How Can You Effectively Save Data in Python?
In the ever-evolving landscape of programming, Python has emerged as a powerhouse, beloved by beginners and seasoned developers alike. One of the fundamental skills every Python programmer should master is the art of saving data. Whether you’re developing a simple script or a complex application, the ability to efficiently store and retrieve information is paramount. This article will guide you through the various methods and best practices for saving data in Python, ensuring that your applications can maintain state and persist user information seamlessly.
At its core, saving data in Python can be approached in several ways, each suited to different needs and scenarios. From handling simple text files to utilizing powerful databases, Python offers a plethora of options that cater to a wide range of use cases. Understanding these methods is crucial for effective data management, as it allows you to choose the right approach based on the complexity and requirements of your project.
In addition to traditional file handling techniques, Python also supports serialization formats like JSON and CSV, as well as object-oriented solutions such as pickling. Each of these methods has its own advantages and limitations, making it essential for developers to grasp the nuances of each approach. As we delve deeper into the world of data persistence in Python, you’ll discover the tools and strategies that will empower you to save data efficiently and reliably, setting
Saving Data to a File
Saving data to a file in Python can be achieved using various methods, depending on the type of data you are working with and the format you prefer. Common file formats include text files, CSV files, JSON, and binary files. Below are methods to save data in different formats.
Saving as Text Files
To save data as a plain text file, you can use the built-in `open()` function along with the `write()` method. This approach is straightforward and useful for simple data storage.
“`python
data = “Hello, World!”
with open(‘output.txt’, ‘w’) as file:
file.write(data)
“`
This code opens (or creates) a file named `output.txt` in write mode (`’w’`) and writes the string `data` into it. Remember to use the context manager (`with` statement) to ensure that the file is properly closed after writing.
Saving as CSV Files
Comma-Separated Values (CSV) files are widely used for storing tabular data. Python’s built-in `csv` module makes it easy to write to CSV files. Here is an example:
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘output.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
In this example, `writerows()` writes multiple rows at once, while the `newline=”` argument prevents extra blank lines from being added.
Saving as JSON Files
JSON (JavaScript Object Notation) is a popular format for data interchange, especially for APIs. To save data as JSON, you can use the `json` module:
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘output.json’, ‘w’) as file:
json.dump(data, file)
“`
The `json.dump()` method serializes the Python dictionary `data` and writes it to `output.json`.
Saving Binary Data
For saving binary data, such as images or other non-text files, you can use the following approach:
“`python
data = b’This is binary data.’
with open(‘output.bin’, ‘wb’) as file:
file.write(data)
“`
Using `’wb’` mode allows you to write binary data to the file.
Comparison of File Formats
The following table summarizes the various file formats and their typical use cases:
File Format | Use Case | Readability |
---|---|---|
Text (.txt) | Simple text data | Human-readable |
CSV (.csv) | Tabular data | Human-readable |
JSON (.json) | Structured data interchange | Human-readable |
Binary (.bin) | Non-text data | Not human-readable |
Understanding these formats and methods allows for effective data storage and retrieval in Python.
Saving Data in Python
Python offers various methods to save data, allowing for flexibility depending on the type and format of the data you wish to store. Below are some common approaches.
Saving to Text Files
To save simple text data, Python provides built-in functions to handle file operations. The `open()` function is essential for creating or opening files, and you can specify the mode of operation, such as writing or appending.
“`python
Example of saving text data
with open(‘example.txt’, ‘w’) as file:
file.write(“Hello, world!\nThis is a text file.”)
“`
- Modes:
- `’w’`: Write mode (overwrites existing files).
- `’a’`: Append mode (adds to the existing file).
- `’r’`: Read mode (default).
Saving Data as CSV
For structured data, the CSV (Comma-Separated Values) format is widely used. The `csv` module simplifies this process.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘data.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
- Advantages:
- Easily readable and editable.
- Compatible with spreadsheet applications.
Saving Data as JSON
JSON (JavaScript Object Notation) is a popular format for storing data structures. Python’s `json` module enables straightforward serialization and deserialization.
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
“`
- Benefits:
- Human-readable format.
- Supports complex data types (lists, dictionaries).
Saving Data with Pickle
The `pickle` module allows you to serialize and deserialize Python objects, making it useful for saving complex data types.
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30, ‘hobbies’: [‘reading’, ‘hiking’]}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
“`
- Considerations:
- Not human-readable.
- Security risks associated with loading untrusted data.
Saving Data in Databases
For larger datasets or when transactional integrity is required, databases are a better option. Python supports various databases through libraries.
- SQLite:
- Built-in support via `sqlite3`.
- Example of saving data:
“`python
import sqlite3
conn = sqlite3.connect(‘example.db’)
cursor = conn.cursor()
cursor.execute(‘CREATE TABLE IF NOT EXISTS users (name TEXT, age INTEGER)’)
cursor.execute(‘INSERT INTO users (name, age) VALUES (?, ?)’, (‘Alice’, 30))
conn.commit()
conn.close()
“`
- Other databases:
- PostgreSQL, MySQL, MongoDB (requires additional libraries).
Choosing the Right Format
Format | Best For | Pros | Cons |
---|---|---|---|
Text | Simple data | Easy to read/write | Limited structure |
CSV | Tabular data | Widely used | Limited data types |
JSON | Nested data structures | Human-readable | Larger file size |
Pickle | Python object serialization | Supports complex objects | Not cross-language |
Database | Large or structured datasets | Efficient querying | Setup complexity |
Selecting the appropriate method depends on the data complexity, size, and intended use case.
Expert Strategies for Saving Data in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “To effectively save data in Python, it is crucial to leverage libraries such as Pandas for structured data and JSON for hierarchical data formats. These tools not only simplify the process but also enhance data integrity and accessibility.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Utilizing the built-in `pickle` module allows for easy serialization of Python objects, making it a powerful method for saving complex data structures. However, one must be cautious about security when loading pickled data from untrusted sources.”
Sarah Thompson (Python Developer, Open Source Advocates). “For saving data in a more human-readable format, consider using CSV files with the `csv` module. This approach is particularly useful for data interchange and ensures compatibility with various data analysis tools.”
Frequently Asked Questions (FAQs)
How can I save data to a file in Python?
You can save data to a file in Python using the built-in `open()` function along with the `write()` method. For example, to save a string to a text file, use:
“`python
with open(‘filename.txt’, ‘w’) as file:
file.write(‘Your data here’)
“`
What are the different file modes available in Python?
Python supports several file modes, including:
- `’r’`: Read (default mode)
- `’w’`: Write (overwrites existing file)
- `’a’`: Append (adds to existing file)
- `’b’`: Binary mode (for non-text files)
- `’x’`: Exclusive creation (fails if file exists)
How can I save a Python dictionary to a file?
You can save a dictionary to a file using the `json` module. First, import the module and then use `json.dump()`. Example:
“`python
import json
data = {‘key’: ‘value’}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
“`
Is it possible to save data in a binary format in Python?
Yes, you can save data in binary format using the `pickle` module. This allows you to serialize Python objects. Example:
“`python
import pickle
data = {‘key’: ‘value’}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
“`
How do I save a NumPy array to a file?
To save a NumPy array, use the `numpy.save()` function. This saves the array in `.npy` format. Example:
“`python
import numpy as np
array = np.array([1, 2, 3])
np.save(‘array.npy’, array)
“`
Can I save data to a CSV file in Python?
Yes, you can save data to a CSV file using the `csv` module or `pandas` library. Using `csv`:
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘data.csv’, ‘w’,
Saving data in Python is a fundamental skill that enables developers to persist information across program executions. There are various methods to save data, depending on the format and structure required. Common approaches include using built-in modules like `pickle` for serializing Python objects, `json` for handling JSON data, and `csv` for working with comma-separated values. Each method has its own use cases, advantages, and limitations, making it essential for developers to choose the appropriate one based on their specific needs.
In addition to these methods, Python also supports more complex data storage solutions, such as databases. Libraries like `sqlite3` allow developers to interact with SQLite databases, providing a robust way to manage larger datasets. For more extensive applications, frameworks like SQLAlchemy can be utilized to facilitate database interactions in a more abstract manner, enhancing code maintainability and scalability.
Moreover, understanding the nuances of file handling, such as opening, reading, writing, and closing files, is crucial when saving data. It is important to handle exceptions properly to prevent data loss or corruption. Utilizing context managers with the `with` statement can simplify file operations and ensure that resources are managed efficiently.
In summary, saving data in Python can be accomplished
Author Profile
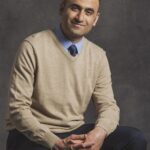
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?