How Can You Effectively Scale Elements Based on Container Width in CSS?
In the ever-evolving world of web design, creating responsive layouts that adapt seamlessly to varying screen sizes has become a fundamental necessity. As more users access websites from a multitude of devices, understanding how to scale elements based on container width using CSS is crucial for delivering an optimal user experience. Whether you’re a seasoned developer or just starting your journey in web design, mastering this skill can elevate your projects and ensure they look stunning on any screen.
Scaling based on container width involves using a combination of CSS properties that allow elements to resize proportionally as the viewport changes. This technique not only enhances the aesthetic appeal of your website but also improves functionality by ensuring that content remains accessible and visually appealing, regardless of the device being used. By leveraging flexible units like percentages, viewport widths, and CSS Grid or Flexbox, designers can create layouts that respond dynamically to the container’s dimensions.
Furthermore, understanding the principles behind responsive design is essential for modern web development. This approach goes beyond mere aesthetics; it encompasses usability and accessibility, ensuring that users have a consistent experience whether they’re browsing on a smartphone, tablet, or desktop. As we delve deeper into the topic of scaling based on container width in CSS, you’ll discover practical strategies and techniques to implement responsive designs effectively, empowering you to create websites that are not
Utilizing CSS Flexbox for Responsive Scaling
To achieve responsive scaling based on container width, one effective method is to utilize CSS Flexbox. Flexbox allows for flexible layouts that can adjust according to the available space in the container, making it ideal for responsive design.
When implementing Flexbox, consider the following properties:
- display: flex; – This enables the flexbox layout on the container.
- flex-direction – Defines the direction of flex items (row, column).
- justify-content – Aligns items along the main axis (flex-start, center, space-between).
- align-items – Aligns items along the cross axis (stretch, center, baseline).
Example CSS for a flex container:
css
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: stretch;
}
This setup will allow items within the container to scale in width as the container itself scales.
Media Queries for Dynamic Adjustments
Media queries are a cornerstone of responsive design, allowing you to apply different styles based on the viewport’s width. This ensures that your layout adapts dynamically as the screen size changes.
A basic media query structure looks like this:
css
@media (max-width: 768px) {
.container {
flex-direction: column; /* Stacks items vertically */
}
}
This example modifies the flex direction when the screen width is 768 pixels or less, enhancing usability on smaller screens.
Scaling Elements with Percentage Widths
Another way to scale elements based on the container width is to use percentage-based widths. This approach ensures that elements maintain a proportional size relative to their parent container.
Example of using percentage widths:
css
.item {
width: 50%; /* Each item takes up half of the container’s width */
}
The benefit of this method is that it allows for easy scaling without needing to specify exact pixel values.
CSS Grid for Advanced Layouts
CSS Grid provides another powerful option for responsive layouts. Unlike Flexbox, which is one-dimensional, Grid allows for two-dimensional layouts, giving you more control over both rows and columns.
Here’s a simple example of a grid setup:
css
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
gap: 10px;
}
In this example, the grid will automatically fill the available space with columns of at least 200 pixels in width, adjusting as the container size changes.
Comparison of CSS Layout Techniques
To help you decide which layout method to use, here’s a comparison table:
Technique | Best For | Advantages | Disadvantages |
---|---|---|---|
Flexbox | One-dimensional layouts | Simple alignment, flexible item sizes | Limited to one direction |
Grid | Two-dimensional layouts | Complex layouts, precise control | Can be more complex to set up |
Media Queries | Responsive design | Adapt styles based on device size | Requires careful planning of breakpoints |
Percentage Widths | Flexible resizing | Simple to implement | Less control over layout behavior |
By choosing the appropriate method or combination of methods, you can effectively scale your elements based on the container width, enhancing the overall user experience across devices.
Understanding Container Width in CSS
The container width in CSS is a fundamental aspect that affects the layout and responsiveness of web design. It defines how elements are proportioned within a container, which can be adjusted dynamically based on the viewport or parent elements.
Key properties that influence container width include:
- Width: Sets the width of the container.
- Max-width: Restricts the width to a maximum value, allowing the container to scale down with the viewport.
- Min-width: Sets a minimum width, preventing the container from becoming too narrow.
Scaling Elements Based on Container Width
To effectively scale elements based on the container width, CSS offers various techniques. Here are several methods:
- Percentage Values: Using percentages allows elements to scale relative to their parent container.
css
.container {
width: 80%; /* Container takes 80% of the viewport width */
}
.child {
width: 50%; /* Child takes 50% of its parent’s width */
}
- Viewport Width (vw): This unit is based on the width of the viewport, allowing for fluid scaling.
css
.responsive-element {
width: 50vw; /* Element takes 50% of the viewport width */
}
- Flexbox: This layout model provides a robust method for creating responsive designs without specifying exact widths.
css
.flex-container {
display: flex;
justify-content: space-between;
}
.flex-item {
flex: 1; /* Items grow and shrink equally */
}
- CSS Grid: A powerful tool for creating complex layouts that can adapt to different container sizes.
css
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr)); /* Creates flexible columns */
}
Media Queries for Responsive Design
Media queries enable you to apply different styles based on the container’s width or the viewport. This technique is crucial for achieving a responsive layout across various devices.
Example of a media query:
css
@media (max-width: 600px) {
.container {
width: 100%; /* Full width on smaller screens */
}
.child {
width: 100%; /* Child elements also full width */
}
}
Using CSS Functions for Dynamic Scaling
CSS provides functions such as `calc()`, `clamp()`, and `min()/max()` to create dynamic and flexible layouts.
- calc(): Combines different units to achieve a desired width.
css
.dynamic-width {
width: calc(100% – 20px); /* Subtracts 20px from the total width */
}
- clamp(): Allows you to set responsive values that stay within a defined range.
css
.responsive-text {
font-size: clamp(1rem, 2vw, 2rem); /* Scales font size between 1rem and 2rem based on viewport width */
}
- min()/max(): Useful for defining minimum or maximum constraints.
css
.flexible-box {
width: min(90%, 600px); /* Width is 90% of the container or 600px, whichever is smaller */
}
Best Practices for Scaling Based on Container Width
Implementing effective strategies for scaling based on container width involves considering the following best practices:
- Use Relative Units: Favor percentages, `em`, `rem`, and viewport units over fixed units like pixels.
- Test on Different Viewports: Always check how your design responds across various screen sizes.
- Keep Accessibility in Mind: Ensure that text remains readable and elements are easily interactable at all sizes.
- Optimize for Performance: Minimize the use of heavy CSS rules that could hinder rendering times.
By adhering to these principles, you can create a responsive design that scales efficiently based on the container’s width, enhancing user experience across devices.
Strategies for Scaling Based on Container Width in CSS
Emily Carter (Front-End Developer, Tech Innovations Inc.). “To effectively scale elements based on container width in CSS, utilizing relative units such as percentages or viewport units (vw, vh) is essential. This approach allows for flexible designs that adapt seamlessly to various screen sizes, enhancing user experience across devices.”
James Thompson (UI/UX Designer, Creative Solutions Agency). “Incorporating CSS Grid and Flexbox can significantly simplify the process of scaling elements according to container width. These layout models provide powerful tools for creating responsive designs that maintain visual hierarchy and alignment, regardless of the viewport dimensions.”
Linda Chen (Web Performance Specialist, Speedy Web Co.). “For optimal performance when scaling based on container width, it is crucial to combine CSS media queries with fluid typography. This ensures that not only do the layout components adjust, but the text remains legible and aesthetically pleasing across all devices.”
Frequently Asked Questions (FAQs)
How can I set an element to scale based on the container width in CSS?
To scale an element based on the container width, use relative units such as percentages or viewport width (vw). For example, setting `width: 50%;` makes the element occupy half the width of its parent container.
What CSS properties are useful for responsive scaling?
Key properties for responsive scaling include `width`, `max-width`, `flex`, and `grid`. These properties allow elements to adapt to different screen sizes and container dimensions effectively.
How do media queries affect scaling based on container width?
Media queries enable you to apply different styles based on the viewport size. You can adjust properties like `width`, `padding`, or `font-size` to ensure elements scale appropriately on various devices.
Can I use CSS Grid to scale elements based on container width?
Yes, CSS Grid allows for responsive layouts. By defining grid columns with relative units (like fractions), elements can scale according to the container’s width, ensuring a flexible and adaptive design.
What is the role of the `calc()` function in scaling elements?
The `calc()` function allows for dynamic calculations in CSS. You can combine different units, such as percentages and pixels, to create more complex responsive designs that scale based on the container width.
How can I ensure images scale based on their container width?
To ensure images scale responsively, set their width to 100% and height to auto with the CSS properties `width: 100%;` and `height: auto;`. This ensures images resize proportionally within their container.
Scaling elements based on container width in CSS is essential for creating responsive web designs that adapt to various screen sizes. By utilizing CSS properties such as percentages, viewport units, and flexible box layouts, designers can ensure that their content remains visually appealing and functional across devices. Techniques like media queries further enhance responsiveness, allowing for tailored adjustments based on specific breakpoints.
One of the most effective methods for achieving scalable designs is the use of relative units like percentages and ems, which allow elements to resize in relation to their parent containers. Additionally, CSS Grid and Flexbox provide powerful tools for creating fluid layouts that automatically adjust based on the available space. These approaches not only improve aesthetics but also enhance user experience by maintaining usability on different devices.
mastering the art of scaling based on container width is crucial for modern web development. By embracing responsive design principles and leveraging CSS capabilities, developers can create websites that are both visually appealing and functionally robust. This adaptability is vital in today’s diverse digital landscape, where users access content from a wide range of devices.
Author Profile
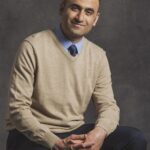
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?