How Can I Effectively Run a Python Script?
Running a Python script can seem daunting for newcomers, but it’s a fundamental skill that opens the door to endless possibilities in programming. Whether you’re diving into data analysis, automating mundane tasks, or developing web applications, understanding how to execute Python scripts is crucial. This article will guide you through the essential steps and considerations, ensuring that you can confidently run your scripts and harness the power of Python.
In the world of programming, executing a script is akin to bringing your code to life. Python, known for its simplicity and versatility, allows users to write scripts that can perform a wide range of functions. From the command line to integrated development environments (IDEs), there are various methods to run Python scripts, each with its own set of advantages. As you embark on this journey, you’ll discover not only the technical aspects of execution but also best practices that can enhance your coding experience.
Moreover, understanding how to run a Python script is just the beginning. As you delve deeper, you’ll uncover the nuances of script execution, including how to manage dependencies, handle errors, and optimize performance. By mastering these skills, you’ll be well-equipped to tackle more complex projects and make the most of Python’s capabilities. So, let’s dive in and demystify the process of
Setting Up Your Environment
To run a Python script, you must first ensure that your environment is correctly set up. This includes having Python installed on your machine along with any dependencies your script requires. The installation process varies depending on your operating system.
- Windows: Download the installer from the [official Python website](https://www.python.org/downloads/). Ensure you check the box that says “Add Python to PATH” during installation.
- macOS: Python 2.7 comes pre-installed on most macOS systems, but you can install the latest version using Homebrew with the command: `brew install python`.
- Linux: Most distributions come with Python pre-installed. You can install or update it using the package manager. For example, on Ubuntu, use: `sudo apt install python3`.
After installation, verify it by opening a terminal or command prompt and typing:
“`bash
python –version
“`
or
“`bash
python3 –version
“`
This should display the version of Python installed.
Executing a Python Script
Once your environment is set up, you can run your Python script. There are multiple methods to execute a script depending on your preference for command line or graphical user interfaces.
Using the Command Line:
- Open your terminal (macOS/Linux) or Command Prompt (Windows).
- Navigate to the directory containing your script using the `cd` command. For example:
“`bash
cd path/to/your/script
“`
- Run the script using either:
“`bash
python script_name.py
“`
or
“`bash
python3 script_name.py
“`
Using an Integrated Development Environment (IDE):
You can also run Python scripts from popular IDEs like PyCharm, Visual Studio Code, or Jupyter Notebook. Each IDE has its own method for running scripts, typically involving a “Run” button or a keyboard shortcut.
Common Command-Line Options
When executing a Python script, you can use various command-line options to modify its behavior. Below is a table summarizing some common options:
Option | Description |
---|---|
-h, –help | Displays help information for the script. |
-m module | Runs a library module as a script. |
–version | Displays the version of the script. |
–verbose | Enables verbose output to see more details during execution. |
These options can be combined with your command when running the script. For instance, to check the version of a script named `example.py`, you would enter:
“`bash
python example.py –version
“`
Handling Dependencies
If your script relies on external libraries, you need to manage these dependencies efficiently. The most common method for handling dependencies in Python is by using `pip`, the package installer for Python.
- Installing Packages: To install a package, use the following command:
“`bash
pip install package_name
“`
- Requirements File: For projects with multiple dependencies, it’s a best practice to create a `requirements.txt` file that lists all necessary packages. You can install all dependencies from this file by running:
“`bash
pip install -r requirements.txt
“`
By following these steps, you can effectively run Python scripts and manage any dependencies they may require.
Running Python Scripts in Different Environments
Running a Python script can vary depending on the environment you are using. Below are detailed instructions for various platforms and methods.
Running Python Scripts from the Command Line
To execute a Python script from the command line, follow these steps:
- Open your Command Line Interface (CLI):
- Windows: Press `Win + R`, type `cmd`, and hit Enter.
- macOS/Linux: Open the Terminal application.
- Navigate to the Script Directory:
Use the `cd` command to change directories to where your script is located. For example:
“`bash
cd path/to/your/script
“`
- Run the Script:
Execute the script by typing the following command:
“`bash
python script_name.py
“`
Replace `script_name.py` with the actual name of your script. If you are using Python 3, you may need to specify `python3` instead of `python`.
Running Python Scripts in Integrated Development Environments (IDEs)
Most IDEs provide a straightforward way to run Python scripts. Here are instructions for popular IDEs:
IDE | Steps to Run a Script |
---|---|
PyCharm | – Open the script. – Click the green ‘Run’ button or right-click and select ‘Run’. |
Visual Studio Code | – Open the script. – Use the shortcut `Ctrl + F5` or click on the play icon in the top right. |
Jupyter Notebook | – Open the notebook. – Select the cell and press `Shift + Enter`. |
Running Python Scripts with Virtual Environments
Using virtual environments is a best practice for managing dependencies. Here’s how to run a script in a virtual environment:
- Create a Virtual Environment (if not already created):
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- Windows:
“`bash
myenv\Scripts\activate
“`
- macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Required Packages (if necessary):
“`bash
pip install -r requirements.txt
“`
- Run the Script:
“`bash
python script_name.py
“`
Running Python Scripts via an Integrated Terminal
Many IDEs include an integrated terminal which allows you to run scripts directly. For instance:
– **VSCode**:
- Open the terminal through `View > Terminal`.
- Navigate to the script’s directory using `cd`.
- Run the script as described earlier.
- PyCharm:
- Open the terminal from the bottom panel.
- Execute the script using the same commands as in the command line.
Running Python Scripts in the Background
If you wish to run a Python script in the background, especially on Unix-like systems, use the following command:
“`bash
nohup python script_name.py &
“`
This allows the script to continue running after you log out. Additionally, you can check the output in `nohup.out`.
Using Python Scripts in Web Applications
For web applications, Python scripts are generally executed on the server-side. Frameworks like Flask or Django allow you to run scripts in response to web requests. Here’s a brief overview of how to set up a simple Flask application:
- Install Flask:
“`bash
pip install Flask
“`
- Create a Simple Application:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def hello_world():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run()
“`
- Run the Application:
“`bash
python app.py
“`
This will start a local server where you can access your script via a web browser.
Running Python Scripts on Remote Servers
To run a Python script on a remote server, SSH into the server and follow the command line instructions provided above. Ensure the necessary environment and dependencies are set up correctly on the server before execution.
Use the following command to connect to the server:
“`bash
ssh username@hostname
“`
Replace `username` and `hostname` with your actual username and server address.
Expert Insights on Running Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a Python script, ensure you have Python installed on your system. You can execute the script by navigating to its directory in the command line and typing ‘python script_name.py’, where ‘script_name.py’ is the name of your Python file.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Using an Integrated Development Environment (IDE) like PyCharm or VSCode can simplify the process of running Python scripts. These tools provide built-in terminals and run configurations that streamline execution.”
Sarah Thompson (Data Scientist, Analytics Hub). “For users working with Jupyter Notebooks, running Python scripts is as simple as executing the cells containing your code. This interactive environment allows for immediate feedback and is especially useful for data analysis tasks.”
Frequently Asked Questions (FAQs)
How do I run a Python script from the command line?
To run a Python script from the command line, open your terminal or command prompt, navigate to the directory containing the script, and execute the command `python script_name.py`, replacing `script_name.py` with the actual name of your script.
What is the difference between running a Python script with ‘python’ and ‘python3’?
The command `python` typically refers to Python 2.x, while `python3` explicitly invokes Python 3.x. It is advisable to use `python3` for scripts that are compatible with Python 3 to avoid compatibility issues.
Can I run a Python script by double-clicking it?
Yes, you can run a Python script by double-clicking it if the file association for `.py` files is set to the Python interpreter. However, this may not always display the output in a terminal window.
How do I run a Python script in an integrated development environment (IDE)?
To run a Python script in an IDE, open the IDE, load your script file, and look for a “Run” button or menu option. Most IDEs also allow you to use keyboard shortcuts, such as F5 or Ctrl+R, to execute the script.
What should I do if I encounter a “Permission denied” error when running a Python script?
A “Permission denied” error typically indicates that you do not have the necessary permissions to execute the script. You can resolve this by changing the file permissions using the command `chmod +x script_name.py` on Unix-like systems or by running the command prompt as an administrator on Windows.
Is it possible to run a Python script in the background?
Yes, you can run a Python script in the background by appending an ampersand (`&`) at the end of the command in Unix-like systems, or by using tools like `nohup` or `screen`. On Windows, you can use the `start` command to run the script in a new command window.
Running a Python script is a straightforward process that can be accomplished through various methods, depending on the environment you are working in. The most common way to execute a Python script is through the command line or terminal. By navigating to the directory where the script is located and using the command `python script_name.py`, users can initiate the execution of their Python code. Additionally, integrated development environments (IDEs) like PyCharm, Visual Studio Code, or Jupyter Notebook provide user-friendly interfaces that allow for easy script execution with just a click or a keyboard shortcut.
It is also essential to ensure that the Python interpreter is correctly installed and configured on your system. This includes verifying that the correct version of Python is being used, as different scripts may require specific versions. Furthermore, setting up a virtual environment can help manage dependencies and avoid conflicts between different projects. This practice not only streamlines the execution process but also enhances the overall development experience by isolating project-specific libraries.
understanding how to run a Python script effectively is crucial for any developer or data scientist. Whether through the command line or an IDE, the ability to execute scripts efficiently enables users to test, debug, and deploy their code seamlessly. By following best practices,
Author Profile
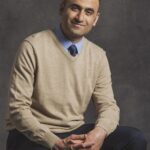
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?