How Can You Skip Lines in Python: A Step-by-Step Guide?
In the world of programming, the ability to manipulate text and data is a fundamental skill that can unlock a myriad of possibilities. Python, renowned for its simplicity and versatility, offers a range of techniques for handling strings and files. One common task that many developers encounter is the need to skip lines in their code—whether it’s to streamline data processing, enhance readability, or manage input files efficiently. Understanding how to skip lines effectively can save time and improve the overall performance of your Python scripts.
When working with files, especially large datasets or logs, you may find that not all lines are relevant to your analysis. Python provides several methods to selectively skip lines, allowing you to focus on the data that matters most. From reading files line by line to using built-in functions that facilitate this process, the language’s capabilities make it easy to tailor your approach based on the specific requirements of your project.
Additionally, skipping lines isn’t just limited to file handling; it can also be applied in various contexts within your code, such as when iterating through lists or processing user input. By mastering these techniques, you can enhance your coding efficiency and create more robust applications. In the following sections, we will delve deeper into the various methods available in Python for skipping lines, providing you with the tools
Using Newline Characters
In Python, skipping lines can be accomplished using newline characters. The most common newline character is `\n`, which represents a line break in text. When writing to a file or printing to the console, you can include this character to create space between lines.
For example:
“`python
print(“Hello, World!\n”)
print(“This is a new line.”)
“`
This code will output:
“`
Hello, World!
This is a new line.
“`
The `\n` character effectively skips a line between the two print statements.
Controlling Output in Loops
When processing data in loops, you may want to skip lines based on specific conditions. This can be achieved using the `continue` statement, which allows you to skip the current iteration and move to the next one.
Example:
“`python
for i in range(10):
if i % 2 == 0:
continue
print(i)
“`
This loop prints only odd numbers from 0 to 9, effectively skipping the even numbers.
Writing to Files with Line Skips
When writing to files, you can also control line spacing by including newline characters. Here’s how to write data to a file while skipping lines:
“`python
with open(‘output.txt’, ‘w’) as f:
f.write(“First Line\n\n”) Skips one line after the first line
f.write(“Second Line\n”)
f.write(“Third Line\n\n”) Skips one line after the third line
f.write(“Fourth Line”)
“`
The content of `output.txt` will appear as follows:
“`
First Line
Second Line
Third Line
Fourth Line
“`
Using the `print` Function’s `end` Parameter
The `print()` function in Python has an `end` parameter that determines what is printed at the end of the output. By default, it adds a newline character, but you can customize this behavior to include multiple newlines.
Example:
“`python
print(“Line 1″, end=”\n\n”) Skips one line
print(“Line 2″, end=”\n\n”) Skips one line
print(“Line 3”)
“`
This will output:
“`
Line 1
Line 2
Line 3
“`
Table: Line Skipping Methods
Method | Description |
---|---|
Newline Character (`\n`) | Directly adds a line break in strings. |
Continue Statement | Skips iterations in loops based on conditions. |
Writing to Files | Includes newline characters to control line spacing in files. |
Print Function `end` | Customizes the output ending for line spacing. |
Using Newline Characters
In Python, skipping lines can be achieved by utilizing newline characters. The newline character `\n` can be included in strings to indicate where a line break should occur.
“`python
print(“First Line\nSecond Line\nThird Line”)
“`
The output will be:
“`
First Line
Second Line
Third Line
“`
To create blank lines, you can include multiple newline characters in succession:
“`python
print(“First Line\n\nSecond Line”)
“`
This will result in:
“`
First Line
Second Line
“`
Reading Files with Skipped Lines
When reading files, you may want to skip certain lines, such as headers or blank lines. This can be done using a loop with conditional checks.
“`python
with open(‘example.txt’, ‘r’) as file:
for line in file:
if line.strip(): Skip blank lines
print(line.strip())
“`
This code reads a file line by line and prints only non-blank lines.
Utilizing the `enumerate` Function
The `enumerate` function can be very useful for skipping lines based on their index. For instance, if you want to skip every second line in a file:
“`python
with open(‘example.txt’, ‘r’) as file:
for index, line in enumerate(file):
if index % 2 == 0: Process only even-indexed lines
print(line.strip())
“`
Here, only lines with even indices (0, 2, 4, …) are printed.
Using List Comprehensions
List comprehensions can also be employed for skipping lines efficiently. If you want to filter out specific lines while reading from a list of strings:
“`python
lines = [“First Line”, “”, “Second Line”, “Third Line”, “”, “Fourth Line”]
non_empty_lines = [line for line in lines if line] Skip empty lines
for line in non_empty_lines:
print(line)
“`
This will output:
“`
First Line
Second Line
Third Line
Fourth Line
“`
Regular Expressions for Advanced Skipping
For more complex line skipping, regular expressions can be used. The `re` module allows for advanced pattern matching.
“`python
import re
with open(‘example.txt’, ‘r’) as file:
for line in file:
if not re.match(r’^\s*$’, line): Skip empty or whitespace-only lines
print(line.strip())
“`
This code will skip any line that consists solely of whitespace.
Using `continue` Statement
The `continue` statement can be employed within loops to skip to the next iteration based on specific conditions.
“`python
with open(‘example.txt’, ‘r’) as file:
for line in file:
if not line.strip(): Skip blank lines
continue
print(line.strip())
“`
This approach is straightforward and maintains readability while effectively skipping undesired lines.
Summary of Methods
Method | Description |
---|---|
Newline Characters | Insert `\n` for line breaks. |
File Reading with Conditions | Skip lines using loops and conditions. |
Enumerate | Use index to selectively process lines. |
List Comprehensions | Filter lines using concise expressions. |
Regular Expressions | Match patterns to skip lines. |
Continue Statement | Skip specific lines in loops. |
Each of these methods serves distinct use cases, allowing flexibility in how lines are managed within Python code.
Expert Insights on Skipping Lines in Python
Dr. Emily Carter (Senior Python Developer, CodeCraft Solutions). “To effectively skip lines in Python, one can utilize the `continue` statement within a loop. This allows for the bypassing of specific iterations based on certain conditions, thereby skipping lines of data as needed.”
Michael Chen (Data Scientist, Analytics Innovations). “When processing text files in Python, using the `readlines()` method in combination with list comprehensions can be an efficient way to skip lines. This approach enables you to filter out unwanted lines based on their index or content.”
Sarah Thompson (Software Engineer, Tech Solutions Inc.). “For skipping lines while reading files, the `for` loop can be paired with conditional checks. By leveraging the `enumerate()` function, developers can easily skip specific lines based on their line number, enhancing code readability and efficiency.”
Frequently Asked Questions (FAQs)
How can I skip lines when reading a file in Python?
You can skip lines when reading a file by using a loop with a conditional statement. For example, using `next(file)` can skip the first line, or you can use a counter to skip multiple lines.
What is the purpose of the `continue` statement in a loop?
The `continue` statement is used to skip the current iteration of a loop and proceed to the next iteration. This is useful when you want to ignore certain conditions while processing data.
Can I skip lines in a list in Python?
Yes, you can skip lines in a list by using list comprehensions or loops with conditional statements. For instance, you can create a new list that excludes specific indices or values.
How do I skip blank lines in a text file while reading it?
To skip blank lines, you can check if a line is empty before processing it. For example, you can use an `if line.strip():` condition to ignore lines that are blank or contain only whitespace.
Is there a built-in function to skip lines in Python?
Python does not have a specific built-in function solely for skipping lines. However, you can achieve this using file methods like `readline()` in combination with control structures to manage which lines to skip.
What is the best practice for skipping lines in CSV files with Python?
The best practice for skipping lines in CSV files is to use the `csv` module and specify the `skiprows` parameter when using `pandas.read_csv()`, or manually skip lines using a loop before processing the data.
In Python, skipping lines in various contexts can be achieved through several methods, depending on the specific requirement. For instance, when reading files, you can use the `readline()` method within a loop to skip specific lines or utilize list comprehensions to filter out unwanted lines. This allows for efficient processing of large files by avoiding unnecessary data. Additionally, the `enumerate()` function can be paired with conditional statements to selectively process lines based on their index.
When working with strings, the `splitlines()` method can be utilized to break a string into a list of lines, which can then be manipulated to skip certain entries. Furthermore, when printing output, the `print()` function can be used with newline characters to create gaps between lines, effectively skipping lines visually. These techniques highlight the flexibility of Python in handling line management across different data types.
Ultimately, understanding how to skip lines in Python enhances the efficiency and clarity of code. By employing the right methods, developers can streamline their data processing tasks and improve overall performance. Mastery of these techniques is essential for anyone looking to work with files or string data in Python, as it allows for more control over the flow of information.
Author Profile
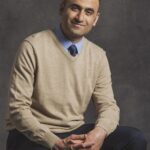
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?