How Can You Easily Square a Number in JavaScript?
Introduction
In the world of programming, mastering the basics is essential for building robust applications, and one of the fundamental operations you’ll encounter is squaring a number. Whether you’re developing a simple calculator, a complex data analysis tool, or a game that relies on mathematical calculations, knowing how to square a number in JavaScript is a skill that can enhance your coding repertoire. This article will guide you through the various methods available in JavaScript for squaring numbers, ensuring you have the tools you need to implement this operation efficiently in your projects.
When working with JavaScript, squaring a number is a straightforward yet crucial task that can be accomplished in several ways. From using the traditional multiplication operator to leveraging built-in functions, each method offers its own advantages and can be applied in different contexts. Understanding these techniques not only improves your coding efficiency but also deepens your grasp of JavaScript’s mathematical capabilities.
As you delve deeper into this topic, you’ll discover not only how to square numbers but also the nuances of each approach. You’ll learn about the performance implications, readability, and best practices that can help you choose the right method for your specific needs. So, whether you’re a beginner looking to solidify your foundational skills or an experienced developer seeking to refine your techniques, this exploration of
Methods to Square a Number
In JavaScript, there are several efficient methods to square a number. Each method varies in syntax and usage, allowing developers to choose based on their specific needs or preferences.
Using the Multiplication Operator
The most straightforward way to square a number in JavaScript is by using the multiplication operator (`*`). This method is intuitive and widely recognized among developers.
javascript
let number = 5;
let squared = number * number; // 25
This approach is effective for squaring any numeric value, including integers and floating-point numbers.
Using the Math.pow() Function
Another common method is to use the built-in `Math.pow()` function, which raises a number to a specified power. To square a number, you can pass the number and the exponent `2` as arguments.
javascript
let number = 5;
let squared = Math.pow(number, 2); // 25
This method is particularly useful for squaring numbers within more complex calculations or when working with exponentiation in general.
Using the Exponentiation Operator
Introduced in ECMAScript 2016 (ES6), the exponentiation operator (`**`) provides a concise syntax for exponentiation. To square a number, you can use it as follows:
javascript
let number = 5;
let squared = number ** 2; // 25
This operator is more modern and can improve readability, especially in mathematical expressions.
Comparison of Methods
The following table summarizes the three methods to square a number in JavaScript, highlighting their syntax and ease of use.
Method | Syntax | Ease of Use |
---|---|---|
Multiplication | number * number | Simple and intuitive |
Math.pow() | Math.pow(number, 2) | Standard library function |
Exponentiation Operator | number ** 2 | Modern and concise |
Choosing the Right Method
When deciding which method to use for squaring a number, consider the following factors:
- Readability: The exponentiation operator (`**`) may improve readability in complex expressions.
- Compatibility: If you’re working in an environment that doesn’t support ES6, the multiplication method or `Math.pow()` may be preferable.
- Performance: All methods are efficient, but the multiplication operator is the most straightforward in terms of performance and clarity.
Each method has its advantages, and the choice largely depends on the context in which you’re working and personal coding style.
Squaring a Number Using the Exponentiation Operator
In JavaScript, the simplest way to square a number is by using the exponentiation operator (`**`). This operator allows you to raise a base to the power of an exponent. To square a number, you raise it to the power of 2.
javascript
let number = 5;
let squared = number ** 2;
console.log(squared); // Output: 25
Using the Math.pow() Method
Another method for squaring a number in JavaScript is through the `Math.pow()` function. This built-in function takes two arguments: the base and the exponent. To square a number, you can pass the number as the first argument and `2` as the second.
javascript
let number = 5;
let squared = Math.pow(number, 2);
console.log(squared); // Output: 25
Multiplication Method
A more straightforward approach to squaring a number involves simple multiplication. You can multiply the number by itself, which is clear and efficient.
javascript
let number = 5;
let squared = number * number;
console.log(squared); // Output: 25
Using Arrow Functions for Reusability
To enhance code reusability, you can define a function using an arrow function syntax. This function can take any number as an argument and return its square.
javascript
const square = (num) => num ** 2;
console.log(square(5)); // Output: 25
console.log(square(10)); // Output: 100
Performance Considerations
When choosing a method to square a number, consider the following factors:
– **Simplicity**: The multiplication method is straightforward and easy to read.
– **Readability**: The exponentiation operator enhances clarity, especially in mathematical contexts.
– **Performance**: All methods are efficient for squaring numbers, with negligible differences for typical use cases.
Method | Syntax | Advantages |
---|---|---|
Exponentiation Operator | `number ** 2` | Clear and concise |
Math.pow() | `Math.pow(number, 2)` | Familiar for those with math background |
Multiplication | `number * number` | Simple and intuitive |
Arrow Function | `(num) => num ** 2` | Reusable and clean |
In JavaScript, squaring a number can be accomplished through various methods, including the exponentiation operator, the `Math.pow()` function, or simple multiplication. Each method has its unique advantages and can be chosen based on the context of use and personal preference.
Expert Insights on Squaring Numbers in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Squaring a number in JavaScript is straightforward and can be accomplished using the exponentiation operator ‘**’ or by simply multiplying the number by itself. This flexibility allows developers to choose the method that best fits their coding style.”
Michael Chen (JavaScript Developer Advocate, CodeCraft). “Utilizing the Math.pow() function is another effective way to square a number in JavaScript. This method not only enhances code readability but also aligns with best practices for mathematical operations in programming.”
Sarah Thompson (Lead Frontend Developer, Creative Solutions). “When squaring numbers in JavaScript, performance considerations may arise in large-scale applications. Using the multiplication method is generally faster than Math.pow(), making it a preferred choice for performance-critical code.”
Frequently Asked Questions (FAQs)
How do I square a number in JavaScript?
You can square a number in JavaScript by multiplying the number by itself, for example: `let squared = number * number;`.
Is there a built-in method to square a number in JavaScript?
JavaScript does not have a specific built-in method for squaring numbers, but you can use the exponentiation operator ``, as in `let squared = number 2;`.
Can I use the Math.pow function to square a number?
Yes, you can use the `Math.pow` function to square a number by calling it with the base as the number and the exponent as 2, like this: `let squared = Math.pow(number, 2);`.
What is the difference between using `*` and `Math.pow` for squaring?
Using `*` is more straightforward and typically faster for squaring a number, while `Math.pow` is more versatile for raising numbers to any power.
Can I square a number stored in a variable?
Yes, you can square a number stored in a variable by applying any of the methods mentioned, such as `let squared = variable * variable;`.
Is there a performance difference between the methods for squaring a number?
In general, using the multiplication operator is faster than using `Math.pow`, especially for squaring, due to the simplicity of the operation.
Squaring a number in JavaScript is a straightforward process that can be accomplished using various methods. The most common approach is to use the exponentiation operator (``), which allows you to raise a number to a specific power. For instance, to square a number, you can simply use `number 2`. Alternatively, you can achieve the same result by multiplying the number by itself, such as `number * number`. Both methods are efficient and widely used in JavaScript programming.
Another method to square a number is by utilizing the `Math.pow()` function, which takes two arguments: the base and the exponent. For squaring, you would call `Math.pow(number, 2)`. While this method is slightly more verbose than the other two, it is still a valid and effective way to perform the operation. Each of these techniques provides flexibility depending on the context in which you are working.
In summary, JavaScript offers multiple ways to square a number, including the exponentiation operator, direct multiplication, and the `Math.pow()` function. Understanding these options allows developers to choose the most appropriate method for their specific needs, whether for simplicity, readability, or compatibility with older JavaScript versions. Overall, squaring
Author Profile
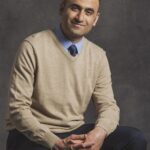
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?