How Can You Effectively Sort an Array in JavaScript?
Sorting an array is a fundamental operation in programming, and in JavaScript, it’s a task that can be both simple and complex, depending on the context and requirements. Whether you’re organizing a list of names, arranging numbers in ascending order, or even sorting objects based on specific properties, mastering array sorting is essential for any developer. In this article, we will explore the various methods available in JavaScript for sorting arrays, the underlying principles that govern these methods, and best practices to ensure your sorting operations are both efficient and effective.
At its core, sorting an array involves rearranging its elements in a specified order, typically ascending or descending. JavaScript provides built-in methods that make this process straightforward, but understanding how these methods work under the hood can significantly enhance your coding skills. From the basic `.sort()` method to more advanced techniques that utilize custom comparator functions, the flexibility of JavaScript allows developers to tailor their sorting logic to fit their specific needs.
As we delve deeper into the topic, we will discuss the nuances of sorting different data types, the impact of sorting on performance, and common pitfalls to avoid. By the end of this article, you will not only be equipped with the knowledge to sort arrays effectively but also gain insights into optimizing your code for better performance in real
Understanding the Sort Method
The `sort()` method in JavaScript is an essential tool for organizing arrays. By default, `sort()` converts elements to strings and sorts them in ascending order based on UTF-16 code unit values. This behavior can lead to unexpected results when sorting numbers or other data types.
To use the `sort()` method, you can call it directly on an array, as shown below:
javascript
let fruits = [‘banana’, ‘apple’, ‘orange’];
fruits.sort(); // [‘apple’, ‘banana’, ‘orange’]
Custom Sorting with a Compare Function
When sorting arrays of numbers or objects, a custom compare function is often necessary. This function takes two arguments and returns:
- A negative value if the first argument is less than the second.
- Zero if they are equal.
- A positive value if the first argument is greater than the second.
Here is an example of sorting an array of numbers in ascending and descending order:
javascript
let numbers = [4, 2, 5, 1, 3];
// Ascending order
numbers.sort((a, b) => a – b); // [1, 2, 3, 4, 5]
// Descending order
numbers.sort((a, b) => b – a); // [5, 4, 3, 2, 1]
Sorting Objects in an Array
When dealing with an array of objects, the compare function can be adapted to sort by specific properties. For example, consider the following array of objects:
javascript
let users = [
{ name: ‘Alice’, age: 25 },
{ name: ‘Bob’, age: 20 },
{ name: ‘Charlie’, age: 30 }
];
To sort by age, the compare function can be defined as follows:
javascript
users.sort((a, b) => a.age – b.age); // Sorts by age in ascending order
Performance Considerations
The performance of the `sort()` method can vary depending on the implementation of the JavaScript engine. Generally, the time complexity is O(n log n), which is efficient for most applications. However, it is essential to keep in mind the following considerations:
- Sorting large arrays can be computationally expensive.
- The stability of the sort may not be guaranteed in all engines.
To benchmark sorting performance, consider using the following table to compare different sorting algorithms:
Algorithm | Time Complexity | Space Complexity | Stable |
---|---|---|---|
Quick Sort | O(n log n) | O(log n) | No |
Merge Sort | O(n log n) | O(n) | Yes |
Bubble Sort | O(n^2) | O(1) | Yes |
The choice of sorting method should be based on the specific requirements of your application, including the need for stability and performance constraints.
Sorting Arrays in JavaScript
JavaScript provides a built-in method, `Array.prototype.sort()`, which can be used to sort arrays in place. By default, this method sorts the elements as strings in ascending order. To sort arrays of numbers or other data types, a compare function must be specified.
Basic Sorting Example
To sort an array of strings or numbers, you can simply call the `sort()` method without any arguments. For instance:
javascript
let fruits = [‘banana’, ‘apple’, ‘cherry’];
fruits.sort();
console.log(fruits); // [‘apple’, ‘banana’, ‘cherry’]
For an array of numbers:
javascript
let numbers = [5, 3, 8, 1];
numbers.sort();
console.log(numbers); // [1, 3, 5, 8] (sorted as strings, not numerically)
Sorting Numbers Correctly
When sorting numbers, you need to provide a compare function to ensure numerical sorting:
javascript
let numbers = [5, 3, 8, 1];
numbers.sort((a, b) => a – b);
console.log(numbers); // [1, 3, 5, 8]
The compare function takes two arguments, `a` and `b`, and returns:
- A negative number if `a` should appear before `b`.
- A positive number if `a` should appear after `b`.
- Zero if they are considered equal.
Sorting in Descending Order
To sort an array in descending order, modify the compare function:
javascript
let numbers = [5, 3, 8, 1];
numbers.sort((a, b) => b – a);
console.log(numbers); // [8, 5, 3, 1]
Sorting Objects in an Array
To sort an array of objects based on a specific property, the compare function can be adjusted accordingly. For example, if you have an array of objects representing people:
javascript
let people = [
{ name: ‘John’, age: 30 },
{ name: ‘Jane’, age: 25 },
{ name: ‘Doe’, age: 20 }
];
people.sort((a, b) => a.age – b.age);
console.log(people);
/*
[
{ name: ‘Doe’, age: 20 },
{ name: ‘Jane’, age: 25 },
{ name: ‘John’, age: 30 }
]
*/
Case-Insensitive Sorting
For string arrays, you may want to sort without considering case. This can be achieved using `localeCompare()`:
javascript
let fruits = [‘banana’, ‘Apple’, ‘cherry’];
fruits.sort((a, b) => a.localeCompare(b, undefined, { sensitivity: ‘base’ }));
console.log(fruits); // [‘Apple’, ‘banana’, ‘cherry’]
Sorting with Custom Logic
You can implement any custom sorting logic by defining your own compare function. For instance, to sort by string length:
javascript
let words = [‘apple’, ‘banana’, ‘kiwi’, ‘grapefruit’];
words.sort((a, b) => a.length – b.length);
console.log(words); // [‘kiwi’, ‘apple’, ‘banana’, ‘grapefruit’]
Utilizing the `sort()` method effectively can greatly enhance your array manipulation capabilities in JavaScript, allowing for both simple and complex sorting operations as needed.
Expert Insights on Sorting Arrays in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When sorting an array in JavaScript, utilizing the built-in `.sort()` method is essential. However, developers must be aware that this method sorts elements as strings by default, which can lead to unexpected results when dealing with numbers. To achieve accurate numerical sorting, a custom comparison function should be implemented.”
Mark Thompson (JavaScript Specialist, CodeMaster Academy). “For optimal performance, especially with large datasets, consider using the `Array.prototype.sort()` method in conjunction with a comparison function that leverages the `localeCompare` method for string arrays. This approach ensures that sorting is not only efficient but also locale-aware, accommodating various character sets.”
Linda Zhang (Lead Frontend Developer, Web Solutions Group). “It is crucial to remember that the `.sort()` method sorts the array in place, meaning the original array is modified. For scenarios where immutability is preferred, developers should create a copy of the array using the spread operator or `Array.from()` before applying the sort method.”
Frequently Asked Questions (FAQs)
How do I sort an array of numbers in ascending order in JavaScript?
To sort an array of numbers in ascending order, use the `sort()` method with a comparison function:
javascript
const numbers = [5, 3, 8, 1];
numbers.sort((a, b) => a – b);
Can I sort an array of strings alphabetically in JavaScript?
Yes, you can sort an array of strings alphabetically by using the `sort()` method without a comparison function, or with one for custom sorting:
javascript
const strings = [‘banana’, ‘apple’, ‘cherry’];
strings.sort();
What is the default behavior of the `sort()` method in JavaScript?
The default behavior of the `sort()` method is to convert elements to strings and sort them in ascending order based on the Unicode code point of each character, which may not yield the expected numerical order.
How can I sort an array of objects by a specific property in JavaScript?
To sort an array of objects by a specific property, use the `sort()` method with a comparison function that accesses the property:
javascript
const items = [{ name: ‘apple’, price: 30 }, { name: ‘banana’, price: 20 }];
items.sort((a, b) => a.price – b.price);
Is it possible to sort an array in descending order in JavaScript?
Yes, you can sort an array in descending order by adjusting the comparison function in the `sort()` method:
javascript
const numbers = [5, 3, 8, 1];
numbers.sort((a, b) => b – a);
What are the performance implications of sorting large arrays in JavaScript?
Sorting large arrays can be computationally intensive, with a time complexity of O(n log n) for most sorting algorithms. Performance may vary based on the implementation of the `sort()` method in different JavaScript engines.
Sorting an array in JavaScript can be accomplished using the built-in `sort()` method, which sorts the elements of an array in place and returns the sorted array. By default, the `sort()` method converts the elements to strings and sorts them in ascending order based on UTF-16 code unit values. This behavior can lead to unexpected results when sorting numbers, as it compares their string representations rather than their numerical values.
To achieve a numerical sort, it is essential to provide a comparison function to the `sort()` method. This function determines the order of the elements by returning a negative, zero, or positive value based on the comparison of two elements. For example, a comparison function that subtracts one number from another will sort the array numerically in ascending order. For descending order, the subtraction can be reversed.
In addition to the basic sorting capabilities, JavaScript also allows for more complex sorting operations. Users can implement custom sorting logic by defining their own comparison functions, which can take into account various criteria such as object properties or specific sorting algorithms. This flexibility makes JavaScript’s array sorting capabilities powerful and adaptable to various use cases.
In summary, understanding how to properly use the `sort()` method and
Author Profile
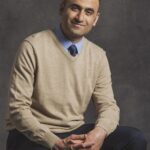
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?