How Can You Run JavaScript in the Terminal?
Introduction
In the ever-evolving landscape of web development, JavaScript stands out as a versatile and powerful programming language. While most people associate it with enhancing user experiences on websites, its capabilities extend far beyond the browser. Imagine harnessing the power of JavaScript directly from your terminal, enabling you to run scripts, automate tasks, and streamline your development workflow. Whether you’re a seasoned developer or a curious beginner, learning how to execute JavaScript in the terminal opens up a world of possibilities that can enhance your coding efficiency and broaden your skill set.
Running JavaScript in the terminal is not just a trend; it’s a practical skill that can significantly improve your productivity. By utilizing environments like Node.js, you can execute JavaScript code outside of a web browser, allowing for a more streamlined and efficient development process. This capability is particularly beneficial for tasks such as server-side programming, building command-line tools, or even testing snippets of code without the overhead of a full web application.
Moreover, the process of executing JavaScript in the terminal can empower developers to experiment and prototype ideas quickly. Whether you’re looking to automate repetitive tasks, manage files, or create complex applications, understanding how to run JavaScript in your terminal can be a game-changer. In the following sections, we will
Using Node.js to Execute JavaScript
One of the most popular ways to run JavaScript in the terminal is by using Node.js, a runtime environment that allows you to execute JavaScript code outside of a web browser. To get started with Node.js, follow these steps:
- Install Node.js: Download the installer from the official [Node.js website](https://nodejs.org/). Choose the version suitable for your operating system (Windows, macOS, or Linux).
- Verify Installation: After installation, open your terminal and type the following commands to check if Node.js and npm (Node Package Manager) are installed correctly:
bash
node -v
npm -v
- Create a JavaScript File: Use a text editor to create a new file with a `.js` extension, for example, `script.js`. Write your JavaScript code in this file.
- Run the JavaScript File: Execute the script using the following command in the terminal:
bash
node script.js
This process will run the JavaScript code contained in `script.js` and display the output in the terminal.
Running JavaScript in the REPL
Node.js also provides a Read-Eval-Print Loop (REPL) that allows you to execute JavaScript code interactively. To access the REPL, simply type `node` in your terminal and hit Enter. This will bring up a prompt where you can type JavaScript commands directly.
– **Basic Commands**: You can perform simple calculations or define functions. For example:
javascript
> 2 + 2
4
> function greet(name) { return `Hello, ${name}!`; }
> greet(‘Alice’)
‘Hello, Alice!’
- Exiting the REPL: To exit the REPL, press `Ctrl + C` twice or type `.exit`.
Using Other JavaScript Runtimes
Besides Node.js, there are other environments where you can run JavaScript in the terminal. Below is a comparison of several options:
Runtime | Installation Method | Usage |
---|---|---|
Node.js | Download from nodejs.org | node script.js |
Deno | Install via shell command | deno run script.ts |
Rhino | Download from mozilla.org | java -jar rhino.jar script.js |
Each of these runtimes has its strengths, and the choice will depend on your specific use case and preferences.
Executing JavaScript from the Command Line
You can also run JavaScript code directly from the command line without creating a separate file. Use the `-e` flag with Node.js to execute inline JavaScript, like this:
bash
node -e “console.log(‘Hello from the command line!’)”
This command will print “Hello from the command line!” directly in the terminal. This method is particularly useful for quick scripts or testing snippets of code without needing to create a file.
Using Node.js to Run JavaScript in the Terminal
Node.js is a powerful JavaScript runtime built on Chrome’s V8 engine, allowing you to execute JavaScript code outside of a web browser. Here’s how to run JavaScript using Node.js in your terminal:
- Install Node.js:
- Download the installer from the official [Node.js website](https://nodejs.org/).
- Follow the installation instructions specific to your operating system.
- Verify the Installation:
- Open your terminal and type:
bash
node -v
- This command will display the installed version of Node.js, confirming successful installation.
- Running JavaScript Files:
- Create a JavaScript file using a text editor. For example, create a file named `script.js`:
javascript
console.log(“Hello, World!”);
- In the terminal, navigate to the directory where your file is located and run the following command:
bash
node script.js
Running JavaScript Directly in the Terminal
You can also execute JavaScript code snippets directly in the terminal without creating a file. This can be particularly useful for testing small pieces of code.
- **Open the Node.js REPL (Read-Eval-Print Loop)**:
- Type `node` in the terminal and press Enter. You will see a prompt indicating you are in the REPL environment.
- You can now type JavaScript code directly. For example:
javascript
> console.log(“Hello from REPL!”);
- Exiting the REPL:
- To exit the REPL, press `Ctrl + C` twice or type `.exit`.
Using NPM Scripts to Run JavaScript
Node Package Manager (NPM) allows you to manage dependencies and scripts for your projects. You can define scripts in your `package.json` file to run JavaScript code conveniently.
- Creating a package.json File:
- In your project directory, initialize a new Node.js project:
bash
npm init -y
- Define a Script:
- Open the `package.json` file and locate the “scripts” section. Add a new script entry:
json
“scripts”: {
“start”: “node script.js”
}
- Running the Script:
- In the terminal, execute the script using:
bash
npm run start
Advanced Techniques: Running with Babel
For modern JavaScript features not supported natively by Node.js, you can use Babel to transpile your code.
- Install Babel:
- First, install Babel and its CLI globally:
bash
npm install –global @babel/core @babel/cli @babel/preset-env
- Create a Babel Configuration File:
- Create a file named `.babelrc` in your project directory with the following content:
json
{
“presets”: [“@babel/preset-env”]
}
- Run JavaScript with Babel:
- Use the following command to transpile and run your JavaScript file:
bash
babel script.js –out-file compiled.js && node compiled.js
This setup allows you to utilize the latest JavaScript features while ensuring compatibility with your environment.
Expert Insights on Running JavaScript in the Terminal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Running JavaScript in the terminal is a powerful way to execute scripts and automate tasks. Utilizing Node.js is essential, as it provides the runtime environment needed to interpret and execute JavaScript code outside of a browser.”
Michael Chen (Lead Developer, CodeCraft Solutions). “To effectively run JavaScript in the terminal, one must ensure that Node.js is installed. After installation, simply using the command ‘node filename.js’ allows for seamless execution of your JavaScript files, making it a straightforward process for developers.”
Sarah Jensen (JavaScript Instructor, Code Academy). “For beginners, running JavaScript in the terminal can seem daunting. However, with tools like Node.js, it becomes accessible. I recommend starting with simple scripts and gradually exploring more complex functionalities to build confidence.”
Frequently Asked Questions (FAQs)
How can I run JavaScript in the terminal?
You can run JavaScript in the terminal using Node.js, a JavaScript runtime. After installing Node.js, you can execute JavaScript files by typing `node filename.js` in the terminal.
Do I need to install anything to run JavaScript in the terminal?
Yes, you need to install Node.js. It includes the Node Package Manager (npm) and allows you to run JavaScript code outside of a web browser.
Can I run JavaScript directly in the terminal without a file?
Yes, you can run JavaScript directly in the terminal by entering the Node.js REPL (Read-Eval-Print Loop). Simply type `node` and press Enter, then you can type JavaScript code interactively.
What are some common commands to use with Node.js in the terminal?
Common commands include `node filename.js` to execute a file, `node` to enter the REPL, and `npm install package-name` to install packages.
Is there a way to run JavaScript in the terminal using other tools?
Yes, you can use tools like Deno, which is a secure runtime for JavaScript and TypeScript. You can run JavaScript files with `deno run filename.js` after installing Deno.
Can I run JavaScript code snippets without creating a file?
Yes, you can use the Node.js REPL to run code snippets directly. Just type your JavaScript code after entering `node` in the terminal, and it will execute immediately.
Running JavaScript in the terminal is a straightforward process that can be accomplished using various tools and environments. The most common method involves using Node.js, a JavaScript runtime built on Chrome’s V8 engine, which allows developers to execute JavaScript code outside of a web browser. By installing Node.js, users can access the terminal and run JavaScript files or commands directly, providing a powerful platform for server-side scripting and automation tasks.
Another approach to executing JavaScript in the terminal is through browser developer tools, which often include a console that can interpret JavaScript commands. However, this method is more limited in scope compared to using Node.js. Additionally, there are other environments and frameworks, such as Deno, that offer alternative ways to run JavaScript in the terminal, each with its own unique features and benefits.
In summary, understanding how to run JavaScript in the terminal expands a developer’s toolkit, enabling them to leverage JavaScript for a variety of applications beyond traditional web development. The use of Node.js is particularly advantageous due to its extensive ecosystem and community support, making it an essential skill for modern developers. By mastering these techniques, individuals can enhance their productivity and versatility in programming tasks.
Author Profile
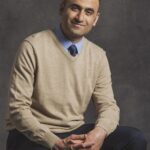
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?