How Can You Squash All Commits in a Branch Efficiently?
In the world of version control, particularly when using Git, the ability to manage your commit history is crucial for maintaining a clean and understandable project narrative. One powerful technique that developers often turn to is squashing commits. But what does it mean to squash commits, and why is it important? Whether you’re looking to streamline your project’s history before merging a feature branch or simply want to tidy up your commit log, understanding how to squash all commits in a branch can significantly enhance your workflow. In this article, we will explore the ins and outs of this essential Git operation, empowering you to take control of your version history like never before.
When you squash commits, you essentially combine multiple commit entries into a single, cohesive commit. This not only simplifies the commit history but also makes it easier for collaborators to follow the evolution of your project. Imagine a scenario where you’ve made numerous small, incremental changes—each one a separate commit. By squashing these commits, you can present a cleaner, more meaningful snapshot of your work that highlights the key features or fixes without the clutter of minor adjustments.
Moreover, squashing commits is not just about aesthetics; it can also help in reducing merge conflicts and improving the overall efficiency of your Git operations. As you delve deeper into the
Understanding Git Rebase
To squash all commits in a branch, it’s essential to understand the concept of Git rebase. Rebasing allows you to integrate changes from one branch into another, effectively rewriting commit history. This is particularly useful when you want to combine multiple commits into a single commit for clarity and simplicity.
When performing a rebase, Git allows you to interactively edit the commit history, providing an opportunity to squash commits. Squashing refers to merging multiple commit entries into one, helping to create a more streamlined and comprehensible commit history.
Steps to Squash Commits
To squash all commits in a branch, follow these steps:
- Checkout to the Target Branch:
Begin by checking out the branch where you want to squash the commits.
“`bash
git checkout your-branch-name
“`
- Initiate Interactive Rebase:
Use the interactive rebase command to start squashing. If you want to squash all commits in the branch, you’ll need to reference the parent of the first commit you want to squash. This is often done with `HEAD~n`, where `n` is the number of commits you wish to squash.
“`bash
git rebase -i HEAD~n
“`
- Modify the Rebase Instructions:
In the text editor that opens, you’ll see a list of commits. Change the word `pick` to `squash` (or simply `s`) for all commits you want to combine into the first commit. The first commit should remain as `pick`.
For example:
“`
pick 1234567 First commit message
squash 89abcde Second commit message
squash fghijkl Third commit message
“`
- Save and Exit:
After making your changes, save the file and exit the editor. Git will then proceed with the rebase and combine the specified commits.
- Edit the Commit Message:
A new editor will appear, allowing you to edit the commit message for the squashed commit. You can combine messages or write a new one that summarizes the changes.
- Finalize the Rebase:
Save your changes and exit the editor to complete the rebase. If there are any conflicts, Git will prompt you to resolve them before proceeding.
Potential Issues and Resolutions
When squashing commits, you might encounter various issues. Here are common problems and their solutions:
Issue | Resolution |
---|---|
Merge Conflicts | Resolve conflicts manually and use `git rebase –continue` to proceed. |
Uncommitted Changes | Ensure all changes are committed before starting the rebase. |
Incorrect Commit History | If you squash incorrectly, you can abort the rebase with `git rebase –abort`. |
Best Practices
When squashing commits, consider the following best practices to maintain a clean commit history:
- Squash Related Commits: Group commits that relate to the same feature or fix.
- Use Descriptive Commit Messages: Ensure that the final commit message accurately reflects the changes made.
- Avoid Squashing Public Commits: If other collaborators have based work on the commits you intend to squash, it may lead to complications in the shared repository.
By following these steps and guidelines, you can effectively squash all commits in a branch, creating a more concise and manageable commit history.
Understanding Git Squashing
Squashing commits in Git allows you to condense multiple commits into a single commit. This is particularly useful for cleaning up your commit history before merging a feature branch into the main branch. It can help maintain a tidy project history by making it easier to understand the changes made.
Prerequisites for Squashing Commits
Before proceeding with squashing commits, ensure that you have the following:
- A branch that contains multiple commits you wish to squash.
- A clean working directory, meaning no uncommitted changes.
- Familiarity with Git commands and terminal usage.
Steps to Squash All Commits in a Branch
To squash all commits in a branch, follow these steps:
- Checkout to the Branch
Use the following command to switch to the branch containing the commits you want to squash:
“`bash
git checkout your-branch-name
“`
- Identify the Base Commit
Determine the base commit from which you want to start squashing. This is typically the commit just before the first commit you want to include. You can find the commit hash using:
“`bash
git log –oneline
“`
- Start an Interactive Rebase
Initiate an interactive rebase using the commit hash of the base commit:
“`bash
git rebase -i base-commit-hash
“`
- Modify the Rebase List
An editor will open showing a list of commits. Change the word `pick` to `squash` (or `s`) for all commits you wish to squash into the first one. Only the first commit should remain as `pick`. For example:
“`
pick 1234567 Commit message 1
squash 2345678 Commit message 2
squash 3456789 Commit message 3
“`
- Save and Exit the Editor
After making the necessary changes, save the file and exit the editor. This will trigger the squashing process.
- Edit the Commit Message
A new editor window will open for you to combine the commit messages. Edit this to create a single cohesive message for the squashed commit.
- Finalize the Rebase
Save and exit the editor again to finalize the rebase. If there are conflicts, resolve them, and use:
“`bash
git rebase –continue
“`
Verifying Squashed Commits
After successfully squashing commits, you can verify the changes by checking the commit history:
“`bash
git log –oneline
“`
You should see that the commits have been successfully squashed into a single commit with the new commit message.
Handling Potential Issues
While squashing commits is straightforward, you may encounter issues:
- Merge Conflicts: If there are conflicts during the rebase, Git will pause and allow you to resolve them. After resolving, use `git rebase –continue`.
- Lost Commits: If you accidentally squash commits you didn’t intend to, use `git reflog` to find the previous state of your branch and reset to that commit.
Alternative: Using the `–squash` Flag
An alternative approach to squashing is to use the `–squash` flag when merging. This method allows you to create a single commit from all changes in a branch without modifying the commit history directly:
“`bash
git merge –squash your-branch-name
“`
This command combines the changes into your current branch, allowing you to create a single new commit, but without retaining the individual commit history from the squashed branch.
Expert Insights on Squashing Commits in Git Branches
Dr. Emily Chen (Senior Software Engineer, CodeCraft Solutions). “Squashing commits is an essential practice for maintaining a clean project history. It allows developers to consolidate multiple changes into a single commit, making it easier to understand the evolution of the codebase and facilitating smoother code reviews.”
Mark Thompson (Git Specialist, DevOps Insights). “To squash all commits in a branch, utilize the interactive rebase feature in Git. This method not only simplifies your commit history but also enables you to edit commit messages, ensuring clarity and context for future reference.”
Lisa Patel (Technical Writer, Open Source Journal). “When squashing commits, it is crucial to communicate with your team. This practice can alter the commit history, so ensuring everyone is on the same page will prevent confusion and potential conflicts in collaborative projects.”
Frequently Asked Questions (FAQs)
What does it mean to squash commits in Git?
Squashing commits in Git refers to the process of combining multiple commit entries into a single commit. This is often done to simplify the commit history and make it easier to understand.
How can I squash all commits in a branch?
To squash all commits in a branch, use the command `git rebase -i HEAD~n`, where `n` is the number of commits you want to squash. In the interactive rebase interface, change the word “pick” to “squash” for all but the first commit, then save and exit.
What happens to the commit history after squashing?
After squashing commits, the commit history will reflect a single commit that represents all the changes made in the squashed commits. This can help in maintaining a cleaner project history.
Is it safe to squash commits that have been pushed to a remote repository?
Squashing commits that have already been pushed to a remote repository is generally not recommended unless you are sure that no one else is working with those commits. It can lead to conflicts and confusion for collaborators.
Can I squash commits using a graphical interface?
Yes, many Git graphical user interfaces (GUIs) provide options to squash commits. Look for features related to interactive rebasing or commit history management in your chosen GUI.
What should I do if I encounter conflicts while squashing commits?
If you encounter conflicts during the squashing process, resolve the conflicts in your files, stage the changes with `git add`, and then continue the rebase process using `git rebase –continue`.
Squashing all commits in a branch is a powerful technique in Git that allows developers to condense multiple commit entries into a single, cohesive commit. This practice is particularly useful for maintaining a clean and organized project history, especially when preparing to merge a feature branch into the main branch. By squashing commits, developers can eliminate unnecessary or redundant commit messages, making the project history easier to read and understand.
The process of squashing commits typically involves using the interactive rebase feature in Git. By executing the command `git rebase -i HEAD~n`, where ‘n’ represents the number of commits you wish to squash, developers can enter an interactive mode that allows them to choose which commits to combine. This method not only simplifies the commit history but also provides an opportunity to edit the commit message, ensuring that it accurately reflects the changes made in the squashed commits.
mastering the technique of squashing commits is essential for developers looking to enhance their workflow and maintain a clean project history. It is a valuable skill that contributes to better collaboration among team members and improves the overall quality of the codebase. By regularly applying this practice, developers can ensure that their contributions are well-documented and easily navigable for future reference.
Author Profile
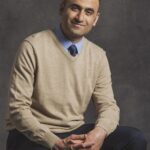
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?