How Can You Effectively Save Data in Python?
In the digital age, data is often referred to as the new oil, and for good reason. Whether you’re a budding programmer, a data scientist, or simply someone looking to manage information more effectively, knowing how to save data in Python is a crucial skill. Python, with its simplicity and versatility, offers a multitude of ways to store data, from basic text files to complex databases. This article will guide you through the various methods available, empowering you to choose the right approach for your projects and ensuring your data is preserved and easily accessible.
Saving data in Python can be as straightforward or as intricate as your needs dictate. At its core, Python provides built-in functions to write to files, allowing you to store everything from simple strings to complex data structures. However, as your projects grow in complexity, you may find yourself needing more robust solutions, such as using libraries designed for data serialization or connecting to databases. Each method has its own advantages, depending on factors like data type, size, and intended use.
In this exploration of data storage in Python, we will touch upon various techniques, including file handling, serialization formats like JSON and CSV, and database interactions. By understanding these options, you’ll be well-equipped to make informed decisions that enhance your programming projects, streamline data management,
Saving Data to Files
When it comes to saving data in Python, one of the most common approaches is to write data to files. Python supports various file formats, allowing users to choose based on their specific needs. The primary file types include text files, CSV files, JSON files, and binary files. Here’s how you can use each of these formats:
Text Files
Saving data to a text file can be done using the built-in `open()` function. You can write data in plain text format, which is human-readable.
“`python
data = “Hello, World!”
with open(‘output.txt’, ‘w’) as file:
file.write(data)
“`
This code snippet creates a text file named `output.txt` and writes “Hello, World!” into it. The `with` statement is a context manager that ensures the file is properly closed after its suite finishes.
CSV Files
CSV (Comma-Separated Values) files are widely used for data storage due to their simplicity and compatibility with various applications. Python’s `csv` module makes it easy to read from and write to CSV files.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘output.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
In this example, a CSV file named `output.csv` is created with a header and two rows of data. The `newline=”` parameter prevents extra blank lines in some environments.
JSON Files
JSON (JavaScript Object Notation) is another popular format for saving data, particularly when working with structured data. Python’s `json` module allows for easy serialization and deserialization of data.
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘output.json’, ‘w’) as file:
json.dump(data, file)
“`
This code saves a dictionary to `output.json`. The `json.dump()` function serializes the dictionary into a JSON formatted string and writes it to the file.
Binary Files
When dealing with non-text data, such as images or audio files, you may want to save data in binary format. You can open a file in binary mode by using `’wb’`.
“`python
data = bytes([120, 3, 255, 0, 100])
with open(‘output.bin’, ‘wb’) as file:
file.write(data)
“`
This example demonstrates saving a byte array to `output.bin`. Using binary mode ensures that the data is written exactly as it is, without any encoding.
Saving Data with Pandas
For data analysis tasks, the Pandas library provides powerful capabilities for saving data in various formats, including CSV, Excel, and HDF5.
“`python
import pandas as pd
df = pd.DataFrame({‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [30, 25]})
df.to_csv(‘output_pandas.csv’, index=)
“`
The `to_csv()` method saves a DataFrame to a CSV file. Other methods like `to_excel()` and `to_hdf()` are available for Excel and HDF5 formats, respectively.
Format | Use Case | Example Method |
---|---|---|
Text | Simple data | open(filename, ‘w’) |
CSV | Tabular data | csv.writer() |
JSON | Structured data | json.dump() |
Binary | Non-text data | open(filename, ‘wb’) |
Pandas | Data analysis | df.to_csv() |
Saving Data to Files
One of the most common ways to save data in Python is by writing it to files. Python supports various file formats, allowing you to choose one that best fits your data needs.
Text Files
To save data as plain text, you can use the built-in `open()` function along with the `write()` method.
“`python
data = “Hello, World!”
with open(‘output.txt’, ‘w’) as file:
file.write(data)
“`
CSV Files
For tabular data, CSV (Comma-Separated Values) format is widely used. The `csv` module provides a straightforward way to handle CSV files.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘output.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
JSON Files
JSON (JavaScript Object Notation) is ideal for storing structured data. The `json` module simplifies saving and loading JSON data.
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘output.json’, ‘w’) as file:
json.dump(data, file)
“`
Saving Data in Databases
For larger datasets or when structured querying is required, databases are preferable. Python supports several database systems, including SQLite, PostgreSQL, and MySQL.
SQLite Example
SQLite is a lightweight, serverless database ideal for small applications.
“`python
import sqlite3
connection = sqlite3.connect(‘example.db’)
cursor = connection.cursor()
cursor.execute(‘CREATE TABLE IF NOT EXISTS users (name TEXT, age INTEGER)’)
cursor.execute(‘INSERT INTO users (name, age) VALUES (?, ?)’, (‘Alice’, 30))
connection.commit()
connection.close()
“`
Saving Data with Pandas
The Pandas library offers powerful data manipulation capabilities and supports various formats for saving data.
Saving DataFrames
You can easily save a DataFrame to different formats like CSV, Excel, and HDF5.
“`python
import pandas as pd
df = pd.DataFrame({‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [30, 25]})
df.to_csv(‘output.csv’, index=) Save as CSV
df.to_excel(‘output.xlsx’, index=) Save as Excel
df.to_hdf(‘output.h5′, key=’df’, mode=’w’) Save as HDF5
“`
Serialization with Pickle
The `pickle` module allows you to serialize Python objects, making it easy to save and load complex data structures.
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
with open(‘data.pkl’, ‘rb’) as file:
loaded_data = pickle.load(file)
“`
Summary of Methods
Here’s a quick overview of the various methods for saving data in Python:
Method | Suitable For | Example Command |
---|---|---|
Text Files | Simple text data | `open(‘file.txt’, ‘w’)` |
CSV | Tabular data | `csv.writer(file)` |
JSON | Structured data | `json.dump(data, file)` |
SQLite | Relational data | `sqlite3.connect(‘database.db’)` |
Pandas | DataFrames | `df.to_csv(‘file.csv’)` |
Pickle | Complex Python objects | `pickle.dump(data, file)` |
Each method has its own advantages, depending on the data type and required operations.
Expert Strategies for Data Preservation in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “When saving data in Python, utilizing libraries such as Pandas for structured data and JSON for lightweight data interchange is crucial. These tools not only facilitate efficient data manipulation but also ensure that the data is easily retrievable and usable across different platforms.”
James Patel (Software Engineer, Cloud Solutions Group). “For persistent storage, I recommend using SQLite with Python’s built-in sqlite3 module. This approach allows for robust data management without the overhead of a full database server, making it ideal for smaller applications or prototypes.”
Linda Martinez (Data Analyst, Analytics Hub). “It is essential to consider data serialization formats like Pickle or HDF5 when saving complex Python objects. These formats preserve the integrity of the data structure and allow for efficient storage and retrieval, especially when dealing with large datasets.”
Frequently Asked Questions (FAQs)
How can I save data to a text file in Python?
You can save data to a text file in Python using the built-in `open()` function along with the `write()` method. For example:
“`python
with open(‘filename.txt’, ‘w’) as file:
file.write(‘Your data here’)
“`
What libraries are commonly used for saving data in Python?
Common libraries for saving data in Python include `pandas` for dataframes, `json` for JSON files, `csv` for CSV files, and `pickle` for serializing Python objects.
How do I save data in JSON format using Python?
To save data in JSON format, use the `json` library. First, convert your data to a JSON-compatible format, then use `json.dump()` to write it to a file:
“`python
import json
data = {‘key’: ‘value’}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
Can I save data in a database using Python?
Yes, you can save data in a database using libraries such as `sqlite3` for SQLite databases or `SQLAlchemy` for more complex database interactions. Use SQL commands to insert data into tables.
What is the method to save data in CSV format in Python?
You can save data in CSV format using the `csv` module. Use `csv.writer()` to write rows to a CSV file:
“`python
import csv
with open(‘data.csv’, ‘w’, newline=”) as csvfile:
writer = csv.writer(csvfile)
writer.writerow([‘Column1’, ‘Column2’])
writer.writerow([‘Value1’, ‘Value2’])
“`
How do I save large datasets efficiently in Python?
For large datasets, consider using the `pandas` library with the `to_csv()` or `to_hdf()` methods, which provide efficient saving options. Alternatively, use formats like Parquet or Feather for better performance and reduced file size.
In Python, saving data can be accomplished through various methods tailored to the specific requirements of the project. The most common approaches include using built-in file handling techniques to write data to text or binary files, utilizing the `pickle` module for serializing Python objects, and leveraging libraries such as `json` for storing data in a readable format. Each method serves distinct purposes and is suitable for different types of data and use cases.
For structured data, the use of databases is often preferred. Python provides several libraries, such as SQLite, SQLAlchemy, and Pandas, which facilitate the interaction with databases and the storage of data in a more organized manner. These tools allow for efficient data management and retrieval, making them ideal for applications requiring persistent data storage.
Additionally, cloud storage solutions and APIs can be integrated into Python applications for saving data remotely. Services like Amazon S3, Google Cloud Storage, and Firebase provide robust options for data storage, ensuring accessibility and scalability. Understanding the various methods for saving data in Python enables developers to choose the most appropriate solution based on their project needs and data characteristics.
Author Profile
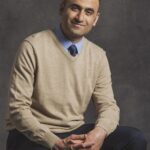
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?