How Can You Implement Sleep Functionality in JavaScript?
### Introduction
In the fast-paced world of web development, where every millisecond counts, managing asynchronous operations effectively is crucial. One common challenge developers face is the need to pause execution temporarily—whether for waiting on a response from an API, throttling requests, or simply creating a delay in a loop. Enter the concept of “sleeping” in JavaScript. While JavaScript is single-threaded and doesn’t have a built-in sleep function like some other programming languages, there are clever techniques and patterns that can help you achieve similar results. In this article, we’ll explore how to implement sleep functionality in JavaScript, allowing you to control the flow of your code with precision.
When you think of sleeping in programming, you might picture a straightforward command that halts execution for a specified time. However, in JavaScript, the approach is a bit more nuanced due to its event-driven nature. Instead of blocking the main thread, which could freeze your application, JavaScript utilizes asynchronous programming paradigms. This means that while you can create delays, they must be handled in a way that keeps your application responsive and efficient.
In the following sections, we will delve into various methods to implement sleep functionality in JavaScript, including the use of promises, async/await syntax, and even
Understanding the Event Loop
JavaScript is single-threaded, meaning it can only execute one piece of code at a time. The event loop is a fundamental concept that allows JavaScript to perform non-blocking operations. When you want to pause execution in JavaScript, you often rely on asynchronous behavior facilitated by the event loop. Understanding how the event loop interacts with asynchronous calls is crucial to implementing any form of “sleep” functionality.
Implementing Sleep with Promises
One common way to implement a sleep function in JavaScript is by using Promises along with the `setTimeout` function. This method provides a clean and readable way to pause execution without blocking the main thread. Below is an example of how to create a sleep function using this approach:
javascript
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
// Usage
async function demo() {
console.log(‘Wait for 2 seconds…’);
await sleep(2000);
console.log(‘2 seconds later!’);
}
demo();
In this example, the `sleep` function takes a number of milliseconds as an argument and returns a Promise that resolves after the specified time using `setTimeout`.
Using Async/Await
The `async/await` syntax in JavaScript allows you to write asynchronous code that looks synchronous. When used with the sleep function defined above, it provides a straightforward way to manage delays in your code.
- Async Functions: Mark a function as `async` to enable the use of `await` within it.
- Awaiting Promises: Use `await` before a Promise to pause execution until the Promise resolves.
Here’s a concise example:
javascript
async function example() {
console.log(‘Start’);
await sleep(1000); // Wait for 1 second
console.log(‘End’);
}
example();
This structure enhances readability and maintains a clear flow of logic.
Comparison of Sleep Implementations
Below is a comparison table that outlines different methods of implementing sleep functionality in JavaScript:
Method | Blocking | Use Case |
---|---|---|
setTimeout | No | Pausing execution without blocking |
Promises | No | Handling asynchronous operations |
setInterval | No | Repeated execution after intervals |
Busy wait (not recommended) | Yes | Not recommended due to performance issues |
Sleep Mechanisms
In summary, while JavaScript does not have a built-in sleep function like some other languages, you can effectively implement sleep behavior using Promises and the event loop. This allows for a non-blocking, efficient way to handle delays in execution. Understanding these concepts will enhance your ability to write asynchronous JavaScript code effectively.
Understanding Sleep in JavaScript
JavaScript, being a single-threaded language, doesn’t natively support a sleep function like some other programming languages. However, developers often need to pause execution for various reasons, such as waiting for asynchronous operations to complete or creating timed delays. Here are several methods to achieve similar functionality:
Using setTimeout
The `setTimeout` function can be employed to delay the execution of a function. It does not block the main thread, allowing other code to run during the wait time.
javascript
console.log(“Start”);
setTimeout(() => {
console.log(“Executed after 2 seconds”);
}, 2000);
console.log(“End”);
- Parameters:
- The first parameter is the function to execute after the delay.
- The second parameter is the delay in milliseconds.
Using Promises with setTimeout
To create a more synchronous-like behavior, you can wrap `setTimeout` in a Promise. This allows you to use `async/await` syntax for cleaner and more readable code.
javascript
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function demo() {
console.log(“Start”);
await sleep(2000);
console.log(“Executed after 2 seconds”);
console.log(“End”);
}
demo();
- Advantages:
- Improves code readability.
- Maintains asynchronous behavior without blocking the main thread.
Using a Busy-Wait Loop (Not Recommended)
Though it’s possible to create a sleep function using a busy-wait loop, it is highly discouraged due to its inefficiency. This method consumes CPU cycles unnecessarily.
javascript
function sleep(milliseconds) {
const end = Date.now() + milliseconds;
while (Date.now() < end) {
// Busy-wait loop
}
}
- Downsides:
- Blocks the main thread.
- Decreases performance and responsiveness of the application.
Using Generators for Sleep Functionality
JavaScript generators can also be utilized to pause execution. By yielding control back to the caller, you can create a sleep-like effect.
javascript
function* sleepGenerator(ms) {
yield new Promise(resolve => setTimeout(resolve, ms));
}
async function run() {
console.log(“Start”);
await sleepGenerator(2000).next().value;
console.log(“Executed after 2 seconds”);
console.log(“End”);
}
run();
- Characteristics:
- Generates a promise that resolves after the specified time.
- Allows for more complex control flow in asynchronous code.
Choosing the Right Method
Method | Blocking | Readability | Recommended Usage |
---|---|---|---|
setTimeout | No | Moderate | Simple delays, UI updates |
Promises with setTimeout | No | High | Asynchronous workflows, cleaner code |
Busy-Wait Loop | Yes | Low | Avoid unless absolutely necessary |
Generators | No | High | Complex control flow in async code |
Selecting the appropriate method depends on the specific requirements of your application. For most cases, utilizing Promises with `setTimeout` provides an optimal balance between performance and code clarity.
Expert Insights on Implementing Sleep in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “In JavaScript, the concept of ‘sleep’ is often misunderstood. While JavaScript does not have a built-in sleep function like some other languages, developers can achieve similar functionality using asynchronous programming techniques, particularly with Promises and async/await syntax.”
Michael Thompson (JavaScript Framework Specialist, CodeMaster Academy). “To effectively implement a sleep-like behavior in JavaScript, one can utilize the setTimeout function. This allows for the execution of code after a specified delay, which is particularly useful in scenarios where you want to pause execution without blocking the main thread.”
Linda Xu (Lead Frontend Developer, Modern Web Solutions). “Understanding the event loop is crucial when simulating sleep in JavaScript. By leveraging async/await in conjunction with a Promise that resolves after a timeout, developers can create a more readable and maintainable code structure that mimics sleep functionality without freezing the UI.”
Frequently Asked Questions (FAQs)
How can I implement a sleep function in JavaScript?
You can create a sleep function using `Promise` and `setTimeout`. For example:
javascript
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
Is there a built-in sleep function in JavaScript?
No, JavaScript does not have a built-in sleep function. However, you can simulate it using asynchronous programming with Promises and `setTimeout`.
Can I use async/await with a sleep function?
Yes, you can use async/await syntax to pause execution in an asynchronous function. For example:
javascript
async function demo() {
console.log(‘Start’);
await sleep(2000);
console.log(‘End’);
}
What is the purpose of using sleep in JavaScript?
The sleep function is often used to delay execution, manage timing in animations, or wait for asynchronous operations to complete before proceeding.
Are there any performance concerns with using sleep in JavaScript?
Using sleep can lead to performance issues if overused, as it blocks the execution of code and can make your application unresponsive. Use it judiciously in asynchronous contexts.
Can I create a sleep function without Promises?
Yes, you can create a sleep function using traditional callback methods, but this approach is less elegant and can lead to callback hell. Using Promises is recommended for cleaner code.
In JavaScript, the concept of “sleeping” or pausing execution is not natively supported in the same way it is in some other programming languages. Instead, developers often use asynchronous programming techniques, such as Promises and the `setTimeout` function, to create a delay in code execution. This approach allows for non-blocking behavior, which is essential in environments like web browsers where maintaining a responsive user interface is crucial.
One common method to implement a sleep-like functionality is by using `setTimeout`, which schedules a function to be executed after a specified delay. However, this does not pause the execution of the entire script; rather, it allows the rest of the code to run while waiting for the timeout to complete. For more modern JavaScript, the introduction of async/await syntax allows developers to write cleaner, more readable code that mimics synchronous behavior while still being asynchronous.
Additionally, libraries such as `sleep-promise` can be utilized to simplify the implementation of sleep functionality. These libraries provide a straightforward way to create pauses in code execution without the complexity of managing callbacks or promises manually. Understanding these techniques is essential for developers looking to control the flow of their JavaScript applications effectively.
In
Author Profile
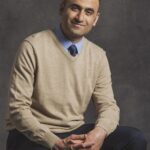
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?