How Can You Check If a File Exists in Python?
When working with files in Python, one of the most fundamental tasks you’ll encounter is checking whether a file exists before attempting to read from or write to it. This seemingly simple operation is crucial for ensuring the robustness of your code and preventing errors that could arise from trying to access a non-existent file. Whether you’re developing a small script or a large application, understanding how to efficiently verify the existence of a file can save you time and headaches down the line.
In Python, there are various methods to check for a file’s existence, each with its own advantages and use cases. From leveraging built-in libraries to utilizing exception handling, Python provides a range of tools that can help you seamlessly integrate file existence checks into your workflows. This not only enhances the reliability of your code but also improves user experience by allowing your programs to handle file-related operations gracefully.
As you dive deeper into the world of file management in Python, you’ll discover that mastering these techniques opens up a realm of possibilities for data manipulation, automation, and more. Whether you’re a beginner looking to understand the basics or an experienced developer seeking to refine your skills, knowing how to check for file existence is an essential building block in your programming toolkit. Get ready to explore the methods and best practices that will empower you to handle files like a
Using the `os` Module
To check if a file exists in Python, the `os` module provides a straightforward method. The `os.path` submodule has a function called `exists()` that returns `True` if the file exists and “ otherwise.
Here’s a simple example demonstrating its usage:
python
import os
file_path = ‘example.txt’
if os.path.exists(file_path):
print(“File exists.”)
else:
print(“File does not exist.”)
This method is effective as it checks for both files and directories.
Using the `pathlib` Module
The `pathlib` module, introduced in Python 3.4, offers an object-oriented approach to handling filesystem paths. The `Path` class has an `exists()` method that can also be utilized to check for file existence.
Here’s how to use `pathlib`:
python
from pathlib import Path
file_path = Path(‘example.txt’)
if file_path.exists():
print(“File exists.”)
else:
print(“File does not exist.”)
This method enhances readability and allows for more complex path manipulations.
Checking for Specific File Types
If you need to check for specific types of files, both `os` and `pathlib` can be used in conjunction with other functions. For instance, you might want to ensure that the file is not only present but is also a file and not a directory.
Using `os`:
python
if os.path.isfile(file_path):
print(“It’s a file.”)
elif os.path.isdir(file_path):
print(“It’s a directory.”)
else:
print(“File does not exist.”)
Using `pathlib`:
python
if file_path.is_file():
print(“It’s a file.”)
elif file_path.is_dir():
print(“It’s a directory.”)
else:
print(“File does not exist.”)
Performance Considerations
When deciding on which method to use, consider the following performance aspects:
Method | Ease of Use | Performance | Compatibility |
---|---|---|---|
os.path.exists() | Easy | Fast | Python 2 and 3 |
pathlib.Path.exists() | Very Easy | Fast | Python 3.4+ |
Choosing between `os` and `pathlib` often depends on your specific needs and Python version. For modern applications, `pathlib` is generally recommended for its clarity and additional functionality.
In summary, checking for file existence in Python can be accomplished through various methods, each with its own advantages. Select the method that best fits your application’s requirements and coding style.
Checking File Existence with `os` Module
The `os` module in Python provides a simple way to check if a file exists. The `os.path` submodule contains the `exists()` method, which returns `True` if the specified path refers to an existing file or directory.
python
import os
file_path = ‘example.txt’
if os.path.exists(file_path):
print(“File exists.”)
else:
print(“File does not exist.”)
This method works well for both files and directories, making it versatile for various applications.
Using `pathlib` for File Existence Check
The `pathlib` module offers an object-oriented approach to handling filesystem paths. The `Path` class includes an `exists()` method that can be used to determine if a file or directory exists.
python
from pathlib import Path
file_path = Path(‘example.txt’)
if file_path.exists():
print(“File exists.”)
else:
print(“File does not exist.”)
This approach is generally more modern and preferred in recent Python versions due to its readability and ease of use.
Checking for Files Specifically
If you need to check specifically for a file (as opposed to a directory), you can use the `isfile()` method from both `os.path` and `pathlib`.
Using `os.path`:
python
if os.path.isfile(file_path):
print(“File exists and is a file.”)
else:
print(“File does not exist or is not a file.”)
Using `pathlib`:
python
if file_path.is_file():
print(“File exists and is a file.”)
else:
print(“File does not exist or is not a file.”)
File Existence in a Try-Except Block
Another method is to attempt to open the file within a try-except block. This can be useful if you plan to work with the file immediately after checking for its existence.
python
try:
with open(‘example.txt’, ‘r’) as file:
print(“File exists and is opened successfully.”)
except FileNotFoundError:
print(“File does not exist.”)
This approach can be beneficial when you want to perform additional operations right after confirming the file’s existence.
Performance Considerations
When checking for file existence, consider the following:
- File System Performance: Repeated checks on the same file can lead to performance overhead; caching results may be necessary for frequent accesses.
- Network Drives: Checking file existence on network drives can be slower than local checks due to latency.
- Error Handling: Always include error handling, especially when dealing with files that may be modified by other processes.
These considerations ensure that your application handles file checks efficiently and reliably.
Expert Insights on Checking File Existence in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the most straightforward way to check if a file exists is by using the `os.path.exists()` function. This method is efficient and provides a reliable boolean response indicating the presence of the specified file.”
Michael Chen (Python Developer, Data Science Solutions). “For a more modern approach, I recommend using the `pathlib` library, specifically `Path.exists()`. This method not only checks for file existence but also enhances code readability and maintainability, making it a preferable choice in contemporary Python development.”
Sarah Thompson (Lead Instructor, Python Programming Academy). “When teaching file handling in Python, I emphasize the importance of error handling alongside existence checks. Utilizing `try` and `except` blocks can help manage exceptions that arise when attempting to access non-existent files, ensuring robust code.”
Frequently Asked Questions (FAQs)
How can I check if a file exists in Python?
You can check if a file exists in Python using the `os.path.exists()` function or the `pathlib.Path.exists()` method. Both methods return `True` if the file exists and “ otherwise.
What is the difference between os.path.exists() and pathlib.Path.exists()?
`os.path.exists()` is part of the older `os` module, while `pathlib` is a more modern approach introduced in Python 3.4. `pathlib` provides an object-oriented interface for file system paths, making it more intuitive for many users.
Can I check for a directory’s existence in the same way?
Yes, you can check for a directory’s existence using the same methods. Both `os.path.exists()` and `pathlib.Path.exists()` will return `True` for directories as well as files.
What happens if I check for a file that is not accessible due to permissions?
If a file is not accessible due to permissions, both `os.path.exists()` and `pathlib.Path.exists()` will still return `True` if the file exists on the filesystem. They do not check for read permissions.
Is there a way to check if a file exists and is a file, not a directory?
Yes, you can use `os.path.isfile()` or `pathlib.Path.is_file()` to specifically check if a path is a file. These methods return `True` only if the path exists and is a regular file.
What is the recommended approach for checking file existence in modern Python code?
The recommended approach is to use the `pathlib` module due to its readability and ease of use. For example, you can create a `Path` object and call the `exists()` method to check for file existence.
In Python, determining whether a file exists is a fundamental task that can be accomplished using various methods. The most common approach is to utilize the `os` module, specifically the `os.path.exists()` function. This function checks the specified path and returns a boolean value indicating the presence of the file. Another effective method is to use the `pathlib` module, which provides an object-oriented interface for filesystem paths. The `Path.exists()` method from this module serves the same purpose, offering a more modern and readable syntax.
Additionally, handling exceptions is a prudent practice when working with file operations. The `try-except` block can be employed to catch `FileNotFoundError`, which occurs when attempting to open a file that does not exist. This approach not only checks for the file’s existence but also allows for graceful error handling, ensuring that the program can continue running without interruption.
In summary, Python offers multiple reliable methods for checking if a file exists, each with its own advantages. The choice between using the `os` module or the `pathlib` module often depends on the specific requirements of the project and personal coding preferences. Understanding these methods enhances a programmer’s ability to manage files effectively and contributes to more robust
Author Profile
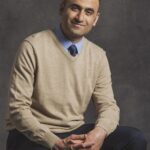
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?