods available, from using the integrated terminal to leveraging extensions that enhance your coding experience. Whether you’re
Setting Up Visual Studio Code for JavaScript
To effectively run JavaScript code in Visual Studio Code, you first need to ensure that your environment is properly set up. Visual Studio Code (VS Code) is a versatile code editor that supports various programming languages, including JavaScript, through extensions and built-in features.
### Installing Node.js
Node.js is a JavaScript runtime that allows you to execute JavaScript code outside of a browser. To get started, download and install Node.js from the official website. This will also install npm (Node Package Manager), which is essential for managing JavaScript packages.
- Visit the [Node.js official site](https://nodejs.org/).
- Download the version suitable for your operating system (LTS version is recommended).
- Follow the installation instructions.
### Configuring VS Code
After installing Node.js, you need to configure Visual Studio Code to run JavaScript files.
- **Open VS Code.**
- **Create a new file** by clicking on `File` > `New File` or using the shortcut `Ctrl + N`.
- **Save the file** with a `.js` extension (e.g., `app.js`) by clicking on `File` > `Save As` or pressing `Ctrl + S`.
### Running JavaScript Code
You can run JavaScript code directly from the terminal within VS Code. Here’s how to do it:
- Open the terminal in VS Code by going to `View` > `Terminal` or pressing “ Ctrl + ` “.
- Navigate to the directory containing your JavaScript file using the `cd` command.
For example:
cd path/to/your/file
- Once in the correct directory, run the JavaScript file with the following command:
node app.js
The output of your JavaScript code will be displayed in the terminal.
### Using the Debugger
VS Code comes with a built-in debugger that allows you to run and debug your JavaScript code interactively.
- Set Breakpoints: Click on the left margin next to the line number where you want to add a breakpoint.
- Open the Debug View: Click on the debug icon on the sidebar or press `Ctrl + Shift + D`.
- Run the Debugger: Click the green play button or press `F5` to start debugging.
### Common Issues and Troubleshooting
If you encounter issues while running JavaScript code, consider the following common troubleshooting steps:
- Check Node.js Installation: Ensure Node.js is installed correctly by running `node -v` in the terminal. It should return the installed version.
- Verify File Path: Make sure you are in the correct directory where your JavaScript file is located.
- Check for Syntax Errors: Look for any syntax errors in your JavaScript code that may prevent it from running.
Issue | Solution |
---|---|
Node is not recognized | Ensure Node.js is installed and added to your system’s PATH. |
File not found | Check the file name and ensure you’re in the correct directory. |
Syntax errors | Review your code for missing brackets, commas, or incorrect syntax. |
With the above steps, you should be able to set up and run JavaScript code seamlessly within Visual Studio Code, taking advantage of its powerful features to enhance your coding experience.
Setting Up Visual Studio Code for JavaScript
To run JavaScript code in Visual Studio Code (VS Code), you need to ensure that your environment is configured correctly. Follow these steps:
- Install Visual Studio Code: Download and install the latest version from the official [VS Code website](https://code.visualstudio.com/).
- Install Node.js: JavaScript can be run in a browser, but for server-side and tooling capabilities, install Node.js from the [Node.js website](https://nodejs.org/). This installation also includes npm (Node Package Manager), which is useful for managing packages.
Creating a New JavaScript File
- Open Visual Studio Code.
- Create a new folder for your project or open an existing one.
- In the Explorer pane, right-click on the folder and select New File.
- Name your file with a `.js` extension, for example, `app.js`.
Writing JavaScript Code
You can now write your JavaScript code in the newly created file. For example:
javascript
console.log(“Hello, world!”);
Running JavaScript Code in Visual Studio Code
To execute your JavaScript code, you can use the integrated terminal in VS Code.
– **Open the Terminal**: Press “ Ctrl + ` “ (backtick) or navigate to **View** > Terminal.
- Run the Code:
- Ensure you are in the directory where your `.js` file is located. You can navigate using the `cd` command.
- Type the command below to execute your JavaScript file:
bash
node app.js
- View Output: The output will display in the terminal. For the above example, it should show `Hello, world!`.
Using Extensions for Enhanced Development
Consider installing extensions to improve your JavaScript development experience. Some recommended extensions include:
Extension Name | Description |
---|---|
ESLint | Linting tool for identifying and fixing problems in your JavaScript code. |
Prettier | Code formatter to ensure consistent code style. |
JavaScript (ES6) code snippets | Provides JavaScript code snippets for faster coding. |
To install an extension:
- Click on the Extensions icon in the Activity Bar on the side.
- Search for the desired extension.
- Click Install.
Debugging JavaScript Code in Visual Studio Code
VS Code provides powerful debugging capabilities.
- Set Breakpoints: Click in the gutter to the left of the line numbers to set breakpoints.
- Open Debugger: Go to the Run and Debug view by clicking the play icon in the Activity Bar.
- Start Debugging: Click on Run and Debug or press `F5`. Select Node.js if prompted.
As you debug, you can inspect variables, view call stacks, and control execution flow.
Using the Live Server Extension for Browser Testing
For web-based JavaScript, consider using the Live Server extension.
- Install the Live Server extension from the Extensions Marketplace.
- Right-click on your HTML file (if applicable) and select Open with Live Server.
- Your default web browser will open, displaying your HTML file. Any changes made to your JavaScript will automatically refresh in the browser.
This setup provides a seamless development experience, allowing you to run and test JavaScript code efficiently within Visual Studio Code.
Expert Insights on Running JavaScript Code in Visual Studio Code
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To effectively run JavaScript code in Visual Studio Code, it is essential to have Node.js installed on your system. This allows you to execute JavaScript files directly from the integrated terminal, providing a seamless development experience.”
Michael Torres (Lead Developer Advocate, CodeCraft). “Utilizing the built-in terminal in Visual Studio Code is a game-changer for running JavaScript code. By leveraging scripts defined in your package.json file, you can streamline your workflow and manage dependencies more efficiently.”
Sarah Patel (Full Stack Developer, FutureTech Labs). “For beginners, I recommend using the Live Server extension in Visual Studio Code. It allows you to run JavaScript code in a browser environment, providing instant feedback and making debugging much simpler.”
Frequently Asked Questions (FAQs)
How do I set up Visual Studio Code for JavaScript development?
To set up Visual Studio Code for JavaScript development, install Visual Studio Code, then ensure you have Node.js installed on your system. You can verify the installation by running `node -v` in the terminal. Optionally, install relevant extensions like ESLint and Prettier for enhanced coding experience.
How can I run a JavaScript file in Visual Studio Code?
To run a JavaScript file in Visual Studio Code, open the terminal within the editor, navigate to the directory containing your JavaScript file using the `cd` command, and execute the file by typing `node filename.js`, replacing “filename.js” with the name of your file.
Is it possible to run JavaScript code directly in the Visual Studio Code editor?
Yes, you can run JavaScript code directly in Visual Studio Code using the built-in terminal or by using extensions like Code Runner. After installing Code Runner, you can right-click on your JavaScript file and select “Run Code” to execute it.
What extensions should I install for better JavaScript support in Visual Studio Code?
For improved JavaScript support, consider installing extensions such as ESLint for linting, Prettier for code formatting, and Live Server for live reloading of web applications. These tools enhance code quality and streamline development.
Can I debug JavaScript code in Visual Studio Code?
Yes, Visual Studio Code has built-in debugging capabilities for JavaScript. You can set breakpoints, inspect variables, and step through code. To start debugging, open the Debug panel, configure your launch settings, and run the debugger.
How do I install Node.js if I haven’t done so yet?
To install Node.js, visit the official Node.js website, download the installer for your operating system, and follow the installation instructions. After installation, verify it by running `node -v` in the terminal to check the version.
Running JavaScript code in Visual Studio Code (VS Code) is a straightforward process that involves setting up the appropriate environment and utilizing the built-in features of the editor. To execute JavaScript code, users typically need to have Node.js installed on their system, as it provides the runtime necessary for executing JavaScript outside of a browser. Once Node.js is installed, users can create a new JavaScript file in VS Code, write their code, and run it directly from the integrated terminal.
Additionally, VS Code offers various extensions that can enhance the JavaScript development experience. Extensions such as ESLint for linting and Prettier for code formatting can help maintain code quality and consistency. Furthermore, the integrated debugging tools in VS Code allow developers to set breakpoints, inspect variables, and step through code, making it easier to troubleshoot and optimize their JavaScript applications.
In summary, running JavaScript in Visual Studio Code involves installing Node.js, creating a JavaScript file, and utilizing the terminal for execution. The editor’s extensibility and powerful debugging features significantly improve the development workflow, making it a preferred choice among developers. By leveraging these tools and practices, developers can efficiently write, test, and debug their JavaScript code in a professional
Author Profile
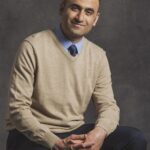
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?