How Can You Efficiently Sort a List of Tuples in Python?
Sorting data is a fundamental operation in programming, and Python provides powerful tools to handle various data structures with ease. Among these structures, tuples stand out for their simplicity and immutability. Whether you’re dealing with a list of coordinates, records, or any collection of paired values, knowing how to sort a list of tuples can significantly enhance your data manipulation capabilities. In this article, we’ll explore the nuances of sorting tuples in Python, equipping you with the skills to organize your data effectively.
At its core, sorting a list of tuples involves determining the order in which the tuples should be arranged based on their elements. Python’s built-in sorting functions allow for flexibility, enabling you to sort by any element within the tuples, whether it’s the first, second, or even a combination of both. This versatility is particularly useful in scenarios where your tuples represent complex data structures, such as records with multiple fields.
Moreover, understanding how to sort tuples can open doors to more advanced data analysis and processing techniques. With the right sorting strategies, you can quickly retrieve the information you need, enhance the readability of your data outputs, and streamline your coding processes. As we delve deeper into the methods and best practices for sorting tuples in Python, you’ll discover how to leverage these tools to elevate your programming
Sorting a List of Tuples
To sort a list of tuples in Python, you can utilize the built-in `sorted()` function or the `.sort()` method available for lists. Both methods allow you to specify sorting criteria, enabling sorting based on the first item, the second item, or any other element within the tuples.
Using the sorted() Function
The `sorted()` function returns a new sorted list from the specified iterable. This function is versatile and can take two key parameters: `key` and `reverse`. The `key` parameter allows you to define a custom sorting order, while `reverse` determines whether to sort in ascending or descending order.
Here’s an example of sorting a list of tuples based on the first element:
“`python
data = [(3, ‘three’), (1, ‘one’), (2, ‘two’)]
sorted_data = sorted(data)
“`
If you want to sort by the second element of each tuple, you can use a lambda function:
“`python
sorted_data_by_second = sorted(data, key=lambda x: x[1])
“`
Using the .sort() Method
The `.sort()` method sorts the list in place and modifies the original list. It also accepts the same `key` and `reverse` parameters. Here is how you can use it:
“`python
data = [(3, ‘three’), (1, ‘one’), (2, ‘two’)]
data.sort()
“`
To sort by the second element using `.sort()`:
“`python
data.sort(key=lambda x: x[1])
“`
Sorting in Descending Order
To sort the tuples in descending order, simply set the `reverse` parameter to `True`. For example:
“`python
sorted_data_descending = sorted(data, reverse=True)
data.sort(key=lambda x: x[1], reverse=True)
“`
Sorting by Multiple Criteria
When dealing with tuples, you may want to sort by multiple criteria. This can be accomplished by passing a tuple to the `key` parameter. For instance, if you have a list of tuples representing (age, name) and want to sort primarily by age and secondarily by name:
“`python
data = [(25, ‘Alice’), (30, ‘Bob’), (25, ‘Charlie’)]
sorted_data = sorted(data, key=lambda x: (x[0], x[1]))
“`
Example Table of Sorting
Here’s a table illustrating how the different sorting methods affect a list of tuples:
Original List | Sorted by First Element | Sorted by Second Element | Sorted by Age then Name |
---|---|---|---|
[(3, ‘three’), (1, ‘one’), (2, ‘two’)] | [(1, ‘one’), (2, ‘two’), (3, ‘three’)] | [(1, ‘one’), (3, ‘three’), (2, ‘two’)] | [(25, ‘Alice’), (25, ‘Charlie’), (30, ‘Bob’)] |
In summary, sorting a list of tuples in Python is a straightforward process that can be achieved using either the `sorted()` function or the `.sort()` method, with options for custom criteria and ordering.
Sorting a List of Tuples
Sorting a list of tuples in Python can be accomplished using the built-in `sorted()` function or the `.sort()` method. Tuples can be sorted by their elements, and you can specify which element to sort by using a key function.
Using the `sorted()` Function
The `sorted()` function returns a new sorted list from the items in an iterable. This function does not modify the original list.
- Syntax:
“`python
sorted(iterable, key=None, reverse=)
“`
- Parameters:
- `iterable`: The collection (e.g., list of tuples) to sort.
- `key`: A function that serves as a key for the sort comparison.
- `reverse`: A boolean value that, when set to `True`, sorts the list in descending order.
Example:
“`python
data = [(3, ‘c’), (1, ‘a’), (2, ‘b’)]
sorted_data = sorted(data)
print(sorted_data) Output: [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
To sort by the second element of each tuple:
“`python
sorted_by_second = sorted(data, key=lambda x: x[1])
print(sorted_by_second) Output: [(1, ‘a’), (3, ‘c’), (2, ‘b’)]
“`
Using the `.sort()` Method
The `.sort()` method sorts the list in place and returns `None`. This method modifies the original list.
- Syntax:
“`python
list.sort(key=None, reverse=)
“`
Example:
“`python
data = [(3, ‘c’), (1, ‘a’), (2, ‘b’)]
data.sort()
print(data) Output: [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
Sorting by the second element in place:
“`python
data.sort(key=lambda x: x[1])
print(data) Output: [(1, ‘a’), (3, ‘c’), (2, ‘b’)]
“`
Sorting by Multiple Criteria
To sort tuples by multiple criteria, you can return a tuple from the key function. This allows for sorting primarily by one element and secondarily by another.
Example:
“`python
data = [(3, ‘c’), (1, ‘a’), (2, ‘b’), (1, ‘b’)]
sorted_multi = sorted(data, key=lambda x: (x[0], x[1]))
print(sorted_multi) Output: [(1, ‘a’), (1, ‘b’), (2, ‘b’), (3, ‘c’)]
“`
Here, the list is sorted first by the first element and then by the second element in case of ties.
Sorting in Descending Order
To sort a list of tuples in descending order, set the `reverse` parameter to `True`.
Example:
“`python
data = [(3, ‘c’), (1, ‘a’), (2, ‘b’)]
sorted_desc = sorted(data, reverse=True)
print(sorted_desc) Output: [(3, ‘c’), (2, ‘b’), (1, ‘a’)]
“`
For descending order by the first element:
“`python
sorted_desc_by_first = sorted(data, key=lambda x: x[0], reverse=True)
print(sorted_desc_by_first) Output: [(3, ‘c’), (2, ‘b’), (1, ‘a’)]
“`
Sorting a list of tuples in Python is straightforward using either the `sorted()` function or the `.sort()` method, with the flexibility to sort by various criteria and in different orders.
Expert Insights on Sorting Lists of Tuples in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Sorting a list of tuples in Python can be efficiently achieved using the built-in `sorted()` function, which allows for custom sorting criteria through the `key` parameter. This flexibility is essential when dealing with complex data structures.”
Michael Thompson (Python Developer, CodeCraft Solutions). “Utilizing the `itemgetter` from the `operator` module can significantly enhance the readability and performance of your sorting operations. This method is particularly useful when you need to sort by multiple tuple elements.”
Linda Chen (Software Engineer, Data Systems Corp.). “When sorting tuples, it is crucial to consider the data types involved. For instance, sorting tuples containing mixed data types may yield unexpected results, so it is advisable to ensure uniformity in the tuple contents before sorting.”
Frequently Asked Questions (FAQs)
How do I sort a list of tuples by the first element?
You can sort a list of tuples by the first element using the `sorted()` function with no additional parameters, as tuples are compared lexicographically by default. For example: `sorted_list = sorted(my_list_of_tuples)`.
Can I sort a list of tuples by a specific index?
Yes, you can specify the index by using a lambda function as the `key` parameter in the `sorted()` function. For example: `sorted_list = sorted(my_list_of_tuples, key=lambda x: x[1])` sorts by the second element.
What if I want to sort in descending order?
To sort in descending order, you can set the `reverse` parameter to `True`. For instance: `sorted_list = sorted(my_list_of_tuples, key=lambda x: x[1], reverse=True)` sorts by the second element in descending order.
Is there a way to sort a list of tuples by multiple criteria?
Yes, you can sort by multiple criteria by providing a tuple to the `key` parameter. For example: `sorted_list = sorted(my_list_of_tuples, key=lambda x: (x[0], x[1]))` sorts first by the first element and then by the second.
What method can I use to sort a list of tuples in place?
You can use the `sort()` method of the list object to sort in place. For example: `my_list_of_tuples.sort(key=lambda x: x[1])` sorts the list by the second element without creating a new list.
Are there any performance considerations when sorting large lists of tuples?
Yes, sorting large lists can be computationally expensive. Python’s built-in sort algorithms are efficient, but for very large datasets, consider using specialized libraries such as NumPy or Pandas for optimized performance.
Sorting a list of tuples in Python can be accomplished effectively using the built-in `sorted()` function or the `sort()` method of a list. Both methods allow for sorting based on the elements of the tuples, either by the first element, second element, or any other specified position. The default behavior sorts tuples by the first element, and if there are ties, it continues to sort by the subsequent elements. This behavior is particularly useful when dealing with multi-dimensional data.
When sorting tuples, it is important to understand how to leverage the `key` parameter to customize the sorting criteria. By providing a function to the `key` parameter, users can sort based on specific elements within the tuples. For instance, sorting by the second element of each tuple can be achieved by using a lambda function. Additionally, the `reverse` parameter can be utilized to sort the list in descending order, offering further flexibility in how the data is organized.
In summary, sorting a list of tuples in Python is straightforward and versatile, accommodating various sorting needs through built-in functionalities. By understanding the use of the `sorted()` function and the `sort()` method, along with the `key` and `reverse` parameters, users can efficiently organize their data
Author Profile
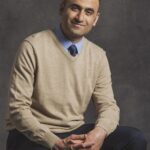
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?