How Can You Set the Working Directory in Python?
When diving into the world of Python programming, one of the fundamental skills every developer should master is the ability to effectively manage their working environment. Among the many tasks that come with writing code, setting the working directory is a crucial step that can streamline your workflow and enhance your productivity. Whether you’re analyzing data, building applications, or automating tasks, understanding how to set your working directory ensures that your scripts can locate the files they need, making your coding experience smoother and more efficient.
The working directory in Python serves as the default folder where your scripts look for files to read from or write to. By default, this directory is often set to the location of the script being executed, but as projects grow in complexity, you may find the need to change this setting. Adjusting your working directory can help you organize your files better and avoid confusion, especially when dealing with multiple datasets or project files scattered across various locations.
In this article, we will explore the various methods to set and change the working directory in Python. From using built-in libraries to leveraging integrated development environments (IDEs), we’ll provide you with the tools and knowledge necessary to customize your working environment effectively. By the end, you’ll be equipped to navigate your projects with confidence, ensuring that your Python scripts run
Setting the Working Directory in Python
In Python, the working directory is the folder where Python looks for files to open or save. Setting the working directory appropriately is crucial for file management and ensuring that scripts run smoothly without errors related to file paths. There are several ways to set or change the working directory in Python.
Using the os Module
The `os` module provides a simple way to change the current working directory. The `os.chdir()` function can be used to set the working directory to a specified path. Here’s how to do it:
“`python
import os
Set the working directory to the specified path
os.chdir(‘/path/to/your/directory’)
“`
To confirm that the working directory has been changed, you can use `os.getcwd()` to print the current working directory:
“`python
print(os.getcwd())
“`
Using the pathlib Module
The `pathlib` module, introduced in Python 3.4, offers an object-oriented approach to handle filesystem paths. You can set the working directory using `pathlib.Path`:
“`python
from pathlib import Path
Set the working directory
path = Path(‘/path/to/your/directory’)
Path.cwd() Get the current working directory
“`
This method provides a more intuitive way to work with paths and directories.
Setting the Working Directory in Jupyter Notebooks
In a Jupyter Notebook, you can set the working directory using a magic command or by using the `os` module. To use a magic command, simply type:
“`python
%cd /path/to/your/directory
“`
Alternatively, the `os` module can be used as previously described.
Common Use Cases
When working with files, the following scenarios often require setting the working directory:
- Loading datasets: When importing data files, setting the working directory to the folder containing the files can simplify file paths.
- Saving outputs: Ensuring that all outputs are saved in a specific folder can help in organizing results.
- Running scripts: If scripts are executed from different directories, setting the working directory can prevent file-not-found errors.
Example Table of Common Commands
Command | Description |
---|---|
os.chdir(path) | Changes the current working directory to the specified path. |
os.getcwd() | Returns the current working directory as a string. |
Path.cwd() | Returns the current working directory using pathlib. |
%cd path | Changes the current directory in a Jupyter Notebook. |
Setting the working directory is a fundamental skill in Python that enhances your ability to manage file operations effectively. By utilizing the appropriate modules and commands, you can streamline your data handling processes.
Setting the Working Directory in Python
To set the working directory in Python, you primarily utilize the `os` module, which provides a portable way of using operating system-dependent functionality, including changing the current working directory.
Using the `os` Module
To change the working directory using the `os` module, follow these steps:
- Import the `os` Module:
“`python
import os
“`
- Set the Working Directory:
Use the `os.chdir()` function to change the working directory to your desired path.
“`python
os.chdir(‘/path/to/your/directory’)
“`
- Verify the Current Working Directory:
You can confirm that the working directory has been changed by using `os.getcwd()`.
“`python
print(os.getcwd())
“`
Examples
- Example of Changing Directory:
“`python
import os
Change to the desired directory
os.chdir(‘/Users/username/Documents’)
Verify the change
print(“Current Working Directory:”, os.getcwd())
“`
- Handling Errors:
It is advisable to handle potential errors when changing directories, especially if the specified path does not exist:
“`python
try:
os.chdir(‘/path/to/nonexistent/directory’)
except FileNotFoundError:
print(“Directory not found. Please check the path.”)
“`
Using `pathlib` for Path Management
An alternative to the `os` module is the `pathlib` module, which provides an object-oriented approach to handling filesystem paths.
- Import the `Path` Class:
“`python
from pathlib import Path
“`
- Set the Working Directory:
“`python
Path(‘/path/to/your/directory’).resolve()
“`
- Changing the Current Directory:
“`python
import os
from pathlib import Path
new_dir = Path(‘/Users/username/Documents’)
os.chdir(new_dir)
print(“Current Working Directory:”, os.getcwd())
“`
Common Use Cases
- Data Analysis: Setting the working directory to the folder where data files are stored.
- Project Organization: Keeping scripts organized in a specific directory structure.
- File Management: Easily managing output files by directing them to a specific folder.
Best Practices
- Always verify the current directory after changing it.
- Use absolute paths to avoid confusion with relative paths.
- Implement error handling to manage exceptions when working with file paths.
- When working in Jupyter Notebooks, you can also set the working directory at the start of your notebook for consistency.
Using these methods, you can effectively manage the working directory in Python, facilitating better organization and access to your project files.
Expert Insights on Setting the Working Directory in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Setting the working directory in Python is crucial for managing file paths effectively. Utilizing the `os` module with `os.chdir()` allows users to define the directory from which they want to operate, ensuring that all file operations are executed in the intended context.”
Michael Thompson (Software Engineer, CodeMasters). “For beginners, it’s essential to understand that the working directory is the default location where Python looks for files. Using `os.getcwd()` can help verify the current directory, while `os.chdir(‘/path/to/directory’)` can change it dynamically, which is particularly useful in project environments.”
Lisa Tran (Python Educator, LearnPython.org). “When teaching Python, I emphasize the importance of the working directory in file management. I recommend using Jupyter Notebooks, as they allow users to set the working directory directly in the interface, simplifying the process for those new to programming.”
Frequently Asked Questions (FAQs)
How do I set the working directory in Python?
You can set the working directory in Python using the `os` module. Use `os.chdir(‘/path/to/directory’)` to change the current working directory to the specified path.
How can I check the current working directory in Python?
To check the current working directory, you can use `os.getcwd()`. This function returns the path of the current working directory.
Can I set the working directory in Jupyter Notebook?
Yes, in Jupyter Notebook, you can set the working directory using the same `os` module. Simply run `import os` followed by `os.chdir(‘/path/to/directory’)` in a cell.
What happens if I set the working directory to a non-existent path?
If you attempt to set the working directory to a non-existent path, Python will raise a `FileNotFoundError`, indicating that the specified directory does not exist.
Is it possible to set the working directory permanently in Python?
Python does not provide a built-in method to set the working directory permanently. However, you can create a startup script or modify your environment variables to achieve a similar effect.
Are there any alternatives to using the `os` module for setting the working directory?
Yes, you can also use the `pathlib` module. With `pathlib.Path().resolve()`, you can change the working directory by combining it with `os.chdir()`, although `os` remains the primary method for this task.
Setting the working directory in Python is a fundamental task that allows users to define the location where their scripts will read from and write to files. This is particularly important when dealing with data files, as it ensures that the code can access the necessary resources without requiring absolute paths. The common method to set the working directory is by using the `os` module, specifically the `os.chdir()` function, which changes the current working directory to the specified path.
Additionally, it is essential to be aware of the current working directory, which can be checked using `os.getcwd()`. This function returns the path of the directory that Python is currently using, providing a convenient way to verify that the working directory has been set correctly. Understanding how to navigate and manipulate working directories is crucial for efficient file management and organization within Python projects.
In summary, mastering the process of setting and checking the working directory in Python enhances the user’s ability to manage files effectively. Utilizing the `os` module streamlines this process, allowing for greater flexibility and efficiency in coding. By establishing a clear working directory, users can avoid common pitfalls associated with file path errors, thereby improving the overall robustness of their Python applications.
Author Profile
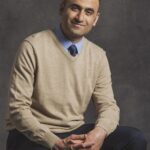
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?