How Can You Set Environment Variables in Python?
In the ever-evolving landscape of software development, environment variables play a crucial role in configuring applications and managing sensitive information. Whether you’re working on a small script or a large-scale application, understanding how to set environment variables in Python can significantly enhance your workflow and security practices. These variables serve as a bridge between your code and the external environment, allowing you to customize settings without hardcoding values directly into your scripts.
Setting environment variables in Python is not just a matter of convenience; it’s a best practice that promotes cleaner code and easier maintenance. By leveraging environment variables, developers can ensure that their applications behave consistently across different environments, such as development, testing, and production. This approach not only safeguards sensitive data, such as API keys and database credentials, but also makes it easier to manage configuration changes without altering the codebase.
In this article, we will explore the various methods for setting and accessing environment variables in Python, including built-in libraries and best practices for managing them effectively. Whether you are a seasoned developer or just starting your coding journey, mastering environment variables will empower you to create more robust and secure applications. Get ready to dive into the world of environment variables and unlock the full potential of your Python projects!
Setting Environment Variables in Python
In Python, environment variables can be managed using the built-in `os` module, which provides a portable way of using operating system-dependent functionality. To set an environment variable, you can use the `os.environ` dictionary, which allows you to access and modify the environment variables.
To set an environment variable, simply assign a value to a key in `os.environ`. Here is a basic example:
python
import os
# Set an environment variable
os.environ[‘MY_VARIABLE’] = ‘my_value’
This command sets the environment variable `MY_VARIABLE` to the value `my_value`. It is important to note that the changes made to `os.environ` only persist for the duration of the Python process. Once the script ends, the changes will be lost.
Accessing Environment Variables
To access an environment variable, you can use the `os.environ.get()` method, which returns `None` if the variable does not exist, or you can use the square bracket notation, which raises a `KeyError` if the variable is absent.
Example:
python
# Accessing an environment variable
my_variable = os.environ.get(‘MY_VARIABLE’)
print(my_variable) # Output: my_value
Alternatively, using the square bracket notation:
python
my_variable = os.environ[‘MY_VARIABLE’]
Removing Environment Variables
If you need to remove an environment variable, the `del` statement can be used in conjunction with `os.environ`. Here’s how to do it:
python
# Remove an environment variable
del os.environ[‘MY_VARIABLE’]
This command will delete `MY_VARIABLE` from the environment, making it unavailable for the rest of the script.
Common Use Cases for Environment Variables
Environment variables are frequently used for:
- Storing configuration settings (e.g., API keys, database URLs).
- Managing secrets in a secure way without hardcoding them into the source code.
- Configuring different settings for development, testing, and production environments.
Example Usage of Environment Variables
Here is an example that demonstrates how to use environment variables in a Python application:
python
import os
# Set environment variables
os.environ[‘DATABASE_URL’] = ‘postgres://user:password@localhost/dbname’
os.environ[‘SECRET_KEY’] = ‘supersecretkey’
# Accessing environment variables
database_url = os.environ.get(‘DATABASE_URL’)
secret_key = os.environ.get(‘SECRET_KEY’)
print(f”Database URL: {database_url}”)
print(f”Secret Key: {secret_key}”)
Variable | Description |
---|---|
DATABASE_URL | Connection string for the database |
SECRET_KEY | Key used for encrypting data |
By utilizing environment variables effectively, you can create flexible and secure applications that are easy to configure and maintain.
Setting Environment Variables in Python
Environment variables can be crucial for configuring applications without hardcoding sensitive information. In Python, there are various methods to set and access these variables.
Using the `os` Module
The `os` module provides a straightforward way to interact with the operating system, including manipulating environment variables. You can set environment variables using `os.environ`.
python
import os
# Setting an environment variable
os.environ[‘MY_VARIABLE’] = ‘my_value’
# Accessing an environment variable
value = os.environ.get(‘MY_VARIABLE’)
print(value) # Output: my_value
- Setting a Variable: Use `os.environ[‘VARIABLE_NAME’] = ‘value’`.
- Getting a Variable: Use `os.environ.get(‘VARIABLE_NAME’)` to safely retrieve a value, returning `None` if not set.
Using `dotenv` for Environment Variables Management
For projects requiring multiple environment variables, using the `python-dotenv` package can simplify management. This allows you to store variables in a `.env` file.
- Install the package:
bash
pip install python-dotenv
- Create a `.env` file:
MY_VARIABLE=my_value
ANOTHER_VARIABLE=another_value
- Load the variables in your script:
python
from dotenv import load_dotenv
import os
load_dotenv() # Load environment variables from .env file
my_variable = os.getenv(‘MY_VARIABLE’)
print(my_variable) # Output: my_value
Setting Environment Variables Temporarily
You may need to set environment variables temporarily for a session. This can be done using the command line before running your Python script.
- Linux/Mac:
bash
export MY_VARIABLE=my_value
python my_script.py
- Windows:
cmd
set MY_VARIABLE=my_value
python my_script.py
In this approach, the environment variable is available only during the script’s execution.
Environment Variables in Configuration Files
Another method is to use configuration management libraries like `configparser` for reading variables from configuration files, allowing more complex setups.
- Create a configuration file (e.g., `config.ini`):
ini
[DEFAULT]
MyVariable = my_value
- Load the configuration in your Python script:
python
import configparser
config = configparser.ConfigParser()
config.read(‘config.ini’)
my_variable = config[‘DEFAULT’][‘MyVariable’]
print(my_variable) # Output: my_value
Best Practices
- Do Not Hardcode Sensitive Information: Always prefer environment variables for sensitive data like API keys.
- Use a `.env` File for Local Development: This keeps local environment settings separate from your codebase.
- Document Required Variables: Maintain clear documentation for variables needed for your application to ensure proper setup.
- Fallback Values: Implement fallback values using `os.getenv(‘VARIABLE_NAME’, ‘default_value’)` to ensure your application runs smoothly even if some variables are missing.
By employing these techniques, you can effectively manage environment variables in your Python applications, enhancing security and configurability.
Expert Insights on Setting Environment Variables in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Setting environment variables in Python is crucial for managing configuration settings securely. Utilizing the `os` module, developers can easily access and modify environment variables, ensuring that sensitive information remains out of the source code.”
Michael Chen (Lead DevOps Consultant, Cloud Solutions Group). “For effective deployment, it is essential to set environment variables correctly. Using `.env` files in conjunction with the `python-dotenv` package allows for a seamless way to manage these variables across different environments without hardcoding them.”
Sarah Thompson (Python Developer Advocate, Open Source Community). “Understanding how to set environment variables in Python can significantly enhance the security and flexibility of your applications. Leveraging tools like `os.environ` not only allows for dynamic configuration but also promotes best practices in application development.”
Frequently Asked Questions (FAQs)
How can I set environment variables in Python?
You can set environment variables in Python using the `os` module. Use `os.environ[‘VARIABLE_NAME’] = ‘value’` to set a variable, which will be available for the duration of the program.
Are environment variables persistent after the Python script ends?
No, environment variables set within a Python script are not persistent. They exist only for the duration of the process and will not affect the system or user environment after the script terminates.
How can I access environment variables in Python?
You can access environment variables using the `os` module as well. Use `os.getenv(‘VARIABLE_NAME’)` to retrieve the value of an environment variable, or `os.environ[‘VARIABLE_NAME’]` for a direct access method.
Can I set multiple environment variables at once in Python?
Yes, you can set multiple environment variables by iterating over a dictionary of key-value pairs. For example:
python
import os
env_vars = {‘VAR1’: ‘value1’, ‘VAR2’: ‘value2’}
for key, value in env_vars.items():
os.environ[key] = value
Is there a way to set environment variables permanently in Python?
Python itself does not provide a built-in way to set environment variables permanently. To set them permanently, you need to modify system configuration files or use shell commands outside of Python.
How can I remove an environment variable in Python?
You can remove an environment variable using the `os` module with the `del` statement. For example, `del os.environ[‘VARIABLE_NAME’]` will remove the specified environment variable from the current process.
Setting environment variables in Python is a crucial practice for managing configuration settings and sensitive information, such as API keys and database credentials. This can be accomplished using the built-in `os` module, which allows developers to access and modify environment variables seamlessly. By utilizing functions like `os.environ` to read and set variables, developers can ensure that their applications remain flexible and secure across different environments, such as development, testing, and production.
One effective method for managing environment variables is through the use of a `.env` file in conjunction with the `python-dotenv` package. This approach not only simplifies the process of loading environment variables but also enhances the organization of configuration settings. By storing sensitive information in a separate file, developers can avoid hardcoding values directly into their source code, thereby reducing the risk of exposing sensitive data in version control systems.
understanding how to set and manage environment variables in Python is essential for building secure and maintainable applications. By leveraging the capabilities of the `os` module and external libraries like `python-dotenv`, developers can create a robust environment configuration strategy. This practice not only promotes best security practices but also facilitates easier collaboration and deployment across various stages of the software development lifecycle.
Author Profile
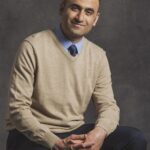
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?