How Can You Effectively Send Messages to a Network Interface?
In our increasingly interconnected world, the ability to communicate effectively with network interfaces is crucial for developers, network engineers, and tech enthusiasts alike. Whether you’re troubleshooting connectivity issues, developing network applications, or simply exploring the intricacies of data transmission, understanding how to send messages to a network interface can unlock a wealth of possibilities. This process not only enhances your technical skill set but also empowers you to harness the full potential of your networked devices.
Sending messages to a network interface involves a series of steps that bridge the gap between software applications and hardware components. At its core, this process requires a solid grasp of networking protocols, data encapsulation, and the underlying architecture of the operating system. By mastering these concepts, you can efficiently transmit data packets, monitor network traffic, and even implement custom communication protocols tailored to your specific needs.
Moreover, the ability to interact with network interfaces opens up a range of applications, from crafting simple scripts that automate routine tasks to developing complex systems that require real-time data exchange. As we delve deeper into the methods and tools available for sending messages to network interfaces, you’ll discover not only the technical know-how but also the creative potential that lies within this essential skill. Prepare to embark on a journey that will enhance your understanding of networking and empower you to communicate seamlessly with
Understanding Network Interfaces
Network interfaces are crucial components in computer networking, serving as the points of interaction between a device and a network. They can be physical, like Ethernet cards, or virtual, such as those created by software-defined networking. When sending messages to a network interface, it is essential to understand the protocols involved and the proper methods of communication.
Protocols for Sending Messages
When sending messages to a network interface, various protocols can be employed depending on the nature of the communication. The most common protocols include:
- TCP (Transmission Control Protocol): Ensures reliable and ordered delivery of messages.
- UDP (User Datagram Protocol): Provides a connectionless communication method, suitable for applications where speed is critical.
- ICMP (Internet Control Message Protocol): Used for diagnostic purposes and network error reporting.
Each protocol has its use cases and implications for message delivery. TCP is ideal for applications requiring data integrity, while UDP is preferred for real-time applications like video streaming.
Methods of Sending Messages
There are several methods to send messages to a network interface. Below are some of the most common approaches:
- Socket Programming: This method involves creating a socket, which acts as an endpoint for sending and receiving messages. Socket programming can be done in various programming languages, such as Python, C, and Java.
- Using Command-Line Tools: Tools like `ping` and `curl` can be used to send messages over the network directly from the command line. These tools can help test connectivity and send HTTP requests, respectively.
- Network APIs: Many platforms offer APIs for network communication, simplifying the process of sending messages. For example, using libraries like `requests` in Python allows easy HTTP requests.
Example of Sending Messages Using Socket Programming
Here is a simple example of how to send a message using Python’s socket library:
“`python
import socket
Create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Define the host and port
host = ‘127.0.0.1’
port = 12345
Connect to the server
s.connect((host, port))
Send a message
s.sendall(b’Hello, Network Interface!’)
Close the socket
s.close()
“`
This code snippet demonstrates the fundamental steps of creating a socket, connecting to a specified host and port, sending a message, and closing the connection.
Common Considerations
When sending messages to a network interface, several factors should be taken into account:
- Network Latency: Understand the potential delays in message delivery, especially in high-traffic networks.
- Security: Implement encryption and secure protocols to protect sensitive information during transmission.
- Error Handling: Prepare for message loss or corruption by incorporating error detection and correction mechanisms.
Summary of Methods and Tools
The following table summarizes the methods and tools available for sending messages to a network interface:
Method | Description | Use Case |
---|---|---|
Socket Programming | Creating a connection through a programming interface | Custom applications requiring direct communication |
Command-Line Tools | Using built-in tools for quick testing and communication | Network diagnostics and simple requests |
Network APIs | Leveraging libraries for easier integration | Web applications and services |
Understanding these methods and considerations will enable effective communication with network interfaces, ensuring reliable data transmission across networks.
Understanding Network Interfaces
Network interfaces serve as the point of communication between devices and networks. They can be physical (like Ethernet ports) or virtual (such as loopback interfaces). To send messages to a network interface, it is crucial to understand the type of interface and the appropriate protocols to utilize.
Protocols for Sending Messages
Different protocols can be used to send messages to a network interface, each serving specific purposes:
- TCP (Transmission Control Protocol): Reliable, connection-oriented protocol used for sending messages that require acknowledgment.
- UDP (User Datagram Protocol): Connectionless protocol ideal for applications that prioritize speed over reliability.
- ICMP (Internet Control Message Protocol): Used for diagnostic purposes, such as sending error messages and operational information.
Methods to Send Messages
There are various methods to send messages to a network interface:
Using Command Line Tools
Several command-line tools can facilitate message sending:
- Ping: Utilizes ICMP to send echo requests to a specified address.
- Command: `ping
`
- Netcat (nc): Versatile tool for reading from and writing to network connections.
- Command: `echo “Hello” | nc
`
- Telnet: Sends messages over TCP to a specified port.
- Command: `telnet
`
Programming Approaches
For automated messaging, programming languages can be employed:
- Python: Libraries like `socket` allow the creation of client-server applications.
Example Code:
“`python
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.sendto(b’Hello, Network!’, (‘
“`
- C/C++: Utilizes sockets for network communication.
Example Code:
“`c
include
include
include
int main() {
int sock;
struct sockaddr_in server;
sock = socket(AF_INET, SOCK_DGRAM, 0);
server.sin_family = AF_INET;
server.sin_port = htons(
server.sin_addr.s_addr = inet_addr(“
sendto(sock, “Hello, Network!”, strlen(“Hello, Network!”), 0, (struct sockaddr*)&server, sizeof(server));
return 0;
}
“`
Considerations for Sending Messages
When sending messages, several factors should be considered:
- Network Configuration: Ensure that firewalls and security settings permit outgoing messages to the target interface.
- Message Size: Be aware of protocol limits; for instance, UDP has a maximum payload size of 65,507 bytes.
- Destination Addressing: Accurate IP addressing is critical for successful communication.
- Error Handling: Implement mechanisms to handle potential errors or dropped packets, especially with UDP.
Testing and Validation
To ensure successful message delivery, testing tools can be employed:
- Wireshark: Network protocol analyzer to inspect traffic and confirm message transmission.
- Traceroute: To determine the path packets take to reach the network interface.
- Curl: For testing web interfaces by sending HTTP requests.
By understanding these methods and tools, one can effectively send messages to a network interface, optimizing communication for various applications.
Expert Insights on Sending Messages to Network Interfaces
Dr. Emily Carter (Network Protocol Specialist, Tech Innovations Inc.). “To effectively send messages to a network interface, one must understand the underlying protocols such as TCP/IP. Utilizing socket programming in languages like Python or C can facilitate direct communication with the network interface, allowing for precise control over data transmission.”
Mark Thompson (Senior Network Engineer, Global Connect Solutions). “When sending messages to a network interface, it is crucial to configure the interface correctly. This includes setting the appropriate IP address and ensuring that firewall settings allow for the desired traffic. Tools like Wireshark can also help in monitoring the messages being sent.”
Linda Chen (Cybersecurity Analyst, SecureNet Labs). “Security considerations are paramount when sending messages to network interfaces. Implementing encryption protocols such as TLS can protect the integrity and confidentiality of the messages. Additionally, always validate the destination to prevent unauthorized access.”
Frequently Asked Questions (FAQs)
What is a network interface?
A network interface is a point of interconnection between a computer and a network. It can be a hardware component, such as a network card, or a software interface that allows communication over a network.
How do I send messages to a network interface in a programming context?
To send messages to a network interface programmatically, you typically use socket programming. This involves creating a socket, binding it to the network interface, and then using functions like `send()` or `sendto()` to transmit data.
What protocols can be used to send messages to a network interface?
Common protocols include Transmission Control Protocol (TCP), User Datagram Protocol (UDP), and Internet Control Message Protocol (ICMP). Each protocol serves different purposes and has its own characteristics regarding reliability and data transmission.
Are there specific tools for sending messages to a network interface?
Yes, tools such as `netcat`, `ping`, and `curl` can be used to send messages or data packets to a network interface. Additionally, programming languages like Python, C, and Java offer libraries for socket communication.
What are the security considerations when sending messages to a network interface?
Security considerations include ensuring data encryption to protect against eavesdropping, implementing authentication mechanisms to verify sender identity, and using firewalls to restrict unauthorized access to the network interface.
Can I send messages to a network interface from a remote location?
Yes, you can send messages to a network interface from a remote location using the appropriate network protocols and addressing. This typically requires knowledge of the destination IP address and any necessary port configurations.
In summary, sending messages to a network interface involves a series of steps that require an understanding of networking protocols and the specific tools available for communication. The process typically begins with selecting the appropriate programming language and libraries that facilitate network communication, such as Python with its socket library or C using raw sockets. These tools allow developers to create, configure, and manage network connections effectively.
Moreover, it is essential to understand the underlying network protocols, such as TCP/IP, which govern how data is transmitted across networks. Knowledge of these protocols enables developers to structure messages correctly, ensuring they are formatted and sent in a way that the receiving interface can interpret. Additionally, proper error handling and debugging practices are crucial for troubleshooting issues that may arise during message transmission.
Finally, security considerations must not be overlooked when sending messages to a network interface. Implementing encryption and authentication mechanisms helps protect data integrity and confidentiality during transmission. By adhering to best practices in network communication, developers can ensure efficient and secure messaging between network interfaces.
Author Profile
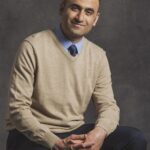
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?