How Can You Run a Python Script Within Python?
Running a Python script within another Python script might seem like a daunting task, especially for those new to programming. However, this capability opens up a world of possibilities for developers, enabling them to create modular, reusable code and streamline complex workflows. Whether you’re looking to enhance your projects, automate tasks, or simply experiment with different functionalities, understanding how to run Python scripts within Python is an essential skill that can elevate your coding prowess.
At its core, executing a Python script from within another script allows you to leverage existing code without duplicating efforts. This practice not only saves time but also promotes better organization and maintainability of your codebase. By utilizing various methods such as importing modules, executing scripts directly, or even using subprocesses, you can integrate functionalities seamlessly and enhance the overall efficiency of your applications.
As you delve into the intricacies of running Python scripts in Python, you’ll discover the versatility of this programming language and the myriad of ways it can be utilized to solve problems. From simple script execution to more advanced techniques like threading and multiprocessing, the journey promises to expand your understanding of Python and its capabilities. Prepare to unlock new dimensions of programming as you explore the various methods and best practices for running Python scripts effectively.
Using the Command Line
To run a Python script using the command line, you need to have Python installed on your system. You can check this by typing `python –version` or `python3 –version` in your terminal. Once confirmed, follow these steps:
- Open your command line interface (Terminal on macOS/Linux or Command Prompt/PowerShell on Windows).
- Navigate to the directory where your Python script is located using the `cd` command. For example:
cd path/to/your/script
- Execute the script by typing:
python script_name.py
or, if your system differentiates between Python versions:
python3 script_name.py
Running Python Scripts from Another Python Script
It is possible to run a Python script from another Python script using various methods. Here are some common approaches:
- Using the `import` statement: This method allows you to run functions or classes defined in another script as part of your current script.
- Using the `exec()` function: This built-in function can execute Python code dynamically, including entire scripts.
- Using the `subprocess` module: This allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes.
Here’s a brief overview of these methods:
Method | Description | Example |
---|---|---|
`import` | Allows access to functions/classes in another script | `import script_name` |
`exec()` | Executes code dynamically | `exec(open(‘script_name.py’).read())` |
`subprocess` | Runs a script as a separate process | `subprocess.run([‘python’, ‘script_name.py’])` |
Using Integrated Development Environments (IDEs)
Most IDEs provide a straightforward way to run Python scripts directly from the development environment. Here’s how to do it in some popular IDEs:
- PyCharm:
- Open your script in PyCharm.
- Right-click on the code editor and select “Run ‘script_name'”.
- Visual Studio Code:
- Open the script file.
- Click on the play button in the top right corner or use the shortcut `Ctrl + F5`.
- Jupyter Notebook:
- Write your script in a cell.
- Press `Shift + Enter` to execute it.
Using Online Python Interpreters
Online interpreters provide an easy way to run Python scripts without local installation. Some popular options include:
- Replit: A collaborative coding environment that supports multiple languages, including Python.
- Google Colab: Ideal for data science and machine learning projects, allowing you to run Python code in a Jupyter-like environment.
To run a script in these environments, simply paste your code into the provided text area and click the “Run” button.
Understanding how to run Python scripts in various environments enhances your productivity and versatility as a programmer. Whether through the command line, from within another script, using an IDE, or employing online interpreters, you have multiple options at your disposal to execute your Python code effectively.
Executing a Python Script from Another Python Script
To run a Python script within another Python script, you can utilize the `import` statement or the `subprocess` module, depending on your requirements.
Using the Import Statement
This method is suitable when you want to use functions or classes defined in another script. Here’s how to do it:
- Ensure the script you wish to run is in the same directory or in the Python path.
- Use the `import` statement to include the script.
Example:
Assuming you have a script named `script_a.py` containing a function `greet()`:
python
# script_a.py
def greet():
print(“Hello from script A!”)
You can call this function from another script as follows:
python
# script_b.py
import script_a
script_a.greet()
Using the Subprocess Module
If you need to run a script as a separate process (for example, when it requires independent execution), the `subprocess` module is the best choice. This allows you to run a script as if it were executed from the command line.
Example:
To run `script_a.py` from `script_b.py`, you can do the following:
python
# script_b.py
import subprocess
subprocess.run([‘python’, ‘script_a.py’])
### Key Points:
- Use `import` for reusing code.
- Use `subprocess.run()` for independent execution.
Passing Arguments to a Python Script
When invoking a script using the subprocess method, you might need to pass arguments to it. This is easily accomplished by extending the list in the `subprocess.run()` call.
Example:
If `script_a.py` accepts arguments:
python
# script_a.py
import sys
def main():
print(f”Arguments passed: {sys.argv[1:]}”)
if __name__ == “__main__”:
main()
You can call it from `script_b.py` like this:
python
# script_b.py
import subprocess
subprocess.run([‘python’, ‘script_a.py’, ‘arg1’, ‘arg2’])
### Argument Passing Summary:
- The first element of `sys.argv` is the script name.
- Subsequent elements are the arguments passed.
Running Scripts in Different Python Environments
Sometimes, you may need to run a script in a specific environment or version of Python. You can specify the Python interpreter in the subprocess call.
Example:
To run a script using Python 3.8:
python
subprocess.run([‘python3.8’, ‘script_a.py’])
### Environment Considerations:
- Ensure the specified Python version is installed.
- Verify that the script’s dependencies are satisfied in that environment.
Handling Output and Errors
You can capture the output and errors from a script executed via the subprocess module. This can be useful for debugging.
Example:
To capture the output:
python
result = subprocess.run([‘python’, ‘script_a.py’], capture_output=True, text=True)
print(“Output:”, result.stdout)
print(“Errors:”, result.stderr)
### Output Handling:
- `capture_output=True` captures standard output and error.
- Use `result.stdout` for standard output and `result.stderr` for errors.
Running Python Scripts
Employing the `import` statement is optimal for code reuse, while the `subprocess` module is preferable for executing scripts independently or in different environments. Ensure proper error handling and output capturing for effective script management.
Expert Insights on Running Python Scripts Effectively
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To run a Python script effectively, one must ensure that the correct version of Python is installed and that the script is executed in the appropriate environment. Utilizing virtual environments can help manage dependencies and avoid conflicts.”
Michael Chen (Lead Software Engineer, CodeCrafters). “Understanding how to execute Python scripts from the command line is crucial for developers. It involves using the command `python script_name.py` in the terminal, which allows for direct execution and debugging.”
Sarah Johnson (Python Educator, LearnPythonNow). “For beginners, using an Integrated Development Environment (IDE) like PyCharm or VSCode simplifies the process of running scripts. These tools provide built-in features that streamline execution and enhance productivity.”
Frequently Asked Questions (FAQs)
How can I run a Python script from the command line?
To run a Python script from the command line, navigate to the directory containing the script using the `cd` command and execute it with the command `python script_name.py`, replacing `script_name.py` with the actual name of your script.
Can I run a Python script directly in an IDE?
Yes, most Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Jupyter Notebook allow you to run Python scripts directly within the interface, typically through a “Run” button or by using a keyboard shortcut.
What is the difference between running a script with ‘python’ and ‘python3’?
The command `python` usually refers to Python 2.x, while `python3` specifically invokes Python 3.x. It is important to use the correct version based on the syntax and features utilized in your script.
How do I run a Python script in the background?
To run a Python script in the background, you can append an ampersand (`&`) at the end of your command in Unix-like systems, like so: `python script_name.py &`. This allows the script to execute without blocking the terminal.
Is it possible to run a Python script from another Python script?
Yes, you can run a Python script from another script using the `import` statement or the `subprocess` module. The `subprocess.run()` function allows you to execute another script as a separate process.
What permissions are required to run a Python script?
To run a Python script, you typically need execute permissions for the script file. On Unix-like systems, you can set this permission using the command `chmod +x script_name.py`.
Running a Python script within another Python script can be accomplished through various methods, each suited to different use cases. The most common approaches include using the `import` statement, the `exec()` function, and the `subprocess` module. The `import` statement allows you to utilize functions and classes defined in another script, promoting code reusability and modular programming. Conversely, the `exec()` function can execute Python code dynamically, which can be useful for running code stored in strings, but it should be used with caution due to potential security risks.
Another effective method is utilizing the `subprocess` module, which enables you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This is particularly beneficial when you want to run a script as a separate program, allowing for greater flexibility and isolation between scripts. Each of these methods has its own advantages and limitations, and the choice largely depends on the specific requirements of the task at hand.
In summary, understanding how to run a Python script within another Python script is crucial for efficient programming and workflow management. By leveraging the appropriate techniques, developers can enhance their code’s modularity, maintainability, and functionality. It is essential to choose the
Author Profile
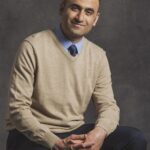
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?