How Do You Run Python Files in the Terminal?
Introduction
In the world of programming, Python stands out as one of the most versatile and user-friendly languages, making it a favorite among both beginners and seasoned developers. Whether you’re automating mundane tasks, developing web applications, or analyzing data, knowing how to efficiently run Python files in the terminal is an essential skill that can significantly enhance your workflow. This article will guide you through the ins and outs of executing Python scripts from the command line, empowering you to harness the full potential of this powerful language.
Running Python files in the terminal is not just about executing code; it’s about understanding the environment in which your code operates. The terminal serves as a direct interface to your operating system, allowing you to interact with Python in a way that can streamline your development process. By mastering this skill, you’ll gain greater control over your scripts, enabling you to debug, test, and deploy your applications with ease.
As we delve deeper into the topic, you’ll discover the various methods to run Python files, the importance of setting up your environment correctly, and some common pitfalls to avoid. Whether you’re looking to execute a simple script or manage complex projects, this guide will provide you with the foundational knowledge and practical tips you need to confidently navigate the terminal and make the most of your Python programming experience.
Setting Up Your Environment
To run Python files in the terminal, you must first ensure that Python is installed on your system. The installation process differs slightly based on your operating system. Below are the steps to check if Python is installed and how to install it if necessary.
- Windows: Open Command Prompt and type `python –version`. If Python is installed, you will see the version number. If not, download it from the [official Python website](https://www.python.org/downloads/).
- macOS: Open Terminal and type `python3 –version`. macOS typically comes with Python pre-installed. If not, you can install it via Homebrew by running `brew install python`.
- Linux: Open Terminal and type `python3 –version`. Most distributions come with Python pre-installed, but if not, use your package manager, such as `sudo apt install python3` for Debian-based systems.
Running Python Files
Once Python is installed, you can run Python files from the terminal. The process is straightforward and varies slightly depending on the version of Python you are using.
- Open your terminal or command prompt.
- Navigate to the directory where your Python file is located using the `cd` (change directory) command. For instance:
bash
cd path/to/your/directory
- Execute the Python file using the command:
- For Python 3:
bash
python3 filename.py
- For Python 2 (if still in use):
bash
python filename.py
Make sure to replace `filename.py` with the actual name of your Python file.
Using Virtual Environments
It is often advisable to use a virtual environment when working on Python projects to manage dependencies effectively. To create and activate a virtual environment, follow these steps:
- Create a virtual environment:
bash
python3 -m venv myenv
- Activate the virtual environment:
- Windows:
bash
myenv\Scripts\activate
- macOS/Linux:
bash
source myenv/bin/activate
Once activated, any Python file you run will use the packages installed in that virtual environment.
Common Errors and Troubleshooting
When running Python files, you may encounter various errors. Here are some common issues and their solutions:
Error Type | Description | Solution |
---|---|---|
`Command not found` | Python is not installed or not added to PATH. | Ensure Python is installed and added to PATH. |
`SyntaxError` | There is a mistake in your Python code. | Check the code for typos or syntax issues. |
`ModuleNotFoundError` | A required module is not installed. | Install the module using pip, e.g., `pip install module_name`. |
By following these guidelines, you should be able to run Python files efficiently in your terminal environment.
Setting Up Your Environment
Before running Python files in the terminal, ensure that Python is installed on your system. You can verify the installation and check the version by executing the following command:
bash
python –version
or for Python 3 specifically:
bash
python3 –version
If Python is not installed, you can download it from the [official Python website](https://www.python.org/downloads/).
Running Python Files
To run a Python file in the terminal, follow these steps:
- Open the Terminal: Access the command line interface on your operating system.
- Windows: Use Command Prompt or PowerShell.
- macOS/Linux: Open the Terminal application.
- Navigate to the Directory: Use the `cd` command to change to the directory where your Python file is located. For example:
bash
cd path/to/your/directory
- Execute the Python File: Use one of the following commands based on your Python version:
- For Python 2.x:
bash
python filename.py
- For Python 3.x:
bash
python3 filename.py
Using the Python Interpreter
You can also run Python files interactively using the Python interpreter. This can be done in two ways:
- Interactive Mode:
- Start the interpreter by typing `python` or `python3` in the terminal.
- Import and run your file within the interpreter:
python
import filename
- Using the `-i` option:
- This allows you to run a file and enter interactive mode afterward:
bash
python -i filename.py
Common Command-Line Options
When running Python files, several command-line options can enhance your experience:
Option | Description |
---|---|
`-h` | Displays help information about the script. |
`-m module` | Runs a library module as a script. |
`-v` | Enables verbose mode, providing detailed output. |
`–version` | Shows the version of Python being used. |
Handling Python Scripts with Arguments
If your script requires command-line arguments, you can pass them directly after the filename. For example:
bash
python filename.py arg1 arg2
In your Python script, you can access these arguments using the `sys` module:
python
import sys
# Access arguments
arg1 = sys.argv[1]
arg2 = sys.argv[2]
Checking for Errors and Debugging
While running Python files, you may encounter errors. To diagnose and debug issues, consider the following approaches:
- Read Tracebacks: Python provides detailed error messages. Carefully read the traceback to identify the issue.
- Use `print()` Statements: Insert print statements to track variable values and flow control.
- Use a Debugger: Python includes a built-in debugger called `pdb`. Start your script with:
bash
python -m pdb filename.py
This will allow you to step through the code line-by-line and inspect variables.
By following these steps and utilizing command-line options effectively, you can efficiently run and manage Python files within the terminal environment.
Expert Insights on Running Python Files in Terminal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively run Python files in the terminal, one must first ensure that Python is installed and properly configured in the system’s PATH. This allows users to execute scripts seamlessly by simply typing ‘python filename.py’ or ‘python3 filename.py’ depending on the version installed.”
Michael Chen (Lead Developer, CodeCraft Solutions). “Utilizing the terminal to run Python scripts enhances productivity, especially when leveraging command-line arguments for dynamic input. A solid understanding of terminal commands, such as navigating directories and using flags, is essential for efficient script execution.”
Sarah Thompson (Python Educator, LearnPython.org). “For beginners, mastering the terminal can be daunting, but it is a crucial skill. I recommend starting with basic commands and gradually incorporating more complex functionalities, such as virtual environments, to manage dependencies effectively while running Python files.”
Frequently Asked Questions (FAQs)
How do I run a Python file in the terminal?
To run a Python file in the terminal, navigate to the directory containing the file using the `cd` command. Then, execute the file by typing `python filename.py` or `python3 filename.py`, depending on your Python installation.
What should I do if I encounter a ‘command not found’ error?
If you see a ‘command not found’ error, ensure that Python is correctly installed and added to your system’s PATH. You can verify the installation by typing `python –version` or `python3 –version` in the terminal.
Can I run Python files with arguments from the terminal?
Yes, you can run Python files with arguments by appending them after the filename. For example, use `python filename.py arg1 arg2` to pass `arg1` and `arg2` to the script.
What is the difference between using ‘python’ and ‘python3’ in the terminal?
The difference lies in the version of Python being invoked. ‘python’ typically refers to Python 2.x, while ‘python3’ explicitly calls Python 3.x. It is advisable to use ‘python3’ for scripts compatible with Python 3.
How can I check if my Python script is executable?
You can check if your Python script is executable by running `ls -l filename.py`. If the script has executable permissions, it will show an ‘x’ in the permission string. If not, you can make it executable with `chmod +x filename.py`.
What should I do if my script requires specific libraries?
If your script requires specific libraries, ensure they are installed in your Python environment. You can install them using `pip install library_name` before running your script.
Running Python files in the terminal is a straightforward process that enhances the efficiency of executing scripts and managing projects. To initiate the execution of a Python file, users must first navigate to the directory containing the script using the ‘cd’ command. Once in the correct directory, the command ‘python filename.py’ or ‘python3 filename.py’ can be used, depending on the Python version installed on the system. This command prompts the terminal to interpret and execute the code within the specified file.
It is essential to ensure that Python is properly installed and configured in the system’s environment variables. Users can verify their installation by running ‘python –version’ or ‘python3 –version’ in the terminal. Additionally, understanding the distinction between Python 2 and Python 3 is crucial, as this affects how scripts are executed and which command is appropriate. Furthermore, utilizing virtual environments can help manage dependencies and versions effectively, promoting a cleaner and more organized workflow.
mastering the process of running Python files in the terminal is a vital skill for developers and programmers. It not only streamlines the workflow but also allows for greater control over the execution of scripts. By following best practices such as verifying installations and using virtual environments, users can enhance
Author Profile
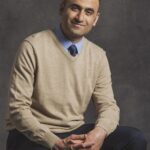
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?