How Can You Run a Laravel App in Docker Effectively?
In the ever-evolving landscape of web development, containerization has emerged as a game-changer, offering developers a streamlined way to build, test, and deploy applications. Among the myriad frameworks available, Laravel stands out for its elegant syntax and robust features, making it a favorite for PHP developers. However, managing dependencies and ensuring consistent environments can be a challenge. Enter Docker—a powerful tool that simplifies these complexities by encapsulating applications and their environments in portable containers. In this article, we will explore how to run a Laravel application in Docker, unlocking the potential for seamless development and deployment.
As we delve into the world of Docker and Laravel, it’s essential to understand the foundational concepts that make this combination so effective. Docker allows you to create isolated environments that mirror production settings, ensuring that your Laravel application runs smoothly regardless of where it is deployed. This not only enhances collaboration among team members but also reduces the “it works on my machine” syndrome that often plagues developers.
Throughout this article, we will guide you through the essential steps to set up a Laravel application within a Docker container. From creating a Dockerfile to configuring your environment, we will provide you with the tools and knowledge needed to harness the full power of Docker for your Laravel projects. Whether you’re
Setting Up Your Docker Environment
To run a Laravel application in Docker, you first need to ensure that your Docker environment is properly set up. This involves installing Docker Desktop on your machine if you haven’t done so already. Follow these steps to prepare your environment:
- Install Docker Desktop from the official Docker website.
- Start Docker Desktop and ensure it is running.
- Verify the installation by running `docker –version` in your terminal.
Creating a Dockerfile
A Dockerfile is essential for defining your application’s environment. Here’s a sample Dockerfile tailored for a Laravel application:
“`dockerfile
FROM php:8.1-fpm
Install system dependencies
RUN apt-get update && apt-get install -y \
libpng-dev \
libjpeg-dev \
libfreetype6-dev \
libzip-dev \
unzip \
git
Install PHP extensions
RUN docker-php-ext-configure gd –with-freetype –with-jpeg \
&& docker-php-ext-install gd zip
Set the working directory
WORKDIR /var/www
Copy the Laravel application files
COPY . .
Install Composer
COPY –from=composer:latest /usr/bin/composer /usr/bin/composer
Install Laravel dependencies
RUN composer install
Expose port
EXPOSE 9000
CMD [“php-fpm”]
“`
This Dockerfile does the following:
- Uses the official PHP image.
- Installs necessary system dependencies and PHP extensions.
- Sets the working directory and copies your Laravel application files.
- Installs Composer and Laravel dependencies.
Creating a docker-compose.yml File
A `docker-compose.yml` file simplifies managing multi-container Docker applications. Below is an example for a Laravel application:
“`yaml
version: ‘3.8’
services:
app:
build:
context: .
dockerfile: Dockerfile
image: laravel-app
container_name: laravel_app
ports:
- “9000:9000”
volumes:
- .:/var/www
depends_on:
- db
db:
image: mysql:5.7
container_name: mysql_db
ports:
- “3306:3306”
environment:
MYSQL_DATABASE: homestead
MYSQL_ROOT_PASSWORD: secret
volumes:
- db_data:/var/lib/mysql
volumes:
db_data:
“`
This configuration defines two services:
- app: The Laravel application container, built from the Dockerfile.
- db: A MySQL database container with environment variables for database configuration.
Building and Running the Containers
With your Dockerfile and `docker-compose.yml` set up, you can now build and run your Laravel application. Use the following commands:
“`bash
docker-compose build
docker-compose up
“`
This will build the images as defined in the Dockerfile and start the containers.
Accessing Your Laravel Application
Once the containers are running, your Laravel application should be accessible via your browser. Open your web browser and navigate to:
“`
http://localhost:9000
“`
Ensure that your application is properly configured to connect to the database. Update the `.env` file in your Laravel project as follows:
“`
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=homestead
DB_USERNAME=root
DB_PASSWORD=secret
“`
Common Commands for Managing Docker
Here is a summary of useful Docker commands when working with your Laravel application:
Command | Description |
---|---|
`docker-compose up` | Build and run the application. |
`docker-compose down` | Stop and remove the containers. |
`docker-compose exec app bash` | Access the application container’s shell. |
`docker-compose logs` | View logs for all services. |
By following these steps and utilizing the provided configurations, you can efficiently run a Laravel application within a Docker environment, enhancing your development workflow and deployment process.
Prerequisites
Before running a Laravel application in Docker, ensure that the following prerequisites are met:
- Docker: Install Docker and Docker Compose on your machine.
- Laravel Application: A working Laravel application that you wish to containerize.
- Basic Knowledge: Familiarity with Docker concepts, such as images, containers, and Docker Compose.
Setting Up Dockerfile
Create a `Dockerfile` in the root of your Laravel project. This file will define the environment for your application. Below is a sample `Dockerfile`:
“`dockerfile
Use the official PHP image with the necessary extensions
FROM php:8.1-fpm
Set working directory
WORKDIR /var/www
Install system dependencies
RUN apt-get update && apt-get install -y libpng-dev libjpeg-dev libfreetype6-dev \
&& docker-php-ext-configure gd –with-freetype –with-jpeg \
&& docker-php-ext-install gd pdo pdo_mysql
Copy existing application directory permissions
COPY –chown=www-data:www-data . .
Install Composer
COPY –from=composer:latest /usr/bin/composer /usr/bin/composer
Install PHP dependencies
RUN composer install
Expose port 9000
EXPOSE 9000
“`
Creating docker-compose.yml
Next, create a `docker-compose.yml` file in the same directory. This file will define the services required for your Laravel application:
“`yaml
version: ‘3.8’
services:
app:
build:
context: .
dockerfile: Dockerfile
image: laravel-app
container_name: laravel_app
ports:
- “9000:9000”
volumes:
- ./:/var/www
networks:
- laravel-network
db:
image: mysql:5.7
container_name: mysql_db
restart: always
environment:
MYSQL_ROOT_PASSWORD: rootpassword
MYSQL_DATABASE: laravel_db
MYSQL_USER: user
MYSQL_PASSWORD: password
ports:
- “3306:3306”
networks:
- laravel-network
networks:
laravel-network:
driver: bridge
“`
Building and Running the Application
To build and run your Laravel application with Docker, execute the following commands in your terminal:
- Build the Docker images:
“`bash
docker-compose build
“`
- Run the Docker containers:
“`bash
docker-compose up -d
“`
This will start your application in the background. You can check the status of your containers with:
“`bash
docker-compose ps
“`
Accessing the Application
After running the containers, your Laravel application should be accessible via your web browser at:
“`
http://localhost:9000
“`
Ensure that your Laravel application is correctly configured to connect to the MySQL database. Update the `.env` file in your Laravel project with the following database credentials:
“`env
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=laravel_db
DB_USERNAME=user
DB_PASSWORD=password
“`
Running Artisan Commands
To run Artisan commands within your Docker container, you can use the `exec` command. For example, to run migrations, execute:
“`bash
docker-compose exec app php artisan migrate
“`
This command allows you to interact with your Laravel application just as you would in a local development environment.
Expert Insights on Running Laravel Apps in Docker
Emily Tran (Senior DevOps Engineer, Tech Innovations Inc.). “To effectively run a Laravel application in Docker, it is essential to create a well-structured Dockerfile that includes all necessary dependencies. This ensures a consistent environment across different stages of development and production.”
Michael Chen (Lead Software Architect, Cloud Solutions Group). “Utilizing Docker Compose can significantly simplify the management of multi-container applications. For Laravel, defining services for the web server, database, and cache in a single docker-compose.yml file streamlines the deployment process.”
Sarah Patel (Full Stack Developer, CodeCraft Labs). “It is crucial to configure your environment variables correctly when running a Laravel app in Docker. This not only enhances security but also allows for greater flexibility in managing different environments, such as staging and production.”
Frequently Asked Questions (FAQs)
How do I set up a Docker environment for a Laravel application?
To set up a Docker environment for a Laravel application, create a `Dockerfile` and a `docker-compose.yml` file in your project directory. The `Dockerfile` should define the PHP environment, while the `docker-compose.yml` should configure services like the web server, database, and any other dependencies.
What services should I include in my docker-compose.yml for a Laravel app?
Typically, you should include services for PHP, a web server (like Nginx or Apache), and a database (such as MySQL or PostgreSQL). You may also want to add services for caching (like Redis) and queue management (like RabbitMQ).
How do I build and run my Laravel app using Docker?
To build and run your Laravel app using Docker, navigate to your project directory in the terminal and execute the command `docker-compose up –build`. This command will build the images and start the containers as defined in your `docker-compose.yml`.
How can I access my Laravel application in the browser?
Once your Docker containers are running, you can access your Laravel application by navigating to `http://localhost:your_port` in your web browser. Ensure that the port specified in your `docker-compose.yml` is correctly mapped to the host.
How do I run migrations and seed the database in a Dockerized Laravel app?
To run migrations and seed the database, use the command `docker-compose exec app php artisan migrate –seed`, where `app` is the name of your PHP service defined in `docker-compose.yml`. This command executes the Artisan commands within the running container.
What should I do if I encounter permission issues with storage and cache in Laravel Docker?
If you encounter permission issues, ensure that the storage and bootstrap/cache directories are writable by the web server user. You can fix this by running `docker-compose exec app chown -R www-data:www-data /path/to/laravel/storage` and `docker-compose exec app chown -R www-data:www-data /path/to/laravel/bootstrap/cache`.
Running a Laravel application in Docker involves several steps that streamline the development and deployment processes. The essential components include creating a Dockerfile to define the application environment, setting up a docker-compose.yml file to manage services such as the web server and database, and ensuring the necessary configurations are in place for seamless integration. By encapsulating the application and its dependencies within containers, developers can ensure consistency across different environments, which is crucial for both development and production settings.
One of the key takeaways from this discussion is the importance of understanding Docker’s architecture, including images, containers, and networks. This knowledge allows developers to leverage Docker’s capabilities effectively, such as isolating dependencies and scaling applications. Additionally, using Docker Compose simplifies the orchestration of multi-container applications, making it easier to manage services like Nginx, MySQL, and Redis alongside the Laravel app.
Moreover, utilizing Docker for Laravel applications enhances collaboration among team members by providing a uniform development environment. This reduces the “it works on my machine” problem, as all developers can run the same containerized setup. Furthermore, the ability to deploy the application in a containerized format leads to more predictable and manageable production releases, ultimately improving the overall development workflow.
Author Profile
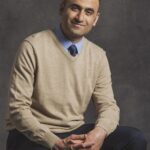
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?