How Can You Easily Run TypeScript Files? A Step-by-Step Guide
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful ally for developers seeking to enhance their JavaScript experience. With its static typing and advanced features, TypeScript not only improves code quality but also boosts productivity. However, for many newcomers and even seasoned developers, the question remains: how do you run TypeScript files effectively? Whether you’re building a small project or a large application, understanding the intricacies of executing TypeScript can pave the way for smoother development and fewer headaches.
To run TypeScript files, you first need to grasp the relationship between TypeScript and JavaScript. TypeScript is a superset of JavaScript, which means that it must be transpiled into JavaScript before it can be executed in a browser or a Node.js environment. This process involves converting your TypeScript code into plain JavaScript, allowing it to be understood and run by JavaScript engines. While this may sound daunting, the tools and workflows available today make it easier than ever to harness the power of TypeScript in your projects.
Additionally, various methods exist to run TypeScript files, each catering to different development needs and preferences. From using command-line tools to integrating TypeScript into popular build systems, the options are plentiful. As you delve deeper into
Setting Up TypeScript Environment
To run TypeScript files, you first need to set up your development environment. This involves installing Node.js, the TypeScript compiler, and optionally a code editor that supports TypeScript.
- Install Node.js: Visit the [Node.js website](https://nodejs.org) and download the installer for your operating system. This will also install npm (Node Package Manager) which is crucial for managing packages.
- Install TypeScript: Once Node.js is installed, you can install TypeScript globally using npm. Open your command line interface (CLI) and run the following command:
“`
npm install -g typescript
“`
- Choose a Code Editor: Use a code editor that provides TypeScript support, such as Visual Studio Code, which offers built-in TypeScript integration.
Compiling TypeScript Files
TypeScript files have the `.ts` extension and need to be compiled into JavaScript before they can be executed. This can be done using the TypeScript compiler (`tsc`).
- To compile a single TypeScript file, run:
“`
tsc yourfile.ts
“`
- To compile all `.ts` files in a directory, simply run:
“`
tsc
“`
You can also create a `tsconfig.json` file in your project directory to specify the compiler options and include/exclude files. Here’s a basic example of a `tsconfig.json`:
“`json
{
“compilerOptions”: {
“target”: “es6”,
“module”: “commonjs”,
“outDir”: “./dist”
},
“include”: [“src/**/*”],
“exclude”: [“node_modules”, “**/*.spec.ts”]
}
“`
Running Compiled JavaScript Files
After compiling, you can run the generated JavaScript files using Node.js. If your compiled file is named `yourfile.js`, you can execute it with the following command:
“`
node yourfile.js
“`
Using ts-node for Direct Execution
For a more streamlined approach, you can use `ts-node`, which allows you to run TypeScript files directly without manually compiling them first.
- Install ts-node: Run the following command to install `ts-node`:
“`
npm install -g ts-node
“`
- Run TypeScript File: You can now execute your TypeScript file directly by running:
“`
ts-node yourfile.ts
“`
This method is particularly useful for development and testing, as it simplifies the workflow.
Comparison of Methods
The following table summarizes the different methods of running TypeScript files:
Method | Description | Use Case |
---|---|---|
tsc + node | Compile TypeScript to JavaScript, then run with Node.js. | Production deployment. |
ts-node | Run TypeScript files directly without compilation. | Development and testing. |
By understanding these methods, you can choose the one that best fits your workflow and project needs.
Prerequisites for Running TypeScript Files
Before executing TypeScript files, ensure that you have the following tools installed:
- Node.js: TypeScript requires Node.js to run. Download it from [nodejs.org](https://nodejs.org/).
- TypeScript Compiler: Install TypeScript globally using npm:
“`bash
npm install -g typescript
“`
Compiling TypeScript Files
TypeScript files must be compiled into JavaScript before they can be executed. Use the TypeScript compiler (`tsc`) for this purpose. The basic command to compile a TypeScript file is:
“`bash
tsc filename.ts
“`
This command generates a JavaScript file named `filename.js` in the same directory.
Running Compiled JavaScript Files
Once you have compiled your TypeScript file, you can run the resulting JavaScript file using Node.js. The command is as follows:
“`bash
node filename.js
“`
Running TypeScript Directly with ts-node
For a more streamlined workflow, you can use `ts-node`, which allows you to run TypeScript files directly without manual compilation. To use `ts-node`, follow these steps:
- Install `ts-node` globally:
“`bash
npm install -g ts-node
“`
- Run your TypeScript file directly:
“`bash
ts-node filename.ts
“`
Using a TypeScript Configuration File
To manage compilation options efficiently, create a `tsconfig.json` file in your project directory. This file allows you to specify compiler options and files to be included. A basic example of a `tsconfig.json` is:
“`json
{
“compilerOptions”: {
“target”: “es6”,
“module”: “commonjs”
},
“include”: [
“src/**/*”
]
}
“`
To compile the project based on this configuration, run:
“`bash
tsc
“`
Using an Integrated Development Environment (IDE)
Many IDEs support TypeScript and can run TypeScript files directly. Popular choices include:
- Visual Studio Code: Offers built-in TypeScript support and terminal access.
- WebStorm: Provides robust TypeScript integration.
In these environments, you can often run TypeScript files directly from the IDE without needing to switch to the terminal.
Common Errors and Troubleshooting
While running TypeScript files, you may encounter some common errors. Here are a few solutions:
Error Message | Possible Cause | Solution |
---|---|---|
Cannot find module ‘ts-node’ | `ts-node` is not installed | Run `npm install -g ts-node` |
TypeScript file not compiled | No `.js` file generated | Ensure `tsc` command is executed |
Unexpected token | Syntax error in TypeScript file | Check the TypeScript syntax |
Ensure to review error messages carefully to identify and resolve issues promptly.
Expert Guidance on Running TypeScript Files
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run TypeScript files, it is crucial to first compile them into JavaScript using the TypeScript compiler (tsc). This can be done by executing the command ‘tsc filename.ts’ in your terminal, which generates a corresponding JavaScript file that can be executed in any JavaScript environment.”
Michael Chen (Lead Developer, CodeCraft Solutions). “For a seamless development experience, I recommend using tools like ts-node, which allows you to run TypeScript files directly without the need for manual compilation. Simply install it via npm and run your TypeScript files with ‘ts-node filename.ts’ for instant execution.”
Sarah Patel (Technical Author and Educator, DevLearn Academy). “Understanding the environment in which you are running TypeScript files is essential. If you are developing for Node.js, ensure you have the appropriate TypeScript configuration in your tsconfig.json file, as this will dictate how your TypeScript code is compiled and executed in your project.”
Frequently Asked Questions (FAQs)
How do I compile a TypeScript file?
To compile a TypeScript file, use the TypeScript compiler (tsc). Run the command `tsc filename.ts` in your terminal, which generates a JavaScript file named `filename.js`.
What command do I use to run a TypeScript file directly?
To run a TypeScript file directly, you can use `ts-node`. Install it via npm with `npm install -g ts-node`, then execute your file using `ts-node filename.ts`.
Do I need to install TypeScript globally to run TypeScript files?
While it’s not mandatory, installing TypeScript globally using `npm install -g typescript` allows you to use the `tsc` command from any directory, simplifying the compilation process.
Can I run TypeScript files in a browser?
TypeScript files cannot be run directly in a browser. They must be compiled to JavaScript first. After compilation, you can include the generated JavaScript file in your HTML.
What is the difference between ‘tsc’ and ‘ts-node’?
The `tsc` command compiles TypeScript files to JavaScript, while `ts-node` allows you to execute TypeScript files directly without manual compilation, making it suitable for development and testing.
How can I set up a TypeScript project for easier file execution?
To set up a TypeScript project, create a `tsconfig.json` file using `tsc –init`. This file allows you to configure compiler options and run the project with `tsc` to compile all files in the project at once.
running TypeScript files requires a clear understanding of the TypeScript compiler and the JavaScript runtime environment. To execute TypeScript code, developers typically need to transpile the TypeScript files (.ts) into JavaScript files (.js) using the TypeScript compiler (tsc). This process converts TypeScript’s advanced features into standard JavaScript that can be executed in any JavaScript environment, such as Node.js or a web browser.
Additionally, developers can streamline their workflow by utilizing tools such as ts-node, which allows for the direct execution of TypeScript files without the need for a separate compilation step. This can significantly enhance productivity during development. Moreover, integrating TypeScript into build systems or using task runners can automate the compilation process, ensuring that TypeScript files are always up-to-date and ready for execution.
Overall, understanding the methods for running TypeScript files not only facilitates effective development practices but also leverages TypeScript’s powerful features. By mastering the compilation and execution processes, developers can enhance their coding efficiency and maintain high-quality codebases that benefit from TypeScript’s type safety and advanced tooling capabilities.
Author Profile
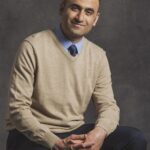
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?