How Can You Send an Email from Python? A Step-by-Step Guide!
In an increasingly digital world, the ability to communicate effectively and efficiently is paramount. Whether you’re automating notifications for your web application, sending out newsletters, or simply reaching out to colleagues, sending emails programmatically can save time and enhance productivity. Python, with its rich ecosystem of libraries and straightforward syntax, makes this task not only feasible but also enjoyable. If you’ve ever wondered how to harness the power of Python to send emails, you’re in the right place.
Sending an email from Python involves a few essential steps, including setting up the right environment and using the appropriate libraries. The process typically begins with importing the necessary modules that facilitate email creation and transmission. From there, you can craft your message, specifying details like the recipient, subject, and body content. Python’s versatility allows you to send plain text emails, HTML messages, or even attachments, catering to a variety of communication needs.
Moreover, understanding how to send an email from Python opens up a world of possibilities for automation and integration with other systems. With just a few lines of code, you can trigger emails based on events in your application, making it easier to keep users informed or to manage tasks efficiently. As we delve deeper into this topic, you’ll discover the tools and techniques that will empower you to master email communication through
Using the smtplib Library
The `smtplib` library in Python is a built-in module that facilitates sending emails using the Simple Mail Transfer Protocol (SMTP). To send an email, you need to establish a connection with an SMTP server and authenticate with your credentials. Below is an example of how to send a basic email.
“`python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
Email configuration
sender_email = “[email protected]”
receiver_email = “[email protected]”
subject = “Test Email”
body = “This is a test email sent from Python.”
Create the email
msg = MIMEMultipart()
msg[‘From’] = sender_email
msg[‘To’] = receiver_email
msg[‘Subject’] = subject
msg.attach(MIMEText(body, ‘plain’))
Send the email
try:
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls() Upgrade the connection to a secure encrypted SSL/TLS connection
server.login(sender_email, ‘your_password’) Authenticate
server.sendmail(sender_email, receiver_email, msg.as_string()) Send the email
print(“Email sent successfully!”)
except Exception as e:
print(f”Error occurred: {e}”)
“`
In this example, you need to replace `smtp.example.com` with your email provider’s SMTP server and use the appropriate port (like 587 for TLS). The email content is created using the `MIMEText` and `MIMEMultipart` classes from the `email` module, which helps structure the email properly.
Using the email Module
The `email` module is integral for crafting complex emails that may include HTML content, attachments, or multiple recipients. This module allows you to construct more intricate email messages beyond plain text.
To create a more sophisticated email, consider the following structure:
- Use `MIMEText` for plain text or HTML content.
- Use `MIMEImage`, `MIMEAudio`, or `MIMEBase` for attachments.
- Utilize `MIMEMultipart` to combine various parts into a single message.
Here’s an example of sending an email with an HTML body:
“`python
html_body = “””\
This is a test email
This email is sent from Python!
“””
msg.attach(MIMEText(html_body, ‘html’))
“`
Email Configuration Table
Field | Description |
---|---|
SMTP Server | The address of the email provider’s SMTP server (e.g., smtp.gmail.com). |
Port | Port number used for SMTP (usually 587 for TLS or 465 for SSL). |
Sender Email | Your email address from which the email is sent. |
Receiver Email | The recipient’s email address. |
Subject | The subject line of the email. |
Body | The content of the email, either plain text or HTML. |
By following these guidelines and utilizing the `smtplib` and `email` modules effectively, you can send various types of emails from Python, enhancing your application’s communication capabilities.
Using the smtplib Library
The `smtplib` library in Python is the built-in way to send emails using the Simple Mail Transfer Protocol (SMTP). This library provides a straightforward interface for connecting to an SMTP server and sending emails.
Basic Example of Sending an Email
Here is a simple example to demonstrate how to send an email using `smtplib`:
“`python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
Email configuration
sender_email = “[email protected]”
receiver_email = “[email protected]”
password = “your_password”
Create the email
msg = MIMEMultipart()
msg[‘From’] = sender_email
msg[‘To’] = receiver_email
msg[‘Subject’] = “Test Email”
Email body
body = “This is a test email sent from Python.”
msg.attach(MIMEText(body, ‘plain’))
Sending the email
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls() Secure the connection
server.login(sender_email, password)
server.send_message(msg)
“`
Components of the Code
- Imports: The `smtplib` library is used for the SMTP protocol, while `MIMEText` and `MIMEMultipart` from the `email.mime` module help in creating the email structure.
- Email Configuration:
- `sender_email`: Your email address.
- `receiver_email`: Recipient’s email address.
- `password`: The password for your email account.
- Email Creation:
- An instance of `MIMEMultipart` is created, which allows you to add multiple parts to your email (like text and attachments).
- The subject and body are defined.
- Sending the Email:
- A connection is made to the SMTP server.
- The connection is secured using `starttls()`.
- The sender logs into the server with their credentials.
- Finally, the email message is sent.
Using Environment Variables for Security
Storing email credentials directly in your code is not secure. Instead, use environment variables to protect sensitive information. Here’s how you can do this:
- Set environment variables in your operating system:
- On Linux or Mac:
“`bash
export EMAIL_USER=”[email protected]”
export EMAIL_PASS=”your_password”
“`
- On Windows:
“`cmd
set [email protected]
set EMAIL_PASS=your_password
“`
- Access them in your Python script:
“`python
import os
sender_email = os.getenv(‘EMAIL_USER’)
password = os.getenv(‘EMAIL_PASS’)
“`
Handling Exceptions
It is important to handle exceptions when sending emails to manage errors gracefully. Here’s an example of how to implement error handling:
“`python
try:
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls()
server.login(sender_email, password)
server.send_message(msg)
except smtplib.SMTPException as e:
print(f”An error occurred: {e}”)
“`
By wrapping the email-sending block in a `try-except` statement, you can catch exceptions specific to SMTP and handle them according to your application’s needs.
Common SMTP Servers and Ports
Service Provider | SMTP Server | Port |
---|---|---|
Gmail | smtp.gmail.com | 587 |
Yahoo Mail | smtp.mail.yahoo.com | 587 |
Outlook | smtp.office365.com | 587 |
SendGrid | smtp.sendgrid.net | 587 |
This table provides a quick reference for common SMTP servers and their corresponding ports, facilitating configuration for various email services.
Expert Insights on Sending Emails with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Sending emails from Python can be efficiently accomplished using the built-in `smtplib` library. This library provides a straightforward interface for connecting to an SMTP server and sending messages, making it an ideal choice for developers looking to automate email notifications.”
Michael Tran (Python Developer, CodeCraft Solutions). “When implementing email functionality in Python, it is crucial to handle exceptions properly. Network issues or authentication failures can occur, so wrapping the email-sending logic in a try-except block will help ensure that your application can gracefully handle errors.”
Sarah Patel (Data Scientist, Analytics Hub). “For projects requiring enhanced email features, such as HTML content or attachments, I recommend using the `email` module in conjunction with `smtplib`. This allows for more complex email structures and improves the overall user experience when sending automated reports.”
Frequently Asked Questions (FAQs)
How can I send an email using Python?
You can send an email using Python by utilizing the built-in `smtplib` library. This library allows you to connect to an SMTP server and send emails programmatically.
What is the basic code structure for sending an email in Python?
The basic structure involves importing `smtplib`, creating a connection to the SMTP server, logging in with your credentials, and using the `sendmail` method to dispatch the email.
Do I need to install any additional libraries to send emails from Python?
No additional libraries are required for basic email sending, as `smtplib` is included in Python’s standard library. However, for HTML emails or attachments, consider using the `email` module.
How do I handle email attachments in Python?
To handle attachments, you can use the `email` module along with `MIME` classes. Create a `MIMEBase` object for the attachment and encode it properly before adding it to the email message.
What should I do if my email fails to send?
If your email fails to send, check the SMTP server settings, ensure your credentials are correct, and verify that your network connection is stable. Additionally, review any error messages for specific issues.
Is it safe to send emails with sensitive information using Python?
Sending sensitive information via email can pose security risks. Always use secure connections (e.g., SMTP over SSL/TLS) and consider encrypting the email content to protect sensitive data.
Sending an email from Python is a straightforward process that can be accomplished using the built-in `smtplib` library. This library provides a simple interface for connecting to an email server and sending messages. To begin, users need to establish a connection with the SMTP server, authenticate using their credentials, and then compose the email content, including the recipient’s address, subject line, and body. Once the email is prepared, it can be sent using the `sendmail` method.
In addition to `smtplib`, Python also supports other libraries such as `email` for constructing email messages in a more structured manner. This library allows users to create multipart emails, attach files, and format the content appropriately. By combining `smtplib` with the `email` library, developers can enhance their email-sending capabilities, making it possible to send rich content and attachments seamlessly.
It is important to consider security when sending emails programmatically. Using secure connections through TLS or SSL is highly recommended to protect sensitive information. Additionally, users should be aware of the limitations and policies of their email service provider, as some may restrict the number of emails sent or require specific configurations for third-party applications.
In summary, sending
Author Profile
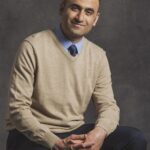
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?