How Can You Run a TypeScript File Effectively?
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful tool that enhances JavaScript with static typing and advanced features. As developers increasingly turn to TypeScript for building robust applications, understanding how to effectively run TypeScript files becomes essential. Whether you’re a seasoned programmer or just starting your coding journey, mastering the execution of TypeScript files will empower you to leverage its full potential and streamline your development process.
To run a TypeScript file, you first need to understand the compilation process that transforms TypeScript code into JavaScript, which is the language that browsers and Node.js can execute. This involves using the TypeScript compiler, commonly referred to as `tsc`, which takes your `.ts` files and compiles them into `.js` files. This step is crucial, as it ensures that your TypeScript code is converted into a format that can be understood and run in various environments.
Additionally, there are various tools and environments that can simplify the process of running TypeScript files. From integrated development environments (IDEs) that offer built-in support for TypeScript to command-line interfaces that allow for quick compilation and execution, the options are plentiful. Understanding these tools and their functionalities will not only enhance your productivity but also provide you with a
Setting Up TypeScript
To run a TypeScript file, you first need to ensure that your environment is properly set up. This involves installing Node.js and TypeScript itself. Node.js is a JavaScript runtime that allows you to run scripts server-side, while TypeScript is a superset of JavaScript that adds static types. Here are the steps to set up your environment:
- Install Node.js from the official website.
- Once Node.js is installed, you can install TypeScript globally using npm (Node Package Manager) by running the following command in your terminal:
“`
npm install -g typescript
“`
You can verify the installation of TypeScript by checking the version:
“`
tsc -v
“`
Creating a TypeScript File
After setting up TypeScript, the next step is to create a TypeScript file. TypeScript files have a `.ts` extension. You can create a simple TypeScript file with the following steps:
- Open your preferred text editor or Integrated Development Environment (IDE).
- Create a new file and save it with a `.ts` extension, for example, `example.ts`.
- Write your TypeScript code. Here is a simple example:
“`typescript
let greeting: string = “Hello, TypeScript!”;
console.log(greeting);
“`
Compiling TypeScript to JavaScript
TypeScript needs to be compiled into JavaScript before it can be executed. This is done using the TypeScript compiler (tsc). To compile your TypeScript file, use the following command in your terminal:
“`
tsc example.ts
“`
This command generates a JavaScript file named `example.js`. You can check the contents of this file to see the compiled code.
Running the Compiled JavaScript File
Once you have compiled your TypeScript file, you can run the resulting JavaScript file using Node.js. Execute the following command in your terminal:
“`
node example.js
“`
This will run the JavaScript code in the `example.js` file, and you should see the output in your terminal.
Table of Commands
Here is a summary of the commands used in the process of running a TypeScript file:
Command | Description |
---|---|
npm install -g typescript | Installs TypeScript globally. |
tsc example.ts | Compiles the TypeScript file to JavaScript. |
node example.js | Runs the compiled JavaScript file. |
Using tsconfig.json for Configuration
For larger projects, it is often beneficial to create a `tsconfig.json` file. This file allows you to specify compiler options and file inclusions/exclusions. To create it, run the following command in your project directory:
“`
tsc –init
“`
This generates a basic `tsconfig.json` file, which you can customize according to your project’s needs. For example, you can set the target version of JavaScript or include specific directories for your TypeScript files.
By following these steps, you can efficiently run TypeScript files in your development environment.
Setting Up TypeScript
To run a TypeScript file, you first need to ensure that TypeScript is installed on your machine. Follow these steps to set up your environment:
- Install Node.js: TypeScript runs on Node.js, so you need to install it first. Download it from the official Node.js website and follow the installation instructions.
- Install TypeScript: Open your terminal or command prompt and execute the following command:
“`bash
npm install -g typescript
“`
- Verify Installation: You can verify that TypeScript is installed correctly by checking its version:
“`bash
tsc -v
“`
Creating a TypeScript File
Once TypeScript is installed, you can create your TypeScript files. Here’s how:
- Create a new file: Use any text editor or IDE (like Visual Studio Code) to create a new file. Save it with a `.ts` extension, for example, `app.ts`.
- Write TypeScript code: Add some TypeScript code to your file. For instance:
“`typescript
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet(‘World’));
“`
Compiling TypeScript to JavaScript
Before you can run a TypeScript file, it must be compiled to JavaScript. Use the TypeScript compiler (`tsc`) to do this:
- Compile the file: In your terminal, navigate to the directory where your TypeScript file is located, and run:
“`bash
tsc app.ts
“`
- Output: This command generates a JavaScript file named `app.js` in the same directory.
Command | Description |
---|---|
`tsc app.ts` | Compiles `app.ts` to `app.js` |
Running the Compiled JavaScript File
After compiling your TypeScript file, you can run the generated JavaScript file using Node.js:
- Run the JavaScript file: Execute the following command:
“`bash
node app.js
“`
- Expected Output: You should see the output in the terminal:
“`
Hello, World!
“`
Using ts-node for Direct Execution
For a more streamlined process, you can use `ts-node`, which allows you to run TypeScript files directly without manual compilation.
- Install ts-node: Run the following command in your terminal:
“`bash
npm install -g ts-node
“`
- Run your TypeScript file directly: Instead of compiling first, you can execute:
“`bash
ts-node app.ts
“`
This will execute your TypeScript file, and you should see the same output as before.
Common Errors and Troubleshooting
When running TypeScript files, you may encounter some common errors. Here are a few troubleshooting tips:
- Type Errors: Ensure that your TypeScript code adheres to the type definitions. Check for type mismatches.
- File Not Found: Confirm the correct file path and name if you receive an error stating that the file cannot be found.
- ts-node Issues: If `ts-node` is not recognized, verify that it is installed globally and that your environment variables are set correctly.
By following these steps and best practices, you can efficiently run and manage TypeScript files in your development workflow.
Expert Insights on Running TypeScript Files
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a TypeScript file, one must first ensure that TypeScript is installed globally using npm. After installation, the command ‘tsc yourfile.ts’ compiles the TypeScript file into JavaScript, which can then be executed using Node.js with ‘node yourfile.js’.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “Utilizing a build tool like Webpack can streamline the process of running TypeScript files. By configuring Webpack with a TypeScript loader, developers can automatically compile and run TypeScript files, enhancing workflow efficiency.”
Linda Zhang (Technical Author, Dev Insights). “For those new to TypeScript, using an integrated development environment (IDE) such as Visual Studio Code simplifies the execution process. The IDE provides built-in support for running TypeScript files directly, making it user-friendly for beginners.”
Frequently Asked Questions (FAQs)
How do I run a TypeScript file directly?
To run a TypeScript file directly, you need to compile it to JavaScript first using the TypeScript compiler (`tsc`). Use the command `tsc filename.ts` to generate a JavaScript file, which can then be executed using Node.js with `node filename.js`.
What is the command to compile a TypeScript file?
The command to compile a TypeScript file is `tsc filename.ts`. This command converts the TypeScript code into JavaScript, which can be run in any JavaScript environment.
Can I run TypeScript files without compiling them first?
Yes, you can run TypeScript files without manually compiling them by using a tool like `ts-node`. This allows you to execute TypeScript files directly with the command `ts-node filename.ts`.
What do I need to install to run TypeScript files?
To run TypeScript files, you need to install Node.js and the TypeScript compiler. You can install TypeScript globally using the command `npm install -g typescript`.
Is it necessary to have a TypeScript configuration file?
While it is not strictly necessary, having a TypeScript configuration file (`tsconfig.json`) is highly recommended. It allows you to specify compiler options and project settings, making it easier to manage larger projects.
How can I execute TypeScript files in a development environment?
In a development environment, you can use tools like Visual Studio Code, which has built-in support for TypeScript. You can run TypeScript files using the integrated terminal with `tsc` or `ts-node`, depending on your setup.
In summary, running a TypeScript file involves several steps that ensure the code is properly compiled and executed. First, it is essential to have Node.js and TypeScript installed on your machine. TypeScript files, with the extension .ts, need to be compiled into JavaScript using the TypeScript compiler (tsc). This process converts TypeScript’s static typing and advanced features into standard JavaScript that can be executed in a runtime environment.
After installing TypeScript, you can compile a TypeScript file by using the command line. The command `tsc filename.ts` will generate a corresponding JavaScript file, which can then be run using Node.js with the command `node filename.js`. This workflow highlights the importance of understanding both TypeScript and JavaScript, as well as the tools required to bridge the two languages effectively.
Additionally, utilizing TypeScript’s configuration file, tsconfig.json, can streamline the compilation process by allowing you to set various compiler options and specify which files to include or exclude. This is particularly useful for larger projects where managing multiple files becomes necessary. By following these steps, developers can efficiently run TypeScript files and leverage the benefits of TypeScript in their projects.
Author Profile
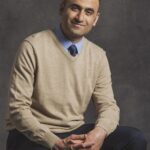
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?