How Can You Check If a Value Exists in a List in Python?
In the world of programming, efficiency and clarity are paramount, especially when working with data structures like lists in Python. Whether you’re a seasoned developer or just starting your coding journey, knowing how to check if a specific value exists within a list is a fundamental skill that can save you time and enhance your code’s functionality. This seemingly simple task can have significant implications in data manipulation, conditional logic, and algorithm design, making it a crucial topic for anyone looking to master Python.
When you find yourself needing to determine the presence of a value in a list, Python offers several straightforward methods to accomplish this. Understanding these techniques not only empowers you to write cleaner code but also equips you with the tools to handle more complex data scenarios. From leveraging built-in functions to utilizing Python’s expressive syntax, there are multiple approaches that cater to different needs and preferences.
As we delve deeper into this topic, we will explore the various methods available for checking the existence of a value in a list. By the end of this article, you’ll have a comprehensive understanding of how to efficiently perform this task, allowing you to enhance your programming skills and tackle more advanced challenges with confidence. Whether you need a quick check or are implementing a more intricate solution, the knowledge you gain here will be invaluable in your Python programming toolkit
Methods to Check for Value in a List
In Python, there are several effective methods to determine whether a specific value exists within a list. Each method varies in terms of syntax and performance, and the choice may depend on the context of use. Below are some of the most common techniques.
Using the `in` Keyword
The simplest and most Pythonic way to check if a value is present in a list is by using the `in` keyword. This approach is not only easy to read but also efficient for most cases.
python
my_list = [1, 2, 3, 4, 5]
value = 3
if value in my_list:
print(“Value found!”)
else:
print(“Value not found.”)
This method returns `True` if the value is found and “ otherwise.
Using the `list.index()` Method
Another method to check for the existence of a value in a list is by using the `index()` method. However, this approach will raise a `ValueError` if the value is not found, so it should be used with caution.
python
my_list = [1, 2, 3, 4, 5]
value = 6
try:
index = my_list.index(value)
print(f”Value found at index {index}.”)
except ValueError:
print(“Value not found.”)
This method is useful when you also need the index of the value in the list.
Using List Comprehension
List comprehension can also be used to check for a value in a list, although it is less straightforward than the `in` keyword. This method creates a new list containing only the matches.
python
my_list = [1, 2, 3, 4, 5]
value = 3
found = [x for x in my_list if x == value]
if found:
print(“Value found!”)
else:
print(“Value not found.”)
While this method works, it is generally less efficient than using `in` due to the overhead of creating a new list.
Performance Comparison
When considering performance, the `in` keyword is typically the best option for average cases. Here’s a brief comparison of the methods:
Method | Time Complexity | Space Complexity |
---|---|---|
in keyword | O(n) | O(1) |
list.index() | O(n) | O(1) |
List comprehension | O(n) | O(n) |
In summary, while all methods effectively check for the existence of a value in a list, the `in` keyword is preferred for its simplicity and efficiency in most scenarios.
Checking for Value in a List
In Python, determining whether a specific value exists within a list can be accomplished using several straightforward methods. The most common approaches include using the `in` keyword, the `index()` method, and the `count()` method. Each method has its own advantages depending on the context of the check.
Using the `in` Keyword
The `in` keyword is the most intuitive way to check for a value’s presence in a list. It returns a boolean value: `True` if the item is found, and “ otherwise.
python
my_list = [1, 2, 3, 4, 5]
value_to_check = 3
if value_to_check in my_list:
print(“Value exists in the list.”)
else:
print(“Value does not exist in the list.”)
Using the `index()` Method
The `index()` method can also be utilized to check for a value’s presence. This method returns the index of the first occurrence of the specified value. If the value is not found, it raises a `ValueError`.
python
my_list = [1, 2, 3, 4, 5]
value_to_check = 6
try:
index = my_list.index(value_to_check)
print(f”Value found at index: {index}.”)
except ValueError:
print(“Value does not exist in the list.”)
Using the `count()` Method
The `count()` method counts the occurrences of a specified value in the list. If the count is greater than zero, the value exists in the list.
python
my_list = [1, 2, 3, 4, 5]
value_to_check = 2
if my_list.count(value_to_check) > 0:
print(“Value exists in the list.”)
else:
print(“Value does not exist in the list.”)
Performance Considerations
When choosing a method to check for a value in a list, consider the following performance aspects:
Method | Time Complexity | Raises Exception |
---|---|---|
`in` | O(n) | No |
`index()` | O(n) | Yes (if not found) |
`count()` | O(n) | No |
- The `in` keyword is generally preferred for its clarity and simplicity.
- The `index()` method is useful when the index of the item is also needed, but it requires error handling.
- The `count()` method is beneficial when you need to know how many times a value appears in the list.
These methods provide flexible options for checking the presence of values in a Python list. Depending on your specific needs—whether you require a simple existence check, the index of the item, or the count of occurrences—Python offers efficient and readable solutions.
Understanding List Value Checks in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To determine if a value exists in a list in Python, the most straightforward method is using the ‘in’ keyword. This approach is both readable and efficient, making it a preferred choice for many developers.”
Michael Chen (Python Developer, CodeMasters). “For larger datasets, utilizing the ‘set’ data structure can significantly improve lookup times. Converting a list to a set allows for average O(1) complexity when checking for membership, compared to O(n) for lists.”
Sarah Patel (Data Scientist, Analytics Hub). “When checking for the presence of a value in a list, it’s essential to consider the context. If the list contains duplicates and you need to know how many times a value appears, using the ‘count()’ method would be more appropriate.”
Frequently Asked Questions (FAQs)
How can I check if a value exists in a list in Python?
You can use the `in` keyword to check for the existence of a value in a list. For example, `value in my_list` will return `True` if the value is found and “ otherwise.
What is the time complexity of checking for a value in a list?
The time complexity for checking if a value is in a list is O(n), where n is the number of elements in the list. This is because the search may need to check each element.
Can I check for multiple values in a list at once?
Yes, you can use a loop or a list comprehension to check for multiple values. For instance, `[value in my_list for value in values_to_check]` will return a list of Boolean values indicating the presence of each value.
Is there a more efficient way to check for values in a large list?
For large datasets, consider using a set instead of a list. Sets provide average time complexity of O(1) for membership tests, making them more efficient for large collections.
What happens if the value I am checking for is not in the list?
If the value is not in the list, the expression `value in my_list` will evaluate to “, and no error will be raised. It simply indicates the absence of the value.
Can I use the `index()` method to check for a value in a list?
Yes, the `index()` method can be used to find the position of a value in a list. However, if the value is not present, it raises a `ValueError`. Therefore, it is safer to use the `in` keyword for checking existence.
In Python, checking if a value exists within a list is a fundamental operation that can be accomplished using several methods. The most straightforward approach is to use the `in` keyword, which returns a boolean indicating whether the specified value is present in the list. For example, the expression `value in my_list` will yield `True` if the value is found and “ otherwise. This method is not only simple but also efficient for most use cases.
Another method to determine if a value is in a list is to utilize the `list.count()` method, which counts the occurrences of a specified value within the list. If the count is greater than zero, it indicates that the value exists in the list. However, this approach is less efficient than using the `in` keyword, especially for large lists, as it requires traversing the entire list to count occurrences.
For more advanced scenarios, such as when dealing with large datasets or needing to check for membership frequently, converting the list to a set can be beneficial. Sets in Python offer average time complexity of O(1) for membership tests, making them significantly faster than lists for this purpose. By using the expression `value in my_set`, one can quickly ascertain the
Author Profile
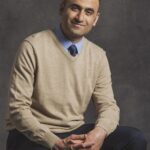
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?