How Can You Monitor API Calls of Websites in Chrome Using Python?
In the ever-evolving landscape of web development and data retrieval, understanding how to interact with APIs has become a vital skill for developers and data enthusiasts alike. Whether you’re building a web application, scraping data for analysis, or simply curious about how different websites communicate with their backend services, knowing how to see API calls can unlock a treasure trove of information. In this article, we will explore the methods and tools available in Google Chrome and Python that allow you to monitor and analyze these API interactions seamlessly.
When you visit a website, your browser engages in a series of behind-the-scenes conversations with various servers, exchanging data through API calls. These interactions can be crucial for understanding how a website functions, what data it retrieves, and how that data is structured. By leveraging the built-in developer tools in Chrome, you can easily track these API requests in real-time, gaining insights into the data flow and the underlying mechanics of the site. This knowledge not only enhances your debugging skills but also empowers you to replicate similar functionalities in your projects.
Moreover, integrating Python into this process opens up a world of possibilities for automation and data manipulation. With libraries designed for web scraping and API interaction, you can programmatically access and analyze the same data you observe in Chrome. This combination of browser-based observation
Using Chrome Developer Tools
To observe API calls made by a website, Chrome Developer Tools (DevTools) is an invaluable resource. Here are the steps to access and utilize it effectively:
- Open Google Chrome and navigate to the desired website.
- Right-click on the page and select Inspect or press `Ctrl + Shift + I` (Windows/Linux) or `Cmd + Option + I` (Mac).
- Navigate to the Network tab within DevTools.
- Reload the page to capture all network requests. Ensure the “Preserve log” checkbox is checked if you want to keep logs of previous requests while navigating.
- Filter the requests by selecting the XHR option, which stands for XMLHttpRequest, commonly used for API calls.
- Click on any request to view detailed information, including headers, response data, and timing.
By following these steps, you can track and analyze how the website interacts with its API.
Using Python for API Call Analysis
While Chrome DevTools provides a straightforward method for inspecting API calls, you can also automate the process using Python. Libraries such as `requests` and `BeautifulSoup` can be utilized to mimic browser behavior and capture API calls programmatically.
Here’s a brief overview of how to implement this:
- Install Required Libraries: Use pip to install the necessary libraries.
bash
pip install requests beautifulsoup4
- Make API Calls: Use the `requests` library to send requests to the API endpoints.
python
import requests
response = requests.get(‘https://api.example.com/data’)
print(response.json()) # Assuming the response is in JSON format
- Parse the Response: Employ `BeautifulSoup` for parsing HTML content if necessary.
python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, ‘html.parser’)
print(soup.title) # Example of extracting the title
This approach allows you to programmatically access and analyze API responses without manual inspection.
Tracking API Calls with Browser Extensions
In addition to using DevTools, several browser extensions can streamline the monitoring of API calls. Here are some popular options:
Extension Name | Description |
---|---|
Postman Interceptor | Captures requests made by the browser for easy testing. |
RESTED | A REST client for Chrome that simplifies API testing. |
Talend API Tester | Offers a comprehensive tool for testing and analyzing APIs. |
To install these extensions, visit the Chrome Web Store, search for the desired extension, and click Add to Chrome. After installation, follow the extension’s instructions to start monitoring API calls.
Summary of Key Techniques
To effectively see API calls from websites in Chrome using Python or browser tools, consider the following techniques:
- Use Chrome DevTools for manual inspection.
- Automate API calls with Python using `requests` and `BeautifulSoup`.
- Utilize browser extensions for enhanced functionality.
These methods provide a robust approach to understanding how web applications communicate with their APIs, facilitating debugging, development, and data extraction tasks.
Using Chrome Developer Tools to Monitor API Calls
To observe API calls made by a website in Google Chrome, you can utilize the built-in Developer Tools. This is a straightforward method that does not require any additional software or programming skills.
- Open Chrome Developer Tools:
- Right-click anywhere on the webpage and select “Inspect” or press `Ctrl + Shift + I` (`Cmd + Option + I` on Mac).
- Navigate to the Network Tab:
- Click on the “Network” tab within the Developer Tools panel.
- Ensure that the “Preserve log” option is checked to retain logs across page navigations.
- Filter for XHR/Fetch Requests:
- Use the filter options to display only XHR or Fetch requests by clicking on the “XHR” button. This will help in isolating the API calls from other network requests.
- Refresh the Page:
- Reload the webpage to capture the API calls made during the initial page load.
- Examine API Calls:
- Click on any of the requests listed in the network panel to view detailed information. You can inspect headers, response data, and timings.
Using Python with Selenium to Capture API Calls
If you prefer to automate the process of monitoring API calls, you can use Python with Selenium, a powerful tool for web automation.
- Install Required Packages:
bash
pip install selenium requests
- Set Up Selenium WebDriver:
python
from selenium import webdriver
# Set up the Chrome WebDriver
options = webdriver.ChromeOptions()
options.add_argument(‘–headless’) # Optional: run in headless mode
driver = webdriver.Chrome(options=options)
- Intercept Network Traffic:
To capture the API calls, you can utilize the `browsermob-proxy` tool alongside Selenium.
bash
pip install browsermob-proxy
python
from browsermobproxy import Server
server = Server(“path/to/browsermob-proxy”) # Specify the path to BrowserMob Proxy
server.start()
proxy = server.create_proxy()
options.add_argument(f’–proxy-server={proxy.proxy}’)
driver = webdriver.Chrome(options=options)
proxy.new_har(“api_calls”) # Start recording the network traffic
driver.get(“https://example.com”) # Navigate to the target website
# Fetch the API call details
har_data = proxy.har # Get the recorded HAR data
- Analyze the HAR Data:
The HAR (HTTP Archive) data contains detailed information about the API calls made during the session. You can parse this data to extract relevant information.
python
for entry in har_data[‘log’][‘entries’]:
request_url = entry[‘request’][‘url’]
response_status = entry[‘response’][‘status’]
print(f”API Call: {request_url}, Status: {response_status}”)
Using Python’s Requests Library for API Testing
For scenarios where you know the API endpoint, you can directly interact with the API using Python’s Requests library.
- Install Requests Library:
bash
pip install requests
- Make API Calls:
python
import requests
url = “https://api.example.com/data”
response = requests.get(url)
if response.status_code == 200:
print(“API Response:”, response.json())
else:
print(“Error:”, response.status_code)
- Handle Authentication if Required:
If the API requires authentication, you can pass the necessary headers or use session-based authentication.
python
headers = {‘Authorization’: ‘Bearer YOUR_TOKEN’}
response = requests.get(url, headers=headers)
By implementing these methods, you can effectively monitor and interact with API calls from websites using Chrome and Python.
Understanding API Calls in Chrome with Python
Dr. Emily Chen (Lead Software Engineer, Tech Innovations Inc.). “To effectively see API calls made by websites in Chrome while using Python, one can utilize the Chrome Developer Tools. By navigating to the ‘Network’ tab, developers can monitor all network requests, including API calls, and analyze their responses. Additionally, employing libraries such as ‘requests’ in Python can help automate the process of making similar API calls.”
Mark Thompson (Web Development Consultant, CodeCraft Solutions). “For those looking to see API calls in Chrome while integrating Python, I recommend using browser extensions like ‘Postman’ or ‘RESTer’. These tools allow users to replicate and inspect API requests easily. Coupled with Python scripts using ‘selenium’, you can automate the browser to capture these calls dynamically.”
Sarah Patel (Data Analyst, Insightful Analytics). “Understanding how to see API calls in Chrome is crucial for debugging and optimizing web applications. Utilizing the ‘Fetch/XHR’ filter in the Network tab of Chrome Developer Tools enables developers to focus specifically on API calls. Furthermore, leveraging Python’s ‘http.client’ module can facilitate deeper analysis and testing of these endpoints.”
Frequently Asked Questions (FAQs)
How can I view API calls made by a website in Chrome?
You can view API calls in Chrome by opening the Developer Tools (F12 or right-click and select “Inspect”), navigating to the “Network” tab, and refreshing the page. Filter by “XHR” to see only API requests.
What tools can I use in Chrome to analyze API calls?
The built-in Developer Tools in Chrome provide a comprehensive suite for analyzing API calls. You can also use extensions like Postman or RESTer for more advanced testing and analysis.
Can I capture API calls made by JavaScript on a webpage?
Yes, the Network tab in Chrome’s Developer Tools captures all network requests, including those initiated by JavaScript. Ensure you have the “Preserve log” option checked to maintain the log during page navigation.
Is it possible to see the response data of API calls in Chrome?
Yes, in the Network tab, click on a specific API request to view its details. You can see the response data in the “Response” section, which displays the returned data in various formats, such as JSON or XML.
How can I make API calls using Python after inspecting them in Chrome?
After inspecting API calls in Chrome, you can replicate them in Python using libraries like `requests`. Extract the necessary headers, parameters, and endpoints from the Network tab to construct your API request.
What are common issues when viewing API calls in Chrome?
Common issues include network throttling, caching, and CORS (Cross-Origin Resource Sharing) restrictions. Ensure that caching is disabled in the Network tab and check for any CORS errors in the console if requests fail.
monitoring API calls made by websites in Chrome can be achieved through various methods, particularly using Python. One effective approach involves utilizing browser developer tools to inspect network activity, which allows users to see all requests and responses made to and from the server. This method is straightforward and does not require any additional programming knowledge, making it accessible to a broad audience.
For those looking to automate the process or integrate it into a larger workflow, libraries such as Selenium can be employed. Selenium allows users to programmatically control a web browser, enabling the capture of API calls during web interactions. Additionally, using Python’s requests library alongside a proxy tool like Fiddler or Charles can facilitate the interception of API calls, providing detailed insights into the data exchanged between the client and server.
Overall, understanding how to observe and analyze API calls is crucial for developers, data analysts, and anyone interested in web development. By leveraging the tools available in Chrome and Python, users can gain a deeper understanding of how websites function, troubleshoot issues, and optimize their own applications. This knowledge is not only beneficial for debugging but also for enhancing the performance and security of web applications.
Author Profile
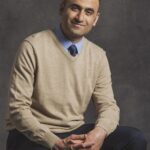
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?