How Do You Run a Python File in the Terminal on a Mac?
Are you ready to unleash the power of Python on your Mac? Whether you’re a seasoned developer or just starting your coding journey, knowing how to run a Python file in the terminal is an essential skill that can elevate your programming experience. The terminal, often seen as a daunting interface, is actually a powerful tool that allows you to execute scripts, manage files, and interact with your system in ways that graphical interfaces simply can’t match. In this article, we’ll guide you through the straightforward process of running Python files in the terminal, empowering you to harness the full potential of your coding projects.
Understanding how to run Python files in the terminal involves a few key concepts that every Mac user should grasp. First, you’ll need to familiarize yourself with the terminal environment, which acts as a command-line interface for your Mac. This knowledge will enable you to navigate your file system, locate your Python scripts, and execute them with ease. Additionally, we’ll touch on the importance of ensuring that Python is properly installed and configured on your machine, as this foundational step is crucial for successful execution.
As we dive deeper into the topic, you’ll discover the commands and techniques that will help you seamlessly run your Python scripts. From simple one-liners to more complex scripts, the terminal provides a versatile platform for executing your
Setting Up Your Terminal for Python
To run a Python file in the terminal on a Mac, you first need to ensure that Python is installed and accessible through the terminal. macOS typically comes with Python pre-installed, but it might not be the latest version. You can check your Python installation by executing the following command in the terminal:
bash
python3 –version
If Python is installed, this command will display the version number. If it is not installed, you can download the latest version from the official Python website or use a package manager like Homebrew.
Running a Python File
Once you have verified that Python is installed, you can run a Python file. Follow these steps:
- Open the Terminal application, which can be found in `Applications > Utilities > Terminal`.
- Navigate to the directory where your Python file is located using the `cd` command. For example:
bash
cd /path/to/your/file
- To run the Python file, use the following command:
bash
python3 yourfile.py
Replace `yourfile.py` with the name of your Python file. This will execute the script in the terminal.
Common Commands and Options
When running Python files, it is useful to know some common command-line options:
- `-m`: Runs a library module as a script.
- `-c`: Allows you to pass a command as a string to Python.
- `-h`: Displays help information.
Here’s an example table summarizing these options:
Option | Description |
---|---|
-m | Run a library module as a script |
-c | Execute Python commands passed as a string |
-h | Display help information |
Using Virtual Environments
If you are working on projects that require different dependencies, using a virtual environment is recommended. This allows you to manage packages on a per-project basis without affecting the global Python installation.
To create and activate a virtual environment, follow these steps:
- Install the `venv` module if it’s not already installed:
bash
python3 -m pip install –user virtualenv
- Create a virtual environment:
bash
python3 -m venv myenv
- Activate the virtual environment:
bash
source myenv/bin/activate
When activated, your terminal prompt will change to indicate that you are now working within the virtual environment. You can run your Python files within this environment to ensure that the correct packages are used.
Debugging and Error Handling
When running Python scripts, errors may occur, and understanding how to debug is crucial. Here are some common error types and their meanings:
- SyntaxError: Indicates a mistake in the syntax of your code.
- IndentationError: Occurs when the indentation is not consistent.
- NameError: Happens when a variable is referenced before it is assigned.
To troubleshoot these errors, carefully read the error message provided in the terminal, which typically includes the line number where the error occurred. You can also use debugging tools like `pdb` (Python Debugger) to step through your code interactively.
By following these guidelines, you can effectively run and manage your Python files in the terminal on a Mac.
Prerequisites
Before executing a Python file in the terminal on a Mac, ensure that you have the following:
- Python Installed: Verify that Python is installed on your system. You can check this by running the command:
bash
python3 –version
- Text Editor: Have a text editor ready for writing your Python script, such as Visual Studio Code, Sublime Text, or even the built-in TextEdit.
Creating a Python File
To create a Python file, follow these steps:
- Open your preferred text editor.
- Write your Python code. For example:
python
print(“Hello, World!”)
- Save the file with a `.py` extension. For instance, you could name it `hello.py`.
Navigating to Your File in Terminal
To run your Python file, you must navigate to the directory where the file is saved using the terminal:
- Open the Terminal application on your Mac.
- Use the `cd` command to change directories. For example:
bash
cd path/to/your/file
Replace `path/to/your/file` with the actual path to your Python file.
Running the Python File
Once you are in the correct directory, execute your Python file using the following command:
- For Python 3:
bash
python3 hello.py
- For Python 2 (if installed):
bash
python hello.py
Common Issues and Solutions
Issue | Solution |
---|---|
Command not found | Ensure Python is installed and added to the PATH. |
Permission denied | Check file permissions; use `chmod +x hello.py`. |
Syntax errors in code | Review the script for errors and correct them. |
Using Virtual Environments
If you are working within a virtual environment, activate it before running your Python script:
- Navigate to your project directory.
- Activate the virtual environment:
bash
source venv/bin/activate
Replace `venv` with your virtual environment’s name.
- Run the Python file as described above.
Using Python Scripts with Arguments
You can pass arguments to your Python scripts. For example, if your script accepts parameters, you could run it like this:
bash
python3 hello.py arg1 arg2
In your script, retrieve the arguments using the `sys` module:
python
import sys
print(sys.argv) # This will print the list of arguments passed
By following these steps, you can effectively run Python files in the terminal on your Mac, troubleshoot common issues, and utilize advanced features like virtual environments and command-line arguments.
Expert Guidance on Running Python Files in Terminal on Mac
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a Python file in the terminal on a Mac, you should first ensure that Python is installed. Open Terminal, navigate to the directory containing your Python file using the ‘cd’ command, and execute the script by typing ‘python3 filename.py’. This method is straightforward and allows for efficient script execution.”
James Liu (Lead Developer, CodeCraft Solutions). “Utilizing the terminal to run Python scripts is a fundamental skill for any developer. After confirming your Python installation, remember to use ‘python3’ instead of ‘python’ to avoid compatibility issues with Python 2. This distinction is crucial for ensuring that your scripts run correctly.”
Sarah Thompson (Technical Writer, Programming Today). “For Mac users, running Python files through the terminal not only enhances productivity but also provides a deeper understanding of the command line. Always check your script’s permissions and consider using ‘chmod +x filename.py’ to make your script executable, adding to your workflow efficiency.”
Frequently Asked Questions (FAQs)
How do I open the terminal on a Mac?
To open the terminal on a Mac, navigate to the Applications folder, then to Utilities, and select Terminal. Alternatively, you can use Spotlight by pressing Command + Space and typing “Terminal.”
What command do I use to run a Python file in the terminal?
To run a Python file in the terminal, use the command `python filename.py` or `python3 filename.py`, depending on your Python installation. Replace `filename.py` with the actual name of your Python file.
How can I check if Python is installed on my Mac?
To check if Python is installed, open the terminal and type `python –version` or `python3 –version`. This will display the installed version of Python if it is present.
What should I do if I get a “command not found” error?
If you receive a “command not found” error, ensure that Python is installed on your Mac. You may need to install it via Homebrew or download it from the official Python website.
Can I run a Python script with arguments in the terminal?
Yes, you can run a Python script with arguments by appending them after the filename in the command. For example, `python filename.py arg1 arg2` passes `arg1` and `arg2` to the script.
How do I set the default Python version on my Mac?
To set the default Python version, you can use a version management tool like `pyenv`. Install it via Homebrew and follow the instructions to set the desired Python version as the global default.
Running a Python file in the terminal on a Mac is a straightforward process that involves a few essential steps. First, it is crucial to ensure that Python is installed on your system. Most macOS versions come with Python pre-installed, but it is advisable to check the version and, if necessary, install the latest version from the official Python website or using a package manager like Homebrew.
Once Python is confirmed to be installed, you can navigate to the directory containing your Python file using the terminal. This is accomplished by using the `cd` command followed by the path to the directory. After reaching the correct directory, executing the Python file can be done by typing `python filename.py` or `python3 filename.py`, depending on the version of Python you wish to use. This command will run the script and display any output directly in the terminal.
In summary, running a Python file in the terminal on a Mac requires verifying the installation of Python, navigating to the appropriate directory, and executing the script using the correct command. Familiarity with these steps not only enhances your coding efficiency but also empowers you to troubleshoot and execute Python scripts effectively in a command-line environment.
Author Profile
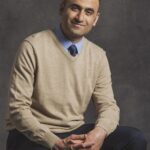
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?