How Can You Set an Environment Variable in Python?
Setting environment variables is a fundamental skill for any developer working with Python, as it allows for the configuration of applications in a flexible and secure manner. Whether you’re developing a web application, managing configurations for different environments, or simply trying to keep sensitive information like API keys out of your source code, understanding how to set and manage environment variables is crucial. In this article, we will explore the various methods to set environment variables in Python, ensuring you have the tools needed to enhance your projects and maintain best practices in your coding endeavors.
Environment variables act as a bridge between your operating system and your Python applications, enabling you to store configuration settings and sensitive data outside of your codebase. This separation not only promotes better security but also makes your applications more portable and easier to manage across different environments, such as development, testing, and production. By leveraging environment variables, you can dynamically adjust your application’s behavior based on the environment it is running in, which is essential for scalable and maintainable software development.
In the following sections, we will delve into the different approaches for setting environment variables in Python, including how to access them, modify them, and utilize them effectively in your projects. Whether you are a seasoned developer or just starting out, this guide will provide you with the knowledge and confidence to
Setting Environment Variables in Python
To set environment variables in Python, you can utilize the `os` module, which provides a portable way of using operating system-dependent functionality. The `os.environ` dictionary allows you to interact with the environment variables directly.
To set an environment variable, you can assign a value to a key in the `os.environ` dictionary as shown below:
“`python
import os
Setting an environment variable
os.environ[‘MY_VARIABLE’] = ‘my_value’
“`
This method modifies the environment variable for the current process. Any subprocesses spawned from this process will inherit the modified environment.
Accessing Environment Variables
To access the environment variables, you can use the `os.environ.get()` method, which retrieves the value associated with a specified key. If the key does not exist, it returns `None` or a default value if provided:
“`python
Accessing an environment variable
my_variable = os.environ.get(‘MY_VARIABLE’, ‘default_value’)
print(my_variable) Outputs: my_value
“`
Using `os.environ.get()` is preferred over directly accessing `os.environ[‘MY_VARIABLE’]` because it avoids a `KeyError` if the variable is not set.
Removing Environment Variables
If you need to remove an environment variable, you can use the `del` statement:
“`python
Removing an environment variable
del os.environ[‘MY_VARIABLE’]
“`
This statement will remove the specified environment variable from the current process’s environment.
Environment Variables in Different Contexts
It’s essential to understand that environment variables set in a Python script will only last for the duration of the process. Once the script exits, the changes to the environment variables will be lost. To make persistent changes, you need to set environment variables outside of Python, such as in the operating system or using a configuration file.
Common Methods to Set Environment Variables:
- Windows:
- Use the Command Prompt:
“`cmd
set MY_VARIABLE=my_value
“`
- Use PowerShell:
“`powershell
$env:MY_VARIABLE = “my_value”
“`
- Linux/MacOS:
- Use the terminal:
“`bash
export MY_VARIABLE=my_value
“`
Table of Common Functions in the os Module
Function | Description |
---|---|
os.environ | Dictionary representing the string environment. |
os.environ.get(key, default) | Get an environment variable value, with optional default. |
del os.environ[key] | Remove an environment variable. |
By leveraging these methods and understanding their scope, you can effectively manage environment variables in Python applications.
Setting Environment Variables in Python
To set environment variables in Python, the `os` module provides a straightforward interface. This module allows you to interact with the operating system and manipulate environment variables effectively.
Using os.environ
The `os.environ` dictionary allows you to access and modify the environment variables. Here’s how you can set a new environment variable or modify an existing one:
“`python
import os
Setting a new environment variable
os.environ[‘MY_VARIABLE’] = ‘my_value’
Modifying an existing environment variable
os.environ[‘MY_VARIABLE’] = ‘new_value’
“`
Once set, these environment variables will be available to the current process and any subprocesses spawned from it.
Accessing Environment Variables
You can retrieve the value of an environment variable using the `os.environ.get()` method, which is safer than directly accessing the dictionary. If the variable does not exist, it returns `None` instead of raising a KeyError.
“`python
Accessing an environment variable
value = os.environ.get(‘MY_VARIABLE’)
print(value) Output: ‘new_value’
“`
You can also provide a default value that will be returned if the environment variable does not exist:
“`python
value = os.environ.get(‘NON_EXISTENT_VARIABLE’, ‘default_value’)
print(value) Output: ‘default_value’
“`
Removing Environment Variables
To delete an environment variable, you can use the `del` statement:
“`python
Deleting an environment variable
del os.environ[‘MY_VARIABLE’]
“`
Attempting to access a deleted variable will return `None` when using the `get()` method, or raise a KeyError if accessed directly.
Persistent Environment Variables
Setting environment variables using `os.environ` is temporary and only lasts for the duration of the program’s execution. To set persistent environment variables, you must modify the system’s environment configuration files. Here are common methods for different operating systems:
Operating System | Method | Example Command |
---|---|---|
Windows | System Properties or Command Prompt | `setx MY_VARIABLE “my_value”` |
macOS/Linux | Shell configuration files (e.g., .bashrc) | `export MY_VARIABLE=”my_value”` |
After editing these files, restart your terminal or log out and back in to apply the changes.
Best Practices
- Use Descriptive Names: Environment variable names should be clear and descriptive to avoid confusion.
- Limit Scope: Only set environment variables that are necessary for the application to function.
- Document Changes: Keep records of environment variables that your application relies on, especially in collaborative environments.
By following these guidelines, you can effectively manage environment variables in your Python applications.
Expert Insights on Setting Environment Variables in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Setting environment variables in Python can be accomplished using the `os` module, specifically with `os.environ`. This allows developers to manage configuration settings effectively, ensuring that sensitive information such as API keys remains secure.”
Michael Chen (DevOps Specialist, Cloud Solutions Group). “For seamless integration in deployment pipelines, it is crucial to set environment variables correctly. Using the `dotenv` package in Python is a best practice, as it allows for loading environment variables from a `.env` file, streamlining the process across different environments.”
Sarah Patel (Python Developer Advocate, Open Source Community). “When working with environment variables in Python, it is essential to remember that they are case-sensitive. Utilizing the `os.getenv()` function not only retrieves the value but also allows for setting default values, which enhances the robustness of the application.”
Frequently Asked Questions (FAQs)
How do I set an environment variable in Python?
You can set an environment variable in Python using the `os` module. Use `os.environ[‘VARIABLE_NAME’] = ‘value’` to set the variable.
Can I set environment variables permanently in Python?
No, setting environment variables using Python’s `os.environ` only affects the current process. For permanent changes, modify your system’s environment variables through the operating system settings.
How can I access an environment variable in Python?
Access environment variables using the `os.environ` dictionary. For example, `value = os.environ.get(‘VARIABLE_NAME’)` retrieves the value of the specified variable.
What happens if I try to access a non-existent environment variable?
If you access a non-existent environment variable with `os.environ.get(‘VARIABLE_NAME’)`, it will return `None` by default. You can also specify a default value as a second argument.
Is it safe to store sensitive information in environment variables?
Storing sensitive information in environment variables is generally considered safer than hardcoding them in your code. However, ensure proper access controls and avoid exposing them in logs or error messages.
Can I set multiple environment variables at once in Python?
Yes, you can set multiple environment variables by iterating over a dictionary. For example:
“`python
for key, value in {‘VAR1’: ‘value1’, ‘VAR2’: ‘value2’}.items():
os.environ[key] = value
“`
Setting an environment variable in Python can be accomplished using the built-in `os` module, which provides a straightforward interface for interacting with the operating system. The primary method to set an environment variable is through the `os.environ` dictionary. By assigning a value to a key in this dictionary, you effectively create or modify an environment variable for the current session of the Python process.
It is important to note that changes made to environment variables using `os.environ` are temporary and only persist for the duration of the Python process. Once the process ends, any modifications to the environment variables will be lost. For permanent changes, users must modify the environment variables at the operating system level, which varies depending on the operating system being used.
Additionally, when working with environment variables, it is crucial to be aware of best practices, such as avoiding hardcoding sensitive information directly in the code. Instead, utilizing environment variables can enhance security by keeping sensitive data, like API keys and database credentials, out of the source code. This practice also promotes better configuration management across different environments, such as development, testing, and production.
In summary, setting environment variables in Python is a simple yet powerful feature that allows for dynamic configuration of applications
Author Profile
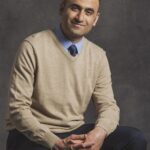
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?