How Can You Start a New Line in Python?
When diving into the world of Python programming, one of the fundamental skills you’ll encounter is the ability to manage output formatting. Among these formatting techniques, knowing how to start a new line in Python is essential for creating clear, organized, and readable code. Whether you’re printing messages to the console or generating text files, mastering this simple yet powerful concept can significantly enhance your programming experience and the usability of your applications.
In Python, starting a new line is not just about aesthetics; it plays a crucial role in how information is presented to users. By understanding the various methods available for line breaks, you can control the flow of your output, making it more intuitive and user-friendly. This knowledge is particularly useful when working with loops, conditional statements, or when displaying multi-line strings.
As you explore the different techniques to insert new lines in your Python scripts, you’ll discover the versatility of string manipulation and the importance of formatting in effective communication through code. From using escape characters to leveraging built-in functions, each approach offers unique advantages that can elevate your coding skills and improve the clarity of your output. Get ready to unravel the straightforward yet impactful ways to enhance your Python programming with the simple act of starting a new line!
Using the Print Function
In Python, one of the simplest ways to start a new line is by using the `print()` function. By default, the `print()` function adds a newline character at the end of its output. This means that subsequent calls to `print()` will start on a new line.
Example:
“`python
print(“Hello, World!”)
print(“This is a new line.”)
“`
Output:
“`
Hello, World!
This is a new line.
“`
Inserting Newline Characters
Another method to create a new line is by explicitly inserting a newline character `\n` in a string. This can be particularly useful when you are constructing strings that need to include line breaks.
Example:
“`python
text = “Hello, World!\nThis is on a new line.”
print(text)
“`
Output:
“`
Hello, World!
This is on a new line.
“`
Using Triple Quotes
For multi-line strings, Python offers triple quotes (either `”’` or `”””`). This allows you to span text across multiple lines without needing to add newline characters manually.
Example:
“`python
text = “””Hello, World!
This is a new line.
And here is another one.”””
print(text)
“`
Output:
“`
Hello, World!
This is a new line.
And here is another one.
“`
Formatting Strings with Newlines
Python’s string formatting methods also allow for inserting new lines. For instance, using f-strings or the `format()` method can help structure output with multiple lines.
Example using f-strings:
“`python
name = “John”
greeting = f”Hello, {name}!\nWelcome to Python programming.”
print(greeting)
“`
Output:
“`
Hello, John!
Welcome to Python programming.
“`
Table of Methods to Start a New Line
Method | Description |
---|---|
print() | Automatically adds a newline after each print call. |
Newline Character (\n) | Explicitly adds a newline within a string. |
Triple Quotes | Allows multi-line strings without additional newline characters. |
String Formatting | Incorporates newlines in formatted strings using f-strings or format(). |
Utilizing these methods, you can effectively manage line breaks in your Python programs, enhancing readability and organization of your output.
Using the `print()` Function
In Python, the most common method to start a new line in output is by using the `print()` function. By default, the `print()` function ends with a newline character, which means each call to `print()` will automatically move to the next line.
Example:
“`python
print(“Hello, World!”)
print(“This will be on a new line.”)
“`
Output:
“`
Hello, World!
This will be on a new line.
“`
Using Escape Sequences
Another way to start a new line within a single string is by using the newline escape sequence, `\n`. This can be particularly useful when constructing longer strings that require multiple lines.
Example:
“`python
message = “Hello, World!\nWelcome to Python programming.”
print(message)
“`
Output:
“`
Hello, World!
Welcome to Python programming.
“`
Using Multiline Strings
For longer text blocks or when you want to maintain formatting, Python allows the use of multiline strings, which are defined using triple quotes (`”’` or `”””`). This approach preserves line breaks as they are written.
Example:
“`python
multiline_message = “””Hello, World!
Welcome to Python programming.
Enjoy coding!”””
print(multiline_message)
“`
Output:
“`
Hello, World!
Welcome to Python programming.
Enjoy coding!
“`
Joining Strings with Newlines
You may also join multiple strings with the newline character using the `join()` method. This is particularly useful for creating formatted output from a list of strings.
Example:
“`python
lines = [“Line 1”, “Line 2”, “Line 3”]
output = “\n”.join(lines)
print(output)
“`
Output:
“`
Line 1
Line 2
Line 3
“`
Writing to Files with New Lines
When working with files, you can write strings containing newlines directly to a file. Each `write()` call does not automatically add a newline, so it’s essential to include `\n` if desired.
Example:
“`python
with open(‘output.txt’, ‘w’) as file:
file.write(“Hello, World!\n”)
file.write(“This is a new line.\n”)
“`
The resulting `output.txt` will contain:
“`
Hello, World!
This is a new line.
“`
Using `print()` with Custom End Parameter
The `print()` function also allows customization of the end character. To use a different character or to prevent moving to a new line, set the `end` parameter.
Example:
“`python
print(“Hello, “, end=””)
print(“World!”)
“`
Output:
“`
Hello, World!
“`
By utilizing these methods, you can effectively manage line breaks in your Python programs, ensuring clarity and readability in both console output and file writing.
Expert Insights on Starting a New Line in Python
Dr. Emily Chen (Senior Software Engineer, CodeCraft Solutions). “To start a new line in Python, the most common method is using the newline character, represented as ‘\n’. This character can be included in strings to indicate where the line break should occur, making it essential for formatting output in console applications.”
Michael Thompson (Python Educator, LearnPythonToday). “When teaching beginners how to start a new line in Python, I emphasize the importance of the print function’s ‘end’ parameter. By default, print adds a newline after each call, but you can customize this behavior by setting ‘end’ to an empty string or any other character, allowing for more control over the output.”
Sarah Patel (Lead Developer, Tech Innovations Inc.). “In addition to using the newline character, it’s important for developers to understand how different environments handle line breaks. For example, while ‘\n’ works universally, Windows systems may require ‘\r\n’ for proper line breaks in certain contexts, especially when writing to files.”
Frequently Asked Questions (FAQs)
How do I start a new line in Python using print statements?
You can start a new line in Python using the `print()` function by including the newline character `\n` within the string. For example, `print(“Hello\nWorld”)` will output:
“`
Hello
World
“`
Can I use triple quotes to create a new line in Python?
Yes, you can use triple quotes (either `”’` or `”””`) to create multi-line strings, which automatically include new lines. For example:
“`python
print(“””Hello
World”””)
“`
What is the purpose of the end parameter in the print function?
The `end` parameter in the `print()` function allows you to specify what to print at the end of the output. By default, it is set to `\n`, but you can change it to an empty string or any other character to control line breaks. For example:
“`python
print(“Hello”, end=” “)
print(“World”)
“`
How can I start a new line in a string variable?
You can include the newline character `\n` directly within the string assigned to a variable. For example:
“`python
my_string = “Hello\nWorld”
print(my_string)
“`
Is there a way to use a raw string to prevent new line characters from being processed?
Yes, you can use raw strings by prefixing the string with an `r`, which prevents escape sequences from being processed. For example:
“`python
raw_string = r”Hello\nWorld”
print(raw_string)
“`
Can I use the newline character in formatted strings?
Yes, you can use the newline character `\n` in formatted strings, such as f-strings. For example:
“`python
name = “World”
print(f”Hello\n{name}”)
“`
In Python, starting a new line can be achieved through various methods, depending on the context of the code. The most straightforward way to create a new line in a string is by using the newline character, represented as `\n`. This character can be included within string literals to indicate where the line break should occur. For example, using `print(“Hello\nWorld”)` will output “Hello” on one line and “World” on the next.
Another method to start a new line is by utilizing the `print()` function’s `end` parameter. By default, the `print()` function ends with a newline, but this can be customized. For instance, `print(“Hello”, end=”\n”)` explicitly specifies a newline, while `print(“Hello”, end=” “)` would keep the output on the same line. This flexibility allows for greater control over output formatting.
In addition to these methods, when working with multi-line strings, Python provides triple quotes (`”’` or `”””`), which allow strings to span multiple lines naturally. This feature is particularly useful for longer text blocks or documentation strings, making the code more readable and organized.
Overall, understanding how to start a new
Author Profile
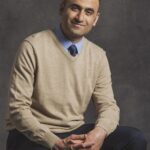
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?